Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / CommonUI / System / Drawing / Advanced / SizeFConverter.cs / 1 / SizeFConverter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Drawing { using System.Runtime.Serialization.Formatters; using System.Runtime.InteropServices; using System.Diagnostics; using Microsoft.Win32; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Globalization; using System.Reflection; using System.Diagnostics.CodeAnalysis; ////// /// SizeFConverter is a class that can be used to convert /// SizeF from one data type to another. Access this /// class through the TypeDescriptor. /// public class SizeFConverter : TypeConverter { ////// /// Determines if this converter can convert an object in the given source /// type to the native type of the converter. /// public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } return base.CanConvertFrom(context, sourceType); } ////// /// public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(InstanceDescriptor)) { return true; } return base.CanConvertTo(context, destinationType); } ///Gets a value indicating whether this converter can /// convert an object to the given destination type using the context. ////// /// Converts the given object to the converter's native type. /// [SuppressMessage("Microsoft.Performance", "CA1808:AvoidCallsThatBoxValueTypes")] public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { string strValue = value as string; if (strValue != null) { string text = strValue.Trim(); if (text.Length == 0) { return null; } else { // Parse 2 integer values. // if (culture == null) { culture = CultureInfo.CurrentCulture; } char sep = culture.TextInfo.ListSeparator[0]; string[] tokens = text.Split(new char[] {sep}); float[] values = new float[tokens.Length]; TypeConverter floatConverter = TypeDescriptor.GetConverter(typeof(float)); for (int i = 0; i < values.Length; i++) { values[i] = (float)floatConverter.ConvertFromString(context, culture, tokens[i]); } if (values.Length == 2) { return new SizeF(values[0], values[1]); } else { throw new ArgumentException(SR.GetString(SR.TextParseFailedFormat, text, "Width,Height")); } } } return base.ConvertFrom(context, culture, value); } ////// /// Converts the given object to another type. The most common types to convert /// are to and from a string object. The default implementation will make a call /// to ToString on the object if the object is valid and if the destination /// type is string. If this cannot convert to the desitnation type, this will /// throw a NotSupportedException. /// [SuppressMessage("Microsoft.Performance", "CA1800:DoNotCastUnnecessarily")] public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == null) { throw new ArgumentNullException("destinationType"); } if (destinationType == typeof(string) && value is SizeF) { SizeF size = (SizeF)value; if (culture == null) { culture = CultureInfo.CurrentCulture; } string sep = culture.TextInfo.ListSeparator + " "; TypeConverter floatConverter = TypeDescriptor.GetConverter(typeof(float)); string[] args = new string[2]; int nArg = 0; args[nArg++] = floatConverter.ConvertToString(context, culture, size.Width); args[nArg++] = floatConverter.ConvertToString(context, culture, size.Height); return string.Join(sep, args); } if (destinationType == typeof(InstanceDescriptor) && value is SizeF) { SizeF size = (SizeF)value; ConstructorInfo ctor = typeof(SizeF).GetConstructor(new Type[] {typeof(float), typeof(float)}); if (ctor != null) { return new InstanceDescriptor(ctor, new object[] {size.Width, size.Height}); } } return base.ConvertTo(context, culture, value, destinationType); } ////// /// Creates an instance