Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Diagnostics / Eventing / Reader / EventLogInformation.cs / 1305376 / EventLogInformation.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: EventLogInformation ** ** Purpose: ** The objects of this class allow access to the run-time ** properties of logs and external log files. An instance of this ** class is obtained from EventLogSession. ** ============================================================*/ using System; using System.Runtime.InteropServices; using Microsoft.Win32; namespace System.Diagnostics.Eventing.Reader { ////// Describes the run-time properties of logs and external log files. An instance /// of this class is obtained from EventLogSession. /// [System.Security.Permissions.HostProtection(MayLeakOnAbort = true)] public sealed class EventLogInformation { DateTime? creationTime; DateTime? lastAccessTime; DateTime? lastWriteTime; long? fileSize; int? fileAttributes; long? recordCount; long? oldestRecordNumber; bool? isLogFull; [System.Security.SecuritySafeCritical] internal EventLogInformation(EventLogSession session, string channelName, PathType pathType) { EventLogPermissionHolder.GetEventLogPermission().Demand(); EventLogHandle logHandle = NativeWrapper.EvtOpenLog(session.Handle, channelName, pathType); using (logHandle) { creationTime = (DateTime?)NativeWrapper.EvtGetLogInfo(logHandle, UnsafeNativeMethods.EvtLogPropertyId.EvtLogCreationTime); lastAccessTime = (DateTime?)NativeWrapper.EvtGetLogInfo(logHandle, UnsafeNativeMethods.EvtLogPropertyId.EvtLogLastAccessTime); lastWriteTime = (DateTime?)NativeWrapper.EvtGetLogInfo(logHandle, UnsafeNativeMethods.EvtLogPropertyId.EvtLogLastWriteTime); fileSize = (long?)((ulong?)NativeWrapper.EvtGetLogInfo(logHandle, UnsafeNativeMethods.EvtLogPropertyId.EvtLogFileSize)); fileAttributes = (int?)((uint?)NativeWrapper.EvtGetLogInfo(logHandle, UnsafeNativeMethods.EvtLogPropertyId.EvtLogAttributes)); recordCount = (long?)((ulong?)NativeWrapper.EvtGetLogInfo(logHandle, UnsafeNativeMethods.EvtLogPropertyId.EvtLogNumberOfLogRecords)); oldestRecordNumber = (long?)((ulong?)NativeWrapper.EvtGetLogInfo(logHandle, UnsafeNativeMethods.EvtLogPropertyId.EvtLogOldestRecordNumber)); isLogFull = (bool?)NativeWrapper.EvtGetLogInfo(logHandle, UnsafeNativeMethods.EvtLogPropertyId.EvtLogFull); } } public DateTime? CreationTime { get { return creationTime; } } public DateTime? LastAccessTime { get { return lastAccessTime; } } public DateTime? LastWriteTime { get { return lastWriteTime; } } public long? FileSize { get { return fileSize; } } public int? Attributes { get { return fileAttributes; } } public long? RecordCount { get { return recordCount; } } public long? OldestRecordNumber { get { return oldestRecordNumber; } } public bool? IsLogFull { get { return isLogFull; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
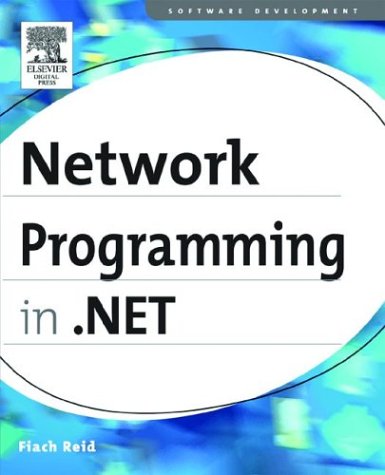
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MenuCommands.cs
- CroppedBitmap.cs
- ArrayExtension.cs
- TogglePattern.cs
- ListViewHitTestInfo.cs
- DBProviderConfigurationHandler.cs
- TableLayoutSettingsTypeConverter.cs
- PtsPage.cs
- ExceptionUtil.cs
- PolyLineSegment.cs
- IndexOutOfRangeException.cs
- TableCell.cs
- Dictionary.cs
- Ref.cs
- XmlSchemaComplexContentExtension.cs
- InfoCardRSAPKCS1KeyExchangeFormatter.cs
- CommunicationObjectManager.cs
- TextPointerBase.cs
- IteratorFilter.cs
- AuthenticatingEventArgs.cs
- SiteOfOriginContainer.cs
- WindowsPrincipal.cs
- ObjectNotFoundException.cs
- KeyConstraint.cs
- TraceUtility.cs
- AccessDataSourceView.cs
- RenderData.cs
- IndexedGlyphRun.cs
- ReferencedType.cs
- ExpressionHelper.cs
- WindowsGraphicsWrapper.cs
- DomNameTable.cs
- clipboard.cs
- Line.cs
- DeflateStream.cs
- CallbackDebugBehavior.cs
- HttpResponse.cs
- StylusEditingBehavior.cs
- CopyCodeAction.cs
- NetStream.cs
- CallInfo.cs
- RuntimeHelpers.cs
- OperatingSystem.cs
- GroupQuery.cs
- CssClassPropertyAttribute.cs
- ReadOnlyHierarchicalDataSource.cs
- RotateTransform3D.cs
- ChannelServices.cs
- Pen.cs
- SerializerDescriptor.cs
- HttpCapabilitiesSectionHandler.cs
- GridEntryCollection.cs
- ProgressChangedEventArgs.cs
- Stack.cs
- ControlParameter.cs
- InputGestureCollection.cs
- FileSecurity.cs
- PrintDialogException.cs
- StringValidatorAttribute.cs
- XmlSchemaValidator.cs
- BooleanExpr.cs
- DataGridDetailsPresenter.cs
- SigningCredentials.cs
- _SslState.cs
- TypeDelegator.cs
- SqlCachedBuffer.cs
- XmlElementCollection.cs
- Misc.cs
- ExtensionQuery.cs
- ComponentGlyph.cs
- QilList.cs
- InputLanguageManager.cs
- WaitHandleCannotBeOpenedException.cs
- relpropertyhelper.cs
- FileDialogCustomPlace.cs
- SQLSingle.cs
- TableRowGroup.cs
- Cursors.cs
- RC2.cs
- XPathNode.cs
- ProcessHostMapPath.cs
- Enum.cs
- RelationalExpressions.cs
- CodeNamespace.cs
- _CommandStream.cs
- TransformerConfigurationWizardBase.cs
- OperationParameterInfo.cs
- DispatcherExceptionEventArgs.cs
- DodSequenceMerge.cs
- TypefaceCollection.cs
- Int16Animation.cs
- ApplicationTrust.cs
- EmptyStringExpandableObjectConverter.cs
- BitmapPalette.cs
- IdleTimeoutMonitor.cs
- SqlDeflator.cs
- smtppermission.cs
- ToolStripSystemRenderer.cs
- SchemaImporter.cs
- ControlAdapter.cs