Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Channels / MsmqInputSessionChannelListener.cs / 1 / MsmqInputSessionChannelListener.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Channels { sealed class MsmqInputSessionChannelListener : MsmqChannelListenerBase{ MsmqReceiveHelper receiver; internal MsmqInputSessionChannelListener(MsmqBindingElementBase bindingElement, BindingContext context, MsmqReceiveParameters receiveParameters) : base(bindingElement, context, receiveParameters, TransportDefaults.GetDefaultMessageEncoderFactory()) { SetSecurityTokenAuthenticator(MsmqUri.NetMsmqAddressTranslator.Scheme, context); this.receiver = new MsmqReceiveHelper( this.ReceiveParameters, this.Uri, new MsmqInputMessagePool((this.ReceiveParameters as MsmqTransportReceiveParameters).MaxPoolSize), null, this ); } internal MsmqReceiveHelper MsmqReceiveHelper { get { return this.receiver; } } protected override void OnCloseCore(bool aborting) { if (this.receiver != null) { this.receiver.Close(); } } protected override void OnOpenCore(TimeSpan timeout) { base.OnOpenCore(timeout); try { this.receiver.Open(); } catch (MsmqException ex) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(ex.Normalized); } } // AcceptChannel public override IInputSessionChannel AcceptChannel() { return AcceptChannel(this.DefaultReceiveTimeout); } // public override IInputSessionChannel AcceptChannel(TimeSpan timeout) { if (DoneReceivingInCurrentState()) return null; MsmqInputMessage msmqMessage = this.receiver.TakeMessage(); try { MsmqMessageProperty property; bool retval = this.receiver.TryReceive(msmqMessage, timeout, MsmqTransactionMode.CurrentOrThrow, out property); if (retval) { if (null != property) { return MsmqDecodeHelper.DecodeTransportSessiongram(this, msmqMessage, property); } else { if (CommunicationState.Opened == this.State) { this.Fault(); } return null; } } else { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new TimeoutException()); } } catch (MsmqException ex) { if (ex.FaultReceiver) this.Fault(); throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(ex.Normalized); } finally { this.receiver.ReturnMessage(msmqMessage); } } // public override IAsyncResult BeginAcceptChannel(AsyncCallback callback, object state) { return BeginAcceptChannel(this.DefaultReceiveTimeout, callback, state); } // public override IAsyncResult BeginAcceptChannel(TimeSpan timeout, AsyncCallback callback, object state) { if (DoneReceivingInCurrentState()) return new DoneReceivingAsyncResult(callback, state); MsmqInputMessage msmqMessage = this.receiver.TakeMessage(); return this.receiver.BeginTryReceive( msmqMessage, timeout, MsmqTransactionMode.CurrentOrThrow, callback, state); } // public override IInputSessionChannel EndAcceptChannel(IAsyncResult result) { if (null == result) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("result"); DoneReceivingAsyncResult doneRecevingResult = result as DoneReceivingAsyncResult; if (doneRecevingResult != null) { DoneReceivingAsyncResult.End(doneRecevingResult); return null; } MsmqInputMessage msmqMessage = null; MsmqMessageProperty property = null; try { bool retval = this.receiver.EndTryReceive(result, out msmqMessage, out property); if (retval) { if (null != property) { return MsmqDecodeHelper.DecodeTransportSessiongram(this, msmqMessage, property); } else { if (CommunicationState.Opened == this.State) { this.Fault(); } return null; } } else { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new TimeoutException()); } } catch (MsmqException ex) { if (ex.FaultReceiver) this.Fault(); throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(ex.Normalized); } finally { if (null != msmqMessage) this.receiver.ReturnMessage(msmqMessage); } } // WaitForChannel