Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / MS / Internal / Printing / Win32PrintDialog.cs / 2 / Win32PrintDialog.cs
// Microsoft Avalon // Copyright (c) Microsoft Corporation, 2005 // // File: Win32PrintDialog.cs // // 05/24/2003 : mharper - created // //------------------------------------------------------------------------------ using System; using System.Drawing.Printing; using System.Printing.Interop; using System.Printing; using System.Runtime.InteropServices; using System.Security; using System.Security.Permissions; using System.Windows.Controls; namespace MS.Internal.Printing { ////// This entire class is implemented in this file. However, the class /// is marked partial because this class utilizes/implements a marshaler /// class that is private to it in another file. The object is called /// PrintDlgExMarshaler and warranted its own file. /// internal partial class Win32PrintDialog { #region Constructor ////// Constructs an instance of the Win32PrintDialog. This class is used for /// displaying the Win32 PrintDlgEx dialog and obtaining a user selected /// printer and PrintTicket for a print operation. /// ////// Critical: - Sets critical data. /// TreatAsSafe: - Sets the critical data to null for initialization. /// [SecurityCritical, SecurityTreatAsSafe] public Win32PrintDialog() { _printTicket = null; _printQueue = null; _minPage = 1; _maxPage = 9999; _pageRangeSelection = PageRangeSelection.AllPages; } #endregion Constructor #region Internal methods ////// Displays a modal Win32 print dialog to allow the user to select the desired /// printer and set the printing options. The data generated by this method /// can be accessed via the properties on the instance of this class. /// ////// Critical: - Create an instance of a critical class, calls critical /// methods on that instance, and retrieves critical properties. /// TreatAsSafe: - This code simply displays a WIN32 based dialog, gets the /// print settings from the user, and saves the data away as /// critical. The "unsafe" data that does into the unmanaged /// are properties marked critical (PrintTicket and PrintQueue) /// on this class. The other data that enters the unmanaged /// API are values that are integer based and uninteresting so /// therefore are not critical (MinPage, MaxPage, and page range /// values. Any data that is created by this method that is /// considered unsafe is marked critical. There are 2 properties /// that are extracted from the unmanaged call that are not considered /// critical. These are _pageRange and _pageRangeSelection. We do not /// care if these are exposed since the data means nothing except to /// the code that is doing the printing. /// [SecurityCritical, SecurityTreatAsSafe] internal UInt32 ShowDialog() { UInt32 result = NativeMethods.PD_RESULT_CANCEL; // // Get the process main window handle // IntPtr owner = IntPtr.Zero; if ((System.Windows.Application.Current != null) && (System.Windows.Application.Current.MainWindow != null)) { System.Windows.Interop.WindowInteropHelper helper = new System.Windows.Interop.WindowInteropHelper(System.Windows.Application.Current.MainWindow); owner = helper.CriticalHandle; } try { if (this._printQueue == null || this._printTicket == null) { // Normally printDlgEx.SyncToStruct() probes the printer if both the print queue and print // ticket are not null. // If either is null we probe the printer ourselves // If we dont end users will get notified that printing is disabled *after* // the print dialog has been displayed. ProbeForPrintingSupport(); } // // Create a PrintDlgEx instance to invoke the Win32 Print Dialog // using (PrintDlgExMarshaler printDlgEx = new PrintDlgExMarshaler(owner, this)) { printDlgEx.SyncToStruct(); // // Display the Win32 print dialog // Int32 hr = UnsafeNativeMethods.PrintDlgEx(printDlgEx.UnmanagedPrintDlgEx); if (hr == MS.Win32.NativeMethods.S_OK) { result = printDlgEx.SyncFromStruct(); } } } catch(PrintingNotSupportedException) { string message = System.Windows.SR.Get(System.Windows.SRID.PrintDialogInstallPrintSupportMessageBox); string caption = System.Windows.SR.Get(System.Windows.SRID.PrintDialogInstallPrintSupportCaption); bool isRtlCaption = caption != null && caption.Length > 0 && caption[0] == RightToLeftMark; System.Windows.MessageBoxOptions mbOptions = isRtlCaption ? System.Windows.MessageBoxOptions.RtlReading : System.Windows.MessageBoxOptions.None; int type = (int) System.Windows.MessageBoxButton.OK | (int) System.Windows.MessageBoxImage.Information | (int) mbOptions; if (owner == IntPtr.Zero) { owner = MS.Win32.UnsafeNativeMethods.GetActiveWindow(); } if(0 != MS.Win32.UnsafeNativeMethods.MessageBox(new HandleRef(null, owner), message, caption, type)) { result = NativeMethods.PD_RESULT_CANCEL; } } return result; } #endregion Internal methods #region Internal properties ////// Critical: Accesses critical data. /// internal PrintTicket PrintTicket { [SecurityCritical] get { return _printTicket; } [SecurityCritical] set { _printTicket = value; } } ////// Critical: Accesses critical data. /// internal PrintQueue PrintQueue { [SecurityCritical] get { return _printQueue; } [SecurityCritical] set { _printQueue = value; } } ////// Gets or sets the minimum page number allowed in the page ranges. /// internal UInt32 MinPage { get { return _minPage; } set { _minPage = value; } } ////// Gets or sets the maximum page number allowed in the page ranges. /// internal UInt32 MaxPage { get { return _maxPage; } set { _maxPage = value; } } ////// Gets or Sets the PageRangeSelection option for the print dialog. /// internal PageRangeSelection PageRangeSelection { get { return _pageRangeSelection; } set { _pageRangeSelection = value; } } ////// Gets or sets a PageRange objects used when the PageRangeSelection /// option is set to UserPages. /// internal PageRange PageRange { get { return _pageRange; } set { _pageRange = value; } } ////// Gets or sets a flag to enable/disable the page range control on the dialog. /// internal bool PageRangeEnabled { get { return _pageRangeEnabled; } set { _pageRangeEnabled = value; } } #endregion Internal properties #region Private methods ////// Probe to see if printing support is installed /// ////// Critical - Asserts DefaultPrinting permission in order to probe to see if a printer is available /// [SecurityCritical] private void ProbeForPrintingSupport() { // Without a print queue object we have to make up a name for the printer. // We will just ---- the print queue exception it generates later. // We could avoid the exception if we had access to // MS.Internal.Printing.Configuration.NativeMethods.BindPTProviderThunk string printerName = (this._printQueue != null) ? this._printQueue.FullName : string.Empty; (new PrintingPermission(PrintingPermissionLevel.DefaultPrinting)).Assert(); //BlessedAssert try { // If printer support is not installed this should throw a PrintingNotSupportedException using (IDisposable converter = new PrintTicketConverter(printerName, 1)) { } } catch (PrintQueueException) { // We can ---- print queue exceptions because they imply that printing // support is installed } finally { PrintingPermission.RevertAssert(); } } #endregion #region Private data ////// Critical: This is the print ticket used for printing /// the current job. It is critical because it /// could contains some user sensitive data /// [SecurityCritical] private PrintTicket _printTicket; ////// Critical: This is an object that represents a print queue. /// Any code that has access to this object has the /// potential to print jobs to this printer so it is /// a critical system resource. /// [SecurityCritical] private PrintQueue _printQueue; private PageRangeSelection _pageRangeSelection; private PageRange _pageRange; private bool _pageRangeEnabled; private UInt32 _minPage; private UInt32 _maxPage; private const char RightToLeftMark = '\u200F'; #endregion Private data } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2005 // // File: Win32PrintDialog.cs // // 05/24/2003 : mharper - created // //------------------------------------------------------------------------------ using System; using System.Drawing.Printing; using System.Printing.Interop; using System.Printing; using System.Runtime.InteropServices; using System.Security; using System.Security.Permissions; using System.Windows.Controls; namespace MS.Internal.Printing { ////// This entire class is implemented in this file. However, the class /// is marked partial because this class utilizes/implements a marshaler /// class that is private to it in another file. The object is called /// PrintDlgExMarshaler and warranted its own file. /// internal partial class Win32PrintDialog { #region Constructor ////// Constructs an instance of the Win32PrintDialog. This class is used for /// displaying the Win32 PrintDlgEx dialog and obtaining a user selected /// printer and PrintTicket for a print operation. /// ////// Critical: - Sets critical data. /// TreatAsSafe: - Sets the critical data to null for initialization. /// [SecurityCritical, SecurityTreatAsSafe] public Win32PrintDialog() { _printTicket = null; _printQueue = null; _minPage = 1; _maxPage = 9999; _pageRangeSelection = PageRangeSelection.AllPages; } #endregion Constructor #region Internal methods ////// Displays a modal Win32 print dialog to allow the user to select the desired /// printer and set the printing options. The data generated by this method /// can be accessed via the properties on the instance of this class. /// ////// Critical: - Create an instance of a critical class, calls critical /// methods on that instance, and retrieves critical properties. /// TreatAsSafe: - This code simply displays a WIN32 based dialog, gets the /// print settings from the user, and saves the data away as /// critical. The "unsafe" data that does into the unmanaged /// are properties marked critical (PrintTicket and PrintQueue) /// on this class. The other data that enters the unmanaged /// API are values that are integer based and uninteresting so /// therefore are not critical (MinPage, MaxPage, and page range /// values. Any data that is created by this method that is /// considered unsafe is marked critical. There are 2 properties /// that are extracted from the unmanaged call that are not considered /// critical. These are _pageRange and _pageRangeSelection. We do not /// care if these are exposed since the data means nothing except to /// the code that is doing the printing. /// [SecurityCritical, SecurityTreatAsSafe] internal UInt32 ShowDialog() { UInt32 result = NativeMethods.PD_RESULT_CANCEL; // // Get the process main window handle // IntPtr owner = IntPtr.Zero; if ((System.Windows.Application.Current != null) && (System.Windows.Application.Current.MainWindow != null)) { System.Windows.Interop.WindowInteropHelper helper = new System.Windows.Interop.WindowInteropHelper(System.Windows.Application.Current.MainWindow); owner = helper.CriticalHandle; } try { if (this._printQueue == null || this._printTicket == null) { // Normally printDlgEx.SyncToStruct() probes the printer if both the print queue and print // ticket are not null. // If either is null we probe the printer ourselves // If we dont end users will get notified that printing is disabled *after* // the print dialog has been displayed. ProbeForPrintingSupport(); } // // Create a PrintDlgEx instance to invoke the Win32 Print Dialog // using (PrintDlgExMarshaler printDlgEx = new PrintDlgExMarshaler(owner, this)) { printDlgEx.SyncToStruct(); // // Display the Win32 print dialog // Int32 hr = UnsafeNativeMethods.PrintDlgEx(printDlgEx.UnmanagedPrintDlgEx); if (hr == MS.Win32.NativeMethods.S_OK) { result = printDlgEx.SyncFromStruct(); } } } catch(PrintingNotSupportedException) { string message = System.Windows.SR.Get(System.Windows.SRID.PrintDialogInstallPrintSupportMessageBox); string caption = System.Windows.SR.Get(System.Windows.SRID.PrintDialogInstallPrintSupportCaption); bool isRtlCaption = caption != null && caption.Length > 0 && caption[0] == RightToLeftMark; System.Windows.MessageBoxOptions mbOptions = isRtlCaption ? System.Windows.MessageBoxOptions.RtlReading : System.Windows.MessageBoxOptions.None; int type = (int) System.Windows.MessageBoxButton.OK | (int) System.Windows.MessageBoxImage.Information | (int) mbOptions; if (owner == IntPtr.Zero) { owner = MS.Win32.UnsafeNativeMethods.GetActiveWindow(); } if(0 != MS.Win32.UnsafeNativeMethods.MessageBox(new HandleRef(null, owner), message, caption, type)) { result = NativeMethods.PD_RESULT_CANCEL; } } return result; } #endregion Internal methods #region Internal properties ////// Critical: Accesses critical data. /// internal PrintTicket PrintTicket { [SecurityCritical] get { return _printTicket; } [SecurityCritical] set { _printTicket = value; } } ////// Critical: Accesses critical data. /// internal PrintQueue PrintQueue { [SecurityCritical] get { return _printQueue; } [SecurityCritical] set { _printQueue = value; } } ////// Gets or sets the minimum page number allowed in the page ranges. /// internal UInt32 MinPage { get { return _minPage; } set { _minPage = value; } } ////// Gets or sets the maximum page number allowed in the page ranges. /// internal UInt32 MaxPage { get { return _maxPage; } set { _maxPage = value; } } ////// Gets or Sets the PageRangeSelection option for the print dialog. /// internal PageRangeSelection PageRangeSelection { get { return _pageRangeSelection; } set { _pageRangeSelection = value; } } ////// Gets or sets a PageRange objects used when the PageRangeSelection /// option is set to UserPages. /// internal PageRange PageRange { get { return _pageRange; } set { _pageRange = value; } } ////// Gets or sets a flag to enable/disable