Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / MS / Internal / AppModel / MimeObjectFactory.cs / 1 / MimeObjectFactory.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // Provides a set of static methods for transforming pairs of // mime type + stream into objects. // // History: // 11/11/2004: Erichar: Initial creation. // //----------------------------------------------------------------------------- using System; using System.Windows; using System.IO; using System.Collections.Generic; using MS.Internal.Utility; using System.Diagnostics; using System.Globalization; using System.Windows.Markup; namespace MS.Internal.AppModel { internal delegate object StreamToObjectFactoryDelegate(Stream s, Uri baseUri, bool canUseTopLevelBrowser, bool sandboxExternalContent, bool allowAsync, bool isJournalNavigation, out XamlReader asyncObjectConverter); internal static class MimeObjectFactory { //----------------------------------------------------- // // Internal Static Methods // //----------------------------------------------------- #region internal static methods // The delegate that we are calling is responsible for closing the stream internal static object GetObjectAndCloseStream(Stream s, ContentType contentType, Uri baseUri, bool canUseTopLevelBrowser, bool sandboxExternalContent, bool allowAsync, bool isJournalNavigation, out XamlReader asyncObjectConverter) { object objToReturn = null; asyncObjectConverter = null; if (contentType != null) { StreamToObjectFactoryDelegate d; if (_objectConverters.TryGetValue(contentType, out d)) { objToReturn = d(s, baseUri, canUseTopLevelBrowser, sandboxExternalContent, allowAsync, isJournalNavigation, out asyncObjectConverter); } } return objToReturn; } // The delegate registered here will be responsible for closing the stream passed to it. internal static void Register(ContentType contentType, StreamToObjectFactoryDelegate method) { _objectConverters[contentType] = method; } #endregion //------------------------------------------------------ // // Private Members // //----------------------------------------------------- #region private members private static readonly Dictionary_objectConverters = new Dictionary (5, new ContentType.WeakComparer()); #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // // // Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // Provides a set of static methods for transforming pairs of // mime type + stream into objects. // // History: // 11/11/2004: Erichar: Initial creation. // //----------------------------------------------------------------------------- using System; using System.Windows; using System.IO; using System.Collections.Generic; using MS.Internal.Utility; using System.Diagnostics; using System.Globalization; using System.Windows.Markup; namespace MS.Internal.AppModel { internal delegate object StreamToObjectFactoryDelegate(Stream s, Uri baseUri, bool canUseTopLevelBrowser, bool sandboxExternalContent, bool allowAsync, bool isJournalNavigation, out XamlReader asyncObjectConverter); internal static class MimeObjectFactory { //----------------------------------------------------- // // Internal Static Methods // //----------------------------------------------------- #region internal static methods // The delegate that we are calling is responsible for closing the stream internal static object GetObjectAndCloseStream(Stream s, ContentType contentType, Uri baseUri, bool canUseTopLevelBrowser, bool sandboxExternalContent, bool allowAsync, bool