Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / CommonUI / System / Drawing / Printing / PrintController.cs / 1 / PrintController.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Printing { using Microsoft.Win32; using System; using System.Diagnostics; using System.Drawing; using System.Runtime.InteropServices; using System.Security; using System.Security.Permissions; ////// /// public abstract class PrintController { // DEVMODEs are pretty expensive, so we cache one here and share it with the // Standard and Preview print controllers. If it weren't for all the rules about API changes, // I'd consider making this protected. internal IntPtr modeHandle = IntPtr.Zero; // initialized in StartPrint ///Controls how a document is printed. ////// /// protected PrintController() { IntSecurity.SafePrinting.Demand(); } ////// Initializes a new instance of the ///class. /// /// /// public virtual bool IsPreview { get{ return false; } } // WARNING: if you have nested PrintControllers, this method won't get called on the inner one. // Add initialization code to StartPrint or StartPage instead. internal void Print(PrintDocument document) { IntSecurity.SafePrinting.Demand(); // Most of the printing security is left to the individual print controller // // Get the PrintAction for this event PrintAction printAction; if (IsPreview) { printAction = PrintAction.PrintToPreview; } else { printAction = document.PrinterSettings.PrintToFile ? PrintAction.PrintToFile : PrintAction.PrintToPrinter; } // Check that user has permission to print to this particular printer PrintEventArgs printEvent = new PrintEventArgs(printAction); document._OnBeginPrint(printEvent); if (printEvent.Cancel) { document._OnEndPrint(printEvent); return; } OnStartPrint(document, printEvent); if (printEvent.Cancel) { document._OnEndPrint(printEvent); OnEndPrint(document, printEvent); return; } bool canceled = true; try { canceled = PrintLoop(document); } finally { try { try { document._OnEndPrint(printEvent); printEvent.Cancel = canceled | printEvent.Cancel; } finally { OnEndPrint(document, printEvent); } } finally { if (!IntSecurity.HasPermission(IntSecurity.AllPrinting)) { // Ensure programs with SafePrinting only get to print once for each time they // throw up the PrintDialog. IntSecurity.AllPrinting.Assert(); document.PrinterSettings.PrintDialogDisplayed = false; } } } } // Returns true if print was aborted. // WARNING: if you have nested PrintControllers, this method won't get called on the inner one // Add initialization code to StartPrint or StartPage instead. private bool PrintLoop(PrintDocument document) { QueryPageSettingsEventArgs queryEvent = new QueryPageSettingsEventArgs((PageSettings) document.DefaultPageSettings.Clone()); for (;;) { document._OnQueryPageSettings(queryEvent); if (queryEvent.Cancel) { return true; } PrintPageEventArgs pageEvent = CreatePrintPageEvent(queryEvent.PageSettings); Graphics graphics = OnStartPage(document, pageEvent); pageEvent.SetGraphics(graphics); try { document._OnPrintPage(pageEvent); OnEndPage(document, pageEvent); } finally { pageEvent.Dispose(); } if (pageEvent.Cancel) { return true; } else if (!pageEvent.HasMorePages) { return false; } else { // loop } } } private PrintPageEventArgs CreatePrintPageEvent(PageSettings pageSettings) { IntSecurity.AllPrintingAndUnmanagedCode.Assert(); Debug.Assert(modeHandle != IntPtr.Zero, "modeHandle is null. Someone must have forgot to call base.StartPrint"); Rectangle pageBounds = pageSettings.GetBounds(modeHandle); Rectangle marginBounds = new Rectangle(pageSettings.Margins.Left, pageSettings.Margins.Top, pageBounds.Width - (pageSettings.Margins.Left + pageSettings.Margins.Right), pageBounds.Height - (pageSettings.Margins.Top + pageSettings.Margins.Bottom)); PrintPageEventArgs pageEvent = new PrintPageEventArgs(null, marginBounds, pageBounds, pageSettings); return pageEvent; } ////// This is new public property which notifies if this controller is used for PrintPreview. /// ////// /// public virtual void OnStartPrint(PrintDocument document, PrintEventArgs e) { IntSecurity.AllPrintingAndUnmanagedCode.Assert(); modeHandle = document.PrinterSettings.GetHdevmode(document.DefaultPageSettings); } ///When overridden in a derived class, begins the control sequence of when and how to print a