Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Xml / System / Xml / XPath / Internal / XPathAncestorQuery.cs / 1305376 / XPathAncestorQuery.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace MS.Internal.Xml.XPath { using System; using System.Xml; using System.Xml.XPath; using System.Diagnostics; using System.Collections.Generic; internal sealed class XPathAncestorQuery : CacheAxisQuery { private bool matchSelf; public XPathAncestorQuery(Query qyInput, string name, string prefix, XPathNodeType typeTest, bool matchSelf) : base(qyInput, name, prefix, typeTest) { this.matchSelf = matchSelf; } private XPathAncestorQuery(XPathAncestorQuery other) : base(other) { this.matchSelf = other.matchSelf; } public override object Evaluate(XPathNodeIterator context) { base.Evaluate(context); XPathNavigator ancestor = null; XPathNavigator input; while ((input = qyInput.Advance()) != null) { if (matchSelf) { if (matches(input)) { if (!Insert(outputBuffer, input)) { // If input is already in output buffer all its ancestors are in a buffer as well. continue; } } } if (ancestor == null || ! ancestor.MoveTo(input)) { ancestor = input.Clone(); } while (ancestor.MoveToParent()) { if (matches(ancestor)) { if (!Insert(outputBuffer, ancestor)) { // If input is already in output buffer all its ancestors are in a buffer as well. break; } } } } return this; } public override XPathNodeIterator Clone() { return new XPathAncestorQuery(this); } public override int CurrentPosition { get { return outputBuffer.Count - count + 1; } } public override QueryProps Properties { get { return base.Properties | QueryProps.Reverse; } } public override void PrintQuery(XmlWriter w) { w.WriteStartElement(this.GetType().Name); if (matchSelf) { w.WriteAttributeString("self", "yes"); } if (NameTest) { w.WriteAttributeString("name", Prefix.Length != 0 ? Prefix + ':' + Name : Name); } if (TypeTest != XPathNodeType.Element) { w.WriteAttributeString("nodeType", TypeTest.ToString()); } qyInput.PrintQuery(w); w.WriteEndElement(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace MS.Internal.Xml.XPath { using System; using System.Xml; using System.Xml.XPath; using System.Diagnostics; using System.Collections.Generic; internal sealed class XPathAncestorQuery : CacheAxisQuery { private bool matchSelf; public XPathAncestorQuery(Query qyInput, string name, string prefix, XPathNodeType typeTest, bool matchSelf) : base(qyInput, name, prefix, typeTest) { this.matchSelf = matchSelf; } private XPathAncestorQuery(XPathAncestorQuery other) : base(other) { this.matchSelf = other.matchSelf; } public override object Evaluate(XPathNodeIterator context) { base.Evaluate(context); XPathNavigator ancestor = null; XPathNavigator input; while ((input = qyInput.Advance()) != null) { if (matchSelf) { if (matches(input)) { if (!Insert(outputBuffer, input)) { // If input is already in output buffer all its ancestors are in a buffer as well. continue; } } } if (ancestor == null || ! ancestor.MoveTo(input)) { ancestor = input.Clone(); } while (ancestor.MoveToParent()) { if (matches(ancestor)) { if (!Insert(outputBuffer, ancestor)) { // If input is already in output buffer all its ancestors are in a buffer as well. break; } } } } return this; } public