Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Common / CommandTrees / DbCommandTree.cs / 1305376 / DbCommandTree.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Data.Common; using System.Data.Common.CommandTrees.Internal; using System.Data.Common.Utils; using System.Data.Metadata.Edm; using System.Diagnostics; using System.Globalization; using System.IO; using System.Linq; using System.Text; using System.Text.RegularExpressions; namespace System.Data.Common.CommandTrees { ////// Describes the different "kinds" (classes) of command trees. /// internal enum DbCommandTreeKind { Query, Update, Insert, Delete, Function, } ////// DbCommandTree is the abstract base type for the Delete, Query, Insert and Update DbCommandTree types. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public abstract class DbCommandTree { // Bid counter private static int s_instanceCount; internal readonly int ObjectId = System.Threading.Interlocked.Increment(ref s_instanceCount); // Metadata collection private readonly MetadataWorkspace _metadata; private readonly DataSpace _dataSpace; ////// Initializes a new command tree with a given metadata workspace. /// /// The metadata workspace against which the command tree should operate. /// The logical 'space' that metadata in the expressions used in this command tree must belong to. internal DbCommandTree(MetadataWorkspace metadata, DataSpace dataSpace) { using (new EntityBid.ScopeAuto("%d#", this.ObjectId)) { // Ensure the metadata workspace is non-null EntityUtil.CheckArgumentNull(metadata, "metadata"); // Ensure that the data space value is valid if (!DbCommandTree.IsValidDataSpace(dataSpace)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_CommandTree_InvalidDataSpace, "dataSpace"); } // // Create the tree's metadata workspace and initalize commonly used types. // MetadataWorkspace effectiveMetadata = new MetadataWorkspace(); //While EdmItemCollection and StorageitemCollections are required //ObjectItemCollection may or may not be registered on the workspace yet. //So register the ObjectItemCollection if it exists. ItemCollection objectItemCollection; if (metadata.TryGetItemCollection(DataSpace.OSpace, out objectItemCollection)) { effectiveMetadata.RegisterItemCollection(objectItemCollection); } effectiveMetadata.RegisterItemCollection(metadata.GetItemCollection(DataSpace.CSpace)); effectiveMetadata.RegisterItemCollection(metadata.GetItemCollection(DataSpace.CSSpace)); effectiveMetadata.RegisterItemCollection(metadata.GetItemCollection(DataSpace.SSpace)); this._metadata = effectiveMetadata; this._dataSpace = dataSpace; } } /// /// Gets the name and corresponding type of each parameter that can be referenced within this command tree. /// public IEnumerable> Parameters { get { using(new EntityBid.ScopeAuto(" %d#", this.ObjectId)) { return this.GetParameters(); } } } #region Internal Implementation /// /// Gets the kind of this command tree. /// internal abstract DbCommandTreeKind CommandTreeKind { get; } ////// Gets the name and type of each parameter declared on the command tree. /// ///internal abstract IEnumerable > GetParameters(); /// /// Gets the metadata workspace used by this command tree. /// internal MetadataWorkspace MetadataWorkspace { get { return _metadata; } } ////// Gets the data space in which metadata used by this command tree must reside. /// internal DataSpace DataSpace { get { return _dataSpace; } } #region Dump/Print Support internal void Dump(ExpressionDumper dumper) { // // Dump information about this command tree to the specified ExpressionDumper // // First dump standard information - the DataSpace of the command tree and its parameters // Dictionaryattrs = new Dictionary (); attrs.Add("DataSpace", this.DataSpace); dumper.Begin(this.GetType().Name, attrs); // // The name and type of each Parameter in turn is added to the output // dumper.Begin("Parameters", null); foreach (KeyValuePair param in this.Parameters) { Dictionary paramAttrs = new Dictionary (); paramAttrs.Add("Name", param.Key); dumper.Begin("Parameter", paramAttrs); dumper.Dump(param.Value, "ParameterType"); dumper.End("Parameter"); } dumper.End("Parameters"); // // Delegate to the derived type's implementation that dumps the structure of the command tree // this.DumpStructure(dumper); // // Matching call to End to correspond with the call to Begin above // dumper.End(this.GetType().Name); } internal abstract void DumpStructure(ExpressionDumper dumper); internal string DumpXml() { // // This is a convenience method that dumps the command tree in an XML format. // This is intended primarily as a debugging aid to allow inspection of the tree structure. // // Create a new MemoryStream that the XML dumper should write to. // MemoryStream stream = new MemoryStream(); // // Create the dumper // XmlExpressionDumper dumper = new XmlExpressionDumper(stream); // // Dump this tree and then close the XML dumper so that the end document tag is written // and the output is flushed to the stream. // this.Dump(dumper); dumper.Close(); // // Construct a string from the resulting memory stream and return it to the caller // return XmlExpressionDumper.DefaultEncoding.GetString(stream.ToArray()); } internal string Print() { return this.PrintTree(new ExpressionPrinter()); } internal abstract string PrintTree(ExpressionPrinter printer); #endregion #region Tracing internal void Trace() { if (EntityBid.AdvancedOn) { EntityBid.PutStrChunked(this.DumpXml()); EntityBid.Trace("\n"); } else if (EntityBid.TraceOn) { EntityBid.PutStrChunked(this.Print()); EntityBid.Trace("\n"); } } #endregion internal static bool IsValidDataSpace(DataSpace dataSpace) { return (DataSpace.OSpace == dataSpace || DataSpace.CSpace == dataSpace || DataSpace.SSpace == dataSpace); } internal static bool IsValidParameterName(string name) { return (!StringUtil.IsNullOrEmptyOrWhiteSpace(name) && _paramNameRegex.IsMatch(name)); } private static readonly Regex _paramNameRegex = new Regex("^([A-Za-z])([A-Za-z0-9_])*$"); #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Data.Common; using System.Data.Common.CommandTrees.Internal; using System.Data.Common.Utils; using System.Data.Metadata.Edm; using System.Diagnostics; using System.Globalization; using System.IO; using System.Linq; using System.Text; using System.Text.RegularExpressions; namespace System.Data.Common.CommandTrees { ////// Describes the different "kinds" (classes) of command trees. /// internal enum DbCommandTreeKind { Query, Update, Insert, Delete, Function, } ////// DbCommandTree is the abstract base type for the Delete, Query, Insert and Update DbCommandTree types. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public abstract class DbCommandTree { // Bid counter private static int s_instanceCount; internal readonly int ObjectId = System.Threading.Interlocked.Increment(ref s_instanceCount); // Metadata collection private readonly MetadataWorkspace _metadata; private readonly DataSpace _dataSpace; ////// Initializes a new command tree with a given metadata workspace. /// /// The metadata workspace against which the command tree should operate. /// The logical 'space' that metadata in the expressions used in this command tree must belong to. internal