Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / CompMod / System / ComponentModel / CategoryAttribute.cs / 1 / CategoryAttribute.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.ComponentModel { using System; using System.ComponentModel; using System.Diagnostics; using System.Security.Permissions; ////// [AttributeUsage(AttributeTargets.All)] public class CategoryAttribute : Attribute { private static CategoryAttribute appearance; private static CategoryAttribute asynchronous; private static CategoryAttribute behavior; private static CategoryAttribute data; private static CategoryAttribute design; private static CategoryAttribute action; private static CategoryAttribute format; private static CategoryAttribute layout; private static CategoryAttribute mouse; private static CategoryAttribute key; private static CategoryAttribute focus; private static CategoryAttribute windowStyle; private static CategoryAttribute dragDrop; private static CategoryAttribute defAttr; private bool localized; ///Specifies the category in which the property or event will be displayed in a /// visual designer. ////// private string categoryValue; ////// Provides the actual category name. /// ////// public static CategoryAttribute Action { get { if (action == null) { action = new CategoryAttribute("Action"); } return action; } } ///Gets the action category attribute. ////// public static CategoryAttribute Appearance { get { if (appearance == null) { appearance = new CategoryAttribute("Appearance"); } return appearance; } } ///Gets the appearance category attribute. ////// public static CategoryAttribute Asynchronous { get { if (asynchronous == null) { asynchronous = new CategoryAttribute("Asynchronous"); } return asynchronous; } } ///Gets the asynchronous category attribute. ////// public static CategoryAttribute Behavior { get { if (behavior == null) { behavior = new CategoryAttribute("Behavior"); } return behavior; } } ///Gets the behavior category attribute. ////// public static CategoryAttribute Data { get { if (data == null) { data = new CategoryAttribute("Data"); } return data; } } ///Gets the data category attribute. ////// public static CategoryAttribute Default { get { if (defAttr == null) { defAttr = new CategoryAttribute(); } return defAttr; } } ///Gets the default category attribute. ////// public static CategoryAttribute Design { get { if (design == null) { design = new CategoryAttribute("Design"); } return design; } } ///Gets the design category attribute. ////// public static CategoryAttribute DragDrop { get { if (dragDrop == null) { dragDrop = new CategoryAttribute("DragDrop"); } return dragDrop; } } ///Gets the drag and drop category attribute. ////// public static CategoryAttribute Focus { get { if (focus == null) { focus = new CategoryAttribute("Focus"); } return focus; } } ///Gets the focus category attribute. ////// public static CategoryAttribute Format { get { if (format == null) { format = new CategoryAttribute("Format"); } return format; } } ///Gets the format category attribute. ////// public static CategoryAttribute Key { get { if (key == null) { key = new CategoryAttribute("Key"); } return key; } } ///Gets the keyboard category attribute. ////// public static CategoryAttribute Layout { get { if (layout == null) { layout = new CategoryAttribute("Layout"); } return layout; } } ///Gets the layout category attribute. ////// public static CategoryAttribute Mouse { get { if (mouse == null) { mouse = new CategoryAttribute("Mouse"); } return mouse; } } ///Gets the mouse category attribute. ////// public static CategoryAttribute WindowStyle { get { if (windowStyle == null) { windowStyle = new CategoryAttribute("WindowStyle"); } return windowStyle; } } ///Gets the window style category /// attribute. ////// public CategoryAttribute() : this("Default") { } ///Initializes