Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / EncryptionUtility.cs / 1 / EncryptionUtility.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.IdentityModel.Selectors; using System.IdentityModel.Tokens; using System.ServiceModel.Security.Tokens; using System.ServiceModel; using System.ServiceModel.Security; using System.IO; using System.Security.Cryptography.X509Certificates; using System.Security.Cryptography.Xml; using System.Xml; using System.Security.Principal; using System.Security.Cryptography; using System.Text; using System.Globalization; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; // // Summary // This class provides utility function to enable encryption of tokens // internal sealed class EncryptionUtility { private EncryptionUtility() { } // // Summary // Encrypt a security token // // Parameters // tokenToBeEncrypted - The security token that needs to be encrypted // cert - The certificate of the party to which the token is to be encrypted // encryptingAlgorithm - The algorithm to use for encryption // public static XmlElement EncryptSecurityToken( SecurityToken tokenToBeEncrypted, X509Certificate2 cert, string encryptingAlgorithm, string asymmetricKeyWrapAlgorithm, ProtocolProfile profile ) { // // create the stream for data to be encrypted // MemoryStream streamToBeEncrypted = new MemoryStream(); XmlDictionaryWriter writer = XmlDictionaryWriter.CreateDictionaryWriter( new XmlTextWriter( new StreamWriter( streamToBeEncrypted ) ) ); profile.TokenSerializer.WriteToken( writer, tokenToBeEncrypted ); writer.Flush(); streamToBeEncrypted.Seek( 0, SeekOrigin.Begin ); return EncryptToken( streamToBeEncrypted, cert, encryptingAlgorithm, asymmetricKeyWrapAlgorithm, profile ); } // // Summary // Encrypt a security token // // Parameters // elem - The security token element that needs to be encrypted // cert - The certificate of the party to which the token is to be encrypted // encryptingAlgorithm - The algorithm to use for encryption // keyWrapAlgorithm - Symmetric P ==> rasoaep. Asymmetric P ==> as specified // public static XmlElement EncryptSecurityToken( XmlElement elem, X509Certificate2 cert, string encryptingAlgorithm, string asymmetricKeyWrapAlgorithm, ProtocolProfile profile ) { // // create the stream for data to be encrypted // MemoryStream streamToBeEncrypted = new MemoryStream(); XmlDictionaryWriter writer = XmlDictionaryWriter.CreateDictionaryWriter( new XmlTextWriter( new StreamWriter( streamToBeEncrypted ) ) ); elem.WriteTo( writer ); writer.Flush(); streamToBeEncrypted.Seek( 0, SeekOrigin.Begin ); return EncryptToken( streamToBeEncrypted, cert, encryptingAlgorithm, asymmetricKeyWrapAlgorithm, profile ); } // // Summary // Encrypt a security token // // Parameters // streamToBeEncrypted - The security token stream that needs to be encrypted // cert - The certificate of the party to which the token is to be encrypted // encryptingAlgorithm - The algorithm to use for encryption // keyWrapAlgorithm - Symmetric P ==> rasoaep. Asymmetric P ==> as specified // private static XmlElement EncryptToken( MemoryStream streamToBeEncrypted, X509Certificate2 cert, string encryptingAlgorithm, string asymmetricKeyWrapAlgorithm, ProtocolProfile profile ) { IDT.TraceDebug( "Encrypting the security token" ); IDT.ThrowInvalidArgumentConditional( String.IsNullOrEmpty( encryptingAlgorithm ), "encryptingAlgorithm" ); IDT.ThrowInvalidArgumentConditional( null == cert, "cert" ); IDT.TraceDebug( "Encrypting issued token with {0} algorithm", encryptingAlgorithm ); IDT.TraceDebug( "Encrypting issued token with {0} certificate", cert.FriendlyName ); SecurityToken encryptingToken = new X509SecurityToken( cert, "id" ); SecurityAlgorithmSuite suite = SecurityAlgorithmSuite.Default; switch( encryptingAlgorithm ) { case SecurityAlgorithms.Aes128Encryption: suite = SecurityAlgorithmSuite.Basic128; break; case SecurityAlgorithms.Aes192Encryption: suite = SecurityAlgorithmSuite.Basic192; break; case SecurityAlgorithms.Aes256Encryption: suite = SecurityAlgorithmSuite.Basic256; break; case SecurityAlgorithms.TripleDesEncryption: suite = SecurityAlgorithmSuite.TripleDes; break; default: throw IDT.ThrowHelperError( new TokenCreationException( SR.GetString( SR.UnsupportedEncryptionAlgorithm, encryptingAlgorithm ) ) ); } // // create the keys to be used for encryption // SecurityKeyIdentifier encryptingKeyIdentifier = new SecurityKeyIdentifier( encryptingToken.CreateKeyIdentifierClause() ); int encryptedKeySize = suite.DefaultEncryptionKeyDerivationLength / 