Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Input / StagingAreaInputItem.cs / 1407647 / StagingAreaInputItem.cs
using System; using System.Collections; using System.Security.Permissions; using System.Security; namespace System.Windows.Input { ////// This class encapsulates an input event while it is being /// processed by the input manager. /// ////// This class just provides the dictionary-based storage for /// all of the listeners of the various input manager events. /// public class StagingAreaInputItem { // Only we can make these. internal StagingAreaInputItem(bool isMarker) { _isMarker = isMarker; } // For performace reasons, we try to reuse these event args. // Allow an existing item to be promoted by keeping the existing dictionary. internal void Reset(InputEventArgs input, StagingAreaInputItem promote) { _input = input; if(promote != null && promote._dictionary != null) { // _dictionary = (Hashtable) promote._dictionary.Clone(); } else { if(_dictionary != null) { _dictionary.Clear(); } else { _dictionary = new Hashtable(); } } } ////// Returns the input event. /// ////// Callers must have UIPermission(PermissionState.Unrestricted) to call this API. /// ////// Defense In Depth - even if this leaks out, we demand here. /// Critical - Performs a Link Demand. The reason these methods are marked critical /// is that security transparent code should not be responsible for verifying /// the security of an operation, and therefore should not be protected from partial /// trust callers with LinkDemands. /// public InputEventArgs Input { [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] get {return _input;} } ////// Provides storage for arbitrary data needed during the /// processing of this input event. /// /// /// An arbitrary key for the data. This cannot be null. /// ////// The data previously set for this key, or null. /// public object GetData(object key) { return _dictionary[key]; } ////// Provides storage for arbitrary data needed during the /// processing of this input event. /// /// /// An arbitrary key for the data. This cannot be null. /// /// /// The data to set for this key. This can be null. /// ////// Callers must have UIPermission(PermissionState.Unrestricted) to call this API. /// ////// Defense In Depth - even if this leaks out, we demand here. /// Critical - Performs a Link Demand. The reason these methods are marked critical /// is that security transparent code should not be responsible for verifying /// the security of an operation, and therefore should not be protected from partial /// trust callers with LinkDemands. /// [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] public void SetData(object key, object value) { _dictionary[key] = value; } internal bool IsMarker {get {return _isMarker;}} private bool _isMarker; private InputEventArgs _input; private Hashtable _dictionary; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
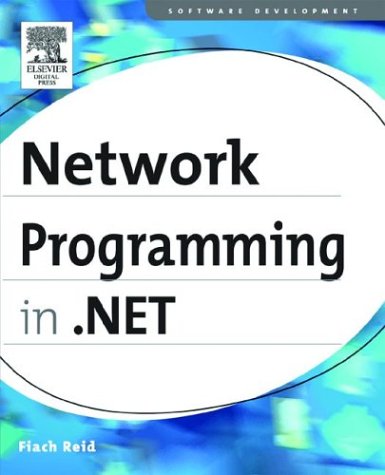
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MailAddressCollection.cs
- RangeBaseAutomationPeer.cs
- HyperLinkField.cs
- ContainerParagraph.cs
- XmlSignatureProperties.cs
- ObjectDataSourceMethodEditor.cs
- AudioFileOut.cs
- MaskedTextBox.cs
- ToolboxItem.cs
- HistoryEventArgs.cs
- XPathEmptyIterator.cs
- Binding.cs
- NavigationEventArgs.cs
- Typography.cs
- ControlUtil.cs
- WebContext.cs
- Validator.cs
- datacache.cs
- ColorTransformHelper.cs
- NullRuntimeConfig.cs
- WindowsFormsSynchronizationContext.cs
- Funcletizer.cs
- RangeContentEnumerator.cs
- FixedPageAutomationPeer.cs
- WebPartChrome.cs
- RemoteWebConfigurationHostServer.cs
- ColumnWidthChangedEvent.cs
- ValueTable.cs
- WinCategoryAttribute.cs
- DropShadowBitmapEffect.cs
- InternalRelationshipCollection.cs
- TextEditorCharacters.cs
- _BasicClient.cs
- LinkTarget.cs
- ObjectDisposedException.cs
- ReferentialConstraintRoleElement.cs
- SHA256CryptoServiceProvider.cs
- ArrayItemReference.cs
- EpmTargetTree.cs
- DataGridViewTextBoxColumn.cs
- AttributeCollection.cs
- _CommandStream.cs
- RangeContentEnumerator.cs
- BamlLocalizableResourceKey.cs
- MultiTargetingUtil.cs
- XmlSiteMapProvider.cs
- _AuthenticationState.cs
- CustomMenuItemCollection.cs
- BuildProviderCollection.cs
- CompilationRelaxations.cs
- BinHexEncoder.cs
- ObjectListShowCommandsEventArgs.cs
- ClientTargetSection.cs
- AssociationSetMetadata.cs
- _NegoState.cs
- DataGridLength.cs
- KeyValuePairs.cs
- InputLanguageManager.cs
- DrawingContextWalker.cs
- ManagedWndProcTracker.cs
- SecurityException.cs
- QilTypeChecker.cs
- TableCell.cs
- ZipArchive.cs
- TextEffect.cs
- SignedXml.cs
- BufferAllocator.cs
- InternalSafeNativeMethods.cs
- MapPathBasedVirtualPathProvider.cs
- NonPrimarySelectionGlyph.cs
- FormViewUpdateEventArgs.cs
- VarRefManager.cs
- COM2AboutBoxPropertyDescriptor.cs
- PropertiesTab.cs
- JobDuplex.cs
- TdsEnums.cs
- RuleSettingsCollection.cs
- HttpConfigurationContext.cs
- FileLogRecordEnumerator.cs
- KernelTypeValidation.cs
- messageonlyhwndwrapper.cs
- CmsInterop.cs
- EncryptedXml.cs
- DbConnectionPool.cs
- DecoderFallbackWithFailureFlag.cs
- ExecutorLocksHeldException.cs
- RuleSetReference.cs
- OdbcCommand.cs
- SourceFilter.cs
- LayoutTable.cs
- XamlLoadErrorInfo.cs
- RuleInfoComparer.cs
- MediaElement.cs
- DataControlFieldTypeEditor.cs
- BinaryObjectWriter.cs
- PeerNeighborManager.cs
- RadioButtonFlatAdapter.cs
- ISAPIWorkerRequest.cs
- RMEnrollmentPage2.cs
- ConvertBinder.cs