Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / Net / System / Net / HttpListenerPrefixCollection.cs / 1 / HttpListenerPrefixCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net { using System; using System.Collections; using System.Collections.Generic; internal class ListenerPrefixEnumerator:IEnumerator{ IEnumerator enumerator; internal ListenerPrefixEnumerator(IEnumerator enumerator){ this.enumerator = enumerator; } public string Current{ get{ return (string)enumerator.Current; } } public bool MoveNext(){ return enumerator.MoveNext(); } public void Dispose(){ } void System.Collections.IEnumerator.Reset(){ enumerator.Reset(); } object System.Collections.IEnumerator.Current{ get{ return enumerator.Current; } } } public class HttpListenerPrefixCollection : ICollection { private HttpListener m_HttpListener; internal HttpListenerPrefixCollection(HttpListener listener) { m_HttpListener = listener; } public void CopyTo(Array array, int offset) { m_HttpListener.CheckDisposed(); if (Count>array.Length) { throw new ArgumentOutOfRangeException("array", SR.GetString(SR.net_array_too_small)); } if (offset+Count>array.Length) { throw new ArgumentOutOfRangeException("offset"); } int index = 0; foreach (string uriPrefix in m_HttpListener.m_UriPrefixes.Keys) { array.SetValue(uriPrefix, offset + index++); } } public void CopyTo(string[] array, int offset) { m_HttpListener.CheckDisposed(); if (Count>array.Length) { throw new ArgumentOutOfRangeException("array", SR.GetString(SR.net_array_too_small)); } if (offset+Count>array.Length) { throw new ArgumentOutOfRangeException("offset"); } int index = 0; foreach (string uriPrefix in m_HttpListener.m_UriPrefixes.Keys) { array[offset + index++] = uriPrefix; } } public int Count { get { return m_HttpListener.m_UriPrefixes.Count; } } public bool IsSynchronized { get { return false; } } public bool IsReadOnly { get { return false; } } public void Add(string uriPrefix) { m_HttpListener.AddPrefix(uriPrefix); } public bool Contains(string uriPrefix) { return m_HttpListener.m_UriPrefixes.Contains(uriPrefix); } System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator() { return null; } public IEnumerator GetEnumerator() { return new ListenerPrefixEnumerator(m_HttpListener.m_UriPrefixes.Keys.GetEnumerator()); } public bool Remove(string uriPrefix) { return m_HttpListener.RemovePrefix(uriPrefix); } public void Clear() { m_HttpListener.RemoveAll(true); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net { using System; using System.Collections; using System.Collections.Generic; internal class ListenerPrefixEnumerator:IEnumerator{ IEnumerator enumerator; internal ListenerPrefixEnumerator(IEnumerator enumerator){ this.enumerator = enumerator; } public string Current{ get{ return (string)enumerator.Current; } } public bool MoveNext(){ return enumerator.MoveNext(); } public void Dispose(){ } void System.Collections.IEnumerator.Reset(){ enumerator.Reset(); } object System.Collections.IEnumerator.Current{ get{ return enumerator.Current; } } } public class HttpListenerPrefixCollection : ICollection { private HttpListener m_HttpListener; internal HttpListenerPrefixCollection(HttpListener listener) { m_HttpListener = listener; } public void CopyTo(Array array, int offset) { m_HttpListener.CheckDisposed(); if (Count>array.Length) { throw new ArgumentOutOfRangeException("array", SR.GetString(SR.net_array_too_small)); } if (offset+Count>array.Length) { throw new ArgumentOutOfRangeException("offset"); } int index = 0; foreach (string uriPrefix in