Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / Data / PriorityBinding.cs / 1 / PriorityBinding.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Defines PriorityBinding object, which stores information // for creating instances of PriorityBindingExpression objects. // // See spec at http://avalon/connecteddata/Specs/Data%20Binding.mht // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.ObjectModel; // Collectionusing System.ComponentModel; using System.Globalization; using System.Windows.Markup; using MS.Internal.Data; using MS.Utility; namespace System.Windows.Data { /// /// Describes a collection of bindings attached to a single property. /// These behave as "priority" bindings, meaning that the property /// receives its value from the first binding in the collection that /// can produce a legal value. /// [ContentProperty("Bindings")] public class PriorityBinding : BindingBase, IAddChild { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- ///Constructor public PriorityBinding() : base() { _bindingCollection = new BindingCollection(this, new BindingCollectionChangedCallback(OnBindingCollectionChanged)); } #region IAddChild ////// Called to Add the object as a Child. /// /// /// Object to add as a child - must have type BindingBase /// void IAddChild.AddChild(Object value) { BindingBase binding = value as BindingBase; if (binding != null) Bindings.Add(binding); else throw new ArgumentException(SR.Get(SRID.ChildHasWrongType, this.GetType().Name, "BindingBase", value.GetType().FullName), "value"); } ////// Called when text appears under the tag in markup /// /// /// Text to Add to the Object /// void IAddChild.AddText(string text) { XamlSerializerUtil.ThrowIfNonWhiteSpaceInAddText(text, this); } #endregion IAddChild //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- ///List of inner bindings [DesignerSerializationVisibility(DesignerSerializationVisibility.Content)] public CollectionBindings { get { return _bindingCollection; } } /// /// This method is used by TypeDescriptor to determine if this property should /// be serialized. /// [EditorBrowsable(EditorBrowsableState.Never)] public bool ShouldSerializeBindings() { return (Bindings != null && Bindings.Count > 0); } //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ ////// Create an appropriate expression for this Binding, to be attached /// to the given DependencyProperty on the given DependencyObject. /// internal override BindingExpressionBase CreateBindingExpressionOverride(DependencyObject target, DependencyProperty dp, BindingExpressionBase owner) { return PriorityBindingExpression.CreateBindingExpression(target, dp, this, owner); } private void OnBindingCollectionChanged() { CheckSealed(); } //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ BindingCollection _bindingCollection; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Defines PriorityBinding object, which stores information // for creating instances of PriorityBindingExpression objects. // // See spec at http://avalon/connecteddata/Specs/Data%20Binding.mht // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.ObjectModel; // Collectionusing System.ComponentModel; using System.Globalization; using System.Windows.Markup; using MS.Internal.Data; using MS.Utility; namespace System.Windows.Data { /// /// Describes a collection of bindings attached to a single property. /// These behave as "priority" bindings, meaning that the property /// receives its value from the first binding in the collection that /// can produce a legal value. /// [ContentProperty("Bindings")] public class PriorityBinding : BindingBase, IAddChild { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- ///Constructor public PriorityBinding() : base() { _bindingCollection = new BindingCollection(this, new BindingCollectionChangedCallback(OnBindingCollectionChanged)); } #region IAddChild ////// Called to Add the object as a Child. /// /// /// Object to add as a child - must have type BindingBase /// void IAddChild.AddChild(Object value) { BindingBase