DbCommandTree(MetadataWorkspace metadata, DataSpace dataSpace) { using (new EntityBid.ScopeAuto("%d#", this.ObjectId)) { // Ensure the metadata workspace is non-null EntityUtil.CheckArgumentNull(metadata, "metadata"); // Ensure that the data space value is valid if (!DbCommandTree.IsValidDataSpace(dataSpace)) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_CommandTree_InvalidDataSpace, "dataSpace"); } // // Create the tree's metadata workspace and initalize commonly used types. // MetadataWorkspace effectiveMetadata = new MetadataWorkspace(); //While EdmItemCollection and StorageitemCollections are required //ObjectItemCollection may or may not be registered on the workspace yet. //So register the ObjectItemCollection if it exists. ItemCollection objectItemCollection; if (metadata.TryGetItemCollection(DataSpace.OSpace, out objectItemCollection)) { effectiveMetadata.RegisterItemCollection(objectItemCollection); } effectiveMetadata.RegisterItemCollection(metadata.GetItemCollection(DataSpace.CSpace)); effectiveMetadata.RegisterItemCollection(metadata.GetItemCollection(DataSpace.CSSpace)); effectiveMetadata.RegisterItemCollection(metadata.GetItemCollection(DataSpace.SSpace)); this._metadata = effectiveMetadata; this._dataSpace = dataSpace; } } /// /// Gets the name and corresponding type of each parameter that can be referenced within this command tree. /// public IEnumerable> Parameters { get { using(new EntityBid.ScopeAuto(" %d#", this.ObjectId)) { return this.GetParameters(); } } } #region Internal Implementation /// /// Gets the kind of this command tree. /// internal abstract DbCommandTreeKind CommandTreeKind { get; } ////// Gets the name and type of each parameter declared on the command tree. /// ///internal abstract IEnumerable > GetParameters(); /// /// Gets the metadata workspace used by this command tree. /// internal MetadataWorkspace MetadataWorkspace { get { return _metadata; } } ////// Gets the data space in which metadata used by this command tree must reside. /// internal DataSpace DataSpace { get { return _dataSpace; } } #region Dump/Print Support internal void Dump(ExpressionDumper dumper) { // // Dump information about this command tree to the specified ExpressionDumper // // First dump standard information - the DataSpace of the command tree and its parameters // Dictionaryattrs = new Dictionary (); attrs.Add("DataSpace", this.DataSpace); dumper.Begin(this.GetType().Name, attrs); // // The name and type of each Parameter in turn is added to the output // dumper.Begin("Parameters", null); foreach (KeyValuePair param in this.Parameters) { Dictionary paramAttrs = new Dictionary (); paramAttrs.Add("Name", param.Key); dumper.Begin("Parameter", paramAttrs); dumper.Dump(param.Value, "ParameterType"); dumper.End("Parameter"); } dumper.End("Parameters"); // // Delegate to the derived type's implementation that dumps the structure of the command tree // this.DumpStructure(dumper); // // Matching call to End to correspond with the call to Begin above // dumper.End(this.GetType().Name); } internal abstract void DumpStructure(ExpressionDumper dumper); internal string DumpXml() { // // This is a convenience method that dumps the command tree in an XML format. // This is intended primarily as a debugging aid to allow inspection of the tree structure. // // Create a new MemoryStream that the XML dumper should write to. // MemoryStream stream = new MemoryStream(); // // Create the dumper // XmlExpressionDumper dumper = new XmlExpressionDumper(stream); // // Dump this tree and then close the XML dumper so that the end document tag is written // and