document. ////// /// public virtual Graphics OnStartPage(PrintDocument document, PrintPageEventArgs e) { return null; } ///When overridden in a derived class, begins the control /// sequence of when and how to print a page in a document. ////// /// public virtual void OnEndPage(PrintDocument document, PrintPageEventArgs e) { } ///When overridden in a derived class, completes the control sequence of when and how /// to print a page in a document. ////// /// public virtual void OnEndPrint(PrintDocument document, PrintEventArgs e) { IntSecurity.UnmanagedCode.Assert(); Debug.Assert(modeHandle != IntPtr.Zero, "modeHandle is null. Someone must have forgot to call base.StartPrint"); SafeNativeMethods.GlobalFree(new HandleRef(this, modeHandle)); modeHandle = IntPtr.Zero; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //When overridden in a derived class, completes the /// control sequence of when and how to print a document. ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Printing { using Microsoft.Win32; using System; using System.Diagnostics; using System.Drawing; using System.Runtime.InteropServices; using System.Security; using System.Security.Permissions; ////// /// public abstract class PrintController { // DEVMODEs are pretty expensive, so we cache one here and share it with the // Standard and Preview print controllers. If it weren't for all the rules about API changes, // I'd consider making this protected. internal IntPtr modeHandle = IntPtr.Zero; // initialized in StartPrint ///Controls how a document is printed. ////// /// protected PrintController() { IntSecurity.SafePrinting.Demand(); } ////// Initializes a new instance of the ///class. /// /// /// public virtual bool IsPreview { get{ return false; } } // WARNING: if you have nested PrintControllers, this method won't get called on the inner one. // Add initialization code to StartPrint or StartPage instead. internal void Print(PrintDocument document) { IntSecurity.SafePrinting.Demand(); // Most of the printing security is left to the individual print controller // // Get the PrintAction for this event PrintAction printAction; if (IsPreview) { printAction = PrintAction.PrintToPreview; } else { printAction = document.PrinterSettings.PrintToFile ? PrintAction.PrintToFile : PrintAction.PrintToPrinter; } // Check that user has permission to print to this particular printer PrintEventArgs printEvent = new PrintEventArgs(printAction); document._OnBeginPrint(printEvent); if (printEvent.Cancel) { document._OnEndPrint(printEvent); return; } OnStartPrint(document, printEvent); if (printEvent.Cancel) { document._OnEndPrint(printEvent); OnEndPrint(document, printEvent); return; } bool canceled = true; try { canceled = PrintLoop(document); } finally { try { try { document._OnEndPrint(printEvent); printEvent.Cancel = canceled | printEvent.Cancel; } finally { OnEndPrint(document, printEvent); } } finally { if (!IntSecurity.HasPermission(IntSecurity.AllPrinting)) { // Ensure programs with SafePrinting only get to print once for each time they // throw up the PrintDialog. IntSecurity.AllPrinting.Assert(); document.PrinterSettings.PrintDialogDisplayed = false; } } } } // Returns true if print was aborted. // WARNING: if you have nested PrintControllers, this method won't get called on the inner one // Add initialization code to StartPrint or StartPage instead. private bool PrintLoop(PrintDocument document) { QueryPageSettingsEventArgs queryEvent = new QueryPageSettingsEventArgs((PageSettings) document.DefaultPageSettings.Clone()); for (;;) { document._OnQueryPageSettings(queryEvent); if (queryEvent.Cancel) { return true; } PrintPageEventArgs pageEvent = CreatePrintPageEvent(queryEvent.PageSettings); Graphics graphics = OnStartPage(document, pageEvent); pageEvent.SetGraphics(graphics); try { document._OnPrintPage(pageEvent); OnEndPage(document, pageEvent); } finally { pageEvent.Dispose(); } if (pageEvent.Cancel) { return true; } else if (!pageEvent.HasMorePages) { return false; } else { // loop } } } private PrintPageEventArgs CreatePrintPageEvent(PageSettings pageSettings) { IntSecurity.AllPrintingAndUnmanagedCode.Assert(); Debug.Assert(modeHandle != IntPtr.Zero, "modeHandle is null. Someone must have forgot to call base.StartPrint"); Rectangle pageBounds = pageSettings.GetBounds(modeHandle); Rectangle