Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Data / System / Data / SqlClient / TdsParameterSetter.cs / 1305376 / TdsParameterSetter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data.SqlClient { using System; using System.Data; using System.Data.SqlTypes; using System.Diagnostics; using Microsoft.SqlServer.Server; // Simple Getter/Setter for structured parameters to allow using common ValueUtilsSmi code. // This is a stand-in to having a true SmiRequestExecutor class for TDS. internal class TdsParameterSetter : SmiTypedGetterSetter { #region Private fields private TdsRecordBufferSetter _target; #if UseSmiForTds private TdsValueSetter _scalarTarget; #endif #endregion #region ctor & control internal TdsParameterSetter(TdsParserStateObject stateObj, SmiMetaData md) { #if UseSmiForTds if (SqlDbType.Structured == md.SqlDbType) { #endif _target = new TdsRecordBufferSetter(stateObj, md); #if UseSmiForTds } else { _scalarTarget = new TdsValueSetter(stateObj, md); } #endif } #endregion #region TypedGetterSetter overrides // Are calls to Get methods allowed? internal override bool CanGet { get { return false; } } // Are calls to Set methods allowed? internal override bool CanSet { get { return true; } } // valid for structured types // This method called for both get and set. internal override SmiTypedGetterSetter GetTypedGetterSetter(SmiEventSink sink, int ordinal) { Debug.Assert(0==ordinal, "TdsParameterSetter only supports 0 for ordinal. Actual = " + ordinal); return _target; } // Set value to null // valid for all types public override void SetDBNull(SmiEventSink sink, int ordinal) { Debug.Assert(0==ordinal, "TdsParameterSetter only supports 0 for ordinal. Actual = " + ordinal); #if UseSmiForTds if (SqlDbType.Structured == md.SqlDbType) { #endif _target.EndElements(sink); #if UseSmiForTds } else { _scalarTarget.SetDBNull(); } #endif } #if UseSmiForTds // valid for SqlDbType.Bit public override void SetBoolean(SmiEventSink sink, int ordinal, Boolean value) { Debug.Assert(0==ordinal, "TdsParameterSetter only supports 0 for ordinal. Actual = " + ordinal); _scalarTarget.SetBoolean(value); } // valid for SqlDbType.TinyInt public override void SetByte(SmiEventSink sink, int ordinal, Byte value) { Debug.Assert(0==ordinal, "TdsParameterSetter only supports 0 for ordinal. Actual = " + ordinal); _scalarTarget.SetByte(value); } // Semantics for SetBytes are to modify existing value, not overwrite // Use in combination with SetLength to ensure overwriting when necessary // valid for SqlDbTypes: Binary, VarBinary, Image, Udt, Xml // (VarBinary assumed for variants) public override int SetBytes(SmiEventSink sink, int ordinal, long fieldOffset, byte[] buffer, int bufferOffset, int length) { Debug.Assert(0==ordinal, "TdsParameterSetter only supports 0 for ordinal. Actual = " + ordinal); return _scalarTarget.SetBytes(fieldOffset, buffer, bufferOffset, length); } public override void SetBytesLength(SmiEventSink sink, int ordinal, long length) { Debug.Assert(0==ordinal, "TdsParameterSetter only supports 0 for ordinal. Actual = " + ordinal); _scalarTarget.SetBytesLength(length); } // Semantics for SetChars are to modify existing value, not overwrite // Use in combination with SetLength to ensure overwriting when necessary // valid for character types: Char, VarChar, Text, NChar, NVarChar, NText // (NVarChar and global clr collation assumed for variants) public override int SetChars(SmiEventSink sink, int ordinal, long fieldOffset, char[] buffer, int bufferOffset, int length) { Debug.Assert(0==ordinal, "TdsParameterSetter only supports 0 for ordinal. Actual = " + ordinal); return _scalarTarget.SetChars(fieldOffset, buffer, bufferOffset, length); } public override