override XPathNodeIterator Clone() { return new XPathAncestorQuery(this); } public override int CurrentPosition { get { return outputBuffer.Count - count + 1; } } public override QueryProps Properties { get { return base.Properties | QueryProps.Reverse; } } public override void PrintQuery(XmlWriter w) { w.WriteStartElement(this.GetType().Name); if (matchSelf) { w.WriteAttributeString("self", "yes"); } if (NameTest) { w.WriteAttributeString("name", Prefix.Length != 0 ? Prefix + ':' + Name : Name); } if (TypeTest != XPathNodeType.Element) { w.WriteAttributeString("nodeType", TypeTest.ToString()); } qyInput.PrintQuery(w); w.WriteEndElement(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
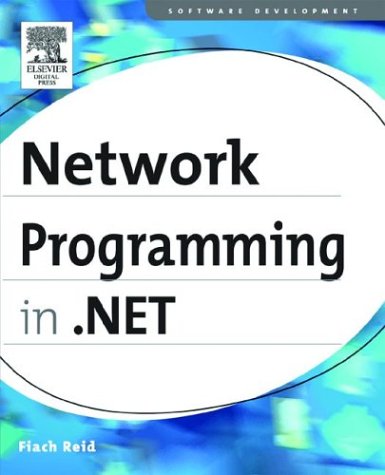
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Odbc32.cs
- XmlQueryTypeFactory.cs
- X500Name.cs
- SelectionEditingBehavior.cs
- KeySplineConverter.cs
- PropertyDescriptorCollection.cs
- AdornerLayer.cs
- WindowsSlider.cs
- LambdaCompiler.Statements.cs
- DesignerActionItem.cs
- ConvertersCollection.cs
- AnonymousIdentificationModule.cs
- FixedSOMContainer.cs
- GraphicsContainer.cs
- AncillaryOps.cs
- SimpleHandlerFactory.cs
- Ipv6Element.cs
- Debugger.cs
- RequestStatusBarUpdateEventArgs.cs
- KerberosTokenFactoryCredential.cs
- RightsManagementInformation.cs
- XmlAttributeCollection.cs
- HostedHttpRequestAsyncResult.cs
- PageRequestManager.cs
- CommandTreeTypeHelper.cs
- SafeRightsManagementPubHandle.cs
- RootBrowserWindowAutomationPeer.cs
- DBConnectionString.cs
- SortedList.cs
- util.cs
- CodeExpressionCollection.cs
- Descriptor.cs
- DataBoundControlAdapter.cs
- ObjectStateManagerMetadata.cs
- RegionInfo.cs
- ProfileModule.cs
- WebPartDisplayMode.cs
- ContainerUIElement3D.cs
- Rotation3DKeyFrameCollection.cs
- ListViewContainer.cs
- DurationConverter.cs
- FormViewDeletedEventArgs.cs
- WindowsAuthenticationModule.cs
- FamilyCollection.cs
- TextElementAutomationPeer.cs
- GridLengthConverter.cs
- AbsoluteQuery.cs
- MenuTracker.cs
- MessageOperationFormatter.cs
- PropertyToken.cs
- ThreadExceptionDialog.cs
- SqlParameter.cs
- SqlMethodAttribute.cs
- VerificationException.cs
- WmlValidationSummaryAdapter.cs
- Int32AnimationUsingKeyFrames.cs
- AliasedSlot.cs
- NativeMethods.cs
- UndoManager.cs
- WindowsProgressbar.cs
- DataServices.cs
- SqlRowUpdatingEvent.cs
- DbProviderConfigurationHandler.cs
- wgx_sdk_version.cs
- ThumbButtonInfoCollection.cs
- ResourceAssociationType.cs
- ServiceDeploymentInfo.cs
- ReadOnlyMetadataCollection.cs
- Funcletizer.cs
- PieceNameHelper.cs
- FileSecurity.cs
- EventHandlers.cs
- SecurityElement.cs
- DSACryptoServiceProvider.cs
- TableItemStyle.cs
- QueryCursorEventArgs.cs
- PermissionToken.cs
- NameSpaceExtractor.cs
- WindowsGraphics2.cs
- NavigationEventArgs.cs
- AppDomain.cs
- SchemaAttDef.cs
- RemotingServices.cs
- DiscoveryDocumentLinksPattern.cs
- MetadataItemCollectionFactory.cs
- ObjectDisposedException.cs
- DynamicQueryableWrapper.cs
- AttributeUsageAttribute.cs
- BaseTemplateParser.cs
- XmlTextEncoder.cs
- EnumConverter.cs
- ZeroOpNode.cs
- ObjectCloneHelper.cs
- ToolStripDesignerUtils.cs
- CellLabel.cs
- TextReader.cs
- XamlHostingSection.cs
- XmlElementCollection.cs
- DbConnectionPoolCounters.cs
- Decimal.cs