of this type given a set of property values /// for the object. This is useful for objects that are immutable, but still /// want to provide changable properties. /// [SuppressMessage("Microsoft.Performance", "CA1808:AvoidCallsThatBoxValueTypes")] public override object CreateInstance(ITypeDescriptorContext context, IDictionary propertyValues) { return new SizeF((float)propertyValues["Width"], (float)propertyValues["Height"]); } ////// /// Determines if changing a value on this object should require a call to /// CreateInstance to create a new value. /// public override bool GetCreateInstanceSupported(ITypeDescriptorContext context) { return true; } ////// /// Retrieves the set of properties for this type. By default, a type has /// does not return any properties. An easy implementation of this method /// can just call TypeDescriptor.GetProperties for the correct data type. /// public override PropertyDescriptorCollection GetProperties(ITypeDescriptorContext context, object value, Attribute[] attributes) { PropertyDescriptorCollection props = TypeDescriptor.GetProperties(typeof(SizeF), attributes); return props.Sort(new string[] {"Width", "Height"}); } ////// /// Determines if this object supports properties. By default, this /// is false. /// public override bool GetPropertiesSupported(ITypeDescriptorContext context) { return true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
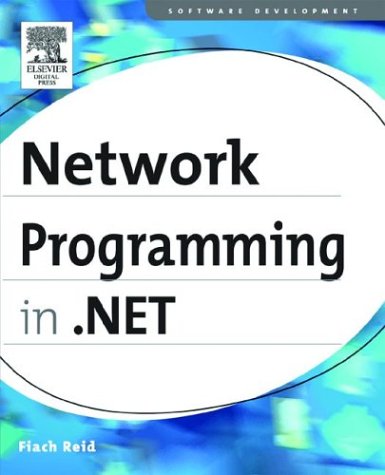
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- KeyTime.cs
- BitmapImage.cs
- CodeArrayIndexerExpression.cs
- CodeDelegateCreateExpression.cs
- BitmapEffectDrawing.cs
- Activity.cs
- DtrList.cs
- IOException.cs
- StringConcat.cs
- MetadataItemCollectionFactory.cs
- EqualityComparer.cs
- ConfigurationLockCollection.cs
- MembershipPasswordException.cs
- NullExtension.cs
- TabPanel.cs
- TextSpan.cs
- CapabilitiesState.cs
- SecurityTokenSerializer.cs
- DataContractJsonSerializer.cs
- VariantWrapper.cs
- FastPropertyAccessor.cs
- CustomErrorsSectionWrapper.cs
- CommentEmitter.cs
- HttpValueCollection.cs
- XmlSerializerImportOptions.cs
- TcpClientSocketManager.cs
- SamlAuthorizationDecisionClaimResource.cs
- Rights.cs
- EventLogPermissionEntry.cs
- InfoCardTraceRecord.cs
- CodeGeneratorOptions.cs
- XmlWriterSettings.cs
- WindowsGrip.cs
- DriveInfo.cs
- ManagementObject.cs
- RuntimeEnvironment.cs
- DesignerDataSourceView.cs
- SettingsPropertyIsReadOnlyException.cs
- NegotiateStream.cs
- TreeNode.cs
- TextEditorMouse.cs
- Choices.cs
- Maps.cs
- PresentationTraceSources.cs
- Processor.cs
- SerializationStore.cs
- TaskFileService.cs
- ThemeableAttribute.cs
- XmlDocumentSurrogate.cs
- HandleRef.cs
- PathStreamGeometryContext.cs
- DesignerActionVerbList.cs
- ViewBox.cs
- AspCompat.cs
- PagesSection.cs
- DataKey.cs
- ValidateNames.cs
- LicenseProviderAttribute.cs
- mansign.cs
- HostedTransportConfigurationManager.cs
- X509Logo.cs
- EmptyEnumerator.cs
- ComponentDispatcherThread.cs
- TemplatedAdorner.cs
- _NTAuthentication.cs
- WsdlWriter.cs
- BindingCompleteEventArgs.cs
- SQLDecimal.cs
- LeaseManager.cs
- FileUtil.cs
- ElementHost.cs
- ReferencedCollectionType.cs
- FunctionNode.cs
- BindingCollection.cs
- TabItem.cs
- OracleString.cs
- IPEndPoint.cs
- ArrayTypeMismatchException.cs
- StackSpiller.Generated.cs
- SiteMapSection.cs
- CommonProperties.cs
- WindowsPen.cs
- assertwrapper.cs
- ItemContainerPattern.cs
- CommandLineParser.cs
- RoleManagerEventArgs.cs
- Util.cs
- UserControl.cs
- TemplateField.cs
- RenderData.cs
- Trace.cs
- WebPartEventArgs.cs
- Floater.cs
- ContractHandle.cs
- TransactionOptions.cs
- SQLGuidStorage.cs
- List.cs
- XLinq.cs
- TransactionScopeDesigner.cs
- TickBar.cs