void SetCharsLength(SmiEventSink sink, int ordinal, long length) { Debug.Assert(0==ordinal, "TdsParameterSetter only supports 0 for ordinal. Actual = " + ordinal); _scalarTarget.SetCharsLength(length); } // valid for character types: Char, VarChar, Text, NChar, NVarChar, NText public override void SetString(SmiEventSink sink, int ordinal, string value, int offset, int length) { Debug.Assert(0==ordinal, "TdsParameterSetter only supports 0 for ordinal. Actual = " + ordinal); _scalarTarget.SetString(value, offset, length); } // valid for SqlDbType.SmallInt public override void SetInt16(SmiEventSink sink, int ordinal, Int16 value) { Debug.Assert(0==ordinal, "TdsParameterSetter only supports 0 for ordinal. Actual = " + ordinal); _scalarTarget.SetInt16(value); } // valid for SqlDbType.Int public override void SetInt32(SmiEventSink sink, int ordinal, Int32 value) { Debug.Assert(0==ordinal, "TdsParameterSetter only supports 0 for ordinal. Actual = " + ordinal); _scalarTarget.SetInt32(value); } // valid for SqlDbType.BigInt, SqlDbType.Money, SqlDbType.SmallMoney public override void SetInt64(SmiEventSink sink, int ordinal, Int64 value) { Debug.Assert(0==ordinal, "TdsParameterSetter only supports 0 for ordinal. Actual = " + ordinal); _scalarTarget.SetInt64(value); } // valid for SqlDbType.Real public override void SetSingle(SmiEventSink sink, int ordinal, Single value) { Debug.Assert(0==ordinal, "TdsParameterSetter only supports 0 for ordinal. Actual = " + ordinal); _scalarTarget.SetSingle(value); } // valid for SqlDbType.Float public override void SetDouble(SmiEventSink sink, int ordinal, Double value) { Debug.Assert(0==ordinal, "TdsParameterSetter only supports 0 for ordinal. Actual = " + ordinal); _scalarTarget.SetDouble(value); } // valid for SqlDbType.Numeric (uses SqlDecimal since Decimal cannot hold full range) public override void SetSqlDecimal(SmiEventSink sink, int ordinal, SqlDecimal value) { Debug.Assert(0==ordinal, "TdsParameterSetter only supports 0 for ordinal. Actual = " + ordinal); _scalarTarget.SetSqlDecimal(value); } // valid for DateTime & SmallDateTime public override void SetDateTime(SmiEventSink sink, int ordinal, DateTime value) { Debug.Assert(0==ordinal, "TdsParameterSetter only supports 0 for ordinal. Actual = " + ordinal); _scalarTarget.SetDateTime(value); } // valid for UniqueIdentifier public override void SetGuid(SmiEventSink sink, int ordinal, Guid value) { Debug.Assert(0==ordinal, "TdsParameterSetter only supports 0 for ordinal. Actual = " + ordinal); _scalarTarget.SetGuid(value); } #endif #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data.SqlClient { using System; using System.Data; using System.Data.SqlTypes; using System.Diagnostics; using Microsoft.SqlServer.Server; // Simple Getter/Setter for structured parameters to allow using common ValueUtilsSmi code. // This is a stand-in to having a true SmiRequestExecutor class for TDS. internal class TdsParameterSetter : SmiTypedGetterSetter { #region Private fields private TdsRecordBufferSetter _target; #if UseSmiForTds private TdsValueSetter _scalarTarget; #endif #endregion #region ctor & control internal TdsParameterSetter(TdsParserStateObject stateObj, SmiMetaData md) { #if UseSmiForTds if (SqlDbType.Structured == md.SqlDbType) { #endif _target = new TdsRecordBufferSetter(stateObj, md); #if UseSmiForTds } else { _scalarTarget = new TdsValueSetter(stateObj, md); } #endif } #endregion #region TypedGetterSetter overrides // Are calls to Get methods allowed? internal override bool CanGet { get { return false; } } // Are calls to Set methods allowed? internal override bool CanSet { get { return true; } } // valid for structured types // This method called for both get and set. internal