Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Xml / System / Xml / XPath / Internal / IDQuery.cs / 1 / IDQuery.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace MS.Internal.Xml.XPath { using System; using System.Xml; using System.Xml.XPath; using System.Xml.Xsl; using System.Diagnostics; using System.Collections.Generic; internal sealed class IDQuery : CacheOutputQuery { public IDQuery(Query arg) : base(arg) {} private IDQuery(IDQuery other) : base(other) { } public override object Evaluate(XPathNodeIterator context) { object argVal = base.Evaluate(context); XPathNavigator contextNode = context.Current.Clone(); switch (GetXPathType(argVal)) { case XPathResultType.NodeSet: XPathNavigator temp; while ((temp = input.Advance()) != null) { ProcessIds(contextNode, temp.Value); } break; case XPathResultType.String: ProcessIds(contextNode, (string)argVal); break; case XPathResultType.Number: ProcessIds(contextNode, StringFunctions.toString((double)argVal)); break; case XPathResultType.Boolean: ProcessIds(contextNode, StringFunctions.toString((bool)argVal)); break; case XPathResultType_Navigator: ProcessIds(contextNode, ((XPathNavigator)argVal).Value); break; } return this; } void ProcessIds(XPathNavigator contextNode, string val) { string[] ids = XmlConvert.SplitString(val); for (int idx = 0; idx < ids.Length; idx++) { if (contextNode.MoveToId(ids[idx])) { Insert(outputBuffer, contextNode); } } } public override XPathNavigator MatchNode(XPathNavigator context) { Evaluate(new XPathSingletonIterator(context, /*moved:*/true)); XPathNavigator result; while ((result = Advance()) != null) { if (result.IsSamePosition(context)) { return context; } } return null; } public override XPathNodeIterator Clone() { return new IDQuery(this); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
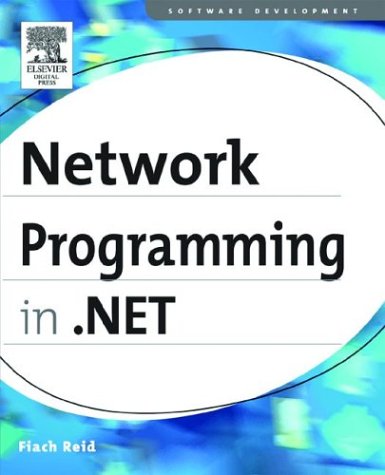
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SequenceDesigner.cs
- DecimalAnimation.cs
- ConfigurationLocation.cs
- WebHeaderCollection.cs
- CodeBlockBuilder.cs
- CryptoHandle.cs
- PartManifestEntry.cs
- TraceUtility.cs
- CommandField.cs
- XmlUnspecifiedAttribute.cs
- MetadataUtil.cs
- TypeListConverter.cs
- WebPartActionVerb.cs
- CalendarModeChangedEventArgs.cs
- QilName.cs
- Button.cs
- HebrewCalendar.cs
- PropertyChangedEventArgs.cs
- SamlNameIdentifierClaimResource.cs
- HtmlForm.cs
- ViewValidator.cs
- SqlCommandSet.cs
- CachedBitmap.cs
- TextRangeEditLists.cs
- COAUTHINFO.cs
- SystemParameters.cs
- ElementUtil.cs
- MarshalDirectiveException.cs
- ReturnType.cs
- PassportPrincipal.cs
- BitmapEffectInputData.cs
- ManagementObjectSearcher.cs
- IItemProperties.cs
- MILUtilities.cs
- EmptyEnumerator.cs
- EncodingTable.cs
- SubMenuStyle.cs
- Point4D.cs
- ProbeMatches11.cs
- DataGridViewButtonCell.cs
- OlePropertyStructs.cs
- RMEnrollmentPage3.cs
- DesignerCategoryAttribute.cs
- InvalidDataException.cs
- HtmlInputReset.cs
- IODescriptionAttribute.cs
- HttpStreamXmlDictionaryWriter.cs
- Splitter.cs
- AttributeProviderAttribute.cs
- DecimalAnimationBase.cs
- PropertyInfoSet.cs
- FontCollection.cs
- XamlReaderConstants.cs
- MatrixKeyFrameCollection.cs
- WizardPanelChangingEventArgs.cs
- PageHandlerFactory.cs
- HorizontalAlignConverter.cs
- Button.cs
- OutputCacheSettingsSection.cs
- PrinterUnitConvert.cs
- PerspectiveCamera.cs
- RowsCopiedEventArgs.cs
- ControlDesigner.cs
- RoutedUICommand.cs
- FrameDimension.cs
- KnownTypeHelper.cs
- ActiveXSite.cs
- StoreAnnotationsMap.cs
- RequiredAttributeAttribute.cs
- WindowsListViewItemStartMenu.cs
- TableSectionStyle.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- EmptyEnumerable.cs
- WorkflowServiceAttributesTypeConverter.cs
- ToolStripLabel.cs
- TraceListeners.cs
- ResourcePermissionBaseEntry.cs
- ApplicationDirectory.cs
- EpmCustomContentWriterNodeData.cs
- RecipientInfo.cs
- ResourceReferenceExpressionConverter.cs
- HandlerBase.cs
- HttpProfileBase.cs
- LazyLoadBehavior.cs
- KeyPullup.cs
- StorageMappingFragment.cs
- GrammarBuilderPhrase.cs
- BuilderPropertyEntry.cs
- x509utils.cs
- DataObjectPastingEventArgs.cs
- RuntimeHandles.cs
- WindowsStatusBar.cs
- SqlDataSourceCommandEventArgs.cs
- DesignTimeResourceProviderFactoryAttribute.cs
- LabelExpression.cs
- DataControlFieldHeaderCell.cs
- DbCommandTree.cs
- WorkflowTimerService.cs
- SqlFileStream.cs
- FrameDimension.cs