Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / IO / MemoryMappedFiles / MemoryMappedViewStream.cs / 1305376 / MemoryMappedViewStream.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: MemoryMappedViewStream ** ** Purpose: View stream for managed MemoryMappedFiles. ** ** Date: February 7, 2007 ** ===========================================================*/ using System; using System.Diagnostics; using System.Security.Permissions; using Microsoft.Win32.SafeHandles; namespace System.IO.MemoryMappedFiles { public sealed class MemoryMappedViewStream : UnmanagedMemoryStream { private MemoryMappedView m_view; //// [System.Security.SecurityCritical] internal unsafe MemoryMappedViewStream(MemoryMappedView view) { Debug.Assert(view != null, "view is null"); m_view = view; Initialize(m_view.ViewHandle, m_view.PointerOffset, m_view.Size, MemoryMappedFile.GetFileAccess(m_view.Access)); } public SafeMemoryMappedViewHandle SafeMemoryMappedViewHandle { //// // [System.Security.SecurityCritical] [SecurityPermissionAttribute(SecurityAction.Demand, Flags = SecurityPermissionFlag.UnmanagedCode)] get { return m_view != null ? m_view.ViewHandle : null; } } public override void SetLength(long value) { throw new NotSupportedException(SR.GetString(SR.NotSupported_MMViewStreamsFixedLength)); } //// // [System.Security.SecurityCritical] protected override void Dispose(bool disposing) { try { if (disposing && m_view != null && !m_view.IsClosed) { Flush(); } } finally { try { if (m_view != null) { m_view.Dispose(); } } finally { base.Dispose(disposing); } } } // Flushes the changes such that they are in [....] with the FileStream bits (ones obtained // with the win32 ReadFile and WriteFile functions). Need to call FileStream's Flush to // flush to the disk. // NOTE: This will flush all bytes before and after the view up until an offset that is a // multiple of SystemPageSize. //// // [System.Security.SecurityCritical] public override void Flush() { if (!CanSeek) { __Error.StreamIsClosed(); } unsafe { if (m_view != null) { m_view.Flush((IntPtr)Capacity); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.//
Link Menu
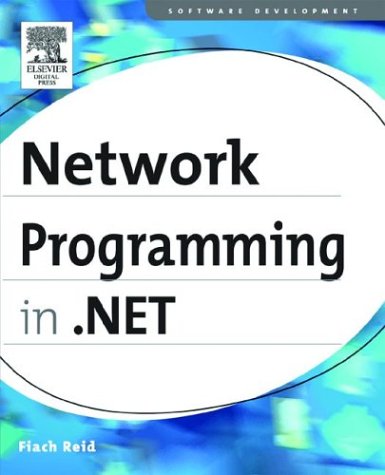
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- OleDbStruct.cs
- StackBuilderSink.cs
- ImageButton.cs
- WindowPatternIdentifiers.cs
- ObjectStateEntry.cs
- TextUtf8RawTextWriter.cs
- SQLBytesStorage.cs
- Compress.cs
- IERequestCache.cs
- MimeObjectFactory.cs
- ProjectionPruner.cs
- ReflectionUtil.cs
- UserMapPath.cs
- PolyQuadraticBezierSegment.cs
- PtsCache.cs
- CodeGroup.cs
- DesignerExtenders.cs
- XmlObjectSerializerReadContext.cs
- DataPager.cs
- DefaultObjectSerializer.cs
- RowType.cs
- DeploymentSectionCache.cs
- PropertyGrid.cs
- WebPartZone.cs
- HttpCapabilitiesSectionHandler.cs
- DataObjectFieldAttribute.cs
- LabelAutomationPeer.cs
- SignatureGenerator.cs
- PrintingPermissionAttribute.cs
- XmlBinaryWriter.cs
- ConfigurationManagerInternalFactory.cs
- ArcSegment.cs
- UrlMappingCollection.cs
- PackageProperties.cs
- TreeViewEvent.cs
- ViewStateException.cs
- InvokeWebServiceDesigner.cs
- SessionIDManager.cs
- ColorAnimation.cs
- SystemUdpStatistics.cs
- WebBrowserSiteBase.cs
- RectAnimationClockResource.cs
- PrintEvent.cs
- AnnotationResourceChangedEventArgs.cs
- ConfigurationStrings.cs
- ObjectQuery.cs
- SapiInterop.cs
- ResourceAssociationTypeEnd.cs
- BrowserDefinition.cs
- recordstate.cs
- ResourceReferenceExpressionConverter.cs
- QuaternionAnimation.cs
- KerberosTokenFactoryCredential.cs
- SingleAnimation.cs
- PathFigure.cs
- SizeLimitedCache.cs
- CounterSampleCalculator.cs
- Listbox.cs
- BinarySerializer.cs
- ReflectionPermission.cs
- RegistryKey.cs
- InstallerTypeAttribute.cs
- DbException.cs
- Debug.cs
- FlowDocumentScrollViewerAutomationPeer.cs
- SafeNativeMethods.cs
- VariableAction.cs
- panel.cs
- DateTimeOffsetAdapter.cs
- CancelRequestedRecord.cs
- DataSourceProvider.cs
- ProxyWebPartConnectionCollection.cs
- Constants.cs
- SpnEndpointIdentity.cs
- DataColumnMappingCollection.cs
- Italic.cs
- XmlNamespaceDeclarationsAttribute.cs
- XmlResolver.cs
- LowerCaseStringConverter.cs
- XmlQueryTypeFactory.cs
- ConfigurationLocationCollection.cs
- SQLDecimalStorage.cs
- X509InitiatorCertificateClientElement.cs
- ImageConverter.cs
- UpdateCompiler.cs
- MarkupCompilePass1.cs
- ExecutedRoutedEventArgs.cs
- InProcStateClientManager.cs
- WorkflowOwnershipException.cs
- ContentDisposition.cs
- FormsAuthenticationEventArgs.cs
- BitmapPalettes.cs
- DispatcherHooks.cs
- Converter.cs
- SafeBuffer.cs
- TableCellAutomationPeer.cs
- InstalledFontCollection.cs
- printdlgexmarshaler.cs
- StructuralObject.cs
- Classification.cs