Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / Condition.cs / 1 / Condition.cs
using MS.Utility; using System.IO; using System.Windows.Markup; using System.ComponentModel; using System; using System.Windows.Data; namespace System.Windows { ////// Condition for a multiple property or data trigger /// public sealed class Condition { ////// Constructor with no property reference nor value /// public Condition() { _property = null; _binding = null; } ////// Constructor for creating a Condition /// public Condition( DependencyProperty conditionProperty, object conditionValue ) : this(conditionProperty, conditionValue, null) { // Call Forwarded } ////// Constructor for creating a Condition with the given property /// and value instead of creating an empty one and setting values later. /// ////// This constructor does parameter validation, which before doesn't /// happen until Seal() is called. We can do it here because we get /// both at the same time. /// public Condition( DependencyProperty conditionProperty, object conditionValue, string sourceName ) { if( conditionProperty == null ) { throw new ArgumentNullException("conditionProperty"); } if( !conditionProperty.IsValidValue( conditionValue ) ) { throw new ArgumentException(SR.Get(SRID.InvalidPropertyValue, conditionValue, conditionProperty.Name)); } _property = conditionProperty; Value = conditionValue; _sourceName = sourceName; } ////// Constructor for creating a Condition with the given binding declaration. /// and value. /// public Condition( BindingBase binding, object conditionValue ) { if( binding == null ) { throw new ArgumentNullException("binding"); } Binding = binding; Value = conditionValue; } ////// DepedencyProperty of the conditional /// [Ambient] [DefaultValue(null)] public DependencyProperty Property { get { return _property; } set { if (_sealed) { throw new InvalidOperationException(SR.Get(SRID.CannotChangeAfterSealed, "Condition")); } if( _binding != null ) { throw new InvalidOperationException(SR.Get(SRID.ConditionCannotUseBothPropertyAndBinding)); } _property = value; } } ////// Binding of the conditional /// [DefaultValue(null)] public BindingBase Binding { get { return _binding; } set { if (_sealed) { throw new InvalidOperationException(SR.Get(SRID.CannotChangeAfterSealed, "Condition")); } if( _property != null ) { throw new InvalidOperationException(SR.Get(SRID.ConditionCannotUseBothPropertyAndBinding)); } _binding = value; } } ////// Value of the condition (equality check) /// public object Value { get { return _value; } set { if (_sealed) { throw new InvalidOperationException(SR.Get(SRID.CannotChangeAfterSealed, "Condition")); } if (value is MarkupExtension) { throw new ArgumentException(SR.Get(SRID.ConditionValueOfMarkupExtensionNotSupported, value.GetType().Name)); } if( value is Expression ) { throw new ArgumentException(SR.Get(SRID.ConditionValueOfExpressionNotSupported)); } _value = value; } } ////// The x:Name of the object whose property shall /// trigger the associated setters to be applied. /// If null, then this is the object being Styled /// and not anything under its Template Tree. /// [DefaultValue(null)] public string SourceName { get { return _sourceName; } set { if( _sealed ) { throw new InvalidOperationException(SR.Get(SRID.CannotChangeAfterSealed, "Condition")); } _sourceName = value; } } ////// Seal the condition so that it can no longer be modified /// internal void Seal(ValueLookupType type) { if (_sealed) { return; } _sealed = true; // Ensure valid condition if (_property != null && _binding != null) throw new InvalidOperationException(SR.Get(SRID.ConditionCannotUseBothPropertyAndBinding)); switch (type) { case ValueLookupType.Trigger: case ValueLookupType.PropertyTriggerResource: if (_property == null) { throw new InvalidOperationException(SR.Get(SRID.NullPropertyIllegal, "Property")); } if (!