isJournalNavigation, out XamlReader asyncObjectConverter) { object objToReturn = null; asyncObjectConverter = null; if (contentType != null) { StreamToObjectFactoryDelegate d; if (_objectConverters.TryGetValue(contentType, out d)) { objToReturn = d(s, baseUri, canUseTopLevelBrowser, sandboxExternalContent, allowAsync, isJournalNavigation, out asyncObjectConverter); } } return objToReturn; } // The delegate registered here will be responsible for closing the stream passed to it. internal static void Register(ContentType contentType, StreamToObjectFactoryDelegate method) { _objectConverters[contentType] = method; } #endregion //------------------------------------------------------ // // Private Members // //----------------------------------------------------- #region private members private static readonly Dictionary_objectConverters = new Dictionary (5, new ContentType.WeakComparer()); #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
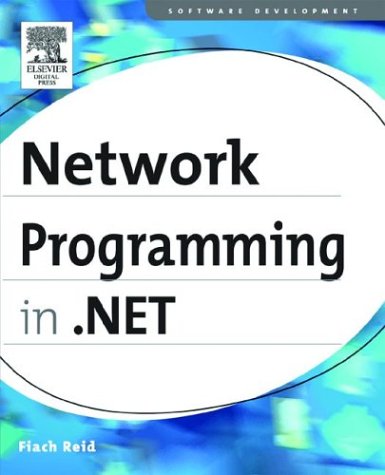
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CheckedListBox.cs
- Calendar.cs
- CultureInfo.cs
- DrawingContextWalker.cs
- RemotingConfigParser.cs
- ScrollItemPattern.cs
- WindowsTokenRoleProvider.cs
- ToggleButtonAutomationPeer.cs
- VSDExceptions.cs
- XmlSchemaCollection.cs
- cookiecollection.cs
- D3DImage.cs
- DesignerHelpers.cs
- ZipIORawDataFileBlock.cs
- ExpandSegment.cs
- SqlMethodCallConverter.cs
- XmlBinaryReader.cs
- ComplexLine.cs
- KnownTypeDataContractResolver.cs
- XmlSerializerAssemblyAttribute.cs
- AdRotatorDesigner.cs
- Matrix3D.cs
- ExpandCollapsePattern.cs
- TableItemPatternIdentifiers.cs
- Int32Rect.cs
- PriorityBinding.cs
- RequestTimeoutManager.cs
- ConfigurationPermission.cs
- PeerCollaboration.cs
- Classification.cs
- RTLAwareMessageBox.cs
- SynchronizedDispatch.cs
- MatchSingleFxEngineOpcode.cs
- StateItem.cs
- NativeMethods.cs
- MoveSizeWinEventHandler.cs
- StdValidatorsAndConverters.cs
- ExceptionRoutedEventArgs.cs
- DesignerAutoFormatStyle.cs
- ConstructorBuilder.cs
- TreeViewImageKeyConverter.cs
- XamlReaderHelper.cs
- CreateWorkflowOwnerCommand.cs
- XmlQueryContext.cs
- RowToParametersTransformer.cs
- HwndTarget.cs
- Header.cs
- KeyTime.cs
- ResourcePool.cs
- CacheVirtualItemsEvent.cs
- MetadataWorkspace.cs
- DebuggerAttributes.cs
- RpcAsyncResult.cs
- MultipartContentParser.cs
- ExpressionEditorAttribute.cs
- ResourceDisplayNameAttribute.cs
- XmlUnspecifiedAttribute.cs
- DataServicePagingProviderWrapper.cs
- SqlRowUpdatedEvent.cs
- LinqDataSource.cs
- RegexCharClass.cs
- ComponentDispatcher.cs
- GridViewEditEventArgs.cs
- LinkedList.cs
- coordinatorfactory.cs
- DataDocumentXPathNavigator.cs
- MemberMaps.cs
- Int32RectValueSerializer.cs
- PerformanceCountersElement.cs
- PathFigureCollection.cs
- OdbcException.cs
- XmlSchemaAttribute.cs
- ToolboxItemAttribute.cs
- StartUpEventArgs.cs
- HttpBufferlessInputStream.cs
- ForEachAction.cs
- CodeBlockBuilder.cs
- TypeToArgumentTypeConverter.cs
- CopyEncoder.cs
- DomainLiteralReader.cs
- ListViewItemSelectionChangedEvent.cs
- AtomServiceDocumentSerializer.cs
- ConfigurationPropertyCollection.cs
- DescendentsWalkerBase.cs
- OdbcConnectionOpen.cs
- TimeSpanValidator.cs
- WindowsTab.cs
- ClientType.cs
- DocumentPageView.cs
- DPCustomTypeDescriptor.cs
- SubMenuStyleCollection.cs
- TypedTableBaseExtensions.cs
- VectorCollectionValueSerializer.cs
- DecoderNLS.cs
- DateTimeSerializationSection.cs
- MetaModel.cs
- SourceFileInfo.cs
- OverrideMode.cs
- InheritanceContextHelper.cs
- SolidBrush.cs