Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WinForms / System / WinForms / Design / ToolStripInSituService.cs / 1 / ToolStripInSituService.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms.Design { using System.Design; using System.Runtime.InteropServices; using System.ComponentModel; using System.Diagnostics; using System; using System.Collections; using Microsoft.Win32; using System.ComponentModel.Design; using System.Drawing; using System.Windows.Forms; using System.Windows.Forms.ComponentModel; using System.Windows.Forms.Design; using System.Windows.Forms.Design.Behavior; ////// /// This class implements the ISupportInSituService which enables some designers to /// go into InSitu Editing when Keys are pressed while the Component is Selected. /// internal class ToolStripInSituService : ISupportInSituService, IDisposable{ private IServiceProvider sp; private IDesignerHost designerHost; private IComponentChangeService componentChangeSvc; private ToolStripDesigner toolDesigner; private ToolStripItemDesigner toolItemDesigner; private ToolStripKeyboardHandlingService toolStripKeyBoardService; ////// /// The constructor for this class which takes the serviceprovider used to get the selectionservice. /// This ToolStripInSituService is ToolStrip specific. /// public ToolStripInSituService(IServiceProvider provider) { this.sp = provider; designerHost = (IDesignerHost)provider.GetService(typeof(IDesignerHost)); Debug.Assert(designerHost != null, "ToolStripKeyboardHandlingService relies on the selection service, which is unavailable."); if (designerHost != null) { designerHost.AddService(typeof(ISupportInSituService), this); } componentChangeSvc = (IComponentChangeService)designerHost.GetService(typeof(IComponentChangeService)); Debug.Assert(componentChangeSvc != null, "ToolStripKeyboardHandlingService relies on the componentChange service, which is unavailable."); if (componentChangeSvc != null) { componentChangeSvc.ComponentRemoved += new ComponentEventHandler(OnComponentRemoved); } } ////// /// Disposes of this object, removing all commands from the menu service. /// public void Dispose() { if (toolDesigner != null) { toolDesigner.Dispose(); toolDesigner = null; } if (toolItemDesigner != null) { toolItemDesigner.Dispose(); toolItemDesigner = null; } if (componentChangeSvc != null) { componentChangeSvc.ComponentRemoved -= new ComponentEventHandler(OnComponentRemoved); componentChangeSvc = null; } } private ToolStripKeyboardHandlingService ToolStripKeyBoardService { get { if (toolStripKeyBoardService == null) { toolStripKeyBoardService = (ToolStripKeyboardHandlingService)sp.GetService(typeof(ToolStripKeyboardHandlingService)); } return toolStripKeyBoardService; } } ////// /// Returning true for IgnoreMessages means that this service is interested in getting the KeyBoard characters. /// public bool IgnoreMessages { get { ISelectionService selectionService = (ISelectionService)sp.GetService(typeof(ISelectionService)); IDesignerHost host = (IDesignerHost)sp.GetService(typeof(IDesignerHost)); if (selectionService != null && host != null) { IComponent comp = selectionService.PrimarySelection as IComponent; if (comp == null) { comp = (IComponent)ToolStripKeyBoardService.SelectedDesignerControl; } if (comp != null) { DesignerToolStripControlHost c = comp as DesignerToolStripControlHost; if (c != null) { ToolStripDropDown dropDown = c.GetCurrentParent() as ToolStripDropDown; if (dropDown != null) { ToolStripDropDownItem parentItem = dropDown.OwnerItem as ToolStripDropDownItem; if (parentItem != null) { ToolStripOverflowButton parent = parentItem as ToolStripOverflowButton; if (parent != null) { //return false ... We are on overflow.. return false; } else { toolItemDesigner = host.GetDesigner(parentItem) as ToolStripMenuItemDesigner; if (toolItemDesigner != null) { toolDesigner = null; return true; } } } } else { MenuStrip tool = c.GetCurrentParent() as MenuStrip; if (tool != null) { toolDesigner = host.GetDesigner(tool) as ToolStripDesigner; if (toolDesigner != null) { toolItemDesigner = null; return true; } } } } else if (comp is ToolStripDropDown) //case for ToolStripDropDown.. { ToolStripDropDownDesigner designer = host.GetDesigner(comp) as ToolStripDropDownDesigner; if (designer != null) { ToolStripMenuItem toolItem = designer.DesignerMenuItem; if (toolItem != null) { toolItemDesigner = host.GetDesigner(toolItem) as ToolStripItemDesigner; if (toolItemDesigner != null) { toolDesigner = null; return true; } } } } else if (comp is MenuStrip) { toolDesigner = host.GetDesigner(comp) as ToolStripDesigner; if (toolDesigner != null) { toolItemDesigner = null; return true; } } else if (comp is ToolStripMenuItem){ toolItemDesigner = host.GetDesigner(comp) as ToolStripItemDesigner; if (toolItemDesigner != null) { toolDesigner = null; return true; } } } } return false; } } ////// /// This function is called on the service when the PBRSFORWARD gets the first WM_CHAR message. /// public void HandleKeyChar() { if (toolDesigner != null || toolItemDesigner != null) { if (toolDesigner != null) { toolDesigner.ShowEditNode(false); } else if (toolItemDesigner != null) { ToolStripMenuItemDesigner menuDesigner = toolItemDesigner as ToolStripMenuItemDesigner; if (menuDesigner != null) { ISelectionService selService = (ISelectionService)sp.GetService(typeof(ISelectionService)); if (selService != null) { object comp = selService.PrimarySelection; if (comp == null) { comp = ToolStripKeyBoardService.SelectedDesignerControl; } DesignerToolStripControlHost designerItem = comp as DesignerToolStripControlHost; if (designerItem != null || comp is ToolStripDropDown) { menuDesigner.EditTemplateNode(false); } else { menuDesigner.ShowEditNode(false); } } } else { toolItemDesigner.ShowEditNode(false); } } } } ////// /// This function returns the Window handle that should get all the Keyboard messages. /// public IntPtr GetEditWindow() { IntPtr hWnd = IntPtr.Zero; if (toolDesigner != null && toolDesigner.Editor != null && toolDesigner.Editor.EditBox != null) { hWnd = (toolDesigner.Editor.EditBox.Visible) ? toolDesigner.Editor.EditBox.Handle : hWnd; } else if (toolItemDesigner != null && toolItemDesigner.Editor != null && toolItemDesigner.Editor.EditBox != null){ hWnd = (toolItemDesigner.Editor.EditBox.Visible) ? toolItemDesigner.Editor.EditBox.Handle : hWnd; } return hWnd; } // Remove the Service when the last toolStrip is removed. private void OnComponentRemoved(object sender, ComponentEventArgs e) { bool toolStripPresent = false; ComponentCollection comps = designerHost.Container.Components; foreach (IComponent comp in comps) { if (comp is ToolStrip) { toolStripPresent = true; break; } } if (!toolStripPresent) { ToolStripInSituService inSituService = (ToolStripInSituService)sp.GetService(typeof(ISupportInSituService)); if (inSituService != null) { //since we are going away .. restore the old commands. designerHost.RemoveService(typeof(ISupportInSituService)); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
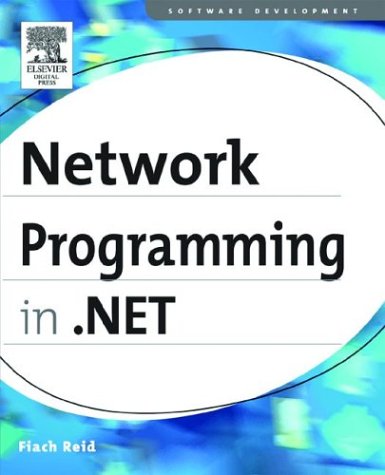
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ListDictionary.cs
- XmlSchemaDatatype.cs
- FileDialogCustomPlace.cs
- XmlMemberMapping.cs
- DiagnosticTraceSource.cs
- TextParagraphView.cs
- OfTypeExpression.cs
- validationstate.cs
- WorkflowPageSetupDialog.cs
- ManualResetEvent.cs
- RtfControlWordInfo.cs
- XmlObjectSerializerWriteContext.cs
- Pen.cs
- XmlnsCompatibleWithAttribute.cs
- InternalBufferManager.cs
- TextTreeExtractElementUndoUnit.cs
- ClaimTypes.cs
- FixedNode.cs
- AttachedAnnotation.cs
- CollectionsUtil.cs
- Mutex.cs
- RedirectionProxy.cs
- SqlCaseSimplifier.cs
- PerformanceCountersElement.cs
- CompareValidator.cs
- FontWeights.cs
- ImageConverter.cs
- Int32Rect.cs
- XmlSchemaDocumentation.cs
- ObjectListShowCommandsEventArgs.cs
- SettingsBindableAttribute.cs
- Cursor.cs
- PropertyDescriptors.cs
- ColumnWidthChangingEvent.cs
- DataGridRowEventArgs.cs
- DataGridItemEventArgs.cs
- BitStream.cs
- DataContractSerializerFaultFormatter.cs
- CryptographicAttribute.cs
- DesignerHost.cs
- TableStyle.cs
- SchemaTableColumn.cs
- Int16AnimationUsingKeyFrames.cs
- RegistrationServices.cs
- DoubleCollectionConverter.cs
- AssociativeAggregationOperator.cs
- StaticExtension.cs
- _ConnectionGroup.cs
- PeerCustomResolverSettings.cs
- Root.cs
- BaseResourcesBuildProvider.cs
- ColorIndependentAnimationStorage.cs
- PartialTrustVisibleAssemblyCollection.cs
- MonitorWrapper.cs
- RegexNode.cs
- CaretElement.cs
- FixedBufferAttribute.cs
- AbstractDataSvcMapFileLoader.cs
- MultiPropertyDescriptorGridEntry.cs
- XamlInterfaces.cs
- FormatPage.cs
- Button.cs
- Main.cs
- RC2CryptoServiceProvider.cs
- PageThemeParser.cs
- IgnoreDeviceFilterElement.cs
- BitmapCacheBrush.cs
- XmlSchemaSimpleContentExtension.cs
- Calendar.cs
- ChangeToolStripParentVerb.cs
- SiteMapPath.cs
- XmlMembersMapping.cs
- Cloud.cs
- TextBoxBase.cs
- TPLETWProvider.cs
- VirtualPathProvider.cs
- WpfGeneratedKnownTypes.cs
- TcpHostedTransportConfiguration.cs
- DurableInstanceContextProvider.cs
- SessionEndingEventArgs.cs
- PropertyTabChangedEvent.cs
- SqlClientFactory.cs
- FormViewModeEventArgs.cs
- RealizedColumnsBlock.cs
- DrawTreeNodeEventArgs.cs
- MouseButtonEventArgs.cs
- ControlBuilder.cs
- WindowsAuthenticationModule.cs
- SecurityTokenSerializer.cs
- RolePrincipal.cs
- TraceSwitch.cs
- VariableQuery.cs
- DependencyObject.cs
- ThicknessKeyFrameCollection.cs
- RecognizerInfo.cs
- CustomPeerResolverService.cs
- KnownColorTable.cs
- Point3DKeyFrameCollection.cs
- NaturalLanguageHyphenator.cs
- ConfigurationFileMap.cs