Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Ink / Events.cs / 1 / Events.cs
using System; using System.Windows.Input; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace System.Windows.Ink { #region Public APIs // ============================================================================================ ////// delegate used for event handlers that are called when a stroke was was added, removed, or modified inside of a Stroke collection /// public delegate void StrokeCollectionChangedEventHandler(object sender, StrokeCollectionChangedEventArgs e); ////// Event arg used when delegate a stroke is was added, removed, or modified inside of a Stroke collection /// public class StrokeCollectionChangedEventArgs : EventArgs { private StrokeCollection.ReadOnlyStrokeCollection _added; private StrokeCollection.ReadOnlyStrokeCollection _removed; private int _index = -1; ///Constructor internal StrokeCollectionChangedEventArgs(StrokeCollection added, StrokeCollection removed, int index) : this(added, removed) { _index = index; } ///Constructor public StrokeCollectionChangedEventArgs(StrokeCollection added, StrokeCollection removed) { if ( added == null && removed == null ) { throw new ArgumentException(SR.Get(SRID.CannotBothBeNull, "added", "removed")); } _added = ( added == null ) ? null : new StrokeCollection.ReadOnlyStrokeCollection(added); _removed = ( removed == null ) ? null : new StrokeCollection.ReadOnlyStrokeCollection(removed); } ///Set of strokes that where added, result may be an empty collection public StrokeCollection Added { get { if ( _added == null ) { _added = new StrokeCollection.ReadOnlyStrokeCollection(new StrokeCollection()); } return _added; } } ///Set of strokes that where removed, result may be an empty collection public StrokeCollection Removed { get { if ( _removed == null ) { _removed = new StrokeCollection.ReadOnlyStrokeCollection(new StrokeCollection()); } return _removed; } } ////// The zero based starting index that was affected /// internal int Index { get { return _index; } } } // =========================================================================================== ////// delegate used for event handlers that are called when a change to the drawing attributes associated with one or more strokes has occurred. /// public delegate void PropertyDataChangedEventHandler(object sender, PropertyDataChangedEventArgs e); ////// Event arg used a change to the drawing attributes associated with one or more strokes has occurred. /// public class PropertyDataChangedEventArgs : EventArgs { private Guid _propertyGuid; private object _newValue; private object _previousValue; ///Constructor public PropertyDataChangedEventArgs(Guid propertyGuid, object newValue, object previousValue) { if ( newValue == null && previousValue == null ) { throw new ArgumentException(SR.Get(SRID.CannotBothBeNull, "newValue", "previousValue")); } _propertyGuid = propertyGuid; _newValue = newValue; _previousValue = previousValue; } ////// Gets the property guid that represents the DrawingAttribute that changed /// public Guid PropertyGuid { get { return _propertyGuid; } } ////// Gets the new value of the DrawingAttribute /// public object NewValue { get { return _newValue; } } ////// Gets the previous value of the DrawingAttribute /// public object PreviousValue { get { return _previousValue; } } } // =========================================================================================== ////// delegate used for event handlers that are called when the Custom attributes associated with an object have changed. /// internal delegate void ExtendedPropertiesChangedEventHandler(object sender, ExtendedPropertiesChangedEventArgs e); ////// Event Arg used when the Custom attributes associated with an object have changed. /// internal class ExtendedPropertiesChangedEventArgs : EventArgs { private ExtendedProperty _oldProperty; private ExtendedProperty _newProperty; ///Constructor internal ExtendedPropertiesChangedEventArgs(ExtendedProperty oldProperty, ExtendedProperty