Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Extensions / UI / WebControls / Expressions / OfTypeExpression.cs / 1305376 / OfTypeExpression.cs
#if ORYX_VNEXT namespace Microsoft.Web.Data.UI.WebControls.Expressions { #else namespace System.Web.UI.WebControls.Expressions { #endif using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Linq; using System.Linq.Expressions; using System.Reflection; using System.Web.Compilation; using System.Web.Resources; using System; using System.Web.UI; public class OfTypeExpression : DataSourceExpression { private MethodInfo _ofTypeMethod; private string _typeName; private MethodInfo OfTypeMethod { get { if (_ofTypeMethod == null) { var type = GetType(TypeName); _ofTypeMethod = GetOfTypeMethod(type); } return _ofTypeMethod; } } [DefaultValue("")] public string TypeName { get { return _typeName ?? String.Empty; } set { if (TypeName != value) { _typeName = value; _ofTypeMethod = null; } } } public OfTypeExpression() { } public OfTypeExpression(Type type) { if (type == null) { throw new ArgumentNullException("type"); } TypeName = type.AssemblyQualifiedName; _ofTypeMethod = GetOfTypeMethod(type); } // internal for unit testing internal OfTypeExpression(Control owner) : base(owner) { } private Type GetType(string typeName) { if (String.IsNullOrEmpty(typeName)) { throw new InvalidOperationException(String.Format(CultureInfo.CurrentCulture, AtlasWeb.OfTypeExpression_TypeNameNotSpecified, Owner.ID)); } try { return BuildManager.GetType(typeName, true /* throwOnError */, true /* ignoreCase */); } catch (Exception e) { throw new InvalidOperationException(String.Format(CultureInfo.CurrentCulture, AtlasWeb.OfTypeExpression_CannotFindType, typeName, Owner.ID), e); } } private static MethodInfo GetOfTypeMethod(Type type) { Debug.Assert(type != null); return typeof(Queryable).GetMethod("OfType").MakeGenericMethod(new Type[] { type }); } public override IQueryable GetQueryable(IQueryable query) { return query.Provider.CreateQuery(Expression.Call(null, OfTypeMethod, query.Expression)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
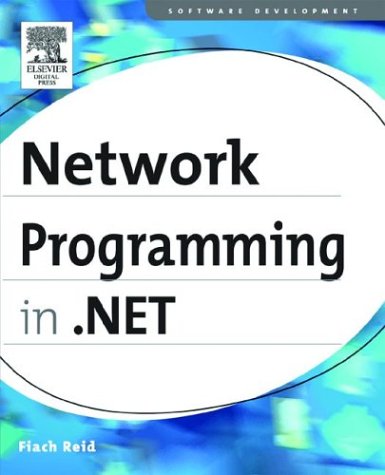
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CheckBoxList.cs
- DataViewSetting.cs
- SwitchElementsCollection.cs
- TransformedBitmap.cs
- SiteMapProvider.cs
- ValidationEventArgs.cs
- DataServiceEntityAttribute.cs
- ExpanderAutomationPeer.cs
- Point3DAnimationBase.cs
- ComPlusDiagnosticTraceSchemas.cs
- CalendarButton.cs
- PlatformCulture.cs
- SystemException.cs
- Focus.cs
- MembershipValidatePasswordEventArgs.cs
- RuntimeEnvironment.cs
- HwndProxyElementProvider.cs
- SQLGuidStorage.cs
- MatchingStyle.cs
- SqlProcedureAttribute.cs
- LifetimeServices.cs
- ReflectionUtil.cs
- ExtensionFile.cs
- Geometry.cs
- HttpsChannelFactory.cs
- XmlWrappingReader.cs
- PermissionToken.cs
- HyperLinkField.cs
- CodeNamespaceImportCollection.cs
- HTTPNotFoundHandler.cs
- VisualBrush.cs
- MembershipUser.cs
- figurelengthconverter.cs
- sqlmetadatafactory.cs
- DefaultDialogButtons.cs
- LogicalTreeHelper.cs
- BitmapImage.cs
- httpapplicationstate.cs
- RSAOAEPKeyExchangeDeformatter.cs
- Compress.cs
- ApplicationSecurityManager.cs
- StatusBarDrawItemEvent.cs
- DocumentViewerHelper.cs
- ValidateNames.cs
- TracingConnectionListener.cs
- AsymmetricCryptoHandle.cs
- NotifyInputEventArgs.cs
- XNodeNavigator.cs
- LinqDataSourceDeleteEventArgs.cs
- NestedContainer.cs
- TablePatternIdentifiers.cs
- DataServiceException.cs
- RadioButtonPopupAdapter.cs
- WebPartUtil.cs
- MatrixConverter.cs
- BinaryCommonClasses.cs
- FileCodeGroup.cs
- CssStyleCollection.cs
- XmlWrappingReader.cs
- ObjectViewQueryResultData.cs
- RectangleGeometry.cs
- ListViewInsertionMark.cs
- BaseTreeIterator.cs
- UndoManager.cs
- DecimalKeyFrameCollection.cs
- SafeViewOfFileHandle.cs
- DataGridViewAutoSizeModeEventArgs.cs
- VisualProxy.cs
- ChooseAction.cs
- LayoutEditorPart.cs
- SessionMode.cs
- SBCSCodePageEncoding.cs
- SQLBinary.cs
- HtmlControl.cs
- __ConsoleStream.cs
- Selection.cs
- XmlDsigSep2000.cs
- CookieProtection.cs
- ListViewSelectEventArgs.cs
- ItemCheckEvent.cs
- DetailsViewPagerRow.cs
- SqlTrackingQuery.cs
- PlanCompilerUtil.cs
- CapabilitiesState.cs
- SignatureToken.cs
- DrawingGroup.cs
- SecureEnvironment.cs
- HtmlElementErrorEventArgs.cs
- GenericTypeParameterBuilder.cs
- ManipulationDelta.cs
- XsdDuration.cs
- JsonEncodingStreamWrapper.cs
- RowVisual.cs
- URI.cs
- Comparer.cs
- TdsParserHelperClasses.cs
- EventPropertyMap.cs
- HttpCacheParams.cs
- PropertyMapper.cs
- EventLogRecord.cs