binding = value as BindingBase; if (binding != null) Bindings.Add(binding); else throw new ArgumentException(SR.Get(SRID.ChildHasWrongType, this.GetType().Name, "BindingBase", value.GetType().FullName), "value"); } ////// Called when text appears under the tag in markup /// /// /// Text to Add to the Object /// void IAddChild.AddText(string text) { XamlSerializerUtil.ThrowIfNonWhiteSpaceInAddText(text, this); } #endregion IAddChild //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- ///List of inner bindings [DesignerSerializationVisibility(DesignerSerializationVisibility.Content)] public CollectionBindings { get { return _bindingCollection; } } /// /// This method is used by TypeDescriptor to determine if this property should /// be serialized. /// [EditorBrowsable(EditorBrowsableState.Never)] public bool ShouldSerializeBindings() { return (Bindings != null && Bindings.Count > 0); } //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ ////// Create an appropriate expression for this Binding, to be attached /// to the given DependencyProperty on the given DependencyObject. /// internal override BindingExpressionBase CreateBindingExpressionOverride(DependencyObject target, DependencyProperty dp, BindingExpressionBase owner) { return PriorityBindingExpression.CreateBindingExpression(target, dp, this, owner); } private void OnBindingCollectionChanged() { CheckSealed(); } //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ BindingCollection _bindingCollection; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
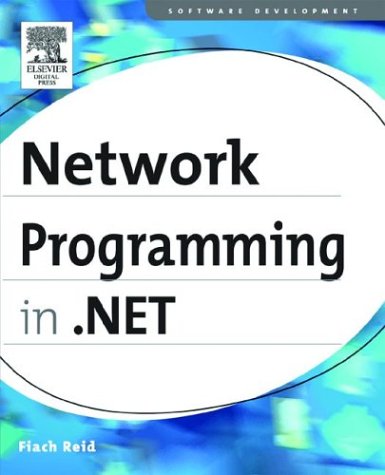
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- OSFeature.cs
- PermissionRequestEvidence.cs
- GeometryHitTestResult.cs
- FrameworkElementFactoryMarkupObject.cs
- SHA512.cs
- EmptyEnumerator.cs
- CounterCreationData.cs
- PolyBezierSegmentFigureLogic.cs
- StyleSelector.cs
- Error.cs
- HealthMonitoringSection.cs
- DeploymentExceptionMapper.cs
- AsyncOperationManager.cs
- Margins.cs
- _TLSstream.cs
- VerificationException.cs
- AssemblyResourceLoader.cs
- UICuesEvent.cs
- mda.cs
- TypeConverterAttribute.cs
- XAMLParseException.cs
- ObjectQueryExecutionPlan.cs
- PropertyMapper.cs
- RectangleGeometry.cs
- _ListenerRequestStream.cs
- BuiltInPermissionSets.cs
- ContactManager.cs
- IncrementalHitTester.cs
- DocumentViewerBaseAutomationPeer.cs
- WindowsGrip.cs
- HwndSourceKeyboardInputSite.cs
- ServicePointManager.cs
- PipelineModuleStepContainer.cs
- XsltLoader.cs
- DbParameterHelper.cs
- WorkflowDefinitionDispenser.cs
- SHA1CryptoServiceProvider.cs
- CompilerTypeWithParams.cs
- PackageRelationshipSelector.cs
- TemplateComponentConnector.cs
- GridView.cs
- SkewTransform.cs
- StatusStrip.cs
- DeflateStream.cs
- DetailsViewInsertEventArgs.cs
- DetailsViewPageEventArgs.cs
- AQNBuilder.cs
- ZipIOLocalFileHeader.cs
- XmlSchemaComplexContentExtension.cs
- PagerSettings.cs
- GuidTagList.cs
- WinFormsSpinner.cs
- ApplicationDirectory.cs
- XdrBuilder.cs
- Parameter.cs
- RegexTree.cs
- CodeNamespaceImport.cs
- Ref.cs
- AesCryptoServiceProvider.cs
- ComponentCollection.cs
- ProfileProvider.cs
- XmlReaderSettings.cs
- ProtectedConfigurationProviderCollection.cs
- ChtmlMobileTextWriter.cs
- StylusPlugin.cs
- PrintController.cs
- Publisher.cs
- DiagnosticsConfigurationHandler.cs
- unsafenativemethodstextservices.cs
- Attributes.cs
- Stopwatch.cs
- DataViewSettingCollection.cs
- ConfigurationManagerInternalFactory.cs
- SessionState.cs
- ZeroOpNode.cs
- WebPartHelpVerb.cs
- GlyphRun.cs
- MessageTraceRecord.cs
- GlyphRun.cs
- ArcSegment.cs
- X509AsymmetricSecurityKey.cs
- Timeline.cs
- ObfuscateAssemblyAttribute.cs
- ImmutableAssemblyCacheEntry.cs
- XmlElementAttribute.cs
- CapabilitiesAssignment.cs
- ObjectDataProvider.cs
- OrderedDictionary.cs
- HtmlFormWrapper.cs
- WebSysDisplayNameAttribute.cs
- HtmlInputHidden.cs
- PeerCollaborationPermission.cs
- PenThreadWorker.cs
- PrivateUnsafeNativeCompoundFileMethods.cs
- ToolStripTextBox.cs
- MetadataArtifactLoaderComposite.cs
- Rule.cs
- CustomValidator.cs
- RbTree.cs
- BasicExpressionVisitor.cs