Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.Activities / System / Activities / Debugger / SourceLocation.cs / 1305376 / SourceLocation.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.Activities.Debugger { using System; using System.Diagnostics; using System.Runtime; using System.Globalization; // Identifies a specific location in the target source code. // // This source information is used in creating PDBs, which will be passed to the debugger, // which will resolve the source file based off its own source paths. // Source ranges can: // * refer to just an entire single line. // * can be a subset within a single line (when StartLine == EndLine) // * can also span multiple lines. // When column info is provided, the debugger will highlight the characters starting at the start line and start column, // and going up to but not including the character specified by the end line and end column. [DebuggerNonUserCode] [Serializable] [Fx.Tag.XamlVisible(false)] public class SourceLocation { string fileName; int startLine; int endLine; int startColumn; int endColumn; // Define a source location from a filename and line-number (1-based). // This is a convenience constructor to specify the entire line. // This does not load the source file to determine column ranges. public SourceLocation(string fileName, int line) : this(fileName, line, 1, line, int.MaxValue) { } // Define a source location in a file. // Line/Column are 1-based. public SourceLocation( string fileName, int startLine, int startColumn, int endLine, int endColumn) { if (startLine <= 0) { throw FxTrace.Exception.Argument("startLine", SR.InvalidSourceLocationLineNumber("startLine", startLine)); } if (startColumn <= 0) { throw FxTrace.Exception.Argument("startColumn", SR.InvalidSourceLocationColumn("startColumn", startColumn)); } if (endLine <= 0) { throw FxTrace.Exception.Argument("endLine", SR.InvalidSourceLocationLineNumber("endLine", endLine)); } if (endColumn <= 0) { throw FxTrace.Exception.Argument("endColumn", SR.InvalidSourceLocationColumn("endColumn", endColumn)); } if (startLine > endLine) { throw FxTrace.Exception.ArgumentOutOfRange("endLine", endLine, SR.OutOfRangeSourceLocationEndLine(startLine)); } if ((startLine == endLine) && (startColumn > endColumn)) { throw FxTrace.Exception.ArgumentOutOfRange("endColumn", endColumn, SR.OutOfRangeSourceLocationEndColumn(startColumn)); } this.fileName = (fileName != null) ? fileName.ToUpperInvariant() : null; this.startLine = startLine; this.endLine = endLine; this.startColumn = startColumn; this.endColumn = endColumn; } public string FileName { get { return this.fileName; } } // Get the 1-based start line. public int StartLine { get { return this.startLine; } } // Get the 1-based starting column. public int StartColumn { get { return this.startColumn; } } // Get the 1-based end line. This should be greater or equal to StartLine. public int EndLine { get { return this.endLine; } } // Get the 1-based ending column. public int EndColumn { get { return this.endColumn; } } public bool IsSingleWholeLine { get { return this.endColumn == int.MaxValue && this.startLine == this.endLine && this.startColumn == 1; } } // Equality comparison function. This checks for strict equality and // not