Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / clr / src / BCL / System / Collections / ObjectModel / KeyedCollection.cs / 1 / KeyedCollection.cs
namespace System.Collections.ObjectModel { using System; using System.Collections.Generic; using System.Diagnostics; [Serializable()] [System.Runtime.InteropServices.ComVisible(false)] [DebuggerTypeProxy(typeof(Mscorlib_KeyedCollectionDebugView<,>))] [DebuggerDisplay("Count = {Count}")] public abstract class KeyedCollection: Collection { const int defaultThreshold = 0; IEqualityComparer comparer; Dictionary dict; int keyCount; int threshold; protected KeyedCollection(): this(null, defaultThreshold) {} protected KeyedCollection(IEqualityComparer comparer): this(comparer, defaultThreshold) {} protected KeyedCollection(IEqualityComparer comparer, int dictionaryCreationThreshold) { if (comparer == null) { comparer = EqualityComparer .Default; } if (dictionaryCreationThreshold == -1) { dictionaryCreationThreshold = int.MaxValue; } if( dictionaryCreationThreshold < -1) { ThrowHelper.ThrowArgumentOutOfRangeException(ExceptionArgument.dictionaryCreationThreshold, ExceptionResource.ArgumentOutOfRange_InvalidThreshold); } this.comparer = comparer; this.threshold = dictionaryCreationThreshold; } public IEqualityComparer Comparer { get { return comparer; } } public TItem this[TKey key] { get { if( key == null) { ThrowHelper.ThrowArgumentNullException(ExceptionArgument.key); } if (dict != null) { return dict[key]; } foreach (TItem item in Items) { if (comparer.Equals(GetKeyForItem(item), key)) return item; } ThrowHelper.ThrowKeyNotFoundException(); return default(TItem); } } public bool Contains(TKey key) { if( key == null) { ThrowHelper.ThrowArgumentNullException(ExceptionArgument.key); } if (dict != null) { return dict.ContainsKey(key); } if (key != null) { foreach (TItem item in Items) { if (comparer.Equals(GetKeyForItem(item), key)) return true; } } return false; } private bool ContainsItem(TItem item) { TKey key; if( (dict == null) || ((key = GetKeyForItem(item)) == null)) { return Items.Contains(item); } TItem itemInDict; bool exist = dict.TryGetValue(key, out itemInDict); if( exist) { return EqualityComparer .Default.Equals(itemInDict, item); } return false; } public bool Remove(TKey key) { if( key == null) { ThrowHelper.ThrowArgumentNullException(ExceptionArgument.key); } if (dict != null) { if (dict.ContainsKey(key)) { return Remove(dict[key]); } return false; } if (key != null) { for (int i = 0; i < Items.Count; i++) { if (comparer.Equals(GetKeyForItem(Items[i]), key)) { RemoveItem(i); return true; } } } return false; } protected IDictionary Dictionary { get { return dict; } } protected void ChangeItemKey(TItem item, TKey newKey) { // check if the item exists in the collection if( !ContainsItem(item)) { ThrowHelper.ThrowArgumentException(ExceptionResource.Argument_ItemNotExist); } TKey oldKey = GetKeyForItem(item); if (!comparer.Equals(oldKey, newKey)) { if (newKey != null) { AddKey(newKey, item); } if (oldKey != null) { RemoveKey(oldKey); } } } protected override void ClearItems() { base.ClearItems(); if (dict != null) { dict.Clear(); } keyCount = 0; } protected abstract TKey GetKeyForItem(TItem item); protected override void InsertItem(int index, TItem item) { TKey key = GetKeyForItem(item); if (key != null) { AddKey(key, item); } base.InsertItem(index, item); } protected override void RemoveItem(int index) { TKey key = GetKeyForItem(Items[index]); if (key != null) { RemoveKey(key); } base.RemoveItem(index); } protected override void SetItem(int index, TItem item) { TKey newKey = GetKeyForItem(item); TKey oldKey = GetKeyForItem(Items[index]); if (comparer.Equals(oldKey, newKey)) { if (newKey != null && dict != null) { dict[newKey] = item; } } else { if (newKey != null) { AddKey(newKey, item); } if (oldKey != null) { RemoveKey(oldKey); } } base.SetItem(index, item); } private void AddKey(TKey key, TItem item) { if (dict != null) { dict.Add(key, item); } else if (keyCount == threshold) { CreateDictionary(); dict.Add(key, item); } else { if (Contains(key)) { ThrowHelper.ThrowArgumentException(ExceptionResource.Argument_AddingDuplicate); } keyCount++; } } private void CreateDictionary() { dict = new Dictionary (comparer); foreach (TItem item in Items) { TKey key = GetKeyForItem(item); if (key != null) { dict.Add(key, item); } } } private void RemoveKey(TKey key) { BCLDebug.Assert(key != null, "key shouldn't be null!"); if (dict != null) { dict.Remove(key); } else { keyCount--; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. namespace System.Collections.ObjectModel { using System; using System.Collections.Generic; using System.Diagnostics; [Serializable()] [System.Runtime.InteropServices.ComVisible(false)] [DebuggerTypeProxy(typeof(Mscorlib_KeyedCollectionDebugView<,>))] [DebuggerDisplay("Count = {Count}")] public abstract class KeyedCollection : Collection { const int defaultThreshold = 0; IEqualityComparer comparer; Dictionary dict; int keyCount; int threshold; protected KeyedCollection(): this(null, defaultThreshold) {} protected KeyedCollection(IEqualityComparer comparer): this(comparer, defaultThreshold) {} protected KeyedCollection(IEqualityComparer comparer, int dictionaryCreationThreshold) { if (comparer == null) { comparer = EqualityComparer .Default; } if (dictionaryCreationThreshold == -1) { dictionaryCreationThreshold = int.MaxValue; } if( dictionaryCreationThreshold < -1) { ThrowHelper.ThrowArgumentOutOfRangeException(ExceptionArgument.dictionaryCreationThreshold, ExceptionResource.ArgumentOutOfRange_InvalidThreshold); } this.comparer = comparer; this.threshold = dictionaryCreationThreshold; } public IEqualityComparer Comparer { get { return comparer; } } public TItem this[TKey key] { get { if( key == null) { ThrowHelper.ThrowArgumentNullException(ExceptionArgument.key); } if (dict != null) { return dict[key]; } foreach (TItem item in Items) { if (comparer.Equals(GetKeyForItem(item), key)) return item; } ThrowHelper.ThrowKeyNotFoundException(); return default(TItem); } } public bool Contains(TKey key) { if( key == null) { ThrowHelper.ThrowArgumentNullException(ExceptionArgument.key); } if (dict != null) { return dict.ContainsKey(key); } if (key != null) { foreach (TItem item in Items) { if (comparer.Equals(GetKeyForItem(item), key)) return true; } } return false; } private bool ContainsItem(TItem item) { TKey key; if( (dict == null) || ((key = GetKeyForItem(item)) == null)) { return Items.Contains(item); } TItem itemInDict; bool exist = dict.TryGetValue(key, out itemInDict); if( exist) { return EqualityComparer .Default.Equals(itemInDict, item); } return false; } public bool Remove(TKey key) { if( key == null) { ThrowHelper.ThrowArgumentNullException(ExceptionArgument.key); } if (dict != null) { if (dict.ContainsKey(key)) { return Remove(dict[key]); } return false; } if (key != null) { for (int i = 0; i < Items.Count; i++) { if (comparer.Equals(GetKeyForItem(Items[i]), key)) { RemoveItem(i); return true; } } } return false; } protected IDictionary Dictionary { get { return dict; } } protected void ChangeItemKey(TItem item, TKey newKey) { // check if the item exists in the collection if( !ContainsItem(item)) { ThrowHelper.ThrowArgumentException(ExceptionResource.Argument_ItemNotExist); } TKey oldKey = GetKeyForItem(item); if (!comparer.Equals(oldKey, newKey)) { if (newKey != null) { AddKey(newKey, item); } if (oldKey != null) { RemoveKey(oldKey); } } } protected override void ClearItems() { base.ClearItems(); if (dict != null) { dict.Clear(); } keyCount = 0; } protected abstract TKey GetKeyForItem(TItem item); protected override void InsertItem(int index, TItem item) { TKey