Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / CompMod / System / CodeDOM / Compiler / TempFiles.cs / 1305376 / TempFiles.cs
//------------------------------------------------------------------------------ //// // //----------------------------------------------------------------------------- namespace System.CodeDom.Compiler { using System; using System.Collections; using System.Diagnostics; using System.IO; using System.Runtime.InteropServices; using System.Text; using Microsoft.Win32; using System.Security; using System.Security.Permissions; using System.Security.Principal; using System.ComponentModel; using System.Security.Cryptography; using System.Globalization; using System.Runtime.Versioning; ///[....] // Copyright (c) Microsoft Corporation. All rights reserved. ///// [PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] [PermissionSet(SecurityAction.InheritanceDemand, Name="FullTrust")] [Serializable] public class TempFileCollection : ICollection, IDisposable { string basePath; string tempDir; bool keepFiles; Hashtable files; ///Represents a collection of temporary file names that are all based on a /// single base filename located in a temporary directory. ////// public TempFileCollection() : this(null, false) { } ///[To be supplied.] ////// public TempFileCollection(string tempDir) : this(tempDir, false) { } ///[To be supplied.] ////// public TempFileCollection(string tempDir, bool keepFiles) { this.keepFiles = keepFiles; this.tempDir = tempDir; #if !FEATURE_CASE_SENSITIVE_FILESYSTEM files = new Hashtable(StringComparer.OrdinalIgnoreCase); #else files = new Hashtable(); #endif } ///[To be supplied.] ////// /// void IDisposable.Dispose() { Dispose(true); GC.SuppressFinalize(this); } protected virtual void Dispose(bool disposing) { // It is safe to call Delete from here even if Dispose is called from Finalizer // because the graph of objects is guaranteed to be there and // neither Hashtable nor String have a finalizer of their own that could // be called before TempFileCollection Finalizer Delete(); } ///To allow it's stuff to be cleaned up ////// ~TempFileCollection() { Dispose(false); } ///[To be supplied.] ////// public string AddExtension(string fileExtension) { return AddExtension(fileExtension, keepFiles); } ///[To be supplied.] ////// public string AddExtension(string fileExtension, bool keepFile) { if (fileExtension == null || fileExtension.Length == 0) throw new ArgumentException(SR.GetString(SR.InvalidNullEmptyArgument, "fileExtension"), "fileExtension"); // fileExtension not specified string fileName = BasePath + "." + fileExtension; AddFile(fileName, keepFile); return fileName; } ///[To be supplied.] ////// public void AddFile(string fileName, bool keepFile) { if (fileName == null || fileName.Length == 0) throw new ArgumentException(SR.GetString(SR.InvalidNullEmptyArgument, "fileName"), "fileName"); // fileName not specified if (files[fileName] != null) throw new ArgumentException(SR.GetString(SR.DuplicateFileName, fileName), "fileName"); // duplicate fileName files.Add(fileName, (object)keepFile); } ///[To be supplied.] ////// public IEnumerator GetEnumerator() { return files.Keys.GetEnumerator(); } ///[To be supplied.] ///IEnumerator IEnumerable.GetEnumerator() { return files.Keys.GetEnumerator(); } /// void ICollection.CopyTo(Array array, int start) { files.Keys.CopyTo(array, start); } /// /// public void CopyTo(string[] fileNames, int start) { files.Keys.CopyTo(fileNames, start); } ///[To be supplied.] ////// public int Count { get { return files.Count; } } ///[To be supplied.] ///int ICollection.Count { get { return files.Count; } } /// object ICollection.SyncRoot { get { return null; } } /// bool ICollection.IsSynchronized { get { return false; } } /// /// public string TempDir { get { return tempDir == null ? string.Empty : tempDir; } } ///[To be supplied.] ////// public string BasePath { get { EnsureTempNameCreated(); return basePath; } } [ResourceExposure(ResourceScope.None)] [ResourceConsumption(ResourceScope.Machine, ResourceScope.Machine)] void EnsureTempNameCreated() { if (basePath == null) { string tempFileName = null; FileStream tempFileStream; bool uniqueFile = false; int retryCount = 5000; do { try { basePath = GetTempFileName(TempDir); string full = Path.GetFullPath(basePath); new FileIOPermission(FileIOPermissionAccess.AllAccess, full).Demand(); // make sure the filename is unique. tempFileName = basePath + ".tmp"; using (tempFileStream = new FileStream(tempFileName, FileMode.CreateNew, FileAccess.Write)) { } uniqueFile = true; } catch (IOException e) { retryCount--; uint HR_ERROR_FILE_EXISTS = unchecked(((uint)0x80070000) | NativeMethods.ERROR_FILE_EXISTS); if (retryCount == 0 || Marshal.GetHRForException(e) != HR_ERROR_FILE_EXISTS) throw; uniqueFile = false; } }while (!uniqueFile); files.Add(tempFileName, keepFiles); } } ///[To be supplied.] ////// public bool KeepFiles { get { return keepFiles; } set { keepFiles = value; } } bool KeepFile(string fileName) { object keep = files[fileName]; if (keep == null) return false; return (bool)keep; } ///[To be supplied.] ////// [ResourceExposure(ResourceScope.None)] [ResourceConsumption(ResourceScope.Machine, ResourceScope.Machine)] public void Delete() { if (files != null && files.Count > 0) { string[] fileNames = new string[files.Count]; files.Keys.CopyTo(fileNames, 0); foreach (string fileName in fileNames) { if (!KeepFile(fileName)) { Delete(fileName); files.Remove(fileName); } } } } // This function deletes files after reverting impersonation. [ResourceExposure(ResourceScope.None)] [ResourceConsumption(ResourceScope.Process, ResourceScope.Process)] internal void SafeDelete() { #if !FEATURE_PAL WindowsImpersonationContext impersonation = Executor.RevertImpersonation(); #endif try{ Delete(); } finally { #if !FEATURE_PAL Executor.ReImpersonate(impersonation); #endif } } [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] void Delete(string fileName) { try { File.Delete(fileName); } catch { // Ignore all exceptions } } [ResourceExposure(ResourceScope.Machine)] [ResourceConsumption(ResourceScope.Machine)] static string GetTempFileName(string tempDir) { string fileName; if (String.IsNullOrEmpty(tempDir)) tempDir = Path.GetTempPath(); string randomFileName = Path.GetFileNameWithoutExtension(Path.GetRandomFileName()); if (tempDir.EndsWith("\\", StringComparison.Ordinal)) fileName = tempDir + randomFileName; else fileName = tempDir + "\\" + randomFileName; return fileName; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
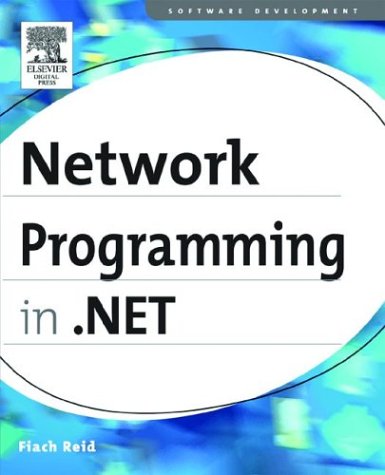
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CaseInsensitiveComparer.cs
- StateWorkerRequest.cs
- WebPartDesigner.cs
- Propagator.cs
- Rotation3D.cs
- NavigationHelper.cs
- InfoCardRSACryptoProvider.cs
- FixedNode.cs
- Geometry3D.cs
- ListControl.cs
- StructuredTypeEmitter.cs
- PrintEvent.cs
- TrustSection.cs
- SynchronizationLockException.cs
- ChannelDispatcher.cs
- XsdDateTime.cs
- ECDiffieHellmanCng.cs
- RowUpdatingEventArgs.cs
- XmlSerializerNamespaces.cs
- DoubleCollection.cs
- MetadataFile.cs
- IntPtr.cs
- XmlILAnnotation.cs
- ColumnTypeConverter.cs
- SectionUpdates.cs
- ECDiffieHellman.cs
- ConfigXmlSignificantWhitespace.cs
- TypefaceMetricsCache.cs
- EntitySqlQueryCacheEntry.cs
- FrameworkContextData.cs
- Rect3DValueSerializer.cs
- TableItemProviderWrapper.cs
- TabControlCancelEvent.cs
- GiveFeedbackEventArgs.cs
- ToolStripOverflow.cs
- RefreshEventArgs.cs
- SqlDataSourceCommandEventArgs.cs
- ProfileBuildProvider.cs
- securestring.cs
- Light.cs
- PrivateUnsafeNativeCompoundFileMethods.cs
- OracleParameter.cs
- DataControlLinkButton.cs
- DataGridTextBox.cs
- LinqDataSourceContextEventArgs.cs
- BrowserCapabilitiesCompiler.cs
- NodeLabelEditEvent.cs
- DetailsViewDeletedEventArgs.cs
- WorkflowRuntimeServiceElementCollection.cs
- ADMembershipUser.cs
- TransformGroup.cs
- NullReferenceException.cs
- ConnectionManagementElementCollection.cs
- ObjectQueryProvider.cs
- MsmqIntegrationBindingElement.cs
- TableColumnCollection.cs
- URLAttribute.cs
- GuidConverter.cs
- SslStreamSecurityUpgradeProvider.cs
- __ComObject.cs
- BitmapFrameEncode.cs
- CachedPathData.cs
- DataGridViewSortCompareEventArgs.cs
- ToolStripSplitStackLayout.cs
- EventLogWatcher.cs
- SelectionGlyphBase.cs
- ZipIOLocalFileHeader.cs
- XmlChoiceIdentifierAttribute.cs
- StorageMappingItemCollection.cs
- NameObjectCollectionBase.cs
- QuaternionAnimation.cs
- UInt64Converter.cs
- OleDbReferenceCollection.cs
- StateManagedCollection.cs
- Int32Rect.cs
- EarlyBoundInfo.cs
- StatusBar.cs
- XPathSelfQuery.cs
- RSAPKCS1KeyExchangeDeformatter.cs
- ExtensionWindowResizeGrip.cs
- DataRowExtensions.cs
- PartialTrustHelpers.cs
- Point3DCollectionConverter.cs
- FastEncoder.cs
- RIPEMD160.cs
- DataGridViewRowStateChangedEventArgs.cs
- Drawing.cs
- MarshalByRefObject.cs
- Attributes.cs
- SByte.cs
- PasswordTextContainer.cs
- altserialization.cs
- UmAlQuraCalendar.cs
- ArrayWithOffset.cs
- WebCategoryAttribute.cs
- MD5.cs
- DataSourceConverter.cs
- SafeLibraryHandle.cs
- TraceSection.cs
- InputLangChangeRequestEvent.cs