Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / NativeMethods.cs / 1 / NativeMethods.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.Security; using System.Text; using Microsoft.Win32.SafeHandles; using System.Runtime.InteropServices; using System.Runtime.ConstrainedExecution; internal static unsafe /*partial*/ class NativeMethods { public const int E_BUSY = unchecked( (int)0x80070047 ); public const int ERROR_ACCESS_DENIED = 5; public const int ERROR_NOT_ENOUGH_MEMORY = 8; public const int ERROR_OUTOFMEMORY = 14; public const int E_NOTIMPL = unchecked( (int) 0x80004001 ); public const int E_ACCESSDENIED = unchecked( (int)0x80070005 ); public const int COR_E_APPLICATION = unchecked((int)0x80131600); public const int COR_E_ARGUMENT = unchecked((int)0x80070057); public const int ERROR_INVALID_DATA = 13; public const int CRYPTPROTECT_LOCAL_MACHINE = 0x04; public const int CRYPTPROTECT_UI_FORBIDDEN = 0x01; public const int CRYPTPROTECT_NO_RECOVERY = 0x20; public const int CRYPTPROTECT_VERIFY_PROTECTION = 0x40; public const int CRYPTPROTECT_AUDIT = 0x10; public const int CRYPTPROTECTMEMORY_SAME_PROCESS = 0x00; public const int CRYPTPROTECTMEMORY_CROSS_PROCESS = 0x01; public const int CRYPTPROTECTMEMORY_SAME_LOGON = 0x02; public const int EVENT_MODIFY_STATE = 0x2; public const int PROCESS_DUP_HANDLE = 0x0040; public const int TOKEN_QUERY = 0x0008; public const int TOKEN_IMPERSONATE = 0x0004; public const int TOKEN_DUPLICATE = 0x0002; public const int TOKEN_ASSIGN_PRIMARY = 0x0001; public const int TOKEN_ALL_ACCESS = 0x01ff; public const uint WAIT_TIMEOUT = 258; public const uint WAIT_ABANDONED = 0x00000080; public const uint WAIT_FAILED = 0xffffffff; public const int CSIDL_LOCAL_APPDATA = 0x001c; public const int SHGFP_TYPE_CURRENT = 0; public const int MAX_PATH = 260; public const int MUTEX_MODIFY_STATE = 0x0002; public const int SYNCHRONIZE = 0x00100000; // // Used for get volume information to detect ntfs / fat. // public const int FILE_PERSISTENT_ACLS = 0x00000008; public const int ERROR_CANCELLED = 1223; public const int SM_TABLETPC = 86; [DllImport( "Crypt32.dll", EntryPoint = "CryptProtectData", CharSet = CharSet.Unicode, ExactSpelling = true, SetLastError = true )] public static extern bool CryptProtectData( [In] IntPtr pDataIn, [In, MarshalAs( UnmanagedType.LPWStr )] string szDataDescr, [In] IntPtr pOptionalEntropy, [In] IntPtr pvReserved, [In] IntPtr pPromptStruct, [In, MarshalAs( UnmanagedType.I4 )] int dwFlags, [In] IntPtr pDataOut ); [DllImport( "Crypt32.dll", EntryPoint = "CryptUnprotectData", CharSet = CharSet.Unicode, ExactSpelling = true, SetLastError = true )] public static extern bool CryptUnprotectData( [In] IntPtr pDataIn, [Out, MarshalAs( UnmanagedType.LPWStr )] System.Text.StringBuilder ppszDataDescr, [In] IntPtr pOptionalEntropy, [In] IntPtr pvReserved, [In] IntPtr pPromptStruct, [In, MarshalAs( UnmanagedType.I4 )] int dwFlags, [In] IntPtr pDataOut ); [DllImport( "Rpcrt4.dll", EntryPoint = "RpcImpersonateClient", CharSet = CharSet.Unicode, ExactSpelling = true, SetLastError = false )] public static extern uint RpcImpersonateClient( [In] IntPtr rpcBindingHandle ); [DllImport( "Rpcrt4.dll", EntryPoint = "RpcRevertToSelfEx", CharSet = CharSet.Unicode, ExactSpelling = true, SetLastError = false )] public static extern uint RpcRevertToSelfEx( [In] IntPtr rpcBindingHandle ); [DllImport( "Rpcrt4.dll", EntryPoint = "I_RpcBindingInqLocalClientPID", CharSet = CharSet.Unicode, ExactSpelling = true, SetLastError = false )] public static extern uint I_RpcBindingInqLocalClientPID( [In] IntPtr rpcBindingHandle, [Out] out uint pid ); [DllImport( "advapi32.dll", EntryPoint = "RevertToSelf", CharSet = CharSet.Unicode, ExactSpelling = true, SetLastError = true )] public static extern bool RevertToSelf(); [DllImport( "advapi32.dll", EntryPoint = "ImpersonateLoggedOnUser", CharSet = CharSet.Unicode, ExactSpelling = true, SetLastError = true )] public static extern bool ImpersonateLoggedOnUser( [In] IntPtr hToken ); [DllImport( "kernel32.dll", EntryPoint = "ProcessIdToSessionId", CharSet = CharSet.Unicode, ExactSpelling = true, SetLastError = true )] public static extern bool ProcessIdToSessionId( [In] int pid, [Out] out int tSSession ); [DllImport( "kernel32.dll", EntryPoint = "OpenProcess", CharSet = CharSet.Unicode, ExactSpelling = true, SetLastError = true )] public static extern SafeNativeHandle OpenProcess( [In] int desiredAccess, [In] bool inheritHandle, [In] int processId ); [DllImport( "kernel32.dll", EntryPoint = "DuplicateHandle", CharSet = CharSet.Unicode, ExactSpelling = true, SetLastError = true )] public static extern bool DuplicateHandle( [In] SafeNativeHandle sourceProcessHandle, [In] SafeWaitHandle sourceHandle, [In] SafeNativeHandle targetProcessHandle, [Out] out SafeWaitHandle targetHandle, [In] int desiredAccess, [In] bool inheritHandle, [In] int options ); [DllImport( "kernel32.dll", EntryPoint = "GetCurrentProcess", CharSet = CharSet.Unicode, ExactSpelling = true, SetLastError = true )] public static extern IntPtr GetCurrentProcess(); public enum SecurityImpersonationLevel { SecurityAnonymous, SecurityIdentification, SecurityImpersonation, SecurityDelegation }; [DllImport( "Kernel32.dll", EntryPoint = "LocalFree", CharSet = CharSet.Unicode, ExactSpelling = true, SetLastError = true ) ] public static extern IntPtr LocalFree( IntPtr hMem ); [DllImport( "advapi32.dll", EntryPoint ="CryptDecrypt", CharSet = CharSet.Unicode, ExactSpelling = true, SetLastError = true ) ] public static extern bool CryptDecrypt( [In] SafeCryptoKeyHandle hKey, [In] IntPtr hHash, [In] UInt32 Final, [In] UInt32 Flags, [In] IntPtr data, [In,Out] ref UInt32 length ); [DllImport( "advapi32.dll", EntryPoint ="CryptEncrypt", CharSet = CharSet.Unicode, ExactSpelling = true, SetLastError = true ) ] public static extern bool CryptEncrypt( [In] SafeCryptoKeyHandle hKey, [In] IntPtr hHash, [In] UInt32 Final, [In] UInt32 Flags, [In] IntPtr data, [In,Out] ref UInt32 length, [In] UInt32 bufLength ); [DllImport("Kernel32.dll", EntryPoint ="RtlZeroMemory", CharSet = CharSet.Unicode, ExactSpelling = true, SetLastError = true ) ] public static extern void ZeroMemory( [In] IntPtr dest, [In] Int32 size ); // // In order to support certs with unicode values, we explicitly specify the wide version of CertGetNameString. // CertGetNameStringW expects an ANSI string as input for pvTypePara when CERT_NAME_ATTR_TYPE is defined- // marshal pvTypePara as an LPStr. // [DllImport( "Crypt32.dll", EntryPoint = "CertGetNameStringW", CharSet = CharSet.Unicode, ExactSpelling = true, SetLastError = false )] public static extern int CertGetNameString( IntPtr pCertContext, int dwType, int dwFlags, [In, MarshalAs( UnmanagedType.LPStr ) ] string