Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Shared / MS / Internal / PartialList.cs / 1 / PartialList.cs
//---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // Description: PartialList is used when the developer needs to pass an IList range to // a function that takes generic IList interface. // // // History: // 06/25/2004 : [....] - Created // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; namespace MS.Internal { ////// PartialList is used when someone needs to pass an IList range to /// a function that takes generic IList interface. It implemented a read-only subset of IList. /// internal class PartialList: IList { private IList _list; private int _initialIndex; private int _count; /// /// Convenience constructor for taking in an entire list. Useful for creating a read-only /// version of the list. /// public PartialList(IListlist) { _list = list; _initialIndex = 0; _count = list.Count; } public PartialList(IList list, int initialIndex, int count) { // make sure early that the caller didn't miscalculate index and count Debug.Assert(initialIndex >= 0 && initialIndex + count <= list.Count); _list = list; _initialIndex = initialIndex; _count = count; } #if !PRESENTATION_CORE /// /// Creates new PartialList object only for true partial ranges. /// Otherwise, returns the original list. /// public static IListCreate(IList list, int initialIndex, int count) { if (list == null) return null; if (initialIndex == 0 && count == list.Count) return list; return new PartialList (list, initialIndex, count); } #endif #region IList Members public void RemoveAt(int index) { // PartialList is read only. throw new NotSupportedException(); } public void Insert(int index, T item) { // PartialList is read only. throw new NotSupportedException(); } public T this[int index] { get { return _list[index + _initialIndex]; } set { // PartialList is read only. throw new NotSupportedException(); } } public int IndexOf(T item) { int index = _list.IndexOf(item); if (index == -1 || index < _initialIndex || index - _initialIndex >= _count) return -1; return index - _initialIndex; } #endregion #region ICollection Members public bool IsReadOnly { get { return true; } } public void Clear() { // PartialList is read only. throw new NotSupportedException(); } public void Add(T item) { // PartialList is read only. throw new NotSupportedException(); } public bool Contains(T item) { return IndexOf(item) != -1; } public bool Remove(T item) { // PartialList is read only. throw new NotSupportedException(); } public int Count { get { return _count; } } public void CopyTo(T[] array, int arrayIndex) { if (arrayIndex < 0) throw new ArgumentOutOfRangeException("arrayIndex"); for (int i = 0; i < _count; ++i) array[arrayIndex + i] = this[i]; } #endregion #region IEnumerable Members IEnumerator IEnumerable .GetEnumerator() { for (int i = _initialIndex; i < _initialIndex + _count; ++i) yield return _list[i]; } #endregion #region IEnumerable Members IEnumerator IEnumerable.GetEnumerator() { return ((IEnumerable )this).GetEnumerator(); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
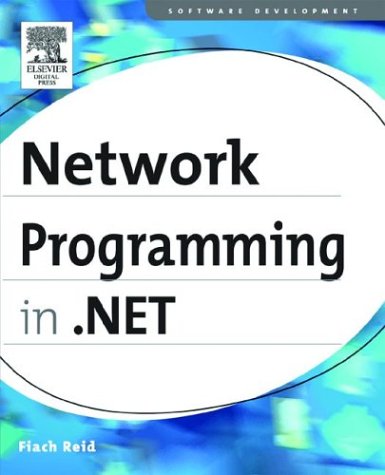
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebPartExportVerb.cs
- SAPIEngineTypes.cs
- DefaultEventAttribute.cs
- SspiNegotiationTokenAuthenticator.cs
- TextElementCollectionHelper.cs
- LOSFormatter.cs
- DataGridViewLinkColumn.cs
- Content.cs
- OperatingSystemVersionCheck.cs
- FontTypeConverter.cs
- EventSourceCreationData.cs
- PaintValueEventArgs.cs
- FileDataSourceCache.cs
- IndexOutOfRangeException.cs
- ProvidePropertyAttribute.cs
- HttpContext.cs
- WindowsTokenRoleProvider.cs
- TextRunCacheImp.cs
- X509Certificate2Collection.cs
- DirectoryObjectSecurity.cs
- InternalConfigHost.cs
- DBCommandBuilder.cs
- CompositeCollectionView.cs
- ContentIterators.cs
- Geometry3D.cs
- ListViewDeleteEventArgs.cs
- TableLayoutStyleCollection.cs
- WorkflowApplicationAbortedException.cs
- ManifestSignatureInformation.cs
- ProgressBarHighlightConverter.cs
- PlaceHolder.cs
- SettingsPropertyValueCollection.cs
- BamlMapTable.cs
- GetPageCompletedEventArgs.cs
- XmlUtf8RawTextWriter.cs
- WaitingCursor.cs
- ResourceDisplayNameAttribute.cs
- IsolationInterop.cs
- StoreContentChangedEventArgs.cs
- SqlVersion.cs
- Quaternion.cs
- EntityObject.cs
- InvariantComparer.cs
- ArrowControl.xaml.cs
- TaskHelper.cs
- KeyedCollection.cs
- HostProtectionPermission.cs
- ScriptResourceAttribute.cs
- StreamWriter.cs
- PageSetupDialog.cs
- Size3D.cs
- StubHelpers.cs
- DesignTimeTemplateParser.cs
- PTConverter.cs
- MimeBasePart.cs
- GenericQueueSurrogate.cs
- BuilderInfo.cs
- ParentUndoUnit.cs
- XmlDataCollection.cs
- HttpServerVarsCollection.cs
- RotateTransform.cs
- InvalidComObjectException.cs
- EmbossBitmapEffect.cs
- ObjectStateFormatter.cs
- Substitution.cs
- MeasureItemEvent.cs
- AdjustableArrowCap.cs
- MessageBox.cs
- HwndProxyElementProvider.cs
- VisualBasicSettingsHandler.cs
- ThreadExceptionEvent.cs
- DefaultMemberAttribute.cs
- ClientScriptItemCollection.cs
- FontFamilyValueSerializer.cs
- PhysicalOps.cs
- CacheOutputQuery.cs
- MachineKeyConverter.cs
- httpstaticobjectscollection.cs
- RepeatInfo.cs
- FlatButtonAppearance.cs
- HTMLTextWriter.cs
- HttpBufferlessInputStream.cs
- CaseStatement.cs
- Point3DCollection.cs
- SystemSounds.cs
- VisualBrush.cs
- ButtonBaseAutomationPeer.cs
- ToolboxComponentsCreatedEventArgs.cs
- ScopelessEnumAttribute.cs
- __Error.cs
- TextMarkerSource.cs
- CssTextWriter.cs
- SqlConnectionPoolGroupProviderInfo.cs
- XpsResource.cs
- ELinqQueryState.cs
- SizeKeyFrameCollection.cs
- DefaultHttpHandler.cs
- XmlElementAttribute.cs
- NativeRecognizer.cs
- HttpListenerPrefixCollection.cs