Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Xml / System / Xml / Serialization / XmlSerializerFactory.cs / 1 / XmlSerializerFactory.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Serialization { using System.Reflection; using System.Collections; using System.IO; using System.Xml.Schema; using System; using System.Text; using System.Threading; using System.Globalization; using System.Security.Permissions; using System.Security.Policy; using System.Xml.Serialization.Configuration; using System.Diagnostics; using System.CodeDom.Compiler; ////// /// public class XmlSerializerFactory { static TempAssemblyCache cache = new TempAssemblyCache(); ///[To be supplied.] ////// /// public XmlSerializer CreateSerializer(Type type, XmlAttributeOverrides overrides, Type[] extraTypes, XmlRootAttribute root, string defaultNamespace) { return CreateSerializer(type, overrides, extraTypes, root, defaultNamespace, null, null); } ///[To be supplied.] ////// /// public XmlSerializer CreateSerializer(Type type, XmlRootAttribute root) { return CreateSerializer(type, null, new Type[0], root, null, null, null); } ///[To be supplied.] ////// /// public XmlSerializer CreateSerializer(Type type, Type[] extraTypes) { return CreateSerializer(type, null, extraTypes, null, null, null, null); } ///[To be supplied.] ////// /// public XmlSerializer CreateSerializer(Type type, XmlAttributeOverrides overrides) { return CreateSerializer(type, overrides, new Type[0], null, null, null, null); } ///[To be supplied.] ////// /// public XmlSerializer CreateSerializer(XmlTypeMapping xmlTypeMapping) { TempAssembly tempAssembly = XmlSerializer.GenerateTempAssembly(xmlTypeMapping); return (XmlSerializer)tempAssembly.Contract.TypedSerializers[xmlTypeMapping.Key]; } ///[To be supplied.] ////// /// public XmlSerializer CreateSerializer(Type type) { return CreateSerializer(type, (string)null); } ///[To be supplied.] ////// /// public XmlSerializer CreateSerializer(Type type, string defaultNamespace) { if (type == null) throw new ArgumentNullException("type"); TempAssembly tempAssembly = cache[defaultNamespace, type]; XmlTypeMapping mapping = null; if (tempAssembly == null) { lock (cache) { tempAssembly = cache[defaultNamespace, type]; if (tempAssembly == null) { XmlSerializerImplementation contract; Assembly assembly = TempAssembly.LoadGeneratedAssembly(type, defaultNamespace, out contract); if (assembly == null) { // need to reflect and generate new serialization assembly XmlReflectionImporter importer = new XmlReflectionImporter(defaultNamespace); mapping = importer.ImportTypeMapping(type, null, defaultNamespace); tempAssembly = XmlSerializer.GenerateTempAssembly(mapping, type, defaultNamespace); } else { tempAssembly = new TempAssembly(contract); } cache.Add(defaultNamespace, type, tempAssembly); } } } if (mapping == null) { mapping = XmlReflectionImporter.GetTopLevelMapping(type, defaultNamespace); } return (XmlSerializer)tempAssembly.Contract.GetSerializer(type); } ///[To be supplied.] ////// /// public XmlSerializer CreateSerializer(Type type, XmlAttributeOverrides overrides, Type[] extraTypes, XmlRootAttribute root, string