for superset or subset relationships. public override bool Equals(object obj) { SourceLocation rsl = obj as SourceLocation; if (rsl == null) { return false; } if (this.FileName != rsl.FileName) { return false; } if (this.StartLine != rsl.StartLine || this.StartColumn != rsl.StartColumn || this.EndLine != rsl.EndLine || this.EndColumn != rsl.EndColumn) { return false; } // everything matches return true; } // Get a hash code. public override int GetHashCode() { return (this.FileName.GetHashCode() ^ this.StartLine.GetHashCode() ^ this.StartColumn.GetHashCode()); } internal static bool IsValidRange(int startLine, int startColumn, int endLine, int endColumn) { return (startLine > 0) && (startColumn > 0) && (endLine > 0) && (endColumn > 0) && ((startLine < endLine) || (startLine == endLine) && (startColumn < endColumn)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
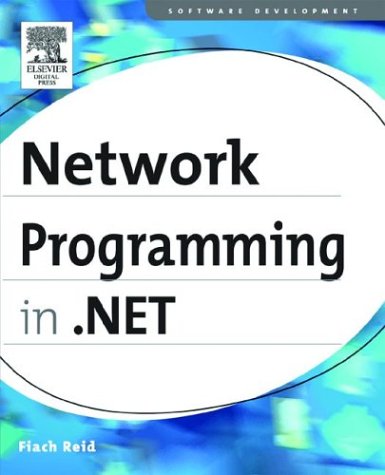
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CommandField.cs
- TextParaLineResult.cs
- SpoolingTask.cs
- Attachment.cs
- Soap11ServerProtocol.cs
- RTLAwareMessageBox.cs
- DispatcherFrame.cs
- XmlAttributeAttribute.cs
- GrabHandleGlyph.cs
- HttpSysSettings.cs
- XPathNavigatorKeyComparer.cs
- DataGridViewTextBoxColumn.cs
- DbModificationCommandTree.cs
- PointCollectionValueSerializer.cs
- MailSettingsSection.cs
- XPathAncestorQuery.cs
- PageParser.cs
- DataGridCommandEventArgs.cs
- TextServicesContext.cs
- PropertyMapper.cs
- FileClassifier.cs
- ListCollectionView.cs
- RootBrowserWindowProxy.cs
- NotifyIcon.cs
- BatchWriter.cs
- Button.cs
- DbgUtil.cs
- ListSourceHelper.cs
- BinHexEncoder.cs
- ElementInit.cs
- StylusPointPropertyInfoDefaults.cs
- CipherData.cs
- ListItemCollection.cs
- NavigatorOutput.cs
- MessagingDescriptionAttribute.cs
- HttpStreamXmlDictionaryReader.cs
- __Filters.cs
- WebCategoryAttribute.cs
- _BaseOverlappedAsyncResult.cs
- XamlFilter.cs
- EntityDataSourceConfigureObjectContextPanel.cs
- RewritingValidator.cs
- FieldMetadata.cs
- HttpCachePolicyElement.cs
- SecUtil.cs
- PortCache.cs
- CodeSubDirectory.cs
- SafeCertificateContext.cs
- TraceUtility.cs
- KerberosSecurityTokenParameters.cs
- Typography.cs
- GregorianCalendarHelper.cs
- DispatcherHooks.cs
- PriorityItem.cs
- BoundField.cs
- ResourceDisplayNameAttribute.cs
- MenuItem.cs
- DiffuseMaterial.cs
- SelectorAutomationPeer.cs
- HttpContext.cs
- Setter.cs
- InvalidDataException.cs
- ACL.cs
- WebPartRestoreVerb.cs
- KeyedHashAlgorithm.cs
- TypeBuilderInstantiation.cs
- ProjectionPathSegment.cs
- DecimalAnimationUsingKeyFrames.cs
- Function.cs
- BitmapDownload.cs
- XmlSchemaAll.cs
- DesignerUtility.cs
- DataListItem.cs
- Link.cs
- Span.cs
- CodeGenerator.cs
- PropertyEmitterBase.cs
- AutomationPeer.cs
- RegistrationServices.cs
- AssemblyContextControlItem.cs
- latinshape.cs
- __ConsoleStream.cs
- Attributes.cs
- ProjectedWrapper.cs
- Script.cs
- ScriptIgnoreAttribute.cs
- XmlUTF8TextWriter.cs
- NameValuePermission.cs
- WizardForm.cs
- ContentElement.cs
- TimeoutValidationAttribute.cs
- WebPartConnectionsDisconnectVerb.cs
- ValidationResult.cs
- ReliabilityContractAttribute.cs
- PagerSettings.cs
- WindowsTooltip.cs
- keycontainerpermission.cs
- XmlDataSourceNodeDescriptor.cs
- CallbackValidator.cs
- UserControl.cs