newProperty) { if ( oldProperty == null && newProperty == null ) { throw new ArgumentNullException("oldProperty"); } _oldProperty = oldProperty; _newProperty = newProperty; } ////// The value of the previous property. If the Changed event was caused /// by an ExtendedProperty being added, this value is null /// internal ExtendedProperty OldProperty { get { return _oldProperty; } } ////// The value of the new property. If the Changed event was caused by /// an ExtendedProperty being removed, this value is null /// internal ExtendedProperty NewProperty { get { return _newProperty; } } } ////// The delegate to use for the DefaultDrawingAttributesReplaced event /// public delegate void DrawingAttributesReplacedEventHandler(object sender, DrawingAttributesReplacedEventArgs e); ////// DrawingAttributesReplacedEventArgs /// public class DrawingAttributesReplacedEventArgs : EventArgs { ////// DrawingAttributesReplacedEventArgs /// ////// This must be public so InkCanvas can instance it /// public DrawingAttributesReplacedEventArgs(DrawingAttributes newDrawingAttributes, DrawingAttributes previousDrawingAttributes) { if ( newDrawingAttributes == null ) { throw new ArgumentNullException("newDrawingAttributes"); } if ( previousDrawingAttributes == null ) { throw new ArgumentNullException("previousDrawingAttributes"); } _newDrawingAttributes = newDrawingAttributes; _previousDrawingAttributes = previousDrawingAttributes; } ////// [TBS] /// public DrawingAttributes NewDrawingAttributes { get { return _newDrawingAttributes; } } ////// [TBS] /// public DrawingAttributes PreviousDrawingAttributes { get { return _previousDrawingAttributes; } } private DrawingAttributes _newDrawingAttributes; private DrawingAttributes _previousDrawingAttributes; } ////// The delegate to use for the StylusPointsReplaced event /// public delegate void StylusPointsReplacedEventHandler(object sender, StylusPointsReplacedEventArgs e); ////// StylusPointsReplacedEventArgs /// public class StylusPointsReplacedEventArgs : EventArgs { ////// StylusPointsReplacedEventArgs /// ////// This must be public so InkCanvas can instance it /// public StylusPointsReplacedEventArgs(StylusPointCollection newStylusPoints, StylusPointCollection previousStylusPoints) { if ( newStylusPoints == null ) { throw new ArgumentNullException("newStylusPoints"); } if ( previousStylusPoints == null ) { throw new ArgumentNullException("previousStylusPoints"); } _newStylusPoints = newStylusPoints; _previousStylusPoints = previousStylusPoints; } ////// [TBS] /// public StylusPointCollection NewStylusPoints { get { return _newStylusPoints; } } ////// [TBS] /// public StylusPointCollection PreviousStylusPoints { get { return _previousStylusPoints; } } private StylusPointCollection _newStylusPoints; private StylusPointCollection _previousStylusPoints; } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Windows.Input; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace System.Windows.Ink { #region Public APIs // ============================================================================================ ////// delegate used for event handlers that are called when a stroke was was added, removed, or modified inside of a Stroke collection /// public delegate void StrokeCollectionChangedEventHandler(object sender, StrokeCollectionChangedEventArgs e); ////// Event arg used when delegate a stroke is was added, removed, or modified inside of a Stroke collection /// public class StrokeCollectionChangedEventArgs : EventArgs { private StrokeCollection.ReadOnlyStrokeCollection _added; private StrokeCollection.ReadOnlyStrokeCollection _removed; private int _index = -1; ///Constructor internal StrokeCollectionChangedEventArgs(StrokeCollection added, StrokeCollection removed, int index) : this(added, removed) { _index = index; } ///Constructor public StrokeCollectionChangedEventArgs(StrokeCollection added, StrokeCollection removed) { if ( added == null && removed == null ) { throw new ArgumentException(SR.Get(SRID.CannotBothBeNull, "added", "removed")); } _added = ( added == null ) ? null : new StrokeCollection.ReadOnlyStrokeCollection(added); _removed = ( removed == null ) ? null : new StrokeCollection.ReadOnlyStrokeCollection(removed); } ///Set of strokes that where added, result may be an empty collection public StrokeCollection Added { get { if ( _added == null ) { _added = new StrokeCollection.ReadOnlyStrokeCollection(new StrokeCollection()); } return _added; } } ///Set of strokes that where removed, result may be an empty collection public StrokeCollection Removed { get { if ( _removed == null ) { _removed = new StrokeCollection.ReadOnlyStrokeCollection(new StrokeCollection()); } return _removed; } } ////// The zero based starting index that was affected /// internal int Index { get { return _index; } } } // =========================================================================================== ////// delegate used for event handlers that are called when a change to the drawing attributes associated with one or more strokes has occurred. /// public delegate void PropertyDataChangedEventHandler(object sender, PropertyDataChangedEventArgs e); ////// Event arg used a change to the drawing attributes associated with one or more strokes has occurred. /// public class PropertyDataChangedEventArgs : EventArgs { private Guid _propertyGuid; private object _newValue; private object _previousValue; ///Constructor public PropertyDataChangedEventArgs(Guid propertyGuid, object newValue, object previousValue) { if ( newValue == null && previousValue == null ) { throw new ArgumentException(SR.Get(SRID.CannotBothBeNull, "newValue", "previousValue")); } _propertyGuid = propertyGuid; _newValue = newValue; _previousValue = previousValue; } ////// Gets the property guid that represents the DrawingAttribute that changed /// public Guid PropertyGuid { get { return _propertyGuid; } } ////// Gets the new value of the DrawingAttribute /// public object NewValue { get { return _newValue; } } ////// Gets the previous value of the DrawingAttribute /// public object PreviousValue { get { return _previousValue; } } } // =========================================================================================== ////// delegate used for event handlers that are called when the Custom attributes associated with an object have changed. /// internal delegate void ExtendedPropertiesChangedEventHandler(object sender, ExtendedPropertiesChangedEventArgs e); ////// Event Arg used when the Custom attributes associated with an object have changed. /// internal class ExtendedPropertiesChangedEventArgs : EventArgs { private ExtendedProperty _oldProperty; private ExtendedProperty _newProperty; ///Constructor internal ExtendedPropertiesChangedEventArgs(ExtendedProperty oldProperty, ExtendedProperty newProperty) { if ( oldProperty == null && newProperty == null ) { throw new ArgumentNullException("oldProperty"); } _oldProperty = oldProperty; _newProperty = newProperty; } ////// The value of the previous property. If the Changed event was caused /// by an ExtendedProperty being added, this value is null /// internal ExtendedProperty OldProperty { get { return _oldProperty; } } ////// The value of the new property. If the Changed event was caused by /// an ExtendedProperty being removed, this value is null /// internal ExtendedProperty NewProperty { get { return _newProperty; } } } ////// The delegate to use for the DefaultDrawingAttributesReplaced event /// public delegate void DrawingAttributesReplacedEventHandler(object sender, DrawingAttributesReplacedEventArgs e); ////// DrawingAttributesReplacedEventArgs /// public class DrawingAttributesReplacedEventArgs : EventArgs { ////// DrawingAttributesReplacedEventArgs /// ////// This must be public so InkCanvas can instance it /// public DrawingAttributesReplacedEventArgs(DrawingAttributes newDrawingAttributes, DrawingAttributes previousDrawingAttributes) { if ( newDrawingAttributes == null ) { throw new ArgumentNullException("newDrawingAttributes"); } if ( previousDrawingAttributes == null ) { throw