Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / MS / Internal / Controls / ActiveXHelper.cs / 1 / ActiveXHelper.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // // // // Description: // Helper functions for ActiveX hosting // // Source copied from axhosthelper.cs // // // History // 04/17/05 [....] Created // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Specialized; using System.Runtime.InteropServices; using System.Security; using MS.Win32; namespace MS.Internal.Controls { // // This class contains static properties/methods that are internal. // It also has types that make sense only for ActiveX hosting classes. // In other words, this is a helper class for the ActiveX hosting classes. // internal class ActiveXHelper { // // Types: // // // Enumeration of the different states of the ActiveX control public enum ActiveXState { Passive = 0, // Not loaded Loaded = 1, // Loaded, but no server [ocx created] Running = 2, // Server running, invisible [depersisted] InPlaceActive = 4, // Server in-place active [visible] UIActive = 8, // Server is UI active [ready to accept input] Open = 16 // Server is opened for editing [not used] } // // Static members: // // // BitVector32 masks for various internal state flags. public static readonly int sinkAttached = BitVector32.CreateMask(); public static readonly int inTransition = BitVector32.CreateMask(sinkAttached); public static readonly int processingKeyUp = BitVector32.CreateMask(inTransition); //[[....]]These are from Winforms code. These transforms were not working correctly //I used Avalon sizes but leaving these in here since they are used in TransformCoordinates //in ActiveXSite. It doesn't seem to be invoked by WebBrowser control // // Gets the LOGPIXELSX of the screen DC. private static int logPixelsX = -1; private static int logPixelsY = -1; private const int HMperInch = 2540; // Prevent compiler from generating public CTOR private ActiveXHelper() { } // // Static helper methods: // public static int Pix2HM(int pix, int logP) { return (HMperInch * pix + (logP >> 1)) / logP; } public static int HM2Pix(int hm, int logP) { return (logP * hm + HMperInch / 2) / HMperInch; } // // We cache LOGPIXELSX for optimization ////// Critical: This code calls critical code. /// TreatAsSafe: This information is safe to expose. /// public static int LogPixelsX { [SecurityCritical, SecurityTreatAsSafe] get { if (logPixelsX == -1) { IntPtr hDC = UnsafeNativeMethods.GetDC(NativeMethods.NullHandleRef); if (hDC != IntPtr.Zero) { logPixelsX = UnsafeNativeMethods.GetDeviceCaps(new HandleRef(null, hDC), NativeMethods.LOGPIXELSX); UnsafeNativeMethods.ReleaseDC(NativeMethods.NullHandleRef, new HandleRef(null, hDC)); } } return logPixelsX; } } public static void ResetLogPixelsX() { logPixelsX = -1; } // // We cache LOGPIXELSY for optimization ////// Critical: This code calls critical code. /// TreatAsSafe: This information is safe to expose. /// public static int LogPixelsY { [SecurityCritical, SecurityTreatAsSafe] get { if (logPixelsY == -1) { IntPtr hDC = UnsafeNativeMethods.GetDC(NativeMethods.NullHandleRef); if (hDC != IntPtr.Zero) { logPixelsY = UnsafeNativeMethods.GetDeviceCaps(new HandleRef(null, hDC), NativeMethods.LOGPIXELSY); UnsafeNativeMethods.ReleaseDC(NativeMethods.NullHandleRef, new HandleRef(null, hDC)); } } return logPixelsY; } } public static void ResetLogPixelsY() { logPixelsY = -1; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
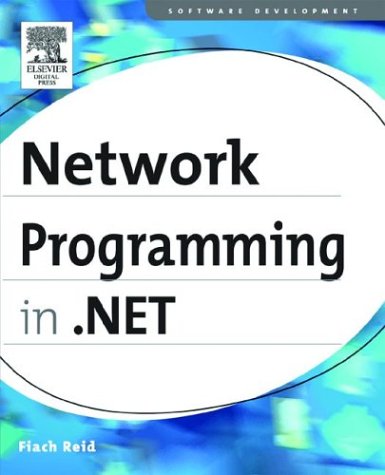
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlLanguageConverter.cs
- ToolboxDataAttribute.cs
- TextElement.cs
- String.cs
- BufferedGraphics.cs
- Vertex.cs
- PixelFormat.cs
- DataColumnCollection.cs
- UseAttributeSetsAction.cs
- XslTransform.cs
- AutoResetEvent.cs
- CngKeyCreationParameters.cs
- GAC.cs
- NumberFormatter.cs
- DataGridViewImageColumn.cs
- XmlEntityReference.cs
- ReaderWriterLockSlim.cs
- DataShape.cs
- Composition.cs
- LinqDataSourceDeleteEventArgs.cs
- StickyNote.cs
- AssemblyCollection.cs
- DiagnosticTrace.cs
- FormViewPagerRow.cs
- ValueHandle.cs
- RandomNumberGenerator.cs
- NoneExcludedImageIndexConverter.cs
- FileDetails.cs
- SqlDataSourceCustomCommandPanel.cs
- documentation.cs
- DSACryptoServiceProvider.cs
- SerialReceived.cs
- TabControl.cs
- BindingExpressionBase.cs
- ListSourceHelper.cs
- FilterQuery.cs
- NotifyInputEventArgs.cs
- ProxyWebPartManager.cs
- CategoryGridEntry.cs
- EntityDataReader.cs
- TypeDescriptionProvider.cs
- XmlDeclaration.cs
- ProfileInfo.cs
- OdbcException.cs
- LogicalCallContext.cs
- EraserBehavior.cs
- Vector3DCollectionConverter.cs
- Deflater.cs
- WebPartAddingEventArgs.cs
- BitmapEffectInputData.cs
- UnsafeNativeMethods.cs
- ZipIORawDataFileBlock.cs
- RegexBoyerMoore.cs
- Validator.cs
- Soap12ProtocolImporter.cs
- AnnotationStore.cs
- SQLCharsStorage.cs
- hwndwrapper.cs
- DoubleAnimationUsingPath.cs
- CommunicationException.cs
- TextEditorTyping.cs
- PropertyRecord.cs
- HttpPostLocalhostServerProtocol.cs
- COAUTHIDENTITY.cs
- TextReader.cs
- TaiwanLunisolarCalendar.cs
- Package.cs
- ParallelDesigner.xaml.cs
- AppDomainProtocolHandler.cs
- HttpApplicationStateBase.cs
- XmlTextReader.cs
- SrgsDocumentParser.cs
- CssClassPropertyAttribute.cs
- COM2TypeInfoProcessor.cs
- DataControlImageButton.cs
- SvcMapFileSerializer.cs
- StagingAreaInputItem.cs
- ButtonFieldBase.cs
- CodeTypeConstructor.cs
- GlobalAllocSafeHandle.cs
- SupportingTokenParameters.cs
- CqlGenerator.cs
- CapabilitiesSection.cs
- ClrPerspective.cs
- TextStore.cs
- ToolStripContentPanel.cs
- LambdaCompiler.Address.cs
- securitycriticaldataClass.cs
- DataGridViewRowErrorTextNeededEventArgs.cs
- ActiveXHost.cs
- InstrumentationTracker.cs
- OwnerDrawPropertyBag.cs
- EntityConnectionStringBuilderItem.cs
- ColorDialog.cs
- InvalidChannelBindingException.cs
- PenThreadWorker.cs
- ThemeDictionaryExtension.cs
- SamlSerializer.cs
- RolePrincipal.cs
- FileLevelControlBuilderAttribute.cs