_property.IsValidValue(_value)) { throw new InvalidOperationException(SR.Get(SRID.InvalidPropertyValue, _value, _property.Name)); } break; case ValueLookupType.DataTrigger: case ValueLookupType.DataTriggerResource: if (_binding == null) { throw new InvalidOperationException(SR.Get(SRID.NullPropertyIllegal, "Binding")); } break; default: throw new InvalidOperationException(SR.Get(SRID.UnexpectedValueTypeForCondition, type)); } // Freeze the condition value StyleHelper.SealIfSealable(_value); } private bool _sealed = false; private DependencyProperty _property; private BindingBase _binding; private object _value = DependencyProperty.UnsetValue; private string _sourceName = null; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using MS.Utility; using System.IO; using System.Windows.Markup; using System.ComponentModel; using System; using System.Windows.Data; namespace System.Windows { ////// Condition for a multiple property or data trigger /// public sealed class Condition { ////// Constructor with no property reference nor value /// public Condition() { _property = null; _binding = null; } ////// Constructor for creating a Condition /// public Condition( DependencyProperty conditionProperty, object conditionValue ) : this(conditionProperty, conditionValue, null) { // Call Forwarded } ////// Constructor for creating a Condition with the given property /// and value instead of creating an empty one and setting values later. /// ////// This constructor does parameter validation, which before doesn't /// happen until Seal() is called. We can do it here because we get /// both at the same time. /// public Condition( DependencyProperty conditionProperty, object conditionValue, string sourceName ) { if( conditionProperty == null ) { throw new ArgumentNullException("conditionProperty"); } if( !conditionProperty.IsValidValue( conditionValue ) ) { throw new ArgumentException(SR.Get(SRID.InvalidPropertyValue, conditionValue, conditionProperty.Name)); } _property = conditionProperty; Value = conditionValue; _sourceName = sourceName; } ////// Constructor for creating a Condition with the given binding declaration. /// and value. /// public Condition( BindingBase binding, object conditionValue ) { if( binding == null ) { throw new ArgumentNullException("binding"); } Binding = binding; Value = conditionValue; } ////// DepedencyProperty of the conditional /// [Ambient] [DefaultValue(null)] public DependencyProperty Property { get { return _property; } set { if (_sealed) { throw new InvalidOperationException(SR.Get(SRID.CannotChangeAfterSealed, "Condition")); } if( _binding != null ) { throw new InvalidOperationException(SR.Get(SRID.ConditionCannotUseBothPropertyAndBinding)); } _property = value; } } ////// Binding of the conditional /// [DefaultValue(null)] public BindingBase Binding { get { return _binding; } set { if (_sealed) { throw new InvalidOperationException(SR.Get(SRID.CannotChangeAfterSealed, "Condition")); } if( _property != null ) { throw new InvalidOperationException(SR.Get(SRID.ConditionCannotUseBothPropertyAndBinding)); } _binding = value; } } ////// Value of the condition (equality check) /// public object Value { get { return _value; } set { if (_sealed) { throw new InvalidOperationException(SR.Get(SRID.CannotChangeAfterSealed, "Condition")); } if (value is MarkupExtension) { throw new ArgumentException(SR.Get(SRID.ConditionValueOfMarkupExtensionNotSupported, value.GetType().Name)); } if( value is Expression ) { throw new ArgumentException(SR.Get(SRID.ConditionValueOfExpressionNotSupported)); } _value = value; } } ////// The x:Name of the object whose property shall /// trigger the associated setters to be applied. /// If null, then this is the object being Styled /// and not anything under its