Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / CompMod / System / Diagnostics / SystemDiagnosticsSection.cs / 1 / SystemDiagnosticsSection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System.Configuration; namespace System.Diagnostics { internal class SystemDiagnosticsSection : ConfigurationSection { private static readonly ConfigurationPropertyCollection _properties; private static readonly ConfigurationProperty _propAssert = new ConfigurationProperty("assert", typeof(AssertSection), new AssertSection(), ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propPerfCounters = new ConfigurationProperty("performanceCounters", typeof(PerfCounterSection), new PerfCounterSection(), ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propSources = new ConfigurationProperty("sources", typeof(SourceElementsCollection), new SourceElementsCollection(), ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propSharedListeners = new ConfigurationProperty("sharedListeners", typeof(SharedListenerElementsCollection), new SharedListenerElementsCollection(), ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propSwitches = new ConfigurationProperty("switches", typeof(SwitchElementsCollection), new SwitchElementsCollection(), ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propTrace = new ConfigurationProperty("trace", typeof(TraceSection), new TraceSection(), ConfigurationPropertyOptions.None); static SystemDiagnosticsSection() { _properties = new ConfigurationPropertyCollection(); _properties.Add(_propAssert); _properties.Add(_propPerfCounters); _properties.Add(_propSources); _properties.Add(_propSharedListeners); _properties.Add(_propSwitches); _properties.Add(_propTrace); } [ConfigurationProperty("assert")] public AssertSection Assert { get { return (AssertSection) base[_propAssert]; } } [ConfigurationProperty("performanceCounters")] public PerfCounterSection PerfCounters { get { return (PerfCounterSection) base[_propPerfCounters]; } } protected override ConfigurationPropertyCollection Properties { get { return _properties; } } [ConfigurationProperty("sources")] public SourceElementsCollection Sources { get { return (SourceElementsCollection ) base[_propSources]; } } [ConfigurationProperty("sharedListeners")] public ListenerElementsCollection SharedListeners { get { return (ListenerElementsCollection) base[_propSharedListeners]; } } [ConfigurationProperty("switches")] public SwitchElementsCollection Switches { get { return (SwitchElementsCollection) base[_propSwitches]; } } [ConfigurationProperty("trace")] public TraceSection Trace { get { return (TraceSection) base[_propTrace]; } } protected override void InitializeDefault() { Trace.Listeners.InitializeDefaultInternal(); } } }
Link Menu
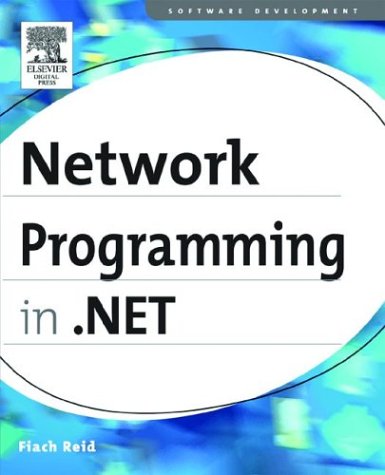
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AutomationPattern.cs
- HttpGetServerProtocol.cs
- DESCryptoServiceProvider.cs
- DynamicActivity.cs
- AttributeConverter.cs
- DefaultAsyncDataDispatcher.cs
- ObjectStateManager.cs
- TagMapInfo.cs
- AnimationClockResource.cs
- ProxyWebPartManager.cs
- AspCompat.cs
- MenuItemBindingCollection.cs
- ClientData.cs
- HybridDictionary.cs
- _SafeNetHandles.cs
- RenderTargetBitmap.cs
- GetIndexBinder.cs
- PathFigureCollection.cs
- ExpressionBinding.cs
- StringCollectionMarkupSerializer.cs
- DataColumn.cs
- DataKeyPropertyAttribute.cs
- PropertyExpression.cs
- ClosableStream.cs
- ExpandSegment.cs
- DataGridViewCellStyleConverter.cs
- HitTestDrawingContextWalker.cs
- FileDialog_Vista.cs
- _ScatterGatherBuffers.cs
- DefaultTraceListener.cs
- XmlDataDocument.cs
- IPAddress.cs
- ConfigXmlComment.cs
- ButtonBase.cs
- CapacityStreamGeometryContext.cs
- XmlHierarchicalDataSourceView.cs
- DataSourceCache.cs
- Semaphore.cs
- StateDesignerConnector.cs
- SqlBinder.cs
- SafeBitVector32.cs
- login.cs
- MsmqIntegrationInputChannel.cs
- SoapCodeExporter.cs
- XamlStream.cs
- TemplateBamlRecordReader.cs
- ConnectionProviderAttribute.cs
- StringCollection.cs
- DataGridViewSortCompareEventArgs.cs
- ConcatQueryOperator.cs
- XmlAttributeHolder.cs
- CreateParams.cs
- ReadOnlyPropertyMetadata.cs
- SessionEndingCancelEventArgs.cs
- DeriveBytes.cs
- NativeMethods.cs
- SqlDataSourceSelectingEventArgs.cs
- ApplicationCommands.cs
- QueryableFilterUserControl.cs
- SoapIncludeAttribute.cs
- LocalIdCollection.cs
- JapaneseLunisolarCalendar.cs
- VectorCollectionConverter.cs
- CodeAttachEventStatement.cs
- PenLineJoinValidation.cs
- WorkflowPageSetupDialog.cs
- PropertyDescriptorCollection.cs
- HttpCapabilitiesEvaluator.cs
- TraceUtility.cs
- COM2AboutBoxPropertyDescriptor.cs
- ContentTextAutomationPeer.cs
- Rotation3D.cs
- Compiler.cs
- RepeatButton.cs
- AnimationStorage.cs
- XmlNodeChangedEventArgs.cs
- ListManagerBindingsCollection.cs
- SqlMultiplexer.cs
- ModelPropertyImpl.cs
- MethodImplAttribute.cs
- DecoratedNameAttribute.cs
- Duration.cs
- BindingList.cs
- PointAnimationClockResource.cs
- InkCanvasFeedbackAdorner.cs
- DocumentViewerConstants.cs
- BaseTemplateCodeDomTreeGenerator.cs
- SettingsPropertyValue.cs
- SystemInformation.cs
- CorrelationResolver.cs
- StrokeIntersection.cs
- AnnotationElement.cs
- PropertyItemInternal.cs
- SoapSchemaImporter.cs
- TypeInfo.cs
- ValidatedControlConverter.cs
- ProfessionalColorTable.cs
- BooleanStorage.cs
- TimelineClockCollection.cs
- SemanticAnalyzer.cs