Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WF / RunTime / Hosting / SharedConnectionInfo.cs / 1305376 / SharedConnectionInfo.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- #region Using directives using System; using System.Diagnostics; using System.Transactions; using System.Data.Common; using System.Threading; #endregion namespace System.Workflow.Runtime.Hosting { ////// This class keeps the following associated with a Transaction /// - a connection that participates in the transaction. /// - an optional local transaction (DbTransaction) generated from the single-phase-committed Transaction. /// The connection and the local transaction are passed around to different host components to /// do transacted DB work using the shared connection. /// internal sealed class SharedConnectionInfo : IDisposable { readonly DbConnection connection; readonly DbTransaction localTransaction; private bool disposed; private ManualResetEvent handle; #region Constructor ////// Instantiate an opened connection enlisted to the Transaction /// if promotable is false, the Transaction wraps a local /// transaction inside and can never be promoted /// /// /// /// internal SharedConnectionInfo( DbResourceAllocator dbResourceAllocator, Transaction transaction, bool wantPromotable, ManualResetEvent handle) { Debug.Assert((transaction != null), "Null Transaction!"); if (null == handle) throw new ArgumentNullException("handle"); this.handle = handle; if (wantPromotable) { // Enlist a newly opened connection to this regular Transaction this.connection = dbResourceAllocator.OpenNewConnection(); this.connection.EnlistTransaction(transaction); } else { // Make this transaction no longer promotable by attaching our // IPromotableSinglePhaseNotification implementation (LocalTranscaction) // and track the DbConnection and DbTransaction associated with the LocalTranscaction LocalTransaction localTransaction = new LocalTransaction(dbResourceAllocator,handle); transaction.EnlistPromotableSinglePhase(localTransaction); this.connection = localTransaction.Connection; this.localTransaction = localTransaction.Transaction; } } #endregion Constructor #region Accessors internal DbConnection DBConnection { get { return this.connection; } } internal DbTransaction DBTransaction { get { return this.localTransaction; } } #endregion Accessors #region IDisposable Members public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } private void Dispose(bool disposing) { if (!this.disposed) { // // If we're using a LocalTransaction it will close the connection // in it's IPromotableSinglePhaseNotification methods if ((this.localTransaction == null)&&(null != connection)) this.connection.Dispose(); } this.disposed = true; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- #region Using directives using System; using System.Diagnostics; using System.Transactions; using System.Data.Common; using System.Threading; #endregion namespace System.Workflow.Runtime.Hosting { ////// This class keeps the following associated with a Transaction /// - a connection that participates in the transaction. /// - an optional local transaction (DbTransaction) generated from the single-phase-committed Transaction. /// The connection and the local transaction are passed around to different host components to /// do transacted DB work using the shared connection. /// internal sealed class SharedConnectionInfo : IDisposable { readonly DbConnection connection; readonly DbTransaction localTransaction; private bool disposed; private ManualResetEvent handle; #region Constructor ////// Instantiate an opened connection enlisted to the Transaction /// if promotable is false, the Transaction wraps a local /// transaction inside and can never be promoted /// /// /// /// internal SharedConnectionInfo( DbResourceAllocator dbResourceAllocator, Transaction transaction, bool wantPromotable, ManualResetEvent handle) { Debug.Assert((transaction != null), "Null