a new instance of the ////// class with the default category. /// public CategoryAttribute(string category) { this.categoryValue = category; this.localized = false; } ///Initializes a new instance of the ///class with /// the specified category name. /// public string Category { get { if (!localized) { localized = true; string localizedValue = GetLocalizedString(categoryValue); if (localizedValue != null) { categoryValue = localizedValue; } } return categoryValue; } } ///Gets the name of the category for the property or event /// that this attribute is bound to. ////// ////// ////// public override bool Equals(object obj){ if (obj == this) { return true; } if (obj is CategoryAttribute){ return Category.Equals(((CategoryAttribute)obj).Category); } return false; } /// /// public override int GetHashCode() { return Category.GetHashCode(); } ///[To be supplied.] ////// protected virtual string GetLocalizedString(string value) { return (string)SR.GetObject("PropertyCategory" + value); } ///Looks up the localized name of a given category. ////// ////// ////// public override bool IsDefaultAttribute() { return Category.Equals(Default.Category); } } }
Link Menu
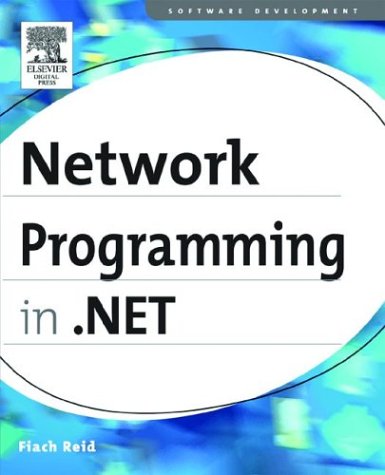
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TrackingMemoryStreamFactory.cs
- BindingExpression.cs
- TrackingAnnotationCollection.cs
- CngKeyCreationParameters.cs
- URI.cs
- UseAttributeSetsAction.cs
- SecurityPermission.cs
- FtpCachePolicyElement.cs
- CodeBinaryOperatorExpression.cs
- MeasureItemEvent.cs
- ReflectionPermission.cs
- ProfessionalColorTable.cs
- RuleRefElement.cs
- FormViewInsertEventArgs.cs
- X509CertificateRecipientClientCredential.cs
- FormViewInsertedEventArgs.cs
- DateTimeConstantAttribute.cs
- KeyGestureConverter.cs
- UTF8Encoding.cs
- TextEncodedRawTextWriter.cs
- FontFamily.cs
- CompositeCollectionView.cs
- PageWrapper.cs
- XmlDictionaryReader.cs
- TableLayoutStyle.cs
- ChangeToolStripParentVerb.cs
- base64Transforms.cs
- QilTargetType.cs
- ConfigurationSettings.cs
- ZeroOpNode.cs
- WpfPayload.cs
- HighlightComponent.cs
- DataGridCommandEventArgs.cs
- DbXmlEnabledProviderManifest.cs
- SqlEnums.cs
- StickyNoteAnnotations.cs
- SslStreamSecurityBindingElement.cs
- ToolStripStatusLabel.cs
- DiagnosticTrace.cs
- SafeMILHandleMemoryPressure.cs
- VisualCollection.cs
- SimpleLine.cs
- RenderData.cs
- JsonSerializer.cs
- ConfigXmlCDataSection.cs
- SQLInt16Storage.cs
- XmlSerializerObjectSerializer.cs
- RSAOAEPKeyExchangeDeformatter.cs
- WebPartsSection.cs
- VSDExceptions.cs
- NavigationHelper.cs
- SimplePropertyEntry.cs
- BitmapCache.cs
- CheckBoxStandardAdapter.cs
- VBCodeProvider.cs
- SoapSchemaImporter.cs
- ClientSettingsStore.cs
- LogReservationCollection.cs
- ShaderEffect.cs
- RpcResponse.cs
- SweepDirectionValidation.cs
- Baml2006KeyRecord.cs
- DetailsViewUpdatedEventArgs.cs
- Animatable.cs
- ComponentEditorPage.cs
- ListBox.cs
- ClientSession.cs
- BidPrivateBase.cs
- StringValueConverter.cs
- ToolBarOverflowPanel.cs
- XmlAttributes.cs
- SpeechSeg.cs
- ObjectSelectorEditor.cs
- OrderByBuilder.cs
- serverconfig.cs
- WithStatement.cs
- BaseParaClient.cs
- DeferredBinaryDeserializerExtension.cs
- TypeRefElement.cs
- ConfigurationPropertyCollection.cs
- RNGCryptoServiceProvider.cs
- RootBrowserWindowAutomationPeer.cs
- ConsumerConnectionPointCollection.cs
- RequiredAttributeAttribute.cs
- SocketPermission.cs
- MetadataArtifactLoaderCompositeResource.cs
- DataGridColumnHeader.cs
- HttpHeaderCollection.cs
- EntityDataSourceStatementEditor.cs
- DataGridViewCellPaintingEventArgs.cs
- StoreAnnotationsMap.cs
- RequestFactory.cs
- DbConnectionStringCommon.cs
- _SafeNetHandles.cs
- ControlPaint.cs
- InputScopeManager.cs
- ListBindingHelper.cs
- PolicyValidationException.cs
- StoreContentChangedEventArgs.cs
- UncommonField.cs