override SmiTypedGetterSetter GetTypedGetterSetter(SmiEventSink sink, int ordinal) { Debug.Assert(0==ordinal, "TdsParameterSetter only supports 0 for ordinal. Actual = " + ordinal); return _target; } // Set value to null // valid for all types public override void SetDBNull(SmiEventSink sink, int ordinal) { Debug.Assert(0==ordinal, "TdsParameterSetter only supports 0 for ordinal. Actual = " + ordinal); #if UseSmiForTds if (SqlDbType.Structured == md.SqlDbType) { #endif _target.EndElements(sink); #if UseSmiForTds } else { _scalarTarget.SetDBNull(); } #endif } #if UseSmiForTds // valid for SqlDbType.Bit public override void SetBoolean(SmiEventSink sink, int ordinal, Boolean value) { Debug.Assert(0==ordinal, "TdsParameterSetter only supports 0 for ordinal. Actual = " + ordinal); _scalarTarget.SetBoolean(value); } // valid for SqlDbType.TinyInt public override void SetByte(SmiEventSink sink, int ordinal, Byte value) { Debug.Assert(0==ordinal, "TdsParameterSetter only supports 0 for ordinal. Actual = " + ordinal); _scalarTarget.SetByte(value); } // Semantics for SetBytes are to modify existing value, not overwrite // Use in combination with SetLength to ensure overwriting when necessary // valid for SqlDbTypes: Binary, VarBinary, Image, Udt, Xml // (VarBinary assumed for variants) public override int SetBytes(SmiEventSink sink, int ordinal, long fieldOffset, byte[] buffer, int bufferOffset, int length) { Debug.Assert(0==ordinal, "TdsParameterSetter only supports 0 for ordinal. Actual = " + ordinal); return _scalarTarget.SetBytes(fieldOffset, buffer, bufferOffset, length); } public override void SetBytesLength(SmiEventSink sink, int ordinal, long length) { Debug.Assert(0==ordinal, "TdsParameterSetter only supports 0 for ordinal. Actual = " + ordinal); _scalarTarget.SetBytesLength(length); } // Semantics for SetChars are to modify existing value, not overwrite // Use in combination with SetLength to ensure overwriting when necessary // valid for character types: Char, VarChar, Text, NChar, NVarChar, NText // (NVarChar and global clr collation assumed for variants) public override int SetChars(SmiEventSink sink, int ordinal, long fieldOffset, char[] buffer, int bufferOffset, int length) { Debug.Assert(0==ordinal, "TdsParameterSetter only supports 0 for ordinal. Actual = " + ordinal); return _scalarTarget.SetChars(fieldOffset, buffer, bufferOffset, length); } public override void SetCharsLength(SmiEventSink sink, int ordinal, long length) { Debug.Assert(0==ordinal, "TdsParameterSetter only supports 0 for ordinal. Actual = " + ordinal); _scalarTarget.SetCharsLength(length); } // valid for character types: Char, VarChar, Text, NChar, NVarChar, NText public override void SetString(SmiEventSink sink, int ordinal, string value, int offset, int length) { Debug.Assert(0==ordinal, "TdsParameterSetter only supports 0 for ordinal. Actual = " + ordinal); _scalarTarget.SetString(value, offset, length); } // valid for SqlDbType.SmallInt public override void SetInt16(SmiEventSink sink, int ordinal, Int16 value) { Debug.Assert(0==ordinal, "TdsParameterSetter only supports 0 for ordinal. Actual = " + ordinal); _scalarTarget.SetInt16(value); } // valid for SqlDbType.Int public override void SetInt32(SmiEventSink sink, int ordinal, Int32 value) { Debug.Assert(0==ordinal, "TdsParameterSetter only supports 0 for ordinal. Actual = " + ordinal); _scalarTarget.SetInt32(value); } // valid for SqlDbType.BigInt, SqlDbType.Money, SqlDbType.SmallMoney public override void SetInt64(SmiEventSink sink, int ordinal, Int64 value) { Debug.Assert(0==ordinal, "TdsParameterSetter only supports 0 for ordinal. Actual = " + ordinal); _scalarTarget.SetInt64(value); } // valid for SqlDbType.Real