m_HttpListener.m_UriPrefixes.Keys) { array.SetValue(uriPrefix, offset + index++); } } public void CopyTo(string[] array, int offset) { m_HttpListener.CheckDisposed(); if (Count>array.Length) { throw new ArgumentOutOfRangeException("array", SR.GetString(SR.net_array_too_small)); } if (offset+Count>array.Length) { throw new ArgumentOutOfRangeException("offset"); } int index = 0; foreach (string uriPrefix in m_HttpListener.m_UriPrefixes.Keys) { array[offset + index++] = uriPrefix; } } public int Count { get { return m_HttpListener.m_UriPrefixes.Count; } } public bool IsSynchronized { get { return false; } } public bool IsReadOnly { get { return false; } } public void Add(string uriPrefix) { m_HttpListener.AddPrefix(uriPrefix); } public bool Contains(string uriPrefix) { return m_HttpListener.m_UriPrefixes.Contains(uriPrefix); } System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator() { return null; } public IEnumerator GetEnumerator() { return new ListenerPrefixEnumerator(m_HttpListener.m_UriPrefixes.Keys.GetEnumerator()); } public bool Remove(string uriPrefix) { return m_HttpListener.RemovePrefix(uriPrefix); } public void Clear() { m_HttpListener.RemoveAll(true); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
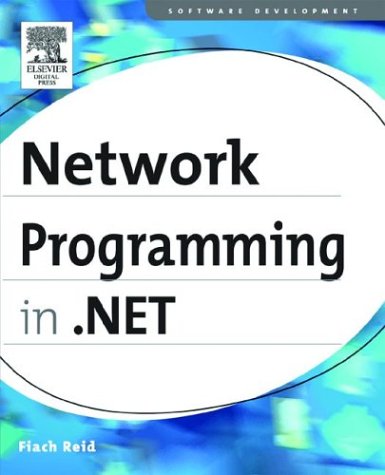
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MatrixIndependentAnimationStorage.cs
- DiscoveryInnerClientAdhocCD1.cs
- OdbcReferenceCollection.cs
- XPathNodePointer.cs
- LexicalChunk.cs
- EntityViewContainer.cs
- FocusTracker.cs
- InternalResources.cs
- EntityDataSourceState.cs
- ToolBarDesigner.cs
- XmlSortKeyAccumulator.cs
- Msec.cs
- TrackingQuery.cs
- SpeakInfo.cs
- Compiler.cs
- MSAAEventDispatcher.cs
- CheckBox.cs
- EdgeProfileValidation.cs
- NetworkCredential.cs
- ConstrainedGroup.cs
- WebPartUtil.cs
- CorrelationRequestContext.cs
- EndpointAddressProcessor.cs
- SqlParameterCollection.cs
- CollectionType.cs
- CombinedGeometry.cs
- OracleMonthSpan.cs
- LinkedList.cs
- _TLSstream.cs
- EditableTreeList.cs
- WebPartTransformerCollection.cs
- AxWrapperGen.cs
- MouseButtonEventArgs.cs
- GridPattern.cs
- UniformGrid.cs
- HttpCacheVaryByContentEncodings.cs
- KeyValueConfigurationElement.cs
- TransformPattern.cs
- UnmanagedHandle.cs
- MobileUITypeEditor.cs
- InteropBitmapSource.cs
- SmiSettersStream.cs
- ClientSideQueueItem.cs
- ClientSideProviderDescription.cs
- SqlDataReader.cs
- ButtonAutomationPeer.cs
- Listbox.cs
- BitSet.cs
- UnsafeNativeMethods.cs
- EventToken.cs
- IDReferencePropertyAttribute.cs
- SerializationHelper.cs
- TheQuery.cs
- itemelement.cs
- Guid.cs
- ContextDataSourceContextData.cs
- DataTemplateKey.cs
- ListItemCollection.cs
- FunctionQuery.cs
- IndexingContentUnit.cs
- StatusBarItem.cs
- CodeLabeledStatement.cs
- EnumerableCollectionView.cs
- FileDialogCustomPlacesCollection.cs
- DataBinder.cs
- TabItem.cs
- MenuItem.cs
- ShapingWorkspace.cs
- LocalBuilder.cs
- Accessible.cs
- QilInvokeEarlyBound.cs
- __FastResourceComparer.cs
- EncodingDataItem.cs
- QilInvoke.cs
- OpCopier.cs
- FacetDescription.cs
- SpAudioStreamWrapper.cs
- ProfileParameter.cs
- WebPartConnection.cs
- FixedTextContainer.cs
- XmlQueryStaticData.cs
- Event.cs
- SimpleHandlerFactory.cs
- WebConfigurationManager.cs
- XslAstAnalyzer.cs
- RequiredFieldValidator.cs
- BridgeDataReader.cs
- HTTPNotFoundHandler.cs
- AngleUtil.cs
- RuleAction.cs
- ListChangedEventArgs.cs
- CodeLabeledStatement.cs
- IChannel.cs
- ErrorWebPart.cs
- CustomDictionarySources.cs
- ConnectionPoolManager.cs
- DWriteFactory.cs
- HttpCapabilitiesEvaluator.cs
- DataControlFieldCollection.cs
- x509utils.cs