Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataEntity / System / Data / Query / InternalTrees / ExplicitDiscriminatorMap.cs / 1 / ExplicitDiscriminatorMap.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Metadata.Edm; using System.Collections.Generic; using System.Linq; namespace System.Data.Query.InternalTrees { ////// Describes user-defined discriminator metadata (e.g. for a basic TPH mapping). Encapsulates /// relevant data from System.Data.Mapping.ViewGenerabetion.DiscriminatorMap (that is to say, /// data relevant to the PlanCompiler). This separate class accomplishes two things: /// /// 1. Maintain separation of ViewGen and PlanCompiler /// 2. Avoid holding references to CQT expressions in ITree ops (which the ViewGen.DiscriminatorMap /// holds a few CQT references) /// internal class ExplicitDiscriminatorMap { private readonly System.Collections.ObjectModel.ReadOnlyCollection> m_typeMap; private readonly EdmMember m_discriminatorProperty; private readonly System.Collections.ObjectModel.ReadOnlyCollection m_properties; internal ExplicitDiscriminatorMap(System.Data.Mapping.ViewGeneration.DiscriminatorMap template) { m_typeMap = template.TypeMap; m_discriminatorProperty = template.Discriminator.Property; m_properties = template.PropertyMap.Select(propertyValuePair => propertyValuePair.Key) .ToList().AsReadOnly(); } /// /// Maps from discriminator value to type. /// internal System.Collections.ObjectModel.ReadOnlyCollection> TypeMap { get { return m_typeMap; } } /// /// Gets property containing discriminator value. /// internal EdmMember DiscriminatorProperty { get { return m_discriminatorProperty; } } ////// All properties for the type hierarchy. /// internal System.Collections.ObjectModel.ReadOnlyCollectionProperties { get { return m_properties; } } /// /// Returns the type id for the given entity type, or null if non exists. /// internal object GetTypeId(EntityType entityType) { object result = null; foreach (var discriminatorTypePair in this.TypeMap) { if (discriminatorTypePair.Value.EdmEquals(entityType)) { result = discriminatorTypePair.Key; break; } } return result; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Metadata.Edm; using System.Collections.Generic; using System.Linq; namespace System.Data.Query.InternalTrees { ////// Describes user-defined discriminator metadata (e.g. for a basic TPH mapping). Encapsulates /// relevant data from System.Data.Mapping.ViewGenerabetion.DiscriminatorMap (that is to say, /// data relevant to the PlanCompiler). This separate class accomplishes two things: /// /// 1. Maintain separation of ViewGen and PlanCompiler /// 2. Avoid holding references to CQT expressions in ITree ops (which the ViewGen.DiscriminatorMap /// holds a few CQT references) /// internal class ExplicitDiscriminatorMap { private readonly System.Collections.ObjectModel.ReadOnlyCollection> m_typeMap; private readonly EdmMember m_discriminatorProperty; private readonly System.Collections.ObjectModel.ReadOnlyCollection m_properties; internal ExplicitDiscriminatorMap(System.Data.Mapping.ViewGeneration.DiscriminatorMap template) { m_typeMap = template.TypeMap; m_discriminatorProperty = template.Discriminator.Property; m_properties = template.PropertyMap.Select(propertyValuePair => propertyValuePair.Key) .ToList().AsReadOnly(); } /// /// Maps