Template Tree. /// [DefaultValue(null)] public string SourceName { get { return _sourceName; } set { if( _sealed ) { throw new InvalidOperationException(SR.Get(SRID.CannotChangeAfterSealed, "Condition")); } _sourceName = value; } } ////// Seal the condition so that it can no longer be modified /// internal void Seal(ValueLookupType type) { if (_sealed) { return; } _sealed = true; // Ensure valid condition if (_property != null && _binding != null) throw new InvalidOperationException(SR.Get(SRID.ConditionCannotUseBothPropertyAndBinding)); switch (type) { case ValueLookupType.Trigger: case ValueLookupType.PropertyTriggerResource: if (_property == null) { throw new InvalidOperationException(SR.Get(SRID.NullPropertyIllegal, "Property")); } if (!_property.IsValidValue(_value)) { throw new InvalidOperationException(SR.Get(SRID.InvalidPropertyValue, _value, _property.Name)); } break; case ValueLookupType.DataTrigger: case ValueLookupType.DataTriggerResource: if (_binding == null) { throw new InvalidOperationException(SR.Get(SRID.NullPropertyIllegal, "Binding")); } break; default: throw new InvalidOperationException(SR.Get(SRID.UnexpectedValueTypeForCondition, type)); } // Freeze the condition value StyleHelper.SealIfSealable(_value); } private bool _sealed = false; private DependencyProperty _property; private BindingBase _binding; private object _value = DependencyProperty.UnsetValue; private string _sourceName = null; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
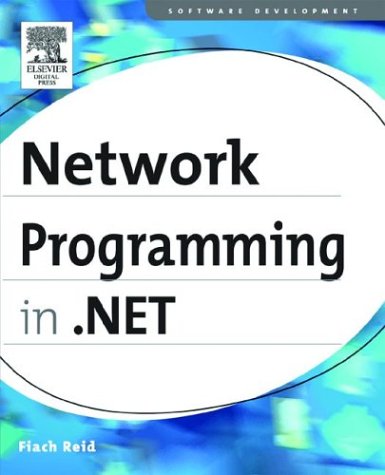
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SubqueryRules.cs
- InputLangChangeEvent.cs
- TripleDESCryptoServiceProvider.cs
- WebPartConnectionsCancelVerb.cs
- InputLanguage.cs
- DefaultValueAttribute.cs
- TransactionException.cs
- FormDocumentDesigner.cs
- UriSectionReader.cs
- Cursors.cs
- Control.cs
- VisualBrush.cs
- MaskedTextBox.cs
- LogEntryHeaderSerializer.cs
- FindRequestContext.cs
- InheritanceAttribute.cs
- WorkflowInlining.cs
- Thumb.cs
- ToolTipAutomationPeer.cs
- SmtpException.cs
- CheckBoxPopupAdapter.cs
- securitycriticaldata.cs
- Grammar.cs
- ObjectDataSource.cs
- DockingAttribute.cs
- CallSiteOps.cs
- ControllableStoryboardAction.cs
- DummyDataSource.cs
- LayoutTable.cs
- DbCommandDefinition.cs
- SystemNetHelpers.cs
- BuildProviderAppliesToAttribute.cs
- SyntaxCheck.cs
- XmlSchemaSubstitutionGroup.cs
- SessionPageStatePersister.cs
- BufferedReadStream.cs
- PackagePart.cs
- FrugalList.cs
- HttpRequestBase.cs
- VirtualPath.cs
- InternalResources.cs
- Byte.cs
- IResourceProvider.cs
- ClientTargetSection.cs
- TreeNodeStyle.cs
- AffineTransform3D.cs
- MessageRpc.cs
- ClrProviderManifest.cs
- UseAttributeSetsAction.cs
- WpfKnownMemberInvoker.cs
- SplashScreenNativeMethods.cs
- EventLogInformation.cs
- AutomationProperty.cs
- UnauthorizedAccessException.cs
- ObjectDataSourceEventArgs.cs
- PixelShader.cs
- Bits.cs
- RectangleF.cs
- ObjectToken.cs
- IFlowDocumentViewer.cs
- LinqDataSource.cs
- CodeObjectCreateExpression.cs
- FullTextLine.cs
- ActiveXHelper.cs
- ClientConvert.cs
- SkipStoryboardToFill.cs
- FontWeights.cs
- UrlMappingCollection.cs
- UntrustedRecipientException.cs
- SystemColors.cs
- EntryWrittenEventArgs.cs
- TextBoxAutomationPeer.cs
- Cursor.cs
- InkCollectionBehavior.cs
- MasterPage.cs
- ColumnBinding.cs
- sitestring.cs
- ToolboxItemCollection.cs
- SubMenuStyle.cs
- BufferedGraphicsManager.cs
- XmlStreamNodeWriter.cs
- AtomicFile.cs
- XmlUtil.cs
- URIFormatException.cs
- QilFunction.cs
- UInt64Converter.cs
- Opcode.cs
- TypeDescriptor.cs
- SiteMapPath.cs
- PropertyInfoSet.cs
- TreeNodeCollection.cs
- ListSortDescriptionCollection.cs
- InkCanvasSelectionAdorner.cs
- TemplatedEditableDesignerRegion.cs
- KeyPressEvent.cs
- BaseDataList.cs
- BinaryObjectInfo.cs
- CacheManager.cs
- ConfigurationManager.cs
- SpotLight.cs