8; byte[ ] keyToWrap = new byte[ encryptedKeySize ]; RNGCryptoServiceProvider random = new RNGCryptoServiceProvider(); random.GetNonZeroBytes( keyToWrap ); WrappedKeySecurityToken wrappedKeyToken = new WrappedKeySecurityToken( string.Empty, keyToWrap, asymmetricKeyWrapAlgorithm, encryptingToken, encryptingKeyIdentifier ); SecurityKeyIdentifier keyIdentifier = new SecurityKeyIdentifier( new EncryptedKeyIdentifierClause( wrappedKeyToken.GetWrappedKey(), wrappedKeyToken.WrappingAlgorithm, wrappedKeyToken.WrappingTokenReference ) ); SymmetricSecurityKey encryptingCrypto = ( SymmetricSecurityKey )wrappedKeyToken.SecurityKeys[ 0 ]; // // Use the algorithm provided and encrypt the data // SymmetricAlgorithm algorithm = encryptingCrypto.GetSymmetricAlgorithm( encryptingAlgorithm ); EncryptedData encryptedData = new EncryptedData(); encryptedData.TokenSerializer = profile.TokenSerializer; encryptedData.KeyIdentifier = keyIdentifier; encryptedData.EncryptionMethod = encryptingAlgorithm; encryptedData.Type = EncryptedXml.XmlEncElementUrl; encryptedData.SetUpEncryption( algorithm, streamToBeEncrypted.GetBuffer(), 0, Convert.ToInt32( streamToBeEncrypted.Length ) ); // // write the encrypted data to a memory stream // IDT.TraceDebug( "Writing encrypted token to memory stream" ); MemoryStream encryptedStream = new MemoryStream(); XmlDictionaryWriter writer = XmlDictionaryWriter.CreateDictionaryWriter( new XmlTextWriter( new StreamWriter( encryptedStream ) ) ); encryptedData.WriteTo( writer ); writer.Flush(); encryptedStream.Seek( 0, SeekOrigin.Begin ); // // Create an XmlElement for the encrypted data // XmlDocument doc = new XmlDocument(); XmlElement tokenXml = ( XmlElement )doc.ReadNode( Utility.CreateReaderWithQuotas( encryptedStream ) ); Array.Clear( streamToBeEncrypted.GetBuffer(), 0, Convert.ToInt32( streamToBeEncrypted.Length ) ); Array.Clear( encryptedStream.GetBuffer(), 0, Convert.ToInt32( encryptedStream.Length ) ); streamToBeEncrypted.Close(); encryptedStream.Close(); return tokenXml; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
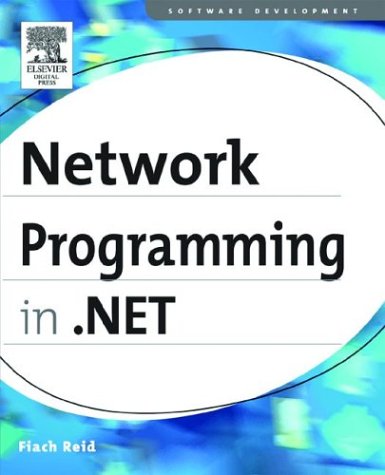
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TcpTransportElement.cs
- PackageFilter.cs
- oledbmetadatacolumnnames.cs
- DataColumnMapping.cs
- StateDesignerConnector.cs
- HwndSourceKeyboardInputSite.cs
- SqlIdentifier.cs
- DesignerRegionMouseEventArgs.cs
- EntityProxyFactory.cs
- CriticalHandle.cs
- SoapHeaderException.cs
- IEnumerable.cs
- CodeDirectoryCompiler.cs
- UriScheme.cs
- ValueQuery.cs
- DBBindings.cs
- OleDbRowUpdatedEvent.cs
- WebPartActionVerb.cs
- SafeLocalMemHandle.cs
- List.cs
- SecurityDescriptor.cs
- FieldBuilder.cs
- StringInfo.cs
- MouseEvent.cs
- GridPatternIdentifiers.cs
- RegexGroup.cs
- AppSettingsExpressionBuilder.cs
- RectangleF.cs
- SafeIUnknown.cs
- ToolStripItemImageRenderEventArgs.cs
- EntitySqlQueryBuilder.cs
- WebBaseEventKeyComparer.cs
- RemoteArgument.cs
- XmlChildNodes.cs
- PaperSource.cs
- PersonalizationAdministration.cs
- ButtonColumn.cs
- ProfessionalColorTable.cs
- securitycriticaldataClass.cs
- AlternateView.cs
- ObjectQueryProvider.cs
- SdlChannelSink.cs
- ThreadNeutralSemaphore.cs
- HeaderCollection.cs
- FilterableData.cs
- DecoderFallbackWithFailureFlag.cs
- CompositeControl.cs
- FontStyleConverter.cs
- FormatVersion.cs
- HttpResponseHeader.cs
- DCSafeHandle.cs
- ExpressionBuilder.cs
- ResourcesGenerator.cs
- ParenthesizePropertyNameAttribute.cs
- OutputScopeManager.cs
- DataTableMappingCollection.cs
- ToolStrip.cs
- MasterPageCodeDomTreeGenerator.cs
- CodeIdentifier.cs
- TableRow.cs
- SimpleMailWebEventProvider.cs
- Ref.cs
- UserControlAutomationPeer.cs
- WebEventTraceProvider.cs
- EventArgs.cs
- InternalControlCollection.cs
- ContentOperations.cs
- MaskedTextBox.cs
- UInt64.cs
- DeploymentSection.cs
- ButtonPopupAdapter.cs
- StrokeCollectionDefaultValueFactory.cs
- XsdValidatingReader.cs
- SafeFindHandle.cs
- SystemNetHelpers.cs
- TreeView.cs
- SslStream.cs
- RuntimeResourceSet.cs
- SAPIEngineTypes.cs
- EventLogException.cs
- IxmlLineInfo.cs
- ValidationSummary.cs
- FactoryMaker.cs
- UnmanagedMemoryAccessor.cs
- ViewCellRelation.cs
- MatrixConverter.cs
- DecoderFallbackWithFailureFlag.cs
- GridViewRowEventArgs.cs
- PolygonHotSpot.cs
- RelativeSource.cs
- TextTreePropertyUndoUnit.cs
- JoinElimination.cs
- EntityDataSourceWrapperPropertyDescriptor.cs
- PropertyGeneratedEventArgs.cs
- ServicesUtilities.cs
- CodeDomComponentSerializationService.cs
- ObjectPropertyMapping.cs
- CodeObject.cs
- LinearGradientBrush.cs
- _LocalDataStoreMgr.cs