protected override bool OnWaitForChannel(TimeSpan timeout) { if (DoneReceivingInCurrentState()) return true; try { return this.receiver.WaitForMessage(timeout); } catch (MsmqException ex) { if (ex.FaultReceiver) this.Fault(); throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(ex.Normalized); } } // protected override IAsyncResult OnBeginWaitForChannel(TimeSpan timeout, AsyncCallback callback, object state) { if (DoneReceivingInCurrentState()) return new DoneAsyncResult(true, callback, state); return this.receiver.BeginWaitForMessage(timeout, callback, state); } // protected override bool OnEndWaitForChannel(IAsyncResult result) { if (null == result) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("result"); DoneAsyncResult doneAsyncResult = result as DoneAsyncResult; if (doneAsyncResult != null) return DoneAsyncResult.End(result); else { try { return this.receiver.EndWaitForMessage(result); } catch (MsmqException ex) { if (ex.FaultReceiver) this.Fault(); throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(ex.Normalized); } } } // class DoneAsyncResult : TypedCompletedAsyncResult { internal DoneAsyncResult(bool data, AsyncCallback callback, object state) : base(data, callback, state) {} } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
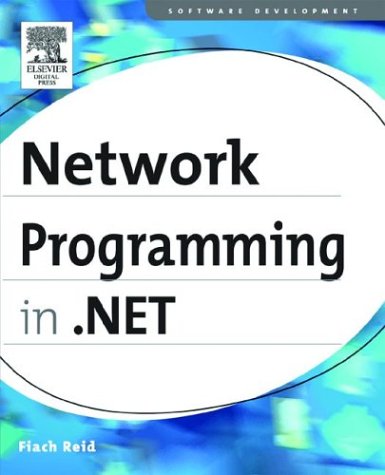
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SmiEventSink_Default.cs
- GlobalProxySelection.cs
- InlineObject.cs
- SystemMulticastIPAddressInformation.cs
- DesignerAdapterUtil.cs
- ByeMessageCD1.cs
- Variable.cs
- BindingUtils.cs
- BindToObject.cs
- CheckPair.cs
- IsolatedStorageFilePermission.cs
- PenContext.cs
- CallSiteOps.cs
- PixelFormats.cs
- HttpHostedTransportConfiguration.cs
- LineGeometry.cs
- KeyboardDevice.cs
- Form.cs
- TreeWalker.cs
- XmlBaseWriter.cs
- TextPattern.cs
- PictureBox.cs
- BinaryReader.cs
- URLIdentityPermission.cs
- HttpClientCertificate.cs
- ProgressBarBrushConverter.cs
- ScriptControlManager.cs
- Clipboard.cs
- XmlSchemaAny.cs
- BindingNavigator.cs
- DiscoveryServerProtocol.cs
- ServerValidateEventArgs.cs
- SubqueryRules.cs
- CloseCollectionAsyncResult.cs
- ToolStripSettings.cs
- TraceHandler.cs
- Page.cs
- Accessible.cs
- SqlRemoveConstantOrderBy.cs
- SessionStateModule.cs
- cryptoapiTransform.cs
- CrossAppDomainChannel.cs
- ConfigXmlText.cs
- ProfileInfo.cs
- XmlQualifiedNameTest.cs
- TableLayoutCellPaintEventArgs.cs
- PageBuildProvider.cs
- GPPOINTF.cs
- ArrangedElement.cs
- HandlerBase.cs
- WorkflowPersistenceService.cs
- ProfileGroupSettingsCollection.cs
- XmlCountingReader.cs
- IisTraceWebEventProvider.cs
- FolderNameEditor.cs
- TailCallAnalyzer.cs
- LiteralControl.cs
- WindowsFormsSynchronizationContext.cs
- ReachVisualSerializer.cs
- PolyBezierSegment.cs
- Decoder.cs
- AutomationPeer.cs
- EventProxy.cs
- PrintPreviewDialog.cs
- ConnectionStringsExpressionBuilder.cs
- GPPOINTF.cs
- CancellationToken.cs
- TimeZone.cs
- AsmxEndpointPickerExtension.cs
- ButtonBaseAutomationPeer.cs
- Transform3DGroup.cs
- AuthorizationRule.cs
- BindingList.cs
- AsymmetricKeyExchangeDeformatter.cs
- ToolboxItemCollection.cs
- IteratorFilter.cs
- XmlCharCheckingWriter.cs
- Scanner.cs
- sqlinternaltransaction.cs
- WizardPanel.cs
- ObjectDataSourceChooseMethodsPanel.cs
- CodeBinaryOperatorExpression.cs
- ElasticEase.cs
- ImageAttributes.cs
- PrinterUnitConvert.cs
- MeasurementDCInfo.cs
- ProxyBuilder.cs
- CombinedGeometry.cs
- DataContractSet.cs
- Identity.cs
- RoleGroupCollection.cs
- SignerInfo.cs
- OracleColumn.cs
- DbConnectionInternal.cs
- KeyboardInputProviderAcquireFocusEventArgs.cs
- PackageDigitalSignature.cs
- Brush.cs
- GenericsInstances.cs
- HeaderLabel.cs
- DesignerDataStoredProcedure.cs