key = GetKeyForItem(item); if (key != null) { AddKey(key, item); } base.InsertItem(index, item); } protected override void RemoveItem(int index) { TKey key = GetKeyForItem(Items[index]); if (key != null) { RemoveKey(key); } base.RemoveItem(index); } protected override void SetItem(int index, TItem item) { TKey newKey = GetKeyForItem(item); TKey oldKey = GetKeyForItem(Items[index]); if (comparer.Equals(oldKey, newKey)) { if (newKey != null && dict != null) { dict[newKey] = item; } } else { if (newKey != null) { AddKey(newKey, item); } if (oldKey != null) { RemoveKey(oldKey); } } base.SetItem(index, item); } private void AddKey(TKey key, TItem item) { if (dict != null) { dict.Add(key, item); } else if (keyCount == threshold) { CreateDictionary(); dict.Add(key, item); } else { if (Contains(key)) { ThrowHelper.ThrowArgumentException(ExceptionResource.Argument_AddingDuplicate); } keyCount++; } } private void CreateDictionary() { dict = new Dictionary (comparer); foreach (TItem item in Items) { TKey key = GetKeyForItem(item); if (key != null) { dict.Add(key, item); } } } private void RemoveKey(TKey key) { BCLDebug.Assert(key != null, "key shouldn't be null!"); if (dict != null) { dict.Remove(key); } else { keyCount--; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
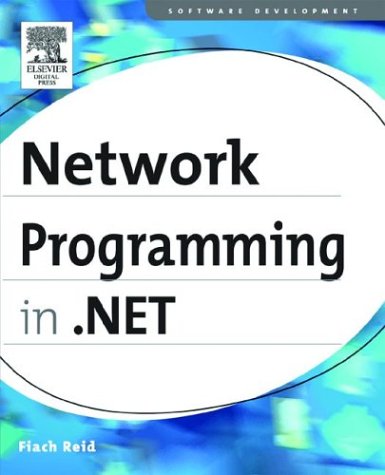
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- xml.cs
- Events.cs
- DataGridViewRowConverter.cs
- SqlMethodTransformer.cs
- BorderGapMaskConverter.cs
- XPathMessageFilterElementComparer.cs
- Psha1DerivedKeyGeneratorHelper.cs
- GridPattern.cs
- UTF7Encoding.cs
- connectionpool.cs
- PngBitmapEncoder.cs
- XamlSerializationHelper.cs
- SqlUserDefinedTypeAttribute.cs
- EntryPointNotFoundException.cs
- TagPrefixCollection.cs
- TimeoutValidationAttribute.cs
- InvalidOleVariantTypeException.cs
- InputLanguageEventArgs.cs
- SecurityTokenParametersEnumerable.cs
- Typography.cs
- GPRECT.cs
- LinkGrep.cs
- Input.cs
- AsyncResult.cs
- SqlCrossApplyToCrossJoin.cs
- RpcCryptoRequest.cs
- HtmlTitle.cs
- AlgoModule.cs
- ScrollItemPattern.cs
- CodeCatchClauseCollection.cs
- VirtualPathUtility.cs
- Point3DKeyFrameCollection.cs
- LogicalChannelCollection.cs
- InstanceContextManager.cs
- SecurityResources.cs
- HandlerBase.cs
- RegularExpressionValidator.cs
- UIHelper.cs
- TextEffect.cs
- ProviderSettingsCollection.cs
- KnownBoxes.cs
- SchemaInfo.cs
- DefaultPrintController.cs
- GradientStop.cs
- FreezableCollection.cs
- Tool.cs
- TextChangedEventArgs.cs
- WebEncodingValidatorAttribute.cs
- XhtmlBasicPanelAdapter.cs
- XslException.cs
- XmlAutoDetectWriter.cs
- PropertyGroupDescription.cs
- RequestUriProcessor.cs
- SchemaHelper.cs
- UdpTransportBindingElement.cs
- BooleanProjectedSlot.cs
- ErrorStyle.cs
- WindowsListViewGroupHelper.cs
- LinqDataSourceHelper.cs
- ScaleTransform3D.cs
- StatusBarAutomationPeer.cs
- DocumentPropertiesDialog.cs
- JsonUriDataContract.cs
- PathSegmentCollection.cs
- SymLanguageType.cs
- AccessibilityHelperForXpWin2k3.cs
- RIPEMD160Managed.cs
- TaiwanCalendar.cs
- CustomAttributeBuilder.cs
- GridViewCancelEditEventArgs.cs
- SubpageParagraph.cs
- HwndHost.cs
- PointAnimationBase.cs
- CompilerResults.cs
- Brush.cs
- shaperfactoryquerycachekey.cs
- CounterSample.cs
- HighlightComponent.cs
- ObjectViewEntityCollectionData.cs
- DataGridViewCellStyle.cs
- NoClickablePointException.cs
- DataSourceSelectArguments.cs
- SortKey.cs
- ValidatingPropertiesEventArgs.cs
- DbDataSourceEnumerator.cs
- InternalConfigSettingsFactory.cs
- DataGridCellsPanel.cs
- WpfKnownType.cs
- ApplicationFileCodeDomTreeGenerator.cs
- ToolStripItemRenderEventArgs.cs
- StickyNoteContentControl.cs
- UnionCodeGroup.cs
- SurrogateSelector.cs
- GenericWebPart.cs
- CodeStatement.cs
- QuerySubExprEliminator.cs
- ByeMessageApril2005.cs
- UnsafeNativeMethods.cs
- _SslStream.cs
- ContentWrapperAttribute.cs