Transaction!"); if (null == handle) throw new ArgumentNullException("handle"); this.handle = handle; if (wantPromotable) { // Enlist a newly opened connection to this regular Transaction this.connection = dbResourceAllocator.OpenNewConnection(); this.connection.EnlistTransaction(transaction); } else { // Make this transaction no longer promotable by attaching our // IPromotableSinglePhaseNotification implementation (LocalTranscaction) // and track the DbConnection and DbTransaction associated with the LocalTranscaction LocalTransaction localTransaction = new LocalTransaction(dbResourceAllocator,handle); transaction.EnlistPromotableSinglePhase(localTransaction); this.connection = localTransaction.Connection; this.localTransaction = localTransaction.Transaction; } } #endregion Constructor #region Accessors internal DbConnection DBConnection { get { return this.connection; } } internal DbTransaction DBTransaction { get { return this.localTransaction; } } #endregion Accessors #region IDisposable Members public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } private void Dispose(bool disposing) { if (!this.disposed) { // // If we're using a LocalTransaction it will close the connection // in it's IPromotableSinglePhaseNotification methods if ((this.localTransaction == null)&&(null != connection)) this.connection.Dispose(); } this.disposed = true; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
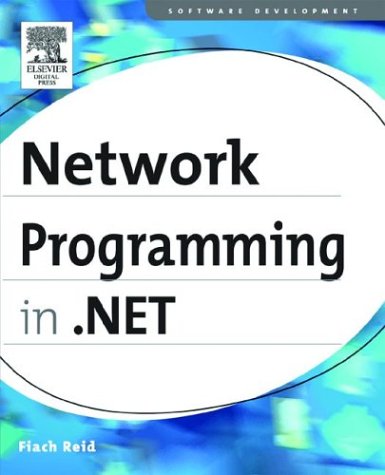
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EntityDataSourceConfigureObjectContext.cs
- LoggedException.cs
- ResolveInfo.cs
- sqlstateclientmanager.cs
- OrthographicCamera.cs
- DataGridViewAccessibleObject.cs
- XmlSerializerAssemblyAttribute.cs
- ConstraintEnumerator.cs
- NotSupportedException.cs
- AspNetHostingPermission.cs
- MonthCalendar.cs
- Point3D.cs
- CachingParameterInspector.cs
- IgnoreSection.cs
- HyperLinkStyle.cs
- PersonalizationAdministration.cs
- UndoManager.cs
- WebContext.cs
- CurrentChangingEventArgs.cs
- Stack.cs
- WebReferencesBuildProvider.cs
- HeaderUtility.cs
- WebSysDisplayNameAttribute.cs
- VirtualizingPanel.cs
- securitymgrsite.cs
- ConstNode.cs
- PrinterUnitConvert.cs
- Rules.cs
- DirectoryObjectSecurity.cs
- SqlExpander.cs
- PropertyKey.cs
- SchemaUtility.cs
- FormViewUpdateEventArgs.cs
- DayRenderEvent.cs
- streamingZipPartStream.cs
- UpdateException.cs
- XmlFormatReaderGenerator.cs
- WriteableBitmap.cs
- ScrollViewerAutomationPeer.cs
- SqlFunctionAttribute.cs
- TimeoutValidationAttribute.cs
- StylusPlugInCollection.cs
- SelectionItemPattern.cs
- CellPartitioner.cs
- PersonalizationProvider.cs
- RowUpdatingEventArgs.cs
- BrowserDefinition.cs
- ConfigsHelper.cs
- WebPartEditorApplyVerb.cs
- PinnedBufferMemoryStream.cs
- ProfileSettings.cs
- LabelLiteral.cs
- FontNamesConverter.cs
- FormViewDesigner.cs
- ExternalFile.cs
- Message.cs
- ReceiveParametersContent.cs
- SqlTypeConverter.cs
- ButtonField.cs
- ResourcePermissionBaseEntry.cs
- DynamicDocumentPaginator.cs
- InstanceData.cs
- OperationParameterInfoCollection.cs
- LayoutExceptionEventArgs.cs
- ISAPIApplicationHost.cs
- storagemappingitemcollection.viewdictionary.cs
- JournalNavigationScope.cs
- CodeGeneratorAttribute.cs
- CallContext.cs
- SymDocumentType.cs
- Update.cs
- DomainUpDown.cs
- ServiceReference.cs
- AspCompat.cs
- ProcessHostConfigUtils.cs
- PreviewKeyDownEventArgs.cs
- FlowSwitch.cs
- DynamicResourceExtension.cs
- DbProviderConfigurationHandler.cs
- ViewManager.cs
- CompilerError.cs
- TextPointer.cs
- FrameworkEventSource.cs
- DataTemplateKey.cs
- ZoneButton.cs
- PersonalizationEntry.cs
- remotingproxy.cs
- TypePropertyEditor.cs
- DBProviderConfigurationHandler.cs
- CustomGrammar.cs
- SoapClientProtocol.cs
- IChannel.cs
- QueryFunctions.cs
- DataBindingCollectionConverter.cs
- ForceCopyBuildProvider.cs
- GiveFeedbackEvent.cs
- SQlBooleanStorage.cs
- DataGridViewRowCancelEventArgs.cs
- BaseAddressPrefixFilterElementCollection.cs
- XMLSyntaxException.cs