Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WCF / infocard / common / managed / HGlobalSafeHandle.cs / 1305376 / HGlobalSafeHandle.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.Runtime.InteropServices; using Microsoft.InfoCards.Diagnostics; using System.Runtime.CompilerServices; using System.Runtime.ConstrainedExecution; using System.Security; using IDT=Microsoft.InfoCards.Diagnostics.InfoCardTrace; // // Summary: // Provides a wrapper over HGlobal alloc'd memory guaranteeing that the // contents will be released in the presence of rude app domain and thread aborts. // internal class HGlobalSafeHandle : SafeHandle { public static HGlobalSafeHandle Construct() { return new HGlobalSafeHandle(); } public static HGlobalSafeHandle Construct( string managedString ) { IDT.DebugAssert( !String.IsNullOrEmpty( managedString) , "null string" ); int bytes = (managedString.Length + 1) * 2; return new HGlobalSafeHandle( Marshal.StringToHGlobalUni( managedString ), bytes ); } public static HGlobalSafeHandle Construct( int bytes ) { IDT.DebugAssert( bytes > 0, "attempt to allocate a handle with <= 0 bytes" ); return new HGlobalSafeHandle( Marshal.AllocHGlobal( bytes ), bytes ); } [SuppressUnmanagedCodeSecurity] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [DllImport("Kernel32.dll", EntryPoint="RtlZeroMemory", SetLastError=false)] public static extern void ZeroMemory( IntPtr dest, Int32 size ); // // The number of bytes allocated. // private int m_bytes; [ReliabilityContract( Consistency.WillNotCorruptState, Cer.Success ) ] private HGlobalSafeHandle( IntPtr toManage, int length ) : base( IntPtr.Zero, true ) { m_bytes = length; SetHandle( toManage ); } private HGlobalSafeHandle() : base( IntPtr.Zero, true ) {} public override bool IsInvalid { get { return ( IntPtr.Zero == base.handle ); } } // // Summary: // Zero the string contents and release the handle // protected override bool ReleaseHandle() { IDT.DebugAssert( !IsInvalid, "handle is invalid in release handle" ); IDT.DebugAssert( 0 != m_bytes, "invalid size" ); ZeroMemory( base.handle, m_bytes ); Marshal.FreeHGlobal( base.handle ); return true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.Runtime.InteropServices; using Microsoft.InfoCards.Diagnostics; using System.Runtime.CompilerServices; using System.Runtime.ConstrainedExecution; using System.Security; using IDT=Microsoft.InfoCards.Diagnostics.InfoCardTrace; // // Summary: // Provides a wrapper over HGlobal alloc'd memory guaranteeing that the // contents will be released in the presence of rude app domain and thread aborts. // internal class HGlobalSafeHandle : SafeHandle { public static HGlobalSafeHandle Construct() { return new HGlobalSafeHandle(); } public static HGlobalSafeHandle Construct( string managedString ) { IDT.DebugAssert( !String.IsNullOrEmpty( managedString) , "null string" ); int bytes = (managedString.Length + 1) * 2; return new HGlobalSafeHandle( Marshal.StringToHGlobalUni( managedString ), bytes ); } public static HGlobalSafeHandle Construct( int bytes ) { IDT.DebugAssert( bytes > 0, "attempt to allocate a handle with <= 0 bytes" ); return new HGlobalSafeHandle( Marshal.AllocHGlobal( bytes ), bytes ); } [SuppressUnmanagedCodeSecurity] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [DllImport("Kernel32.dll", EntryPoint="RtlZeroMemory", SetLastError=false)] public