Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Internal / Synthesis / AudioFileOut.cs / 1 / AudioFileOut.cs
//------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // // // This class defines the header used to identify a waveform-audio // buffer. // // History: // 2/1/2005 [....] Created from the Sapi Managed code //----------------------------------------------------------------- using System; using System.IO; using System.Speech.AudioFormat; using System.Threading; using System.Diagnostics; namespace System.Speech.Internal.Synthesis { ////// Encapsulates Waveform Audio Interface playback functions and provides a simple /// interface for playing audio. /// internal class AudioFileOut : AudioBase, IDisposable { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors ////// Create an instance of AudioFileOut. /// internal AudioFileOut (Stream stream, SpeechAudioFormatInfo formatInfo, bool headerInfo, IAsyncDispatch asyncDispatch) { _asyncDispatch = asyncDispatch; _stream = stream; _startStreamPosition = _stream.Position; _hasHeader = headerInfo; _wfxOut = new WAVEFORMATEX (); // if we have a formatInfo object, format conversion may be necessary if (formatInfo != null) { // Build the Wave format from the formatInfo _wfxOut.wFormatTag = (short) formatInfo.EncodingFormat; _wfxOut.wBitsPerSample = (short) formatInfo.BitsPerSample; _wfxOut.nSamplesPerSec = formatInfo.SamplesPerSecond; _wfxOut.nChannels = (short) formatInfo.ChannelCount; } else { // Set the default values _wfxOut = WAVEFORMATEX.Default; } _wfxOut.nBlockAlign = (short) (_wfxOut.nChannels * _wfxOut.wBitsPerSample / 8); _wfxOut.nAvgBytesPerSec = _wfxOut.wBitsPerSample * _wfxOut.nSamplesPerSec * _wfxOut.nChannels / 8; } public void Dispose () { _evt.Close (); } #endregion //******************************************************************** // // Internal Methods // //******************************************************************* #region Internal Methods ////// Begin to play /// /// override internal void Begin (byte [] wfx) { if (_deviceOpen) { System.Diagnostics.Debug.Assert (false); throw new InvalidOperationException (); } // Get the audio format if conversion is needed _wfxIn = WAVEFORMATEX.ToWaveHeader (wfx); _doConversion = _pcmConverter.PrepareConverter (ref _wfxIn, ref _wfxOut); if (_totalByteWrittens == 0 && _hasHeader) { WriteWaveHeader (_stream, _wfxOut, _startStreamPosition, 0); } _bytesWritten = 0; // set the flags _aborted = false; _deviceOpen = true; } ////// Begin to play /// override internal void End () { if (!_deviceOpen) { System.Diagnostics.Debug.Assert (false); throw new InvalidOperationException (); } _deviceOpen = false; if (!_aborted) { if (_hasHeader) { long position = _stream.Position; WriteWaveHeader (_stream, _wfxOut, _startStreamPosition, _totalByteWrittens); _stream.Seek (position, SeekOrigin.Begin); } } } #region AudioDevice implementation ////// Play a wave file. /// /// override internal void Play (byte [] buffer) { if (!_deviceOpen) { System.Diagnostics.Debug.Assert (false); } else { byte [] abOut = _doConversion ? _pcmConverter.ConvertSamples (buffer) : buffer; if (_paused) { _evt.WaitOne (); _evt.Reset (); } if (!_aborted) { _stream.Write (abOut, 0, abOut.Length); _totalByteWrittens += abOut.Length; _bytesWritten += abOut.Length; } } } ////// Pause the playback of a sound. /// ///MMSYSERR.NOERROR if successful override internal void Pause () { if (!_aborted && !_paused) { lock (_noWriteOutLock) { _paused = true; } } } ////// Resume the playback of a paused sound. /// ///MMSYSERR.NOERROR if successful override internal void Resume () { if (!_aborted && _paused) { lock (_noWriteOutLock) { _paused = false; _evt.Set (); } } } ////// Wait for all the queued buffers to be played /// override internal void Abort () { lock (_noWriteOutLock) { _aborted = true; _paused = false; _evt.Set (); } } override internal void InjectEvent (TTSEvent ttsEvent) { if (!