pvTypePara, [Out] System.Text.StringBuilder pszNameString, int cchNameString ); [DllImport("Kernel32.dll", EntryPoint ="WTSGetActiveConsoleSessionId", CharSet = CharSet.Unicode, ExactSpelling = true, SetLastError = false )] public static extern int WTSGetActiveConsoleSessionId(); [DllImport("user32.dll", EntryPoint ="GetSystemMetrics", CharSet = CharSet.Unicode, ExactSpelling = true, SetLastError = false )] public static extern int GetSystemMetrics( int nIndex ); // // CloseHandle should only be called by SafeHandle classes to ensure we do not // corrupt state of the IntPtr passed in // public sealed class SafeHandleOnlyMethods { private SafeHandleOnlyMethods() { } [DllImport( "Kernel32.dll", EntryPoint = "CloseHandle", CharSet = CharSet.Unicode, ExactSpelling = true, SetLastError = true )] [ReliabilityContract( Consistency.WillNotCorruptState, Cer.Success )] public static extern bool CloseHandle( [In] IntPtr handle ); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
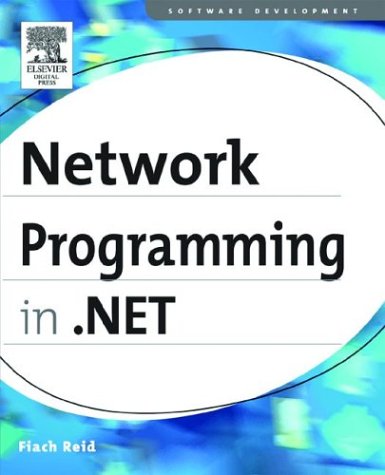
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WorkingDirectoryEditor.cs
- BinHexEncoder.cs
- TextChangedEventArgs.cs
- Tag.cs
- InstanceDataCollectionCollection.cs
- BindingExpressionBase.cs
- FixedStringLookup.cs
- SemanticResolver.cs
- OlePropertyStructs.cs
- DeviceSpecificDesigner.cs
- SizeAnimation.cs
- DbDataAdapter.cs
- BinaryParser.cs
- OfTypeExpression.cs
- QueueProcessor.cs
- Operand.cs
- ExpanderAutomationPeer.cs
- TraceListeners.cs
- XamlHostingSection.cs
- StringConverter.cs
- VisualBrush.cs
- XmlName.cs
- StringReader.cs
- InternalConfigSettingsFactory.cs
- TypographyProperties.cs
- MimeMultiPart.cs
- CaseCqlBlock.cs
- PrintPreviewDialog.cs
- IgnoreFlushAndCloseStream.cs
- RegexCode.cs
- Win32MouseDevice.cs
- ValidationEventArgs.cs
- DataGridViewTopRowAccessibleObject.cs
- TabControl.cs
- CoreSwitches.cs
- StringFormat.cs
- MobileResource.cs
- CollectionViewGroup.cs
- PathFigureCollection.cs
- LinkTarget.cs
- StringSorter.cs
- DataObjectAttribute.cs
- X509SecurityToken.cs
- SafeSystemMetrics.cs
- Attachment.cs
- DataGridTextBox.cs
- QueryRewriter.cs
- NameValueSectionHandler.cs
- DataGridViewRowsAddedEventArgs.cs
- SourceLocation.cs
- ItemContainerPattern.cs
- PropertyGeneratedEventArgs.cs
- OracleCommandBuilder.cs
- LabelInfo.cs
- MimeImporter.cs
- PathGeometry.cs
- DataGridViewUtilities.cs
- ControlCommandSet.cs
- SafeRegistryHandle.cs
- SamlConditions.cs
- PersonalizationStateQuery.cs
- SqlXml.cs
- WebPartConnectionsEventArgs.cs
- DataGridViewTopLeftHeaderCell.cs
- Win32Exception.cs
- Span.cs
- PartManifestEntry.cs
- SqlMethods.cs
- ResourcePart.cs
- BaseCodeDomTreeGenerator.cs
- UrlMappingsModule.cs
- TabControl.cs
- BinaryEditor.cs
- WebPartUserCapability.cs
- TableRowCollection.cs
- PopOutPanel.cs
- KnownIds.cs
- WindowsFormsHelpers.cs
- CollectionViewGroupInternal.cs
- RootDesignerSerializerAttribute.cs
- WebPartConnectionsDisconnectVerb.cs
- PropertyNames.cs
- EventDescriptor.cs
- XsdDuration.cs
- InkCanvasFeedbackAdorner.cs
- DataGridViewColumnEventArgs.cs
- SqlFunctionAttribute.cs
- ColorAnimationBase.cs
- UIElementIsland.cs
- TypeGenericEnumerableViewSchema.cs
- ToolStripItem.cs
- AssemblyName.cs
- TrustManager.cs
- DrawingDrawingContext.cs
- StatusBarAutomationPeer.cs
- precedingsibling.cs
- MetadataSource.cs
- ReadWriteSpinLock.cs
- XmlSchemaRedefine.cs
- SaveFileDialog.cs