new ArgumentNullException("previousDrawingAttributes"); } _newDrawingAttributes = newDrawingAttributes; _previousDrawingAttributes = previousDrawingAttributes; } ////// [TBS] /// public DrawingAttributes NewDrawingAttributes { get { return _newDrawingAttributes; } } ////// [TBS] /// public DrawingAttributes PreviousDrawingAttributes { get { return _previousDrawingAttributes; } } private DrawingAttributes _newDrawingAttributes; private DrawingAttributes _previousDrawingAttributes; } ////// The delegate to use for the StylusPointsReplaced event /// public delegate void StylusPointsReplacedEventHandler(object sender, StylusPointsReplacedEventArgs e); ////// StylusPointsReplacedEventArgs /// public class StylusPointsReplacedEventArgs : EventArgs { ////// StylusPointsReplacedEventArgs /// ////// This must be public so InkCanvas can instance it /// public StylusPointsReplacedEventArgs(StylusPointCollection newStylusPoints, StylusPointCollection previousStylusPoints) { if ( newStylusPoints == null ) { throw new ArgumentNullException("newStylusPoints"); } if ( previousStylusPoints == null ) { throw new ArgumentNullException("previousStylusPoints"); } _newStylusPoints = newStylusPoints; _previousStylusPoints = previousStylusPoints; } ////// [TBS] /// public StylusPointCollection NewStylusPoints { get { return _newStylusPoints; } } ////// [TBS] /// public StylusPointCollection PreviousStylusPoints { get { return _previousStylusPoints; } } private StylusPointCollection _newStylusPoints; private StylusPointCollection _previousStylusPoints; } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
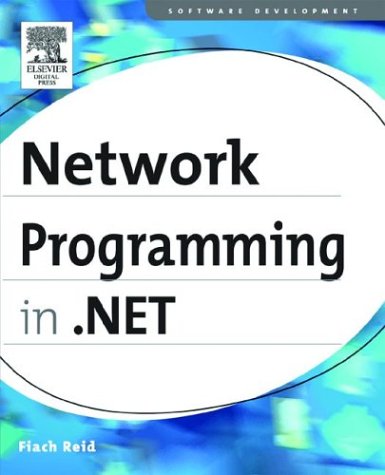
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ConnectionInterfaceCollection.cs
- SafeSystemMetrics.cs
- ContainerUtilities.cs
- DataColumnCollection.cs
- SqlCharStream.cs
- StrongNameIdentityPermission.cs
- FlagsAttribute.cs
- ConversionContext.cs
- UshortList2.cs
- FontStyles.cs
- OpacityConverter.cs
- Point3DAnimation.cs
- ModelItemDictionary.cs
- XmlEntityReference.cs
- ToolbarAUtomationPeer.cs
- FamilyTypefaceCollection.cs
- TemplateXamlTreeBuilder.cs
- ParallelDesigner.cs
- PropertyPath.cs
- RuntimeVariablesExpression.cs
- ReaderWriterLockWrapper.cs
- SqlResolver.cs
- ValidationErrorInfo.cs
- CollectionViewGroup.cs
- SelectionRangeConverter.cs
- ListSortDescriptionCollection.cs
- ScriptingRoleServiceSection.cs
- PropertyRef.cs
- StringInfo.cs
- ScaleTransform.cs
- ViewValidator.cs
- DataGridViewComboBoxColumnDesigner.cs
- GridViewColumnHeaderAutomationPeer.cs
- FormatterServices.cs
- ListParaClient.cs
- SqlDataSourceCommandParser.cs
- CryptoHelper.cs
- __Error.cs
- File.cs
- GridView.cs
- ZoneLinkButton.cs
- NegatedConstant.cs
- GC.cs
- ReferencedCollectionType.cs
- TreeViewItem.cs
- GenericArgumentsUpdater.cs
- ViewManager.cs
- CompilationPass2TaskInternal.cs
- WebPartDisplayMode.cs
- GridViewEditEventArgs.cs
- ContextProperty.cs
- HtmlInputRadioButton.cs
- TableLayoutStyle.cs
- Int64.cs
- SurrogateEncoder.cs
- ScriptResourceDefinition.cs
- TrackingMemoryStream.cs
- Condition.cs
- DependencyPropertyDescriptor.cs
- ExpressionBindingCollection.cs
- TableLayoutPanel.cs
- FaultHandlingFilter.cs
- FormViewPageEventArgs.cs
- HtmlInputRadioButton.cs
- ZipPackagePart.cs
- PeerObject.cs
- DeviceSpecificDesigner.cs
- TableLayoutSettingsTypeConverter.cs
- CurrentChangingEventArgs.cs
- ClientConfigPaths.cs
- PointAnimationClockResource.cs
- NumericUpDownAcceleration.cs
- XmlRootAttribute.cs
- Code.cs
- ComboBox.cs
- DataViewListener.cs
- Grid.cs
- MatrixValueSerializer.cs
- SoapProtocolImporter.cs
- AssociationSetMetadata.cs
- IndexedGlyphRun.cs
- Model3DGroup.cs
- QueryOptionExpression.cs
- Bits.cs
- ControlEvent.cs
- WebServiceAttribute.cs
- RequestCacheValidator.cs
- XmlWellformedWriter.cs
- ClearCollection.cs
- Process.cs
- UidManager.cs
- PerfCounters.cs
- LayoutSettings.cs
- TextWriterTraceListener.cs
- ScrollBarRenderer.cs
- ListDataBindEventArgs.cs
- EventHandlersStore.cs
- EncodingInfo.cs
- Interlocked.cs
- LocalizableResourceBuilder.cs