Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / DynamicData / DynamicData / QueryableFilterUserControl.cs / 1305376 / QueryableFilterUserControl.cs
using System.Web.UI; using System.Web.UI.WebControls; using System.Diagnostics.CodeAnalysis; using System.Linq; using System.Collections.Generic; using System.Linq.Expressions; using System.Diagnostics; using System.Collections; #if !ORYX_VNEXT using System.Web.UI.WebControls.Expressions; using System.Web.DynamicData.Util; #else using Microsoft.Web.Data.UI.WebControls.Expressions; using Microsoft.Web.Data.UI.WebControls; using System.Web.DynamicData.Util; #endif namespace System.Web.DynamicData { public abstract class QueryableFilterUserControl : UserControl { private HttpContextBase _context; private DefaultValueMapping _defaultValueMapping; // internal for unit tests internal protected MetaColumn Column { get; private set; } private IQueryableDataSource QueryableDataSource { get; set; } public abstract IQueryable GetQueryable(IQueryable source); internal void Initialize(MetaColumn column, IQueryableDataSource iQueryableDataSource, HttpContextBase context) { QueryableDataSource = iQueryableDataSource; Column = column; _context = context ?? new HttpContextWrapper(Context); } public event EventHandler FilterChanged; ////// Returns the data control that handles the filter inside the filter template. Can be null if the filter template does not override it. /// public virtual Control FilterControl { get { return null; } } ////// Populate a ListControl with all the items in the foreign table (or true/false for boolean fields) /// /// public void PopulateListControl(ListControl listControl) { Type enumType; if (Column is MetaForeignKeyColumn) { MetaTable FilterTable = ((MetaForeignKeyColumn)Column).ParentTable; Misc.FillListItemCollection(FilterTable, listControl.Items); } else if (Column.IsEnumType(out enumType)) { Debug.Assert(enumType != null); FillEnumListControl(listControl, enumType); } } private void FillEnumListControl(ListControl list, Type enumType) { foreach (DictionaryEntry entry in Misc.GetEnumNamesAndValues(enumType)) { list.Items.Add(new ListItem((string)entry.Key, (string)entry.Value)); } } ////// Raises the FilterChanged event. This is necessary to notify the data source that the filter selection /// has changed and the query needs to be reevaluated. /// protected void OnFilterChanged() { // Clear the default value for this column ClearDefaultValues(); EventHandler eventHandler = FilterChanged; if (eventHandler != null) { eventHandler(this, EventArgs.Empty); } QueryableDataSource.RaiseViewChanged(); } private void ClearDefaultValues() { IDictionarydefaultValues = DefaultValues; if (defaultValues != null) { MetaForeignKeyColumn foreignKeyColumn = Column as MetaForeignKeyColumn; if (foreignKeyColumn != null) { foreach (var fkName in foreignKeyColumn.ForeignKeyNames) { defaultValues.Remove(fkName); } } else { defaultValues.Remove(Column.Name); } } } public string DefaultValue { get { IDictionary defaultValues = DefaultValues; if (defaultValues == null || !DefaultValueMapping.Contains(Column)) { return null; } MetaForeignKeyColumn foreignKeyColumn = Column as MetaForeignKeyColumn; if (foreignKeyColumn != null) { return foreignKeyColumn.GetForeignKeyString(DefaultValueMapping.Instance); } object value; if (defaultValues.TryGetValue(Column.Name, out value)) { return Misc.ChangeType (value); } return null; } } public IDictionary DefaultValues { get { // Get the default values mapped for this datasource if any if (DefaultValueMapping != null) { return DefaultValueMapping.Values; } return null; } } private DefaultValueMapping DefaultValueMapping { get { if (_defaultValueMapping == null) { Debug.Assert(_context != null); _defaultValueMapping = DynamicDataExtensions.GetDefaultValueMapping(QueryableDataSource, _context); } return _defaultValueMapping; } } // Method copied from ExpressionHelper private