public override void SetSingle(SmiEventSink sink, int ordinal, Single value) { Debug.Assert(0==ordinal, "TdsParameterSetter only supports 0 for ordinal. Actual = " + ordinal); _scalarTarget.SetSingle(value); } // valid for SqlDbType.Float public override void SetDouble(SmiEventSink sink, int ordinal, Double value) { Debug.Assert(0==ordinal, "TdsParameterSetter only supports 0 for ordinal. Actual = " + ordinal); _scalarTarget.SetDouble(value); } // valid for SqlDbType.Numeric (uses SqlDecimal since Decimal cannot hold full range) public override void SetSqlDecimal(SmiEventSink sink, int ordinal, SqlDecimal value) { Debug.Assert(0==ordinal, "TdsParameterSetter only supports 0 for ordinal. Actual = " + ordinal); _scalarTarget.SetSqlDecimal(value); } // valid for DateTime & SmallDateTime public override void SetDateTime(SmiEventSink sink, int ordinal, DateTime value) { Debug.Assert(0==ordinal, "TdsParameterSetter only supports 0 for ordinal. Actual = " + ordinal); _scalarTarget.SetDateTime(value); } // valid for UniqueIdentifier public override void SetGuid(SmiEventSink sink, int ordinal, Guid value) { Debug.Assert(0==ordinal, "TdsParameterSetter only supports 0 for ordinal. Actual = " + ordinal); _scalarTarget.SetGuid(value); } #endif #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
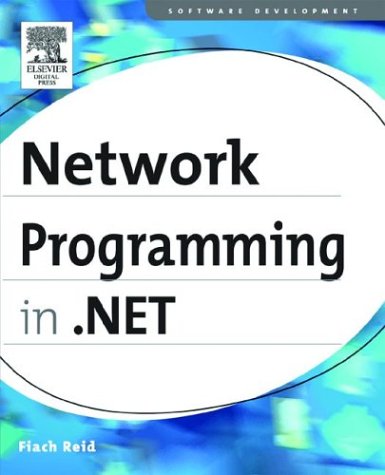
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Pen.cs
- SymbolType.cs
- StaticTextPointer.cs
- FormViewPagerRow.cs
- EntityCollection.cs
- FixedSOMLineRanges.cs
- KerberosReceiverSecurityToken.cs
- WinInetCache.cs
- XmlSchema.cs
- Merger.cs
- FileSystemInfo.cs
- SourceElementsCollection.cs
- SystemDiagnosticsSection.cs
- ClientBuildManagerCallback.cs
- ContextMenuStrip.cs
- SecurityDescriptor.cs
- StrokeDescriptor.cs
- AccessDataSourceView.cs
- InlineCategoriesDocument.cs
- ExecutorLocksHeldException.cs
- PngBitmapDecoder.cs
- XMLDiffLoader.cs
- ImageIndexConverter.cs
- SEHException.cs
- Comparer.cs
- CommandLibraryHelper.cs
- OdbcEnvironmentHandle.cs
- LowerCaseStringConverter.cs
- PingOptions.cs
- BinHexEncoding.cs
- NativeMethods.cs
- HttpHostedTransportConfiguration.cs
- ProtocolsConfiguration.cs
- CustomAttribute.cs
- SQLGuidStorage.cs
- HttpPostServerProtocol.cs
- EdmType.cs
- PromptEventArgs.cs
- CharacterHit.cs
- CheckBoxList.cs
- DeviceContext2.cs
- Point3DConverter.cs
- XmlWrappingReader.cs
- DefaultValueTypeConverter.cs
- PriorityQueue.cs
- Cursor.cs
- XmlElementAttribute.cs
- LongTypeConverter.cs
- Block.cs
- WmpBitmapDecoder.cs
- TypeProvider.cs
- HttpGetServerProtocol.cs
- ConnectionPointGlyph.cs
- RootBrowserWindow.cs
- SqlDataReaderSmi.cs
- CodeComment.cs
- SpotLight.cs
- Exceptions.cs
- ItemCollection.cs
- StrokeSerializer.cs
- PerformanceCounterLib.cs
- TargetControlTypeCache.cs
- base64Transforms.cs
- xmlsaver.cs
- DesignerDataStoredProcedure.cs
- CustomErrorsSectionWrapper.cs
- HttpHandlerActionCollection.cs
- HuffModule.cs
- ObjectItemNoOpAssemblyLoader.cs
- RegistryPermission.cs
- SubstitutionDesigner.cs
- Rect3D.cs
- RadioButton.cs
- DockPattern.cs
- AuthenticationConfig.cs
- ConnectionInterfaceCollection.cs
- PropertyGrid.cs
- SamlAttributeStatement.cs
- ListViewItem.cs
- MetadataCacheItem.cs
- mediapermission.cs
- AdjustableArrowCap.cs
- FlowDocumentScrollViewerAutomationPeer.cs
- IEnumerable.cs
- GridViewCancelEditEventArgs.cs
- Function.cs
- SqlCacheDependencySection.cs
- TypeGenericEnumerableViewSchema.cs
- DataGridViewColumnStateChangedEventArgs.cs
- EventlogProvider.cs
- ConfigurationElementCollection.cs
- Socket.cs
- ButtonFieldBase.cs
- EncodingInfo.cs
- EventHandlersStore.cs
- VarRefManager.cs
- MetadataFile.cs
- DataObjectSettingDataEventArgs.cs
- DisplayInformation.cs
- coordinatorscratchpad.cs