the page range control on the dialog. /// internal bool PageRangeEnabled { get { return _pageRangeEnabled; } set { _pageRangeEnabled = value; } } #endregion Internal properties #region Private methods ////// Probe to see if printing support is installed /// ////// Critical - Asserts DefaultPrinting permission in order to probe to see if a printer is available /// [SecurityCritical] private void ProbeForPrintingSupport() { // Without a print queue object we have to make up a name for the printer. // We will just ---- the print queue exception it generates later. // We could avoid the exception if we had access to // MS.Internal.Printing.Configuration.NativeMethods.BindPTProviderThunk string printerName = (this._printQueue != null) ? this._printQueue.FullName : string.Empty; (new PrintingPermission(PrintingPermissionLevel.DefaultPrinting)).Assert(); //BlessedAssert try { // If printer support is not installed this should throw a PrintingNotSupportedException using (IDisposable converter = new PrintTicketConverter(printerName, 1)) { } } catch (PrintQueueException) { // We can ---- print queue exceptions because they imply that printing // support is installed } finally { PrintingPermission.RevertAssert(); } } #endregion #region Private data ////// Critical: This is the print ticket used for printing /// the current job. It is critical because it /// could contains some user sensitive data /// [SecurityCritical] private PrintTicket _printTicket; ////// Critical: This is an object that represents a print queue. /// Any code that has access to this object has the /// potential to print jobs to this printer so it is /// a critical system resource. /// [SecurityCritical] private PrintQueue _printQueue; private PageRangeSelection _pageRangeSelection; private PageRange _pageRange; private bool _pageRangeEnabled; private UInt32 _minPage; private UInt32 _maxPage; private const char RightToLeftMark = '\u200F'; #endregion Private data } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
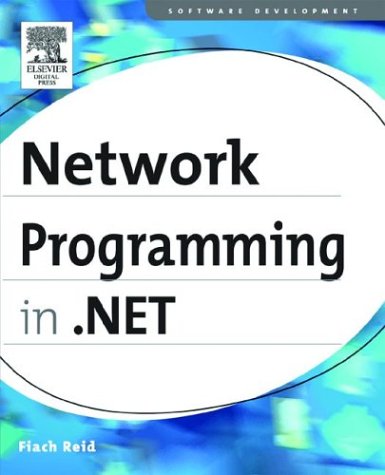
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Models.cs
- MsmqMessageProperty.cs
- RoutingExtensionElement.cs
- CorrelationResolver.cs
- FlowDocumentReaderAutomationPeer.cs
- TemplateDefinition.cs
- XmlMtomReader.cs
- _ConnectOverlappedAsyncResult.cs
- ObjectDataSourceStatusEventArgs.cs
- PlaceHolder.cs
- ActivityCodeGenerator.cs
- TraceContext.cs
- ByteStack.cs
- RecordsAffectedEventArgs.cs
- QueryContinueDragEvent.cs
- StringConverter.cs
- ExpressionVisitorHelpers.cs
- SelectorAutomationPeer.cs
- UnsafeNativeMethods.cs
- QueueException.cs
- EasingKeyFrames.cs
- FormatConvertedBitmap.cs
- TextSelection.cs
- ColorPalette.cs
- Blend.cs
- Menu.cs
- EnumCodeDomSerializer.cs
- ModelFunctionTypeElement.cs
- SecurityRuntime.cs
- SmiGettersStream.cs
- DataGridViewRowPostPaintEventArgs.cs
- InkCanvasSelection.cs
- HtmlLink.cs
- Help.cs
- SqlFactory.cs
- KnowledgeBase.cs
- MemoryFailPoint.cs
- XmlSchemaSimpleTypeRestriction.cs
- TextBreakpoint.cs
- ServiceObjectContainer.cs
- TextElementEditingBehaviorAttribute.cs
- ToolStripRendererSwitcher.cs
- DependencyObjectPropertyDescriptor.cs
- WebServiceResponseDesigner.cs
- KerberosRequestorSecurityToken.cs
- DescendantBaseQuery.cs
- Utils.cs
- CommandBinding.cs
- TagMapCollection.cs
- ScriptServiceAttribute.cs
- LabelEditEvent.cs
- SelectionItemProviderWrapper.cs
- ProviderCollection.cs
- CollectionViewGroupRoot.cs
- Cursors.cs
- DynamicDocumentPaginator.cs
- GridViewUpdateEventArgs.cs
- NamespaceMapping.cs
- GestureRecognizer.cs
- EmbossBitmapEffect.cs
- X509CertificateValidator.cs
- configsystem.cs
- WebAdminConfigurationHelper.cs
- GlyphTypeface.cs
- CompilationAssemblyInstallComponent.cs
- MemoryFailPoint.cs
- ModelItemCollectionImpl.cs
- PartialList.cs
- EncoderParameters.cs
- TreeViewHitTestInfo.cs
- EntityDataSourceColumn.cs
- CultureInfoConverter.cs
- QualificationDataItem.cs
- BinaryObjectReader.cs
- srgsitem.cs
- FontCacheUtil.cs
- FormsAuthenticationEventArgs.cs
- EntityCommandExecutionException.cs
- FocusChangedEventArgs.cs
- TextWriter.cs
- TdsParameterSetter.cs
- TableDetailsRow.cs
- C14NUtil.cs
- OletxResourceManager.cs
- ApplicationProxyInternal.cs
- DelegateTypeInfo.cs
- ConstraintEnumerator.cs
- PasswordPropertyTextAttribute.cs
- EditingCoordinator.cs
- ProfileManager.cs
- TrackingValidationObjectDictionary.cs
- OperationParameterInfo.cs
- SafeProcessHandle.cs
- COM2FontConverter.cs
- SafeTimerHandle.cs
- ListControl.cs
- Keyboard.cs
- EntityDataSourceWrapperCollection.cs
- ReliableInputConnection.cs
- ByteAnimation.cs