Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / untmp / whidbey / QFE / ndp / fx / src / xsp / System / Web / Security / WindowsAuthenticationModule.cs / 3 / WindowsAuthenticationModule.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * WindowsAuthenticationModule class * * Copyright (c) 1999 Microsoft Corporation */ namespace System.Web.Security { using System.Web; using System.Web.Configuration; using System.Security.Principal; using System.Security.Permissions; using System.Globalization; using System.Web.Management; using System.Web.Util; using System.Web.Hosting; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class WindowsAuthenticationModule : IHttpModule { private WindowsAuthenticationEventHandler _eventHandler; private static bool _fAuthChecked; private static bool _fAuthRequired; private static WindowsIdentity _anonymousIdentity; private static WindowsPrincipal _anonymousPrincipal; ////// Allows ASP.NET applications to use Windows/IIS authentication. /// ////// [SecurityPermission(SecurityAction.Demand, Unrestricted=true)] public WindowsAuthenticationModule() { } ////// Initializes a new instance of the ////// class. /// /// This is a global.asax event that must be /// named WindowsAuthenticate_OnAuthenticate event. It's used primarily to attach a /// custom IPrincipal object to the context. /// public event WindowsAuthenticationEventHandler Authenticate { add { _eventHandler += value; } remove { _eventHandler -= value; } } ////// public void Dispose() { } ///[To be supplied.] ////// public void Init(HttpApplication app) { app.AuthenticateRequest += new EventHandler(this.OnEnter); } //////////////////////////////////////////////////////////// // OnAuthenticate: Custom Authentication modules can override // this method to create a custom IPrincipal object from // a WindowsIdentity ///[To be supplied.] ////// Calls the /// WindowsAuthentication_OnAuthenticate handler if one exists. /// void OnAuthenticate(WindowsAuthenticationEventArgs e) { //////////////////////////////////////////////////////////// // If there are event handlers, invoke the handlers if (_eventHandler != null) _eventHandler(this, e); if (e.Context.User == null) { if (e.User != null) e.Context.User = e.User; else if (e.Identity == _anonymousIdentity) e.Context.SetPrincipalNoDemand(_anonymousPrincipal, false /*needToSetNativePrincipal*/); else e.Context.SetPrincipalNoDemand(new WindowsPrincipal(e.Identity), false /*needToSetNativePrincipal*/); } } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// // Methods for internal implementation ////// /// void OnEnter(Object source, EventArgs eventArgs) { if (!IsEnabled) return; HttpApplication app = (HttpApplication)source; HttpContext context = app.Context;; WindowsIdentity identity = null; ////////////////////////////////////////////////////////////////// // Step 2: Create a Windows Identity from the credentials from IIS if (HttpRuntime.UseIntegratedPipeline) { // The native WindowsAuthenticationModule sets the user principal in IIS7WorkerRequest.SynchronizeVariables. // The managed WindowsAuthenticationModule provides backward compatibility by rasing the OnAuthenticate event. WindowsPrincipal user = context.User as WindowsPrincipal; if (user != null) { // identity will be null if this is not a WindowsIdentity identity = user.Identity as WindowsIdentity; // clear Context.User for backward compatibility (it will be set in OnAuthenticate) context.SetPrincipalNoDemand(null, false /*needToSetNativePrincipal*/); } } else { String strLogonUser = context.WorkerRequest.GetServerVariable("LOGON_USER"); String strAuthType = context.WorkerRequest.GetServerVariable("AUTH_TYPE"); if (strLogonUser == null) { strLogonUser = String.Empty; } if (strAuthType == null) { strAuthType = String.Empty; } if (strLogonUser.Length == 0 && (strAuthType.Length == 0 || StringUtil.EqualsIgnoreCase(strAuthType, "basic"))) { //////////////////////////////////////////////////////// // Step 2a: Use the anonymous identity identity = _anonymousIdentity; } else { identity = new WindowsIdentity( context.WorkerRequest.GetUserToken(), strAuthType, WindowsAccountType.Normal, true); } } /////////////////////////////////////////////////////////////////////////////////// // Step 3: Call OnAuthenticate to create IPrincipal for this request. if (identity != null) { OnAuthenticate( new WindowsAuthenticationEventArgs(identity, context) ); } } internal static IPrincipal AnonymousPrincipal { get { return _anonymousPrincipal; } } internal static bool IsEnabled { get { if (!_fAuthChecked) { AuthenticationSection settings = RuntimeConfig.GetAppConfig().Authentication; settings.ValidateAuthenticationMode(); _fAuthRequired = (settings.Mode == AuthenticationMode.Windows); if (_fAuthRequired) { _anonymousIdentity = WindowsIdentity.GetAnonymous(); _anonymousPrincipal = new WindowsPrincipal(_anonymousIdentity); } _fAuthChecked = true; } return _fAuthRequired; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
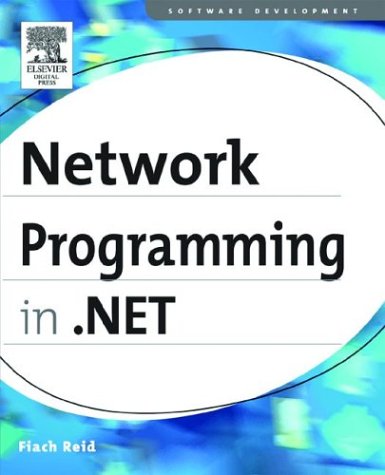
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GridViewEditEventArgs.cs
- XmlSchemaSimpleContent.cs
- Profiler.cs
- IDispatchConstantAttribute.cs
- ProcessHostMapPath.cs
- AspNetRouteServiceHttpHandler.cs
- ClientData.cs
- GridItemPatternIdentifiers.cs
- WindowsListViewGroup.cs
- PasswordBox.cs
- XPathSelfQuery.cs
- ProtectedProviderSettings.cs
- shaperfactory.cs
- GestureRecognizer.cs
- SignedPkcs7.cs
- LowerCaseStringConverter.cs
- RegexTree.cs
- CodeStatementCollection.cs
- IntSecurity.cs
- ErrorHandler.cs
- DirectionalLight.cs
- LiteralControl.cs
- SQLCharsStorage.cs
- Timeline.cs
- DataGridViewAddColumnDialog.cs
- SamlAssertion.cs
- UpdateManifestForBrowserApplication.cs
- ProjectionPruner.cs
- MethodBuilderInstantiation.cs
- MsmqInputSessionChannelListener.cs
- StandardBindingReliableSessionElement.cs
- ClientApiGenerator.cs
- AmbientLight.cs
- ServiceModelConfiguration.cs
- OutOfProcStateClientManager.cs
- GcHandle.cs
- XmlSchemaAny.cs
- ApplicationInfo.cs
- TextParagraphCache.cs
- TypeDescriptionProviderAttribute.cs
- InheritablePropertyChangeInfo.cs
- HitTestWithGeometryDrawingContextWalker.cs
- Ops.cs
- DataGridViewRowCollection.cs
- SqlResolver.cs
- ScaleTransform.cs
- EntityDataSourceView.cs
- Schema.cs
- SHA384.cs
- MdiWindowListItemConverter.cs
- SqlServer2KCompatibilityCheck.cs
- TableRowCollection.cs
- OleDbDataReader.cs
- GroupBoxAutomationPeer.cs
- ScriptReferenceBase.cs
- ObjectNotFoundException.cs
- Odbc32.cs
- TogglePattern.cs
- CheckPair.cs
- SatelliteContractVersionAttribute.cs
- PositiveTimeSpanValidatorAttribute.cs
- EdmValidator.cs
- graph.cs
- ISAPIRuntime.cs
- FontStretch.cs
- DataGridViewCellPaintingEventArgs.cs
- ResXFileRef.cs
- BrushValueSerializer.cs
- X509PeerCertificateElement.cs
- EntityWrapperFactory.cs
- TextSearch.cs
- Attributes.cs
- MailBnfHelper.cs
- Window.cs
- MobileUITypeEditor.cs
- FlowLayoutSettings.cs
- ActivityValidator.cs
- MenuRenderer.cs
- WrappedOptions.cs
- PermissionToken.cs
- DbDataReader.cs
- SmiRecordBuffer.cs
- XslUrlEditor.cs
- SafeProcessHandle.cs
- VScrollProperties.cs
- ImmutableDispatchRuntime.cs
- MSHTMLHost.cs
- RawStylusSystemGestureInputReport.cs
- PeerCollaboration.cs
- HMACSHA256.cs
- DebugView.cs
- CharEnumerator.cs
- Stroke.cs
- IdentifierService.cs
- DragStartedEventArgs.cs
- XmlSchemaSet.cs
- XmlDownloadManager.cs
- GridViewRowPresenterBase.cs
- PackageStore.cs
- HeaderedItemsControl.cs