_aborted && _asyncDispatch != null) { _asyncDispatch.Post (ttsEvent); } } ////// File operation are basically synchonous /// override internal void WaitUntilDone () { lock (_noWriteOutLock) { } } #endregion #endregion //******************************************************************** // // Internal Fields // //******************************************************************** #region Internal Fields override internal TimeSpan Duration { get { if (_wfxIn.nAvgBytesPerSec == 0) { return new TimeSpan (0); } return new TimeSpan ((_bytesWritten * TimeSpan.TicksPerSecond) / _wfxIn.nAvgBytesPerSec); } } override internal long Position { get { return _stream.Position; } } internal override byte [] WaveFormat { get { return _wfxOut.ToBytes (); } } #endregion //******************************************************************* // // Protected Fields // //******************************************************************** #region Private Fields protected ManualResetEvent _evt = new ManualResetEvent (false); protected bool _deviceOpen; protected Stream _stream; protected PcmConverter _pcmConverter = new PcmConverter (); protected bool _doConversion; protected bool _paused; protected int _totalByteWrittens; protected int _bytesWritten; #endregion //******************************************************************* // // Private Fields // //******************************************************************* #region Private Fields private IAsyncDispatch _asyncDispatch; private object _noWriteOutLock = new object (); private WAVEFORMATEX _wfxIn; private WAVEFORMATEX _wfxOut; private bool _hasHeader; private long _startStreamPosition; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
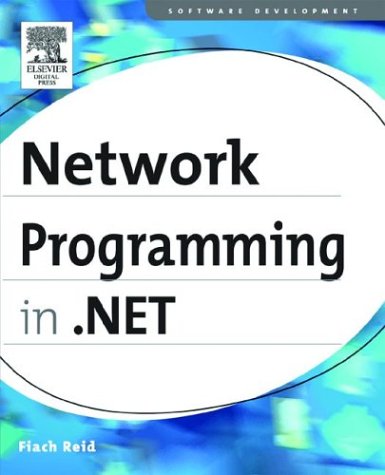
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HttpServerVarsCollection.cs
- SetStateDesigner.cs
- SQLString.cs
- DataListCommandEventArgs.cs
- ProjectionCamera.cs
- GeometryGroup.cs
- NonVisualControlAttribute.cs
- PackageDocument.cs
- OrderByQueryOptionExpression.cs
- PointCollection.cs
- SAPICategories.cs
- KeySpline.cs
- SqlRecordBuffer.cs
- GridViewHeaderRowPresenterAutomationPeer.cs
- ColumnPropertiesGroup.cs
- TemplateBindingExpression.cs
- KerberosTicketHashIdentifierClause.cs
- AssemblyGen.cs
- LateBoundBitmapDecoder.cs
- RequestCacheEntry.cs
- KoreanCalendar.cs
- Mutex.cs
- ProviderSettings.cs
- SolidColorBrush.cs
- WebPartExportVerb.cs
- ContentHostHelper.cs
- OracleInternalConnection.cs
- DropDownList.cs
- KeyEventArgs.cs
- PageEventArgs.cs
- WsatExtendedInformation.cs
- SharedHttpsTransportManager.cs
- Accessible.cs
- LogicalExpr.cs
- ProviderBase.cs
- TraceRecord.cs
- TemplateControlCodeDomTreeGenerator.cs
- PropertyDescriptorCollection.cs
- UserNamePasswordValidator.cs
- EdmPropertyAttribute.cs
- PagePropertiesChangingEventArgs.cs
- Debug.cs
- EventLogEntry.cs
- UmAlQuraCalendar.cs
- AuthenticateEventArgs.cs
- ObjectKeyFrameCollection.cs
- httpserverutility.cs
- Trace.cs
- OpenTypeCommon.cs
- HelpEvent.cs
- Composition.cs
- CodeValidator.cs
- Win32PrintDialog.cs
- InkCollectionBehavior.cs
- CodeNamespaceImportCollection.cs
- RefType.cs
- MemberMaps.cs
- StatusBarDrawItemEvent.cs
- DataTableReaderListener.cs
- GraphicsPathIterator.cs
- SafeFileMappingHandle.cs
- GenericWebPart.cs
- ToolStripItemCollection.cs
- DataTableReader.cs
- UrlMappingCollection.cs
- PtsHelper.cs
- MarkupExtensionReturnTypeAttribute.cs
- MethodBuilder.cs
- DrawingImage.cs
- JournalNavigationScope.cs
- XMLSyntaxException.cs
- ProfileServiceManager.cs
- SafeEventLogWriteHandle.cs
- ItemMap.cs
- OutputCacheProfile.cs
- XmlEntity.cs
- EntityDataSourceConfigureObjectContextPanel.cs
- ResourceWriter.cs
- SaveFileDialog.cs
- ConfigXmlCDataSection.cs
- ModelTypeConverter.cs
- CrossContextChannel.cs
- FieldNameLookup.cs
- AndCondition.cs
- Publisher.cs
- SafePipeHandle.cs
- TypeGeneratedEventArgs.cs
- TaskbarItemInfo.cs
- ProfileModule.cs
- AvtEvent.cs
- WorkflowCompensationBehavior.cs
- ResourceDisplayNameAttribute.cs
- Underline.cs
- ReferenceEqualityComparer.cs
- ProfileInfo.cs
- COAUTHINFO.cs
- Int16AnimationUsingKeyFrames.cs
- HtmlControlPersistable.cs
- ObjectHandle.cs
- AuthenticateEventArgs.cs