the output is flushed to the stream. // this.Dump(dumper); dumper.Close(); // // Construct a string from the resulting memory stream and return it to the caller // return XmlExpressionDumper.DefaultEncoding.GetString(stream.ToArray()); } internal string Print() { return this.PrintTree(new ExpressionPrinter()); } internal abstract string PrintTree(ExpressionPrinter printer); #endregion #region Tracing internal void Trace() { if (EntityBid.AdvancedOn) { EntityBid.PutStrChunked(this.DumpXml()); EntityBid.Trace("\n"); } else if (EntityBid.TraceOn) { EntityBid.PutStrChunked(this.Print()); EntityBid.Trace("\n"); } } #endregion internal static bool IsValidDataSpace(DataSpace dataSpace) { return (DataSpace.OSpace == dataSpace || DataSpace.CSpace == dataSpace || DataSpace.SSpace == dataSpace); } internal static bool IsValidParameterName(string name) { return (!StringUtil.IsNullOrEmptyOrWhiteSpace(name) && _paramNameRegex.IsMatch(name)); } private static readonly Regex _paramNameRegex = new Regex("^([A-Za-z])([A-Za-z0-9_])*$"); #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
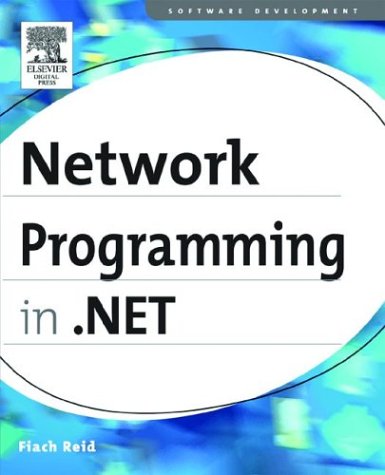
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CodePageUtils.cs
- DBSchemaRow.cs
- LambdaSerializationException.cs
- AsnEncodedData.cs
- TriggerActionCollection.cs
- ReadWriteSpinLock.cs
- DbConnectionPoolCounters.cs
- MetadataItemCollectionFactory.cs
- UnsettableComboBox.cs
- SiteMapProvider.cs
- Point4D.cs
- ArcSegment.cs
- DefaultValueConverter.cs
- ObjectStateManager.cs
- ZipIOLocalFileBlock.cs
- PointCollection.cs
- HttpCookieCollection.cs
- WebPartManager.cs
- WindowsAuthenticationEventArgs.cs
- DesignTableCollection.cs
- UnmanagedHandle.cs
- SqlLiftIndependentRowExpressions.cs
- ProfilePropertyNameValidator.cs
- FileResponseElement.cs
- PropertyGeneratedEventArgs.cs
- xdrvalidator.cs
- UrlParameterReader.cs
- ExceptionHandlersDesigner.cs
- IncrementalHitTester.cs
- SqlUtil.cs
- ExpressionPrinter.cs
- PersonalizationProviderHelper.cs
- XmlWrappingReader.cs
- NameValuePair.cs
- OutputScopeManager.cs
- TransformValueSerializer.cs
- Set.cs
- DataGridViewRowPostPaintEventArgs.cs
- RsaSecurityTokenAuthenticator.cs
- ObjectStateEntry.cs
- Glyph.cs
- HttpAsyncResult.cs
- util.cs
- DataGridRelationshipRow.cs
- MemoryFailPoint.cs
- Oid.cs
- TypeRestriction.cs
- _SslSessionsCache.cs
- DLinqAssociationProvider.cs
- CqlBlock.cs
- Model3DGroup.cs
- RoleGroupCollection.cs
- TypeUnloadedException.cs
- SwitchLevelAttribute.cs
- WebPartUserCapability.cs
- PermissionSet.cs
- DefaultHttpHandler.cs
- ApplicationSecurityInfo.cs
- XmlElementList.cs
- TemplatePagerField.cs
- BrowserCapabilitiesFactory.cs
- VideoDrawing.cs
- RoleManagerSection.cs
- MLangCodePageEncoding.cs
- LocalizableAttribute.cs
- DrawingCollection.cs
- ClaimSet.cs
- RbTree.cs
- UIElementHelper.cs
- WebAdminConfigurationHelper.cs
- HttpGetClientProtocol.cs
- DataColumnChangeEvent.cs
- ParameterCollection.cs
- MenuItemBinding.cs
- MarkedHighlightComponent.cs
- FreezableOperations.cs
- ApplicationDirectoryMembershipCondition.cs
- GeneralTransform3DTo2DTo3D.cs
- WindowsTab.cs
- _emptywebproxy.cs
- Vars.cs
- XmlSiteMapProvider.cs
- VisualStyleRenderer.cs
- UrlAuthFailedErrorFormatter.cs
- Interfaces.cs
- NativeMethods.cs
- MdImport.cs
- DesignTimeResourceProviderFactoryAttribute.cs
- OrderPreservingPipeliningSpoolingTask.cs
- FileLogRecordEnumerator.cs
- DbConnectionHelper.cs
- ProfileSection.cs
- InputBuffer.cs
- HttpListenerRequestUriBuilder.cs
- EventPropertyMap.cs
- ManifestBasedResourceGroveler.cs
- WebPartZoneDesigner.cs
- ExpressionDumper.cs
- ToolStrip.cs
- XmlSchemaAnnotation.cs