static Expression CreatePropertyExpression(Expression parameterExpression, string propertyName) { Expression propExpression = null; string[] props = propertyName.Split('.'); foreach (var p in props) { if (propExpression == null) { propExpression = Expression.PropertyOrField(parameterExpression, p); } else { propExpression = Expression.PropertyOrField(propExpression, p); } } return propExpression; } public static IQueryable ApplyEqualityFilter(IQueryable source, string propertyName, object value) { ParameterExpression parameterExpression = Expression.Parameter(source.ElementType, String.Empty); Expression propertyExpression = CreatePropertyExpression(parameterExpression, propertyName); if (Nullable.GetUnderlyingType(propertyExpression.Type) != null && value != null) { propertyExpression = Expression.Convert(propertyExpression, Misc.RemoveNullableFromType(propertyExpression.Type)); } value = Misc.ChangeType(value, propertyExpression.Type); Expression compareExpression = Expression.Equal(propertyExpression, Expression.Constant(value)); LambdaExpression lambda = Expression.Lambda(compareExpression, parameterExpression); MethodCallExpression whereCall = Expression.Call(typeof(Queryable), "Where", new Type[] { source.ElementType }, source.Expression, Expression.Quote(lambda)); return source.Provider.CreateQuery(whereCall); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
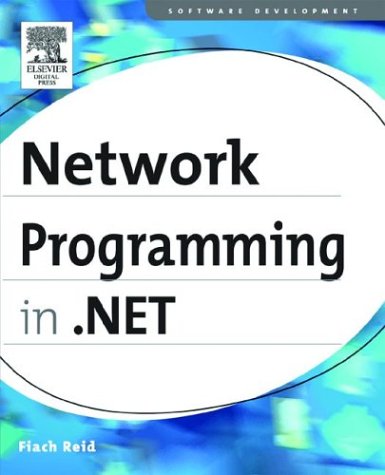
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PropertyValueUIItem.cs
- ArrayList.cs
- DataGridViewRowPrePaintEventArgs.cs
- StructuralType.cs
- ConfigurationCollectionAttribute.cs
- BufferAllocator.cs
- ProxyWebPartConnectionCollection.cs
- InheritanceRules.cs
- PropertyItem.cs
- ColorMap.cs
- ComAdminInterfaces.cs
- PerformanceCounter.cs
- SecurityCriticalDataForSet.cs
- BindingCompleteEventArgs.cs
- MaterialGroup.cs
- DataGridColumnStyleMappingNameEditor.cs
- WindowAutomationPeer.cs
- TemplatedMailWebEventProvider.cs
- DataPagerFieldCommandEventArgs.cs
- UnsafeNativeMethods.cs
- VariableBinder.cs
- StyleXamlParser.cs
- ScriptHandlerFactory.cs
- SpoolingTask.cs
- RIPEMD160Managed.cs
- InputMethod.cs
- HttpClientCertificate.cs
- PathData.cs
- TreeView.cs
- XmlSchemaGroup.cs
- WindowHelperService.cs
- XsdValidatingReader.cs
- SoapAttributeAttribute.cs
- PeerContact.cs
- Executor.cs
- UnsafeNativeMethods.cs
- DoubleIndependentAnimationStorage.cs
- DesignerGenericWebPart.cs
- SkipQueryOptionExpression.cs
- ArcSegment.cs
- TimelineCollection.cs
- WebScriptMetadataMessageEncoderFactory.cs
- Attributes.cs
- StagingAreaInputItem.cs
- NativeMethods.cs
- UIElement.cs
- OrderedDictionaryStateHelper.cs
- PathFigure.cs
- DataGridSortCommandEventArgs.cs
- CompoundFileReference.cs
- BamlRecordHelper.cs
- Win32SafeHandles.cs
- BinaryWriter.cs
- storepermission.cs
- LineMetrics.cs
- Baml2006ReaderSettings.cs
- SchemaImporterExtensionElement.cs
- _TLSstream.cs
- ASCIIEncoding.cs
- BitmapSourceSafeMILHandle.cs
- DataControlImageButton.cs
- GifBitmapDecoder.cs
- SizeF.cs
- IItemProperties.cs
- TreeNodeConverter.cs
- CollectionViewGroupRoot.cs
- TagPrefixCollection.cs
- SynchronizingStream.cs
- OdbcCommand.cs
- LayoutEditorPart.cs
- RichTextBox.cs
- RequiredAttributeAttribute.cs
- smtpconnection.cs
- CorrelationHandle.cs
- ProviderConnectionPointCollection.cs
- ParameterExpression.cs
- TypeUsageBuilder.cs
- HtmlElement.cs
- ValidatorAttribute.cs
- HMACSHA512.cs
- CompositeDataBoundControl.cs
- SmtpDigestAuthenticationModule.cs
- KnownAssemblyEntry.cs
- DataMisalignedException.cs
- DbResourceAllocator.cs
- UpdateTracker.cs
- wgx_exports.cs
- RequestTimeoutManager.cs
- WebServiceEnumData.cs
- QueryExtender.cs
- AuthenticationManager.cs
- LinqTreeNodeEvaluator.cs
- Stacktrace.cs
- SatelliteContractVersionAttribute.cs
- ConnectionProviderAttribute.cs
- SubMenuStyleCollection.cs
- XPathNodeInfoAtom.cs
- IBuiltInEvidence.cs
- ConnectionPoint.cs
- SoapException.cs