static extern void ZeroMemory( IntPtr dest, Int32 size ); // // The number of bytes allocated. // private int m_bytes; [ReliabilityContract( Consistency.WillNotCorruptState, Cer.Success ) ] private HGlobalSafeHandle( IntPtr toManage, int length ) : base( IntPtr.Zero, true ) { m_bytes = length; SetHandle( toManage ); } private HGlobalSafeHandle() : base( IntPtr.Zero, true ) {} public override bool IsInvalid { get { return ( IntPtr.Zero == base.handle ); } } // // Summary: // Zero the string contents and release the handle // protected override bool ReleaseHandle() { IDT.DebugAssert( !IsInvalid, "handle is invalid in release handle" ); IDT.DebugAssert( 0 != m_bytes, "invalid size" ); ZeroMemory( base.handle, m_bytes ); Marshal.FreeHGlobal( base.handle ); return true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
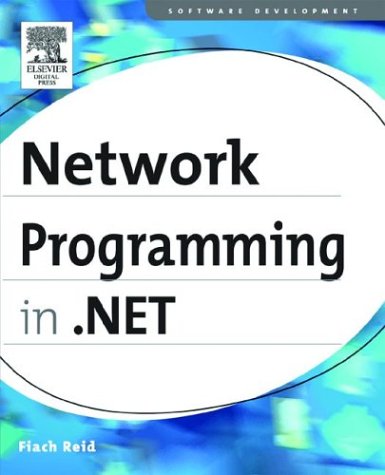
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CheckBox.cs
- BitmapMetadataBlob.cs
- DockingAttribute.cs
- AttributeCollection.cs
- MetadataItem_Static.cs
- CollectionEditVerbManager.cs
- LogLogRecord.cs
- RowUpdatedEventArgs.cs
- ApplicationDirectory.cs
- ConnectionStringsExpressionEditor.cs
- DirectoryInfo.cs
- SecurityDocument.cs
- WsatServiceCertificate.cs
- DataGridViewCellPaintingEventArgs.cs
- XmlSchemas.cs
- LinqDataSourceInsertEventArgs.cs
- PropertyInfoSet.cs
- ChangeTracker.cs
- GeometryDrawing.cs
- AsmxEndpointPickerExtension.cs
- SqlNotificationEventArgs.cs
- AssemblyName.cs
- SecurityManager.cs
- ViewPort3D.cs
- XmlAnyAttributeAttribute.cs
- FaultCode.cs
- CodeTypeReferenceExpression.cs
- CommentGlyph.cs
- WeakEventManager.cs
- ComponentResourceKeyConverter.cs
- FontResourceCache.cs
- IPEndPoint.cs
- SqlUDTStorage.cs
- SqlTypeSystemProvider.cs
- CollectionView.cs
- Image.cs
- System.Data_BID.cs
- DocumentationServerProtocol.cs
- FileLoadException.cs
- HttpCapabilitiesSectionHandler.cs
- WebServiceParameterData.cs
- QuaternionAnimation.cs
- SuppressIldasmAttribute.cs
- PerformanceCountersElement.cs
- AssertFilter.cs
- Parser.cs
- Knowncolors.cs
- RMPublishingDialog.cs
- PolyBezierSegment.cs
- DependencySource.cs
- NotifyIcon.cs
- MasterPageParser.cs
- HuffCodec.cs
- CodeParameterDeclarationExpression.cs
- ScriptResourceHandler.cs
- CodeArrayIndexerExpression.cs
- TextHidden.cs
- JoinElimination.cs
- FixedSOMPage.cs
- ManagedFilter.cs
- StrokeNodeData.cs
- PageParser.cs
- TemplateAction.cs
- DispatchRuntime.cs
- DebugView.cs
- EventLogPermissionEntry.cs
- PriorityRange.cs
- SqlConnectionPoolProviderInfo.cs
- Statements.cs
- PropertyConverter.cs
- DynamicResourceExtensionConverter.cs
- Quad.cs
- GridPattern.cs
- MetadataItem_Static.cs
- ZoneButton.cs
- XamlFilter.cs
- GuidelineCollection.cs
- SqlDataSourceFilteringEventArgs.cs
- BlurBitmapEffect.cs
- SafeFileMapViewHandle.cs
- selecteditemcollection.cs
- Publisher.cs
- VirtualPath.cs
- QueryStringConverter.cs
- XmlFormatExtensionAttribute.cs
- DatatypeImplementation.cs
- ManagementClass.cs
- InvalidAsynchronousStateException.cs
- WebPartDisplayModeCollection.cs
- cookie.cs
- WindowsGraphicsCacheManager.cs
- Style.cs
- ClientBuildManagerCallback.cs
- Icon.cs
- ToggleProviderWrapper.cs
- LineUtil.cs
- DesignTimeTemplateParser.cs
- ExpressionPrefixAttribute.cs
- OuterGlowBitmapEffect.cs
- WindowsSolidBrush.cs