defaultNamespace, string location, Evidence evidence) { if (type == null) throw new ArgumentNullException("type"); XmlReflectionImporter importer = new XmlReflectionImporter(overrides, defaultNamespace); for (int i = 0; i < extraTypes.Length; i++) importer.IncludeType(extraTypes[i]); XmlTypeMapping mapping = importer.ImportTypeMapping(type, root, defaultNamespace); TempAssembly tempAssembly = XmlSerializer.GenerateTempAssembly(mapping, type, defaultNamespace, location, evidence); return (XmlSerializer)tempAssembly.Contract.TypedSerializers[mapping.Key]; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.[To be supplied.] ///
Link Menu
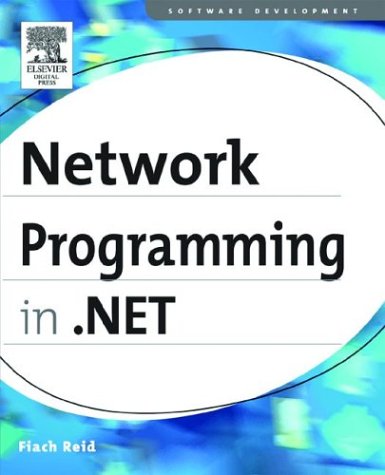
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ArraySet.cs
- DecoderFallbackWithFailureFlag.cs
- _AutoWebProxyScriptWrapper.cs
- SafeSecurityHandles.cs
- DataShape.cs
- InternalBufferOverflowException.cs
- ComponentTray.cs
- MimeWriter.cs
- AdRotator.cs
- ThemeDirectoryCompiler.cs
- ContentFilePart.cs
- OdbcInfoMessageEvent.cs
- Double.cs
- SoapObjectReader.cs
- ConstructorNeedsTagAttribute.cs
- DataGridViewRowEventArgs.cs
- StringResourceManager.cs
- SdlChannelSink.cs
- RuleSettingsCollection.cs
- SoapIgnoreAttribute.cs
- basevalidator.cs
- PointConverter.cs
- ResolveDuplex11AsyncResult.cs
- safemediahandle.cs
- ColumnResizeUndoUnit.cs
- VersionedStream.cs
- FactoryRecord.cs
- SemanticResultValue.cs
- PageAsyncTaskManager.cs
- FontResourceCache.cs
- XmlIgnoreAttribute.cs
- wmiprovider.cs
- Listener.cs
- XsltException.cs
- WorkflowLayouts.cs
- DrawingServices.cs
- TransportConfigurationTypeElementCollection.cs
- LoginViewDesigner.cs
- contentDescriptor.cs
- ViewStateChangedEventArgs.cs
- DataGridViewCellStyleConverter.cs
- StyleModeStack.cs
- StyleModeStack.cs
- CachedPathData.cs
- Latin1Encoding.cs
- WebPartConnectionsEventArgs.cs
- DataGridViewComboBoxEditingControl.cs
- IpcManager.cs
- XmlDataSourceView.cs
- Helper.cs
- CodeTypeConstructor.cs
- CodeLinePragma.cs
- ELinqQueryState.cs
- FormViewPageEventArgs.cs
- Inline.cs
- AssemblyBuilder.cs
- PlainXmlSerializer.cs
- SqlRetyper.cs
- WSDualHttpSecurityElement.cs
- FormatterServices.cs
- MessageBuffer.cs
- ArglessEventHandlerProxy.cs
- FontCacheUtil.cs
- SQLMoneyStorage.cs
- Configuration.cs
- SubtreeProcessor.cs
- SpellerHighlightLayer.cs
- ProcessModelSection.cs
- PresentationTraceSources.cs
- DecoderNLS.cs
- MappingSource.cs
- MembershipPasswordException.cs
- KeyPullup.cs
- IResourceProvider.cs
- DataServiceKeyAttribute.cs
- TableLayoutPanel.cs
- BuildProviderCollection.cs
- CurrentTimeZone.cs
- SettingsPropertyWrongTypeException.cs
- GreenMethods.cs
- AutoFocusStyle.xaml.cs
- ConfigXmlAttribute.cs
- SingleAnimationUsingKeyFrames.cs
- AuthenticationModuleElementCollection.cs
- InvalidAsynchronousStateException.cs
- DataGridRow.cs
- ProcessManager.cs
- SqlTypeConverter.cs
- GPRECTF.cs
- WebRequestModuleElementCollection.cs
- Listbox.cs
- EmptyEnumerator.cs
- SecurityDocument.cs
- PerformanceCountersElement.cs
- ChannelManager.cs
- Identifier.cs
- RayHitTestParameters.cs
- WebContext.cs
- ButtonStandardAdapter.cs
- Rotation3DAnimationUsingKeyFrames.cs