marginBounds = new Rectangle(pageSettings.Margins.Left, pageSettings.Margins.Top, pageBounds.Width - (pageSettings.Margins.Left + pageSettings.Margins.Right), pageBounds.Height - (pageSettings.Margins.Top + pageSettings.Margins.Bottom)); PrintPageEventArgs pageEvent = new PrintPageEventArgs(null, marginBounds, pageBounds, pageSettings); return pageEvent; } ////// This is new public property which notifies if this controller is used for PrintPreview. /// ////// /// public virtual void OnStartPrint(PrintDocument document, PrintEventArgs e) { IntSecurity.AllPrintingAndUnmanagedCode.Assert(); modeHandle = document.PrinterSettings.GetHdevmode(document.DefaultPageSettings); } ///When overridden in a derived class, begins the control sequence of when and how to print a document. ////// /// public virtual Graphics OnStartPage(PrintDocument document, PrintPageEventArgs e) { return null; } ///When overridden in a derived class, begins the control /// sequence of when and how to print a page in a document. ////// /// public virtual void OnEndPage(PrintDocument document, PrintPageEventArgs e) { } ///When overridden in a derived class, completes the control sequence of when and how /// to print a page in a document. ////// /// public virtual void OnEndPrint(PrintDocument document, PrintEventArgs e) { IntSecurity.UnmanagedCode.Assert(); Debug.Assert(modeHandle != IntPtr.Zero, "modeHandle is null. Someone must have forgot to call base.StartPrint"); SafeNativeMethods.GlobalFree(new HandleRef(this, modeHandle)); modeHandle = IntPtr.Zero; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.When overridden in a derived class, completes the /// control sequence of when and how to print a document. ///
Link Menu
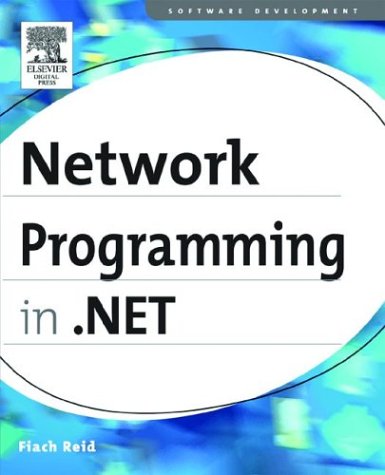
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TemplateModeChangedEventArgs.cs
- DbDataReader.cs
- NavigationService.cs
- AutomationFocusChangedEventArgs.cs
- TypeGenericEnumerableViewSchema.cs
- RemotingServices.cs
- CheckBoxAutomationPeer.cs
- FileDetails.cs
- DiscoveryVersion.cs
- ConfigXmlSignificantWhitespace.cs
- SymbolTable.cs
- HashAlgorithm.cs
- PolicyLevel.cs
- SourceItem.cs
- StringWriter.cs
- ScriptingJsonSerializationSection.cs
- ChildTable.cs
- CqlLexer.cs
- returneventsaver.cs
- NegatedCellConstant.cs
- XmlSerializationGeneratedCode.cs
- GridViewColumnCollectionChangedEventArgs.cs
- ToolStripContentPanelDesigner.cs
- Base64Decoder.cs
- StrokeNodeOperations2.cs
- StorageComplexPropertyMapping.cs
- ViewGenResults.cs
- WorkflowApplicationEventArgs.cs
- SQLSingle.cs
- XmlTextReader.cs
- CommonDialog.cs
- DesignOnlyAttribute.cs
- FixedTextSelectionProcessor.cs
- AnnotationHighlightLayer.cs
- CodeGroup.cs
- AnonymousIdentificationModule.cs
- TransformGroup.cs
- DetailsView.cs
- AllMembershipCondition.cs
- Match.cs
- SystemDiagnosticsSection.cs
- AuthorizationRuleCollection.cs
- FloaterBaseParagraph.cs
- FormViewUpdateEventArgs.cs
- ImageSource.cs
- LambdaCompiler.cs
- WebRequest.cs
- ListCollectionView.cs
- HandlerFactoryWrapper.cs
- DbConnectionPoolCounters.cs
- SettingsPropertyWrongTypeException.cs
- DependencyObjectType.cs
- ItemsPresenter.cs
- SurrogateEncoder.cs
- ScaleTransform3D.cs
- ResXDataNode.cs
- BuildProviderAppliesToAttribute.cs
- InteropAutomationProvider.cs
- PerfCounterSection.cs
- SimpleColumnProvider.cs
- ParallelDesigner.xaml.cs
- ComplexType.cs
- TransformProviderWrapper.cs
- CompilerTypeWithParams.cs
- ChtmlTextWriter.cs
- FileFormatException.cs
- InputLanguageManager.cs
- CodeTypeParameterCollection.cs
- FunctionNode.cs
- SafeBuffer.cs
- SqlDuplicator.cs
- FileDialog_Vista_Interop.cs
- XmlNamespaceManager.cs
- RSAPKCS1SignatureFormatter.cs
- ProfileBuildProvider.cs
- coordinator.cs
- InfoCardAsymmetricCrypto.cs
- ReservationNotFoundException.cs
- GraphicsPath.cs
- IisTraceListener.cs
- ExtensionSimplifierMarkupObject.cs
- SqlInfoMessageEvent.cs
- ThreadStateException.cs
- OutgoingWebResponseContext.cs
- ScrollData.cs
- ImmutableObjectAttribute.cs
- ReadOnlyCollectionBase.cs
- ZipIOCentralDirectoryBlock.cs
- DocumentGrid.cs
- ListViewGroupConverter.cs
- NativeMethods.cs
- DataTableMapping.cs
- MatrixConverter.cs
- FixedSOMPage.cs
- OdbcHandle.cs
- PrtTicket_Public.cs
- Crc32.cs
- CodeBinaryOperatorExpression.cs
- DataGridViewImageCell.cs
- MessageAction.cs