from discriminator value to type. /// internal System.Collections.ObjectModel.ReadOnlyCollection> TypeMap { get { return m_typeMap; } } /// /// Gets property containing discriminator value. /// internal EdmMember DiscriminatorProperty { get { return m_discriminatorProperty; } } ////// All properties for the type hierarchy. /// internal System.Collections.ObjectModel.ReadOnlyCollectionProperties { get { return m_properties; } } /// /// Returns the type id for the given entity type, or null if non exists. /// internal object GetTypeId(EntityType entityType) { object result = null; foreach (var discriminatorTypePair in this.TypeMap) { if (discriminatorTypePair.Value.EdmEquals(entityType)) { result = discriminatorTypePair.Key; break; } } return result; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
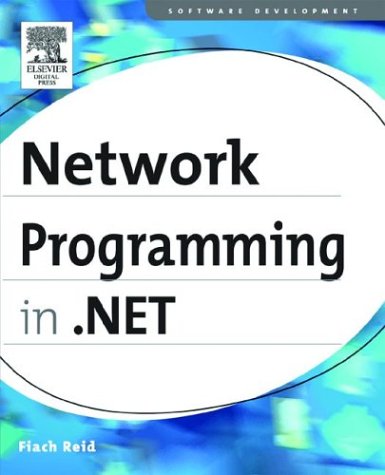
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ExceptionDetail.cs
- BamlRecordReader.cs
- Handle.cs
- OneOf.cs
- ClonableStack.cs
- TemplateNameScope.cs
- DataMisalignedException.cs
- WebPageTraceListener.cs
- HtmlElementCollection.cs
- FtpCachePolicyElement.cs
- DBConnection.cs
- SystemColorTracker.cs
- EventLogPermission.cs
- SR.cs
- MetadataPropertyCollection.cs
- ValueSerializerAttribute.cs
- SendingRequestEventArgs.cs
- ElementNotEnabledException.cs
- NativeCppClassAttribute.cs
- GeometryCombineModeValidation.cs
- UnsupportedPolicyOptionsException.cs
- LineServicesRun.cs
- FontStyles.cs
- HtmlInputHidden.cs
- ReferentialConstraint.cs
- CheckBoxAutomationPeer.cs
- ManagementQuery.cs
- DataRelationCollection.cs
- ConstraintConverter.cs
- AssemblyInfo.cs
- SharedConnectionInfo.cs
- MultilineStringConverter.cs
- Stackframe.cs
- MailDefinition.cs
- WebSysDefaultValueAttribute.cs
- WebPartHelpVerb.cs
- RadioButton.cs
- SmiConnection.cs
- CancelEventArgs.cs
- RemotingSurrogateSelector.cs
- ParseChildrenAsPropertiesAttribute.cs
- TreeView.cs
- XmlAutoDetectWriter.cs
- KnownBoxes.cs
- PropertyChangedEventManager.cs
- ToolStripContentPanelDesigner.cs
- ComponentDispatcher.cs
- NGCSerializer.cs
- Tag.cs
- SocketStream.cs
- PenCursorManager.cs
- SmtpLoginAuthenticationModule.cs
- MexTcpBindingCollectionElement.cs
- SqlDataSourceStatusEventArgs.cs
- ImageButton.cs
- SerializerProvider.cs
- ExplicitDiscriminatorMap.cs
- FlowPosition.cs
- NopReturnReader.cs
- Rect.cs
- DataGridBeginningEditEventArgs.cs
- IntellisenseTextBox.cs
- IArgumentProvider.cs
- XmlStringTable.cs
- RectangleGeometry.cs
- RewritingPass.cs
- ExpressionParser.cs
- RTLAwareMessageBox.cs
- Variable.cs
- MultiPageTextView.cs
- ServiceModelStringsVersion1.cs
- CssClassPropertyAttribute.cs
- PhysicalOps.cs
- Geometry3D.cs
- InstanceDescriptor.cs
- MenuItemBindingCollection.cs
- IndexingContentUnit.cs
- InternalSafeNativeMethods.cs
- BinHexEncoder.cs
- SqlConnectionFactory.cs
- CollectionTraceRecord.cs
- WebConfigurationFileMap.cs
- ResolveNextArgumentWorkItem.cs
- CircleHotSpot.cs
- XmlTextReaderImpl.cs
- BidOverLoads.cs
- WindowsRichEdit.cs
- ManagedIStream.cs
- OleDbConnectionInternal.cs
- ComponentSerializationService.cs
- IisTraceListener.cs
- BinaryMethodMessage.cs
- PenContexts.cs
- NopReturnReader.cs
- Geometry3D.cs
- SqlConnectionManager.cs
- ContentType.cs
- ExtenderControl.cs
- CapabilitiesSection.cs
- ScriptMethodAttribute.cs