Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / XmlUtils / System / Xml / Xsl / XmlQualifiedNameTest.cs / 1 / XmlQualifiedNameTest.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Xml; using System.Xml.Schema; using System.Collections.Generic; using System.Diagnostics; using System.Text; namespace System.Xml.Xsl { ////// XmlQualifiedName extends XmlQualifiedName to support wildcards and adds nametest functionality /// Following are the examples: /// {A}:B XmlQualifiedNameTest.New("B", "A") Match QName with namespace A and local name B /// * XmlQualifiedNameTest.New(null, null) Match any QName /// {A}:* XmlQualifiedNameTest.New(null, "A") Match QName with namespace A and any local name /// XmlQualifiedNameTest.New("A", false) /// *:B XmlQualifiedNameTest.New("B", null) Match QName with any namespace and local name B /// ~{A}:* XmlQualifiedNameTest.New("B", "A") Match QName with namespace not A and any local name /// {~A}:B only as a result of the intersection Match QName with namespace not A and local name B /// internal class XmlQualifiedNameTest : XmlQualifiedName { bool exclude; const string wildcard = "*"; static XmlQualifiedNameTest wc = XmlQualifiedNameTest.New(wildcard, wildcard); ////// Full wildcard /// public static XmlQualifiedNameTest Wildcard { get { return wc; } } ////// Constructor /// private XmlQualifiedNameTest(string name, string ns, bool exclude) : base (name, ns) { this.exclude = exclude; } ////// Construct new from name and namespace. Returns singleton Wildcard in case full wildcard /// public static XmlQualifiedNameTest New(string name, string ns) { if (ns == null && name == null) { return Wildcard; } else { return new XmlQualifiedNameTest(name == null ? wildcard : name, ns == null ? wildcard : ns, false); } } ////// True if matches any name and any namespace /// public bool IsWildcard { get { return (object)this == (object)Wildcard; } } ////// True if matches any name /// public bool IsNameWildcard { get { return (object)this.Name == (object)wildcard; } } ////// True if matches any namespace /// public bool IsNamespaceWildcard { get { return (object)this.Namespace == (object)wildcard; } } private bool IsNameSubsetOf(XmlQualifiedNameTest other) { return other.IsNameWildcard || this.Name == other.Name; } // private bool IsNamespaceSubsetOf(XmlQualifiedNameTest other) { return other.IsNamespaceWildcard || (this.exclude == other.exclude && this.Namespace == other.Namespace) || (other.exclude && !this.exclude && this.Namespace != other.Namespace); } ////// True if this matches every QName other does /// public bool IsSubsetOf(XmlQualifiedNameTest other) { return IsNameSubsetOf(other) && IsNamespaceSubsetOf(other); } ////// Return true if the result of intersection with other is not empty /// public bool HasIntersection(XmlQualifiedNameTest other) { return (IsNamespaceSubsetOf(other) || other.IsNamespaceSubsetOf(this)) && (IsNameSubsetOf(other) || other.IsNameSubsetOf(this)); } ////// String representation /// public override string ToString() { if ((object)this == (object)Wildcard) { return "*"; } else { if (this.Namespace.Length == 0) { return this.Name; } else if ((object)this.Namespace == (object)wildcard) { return "*:" + this.Name; } else if (this.exclude) { return "{~" + this.Namespace + "}:" + this.Name; } else { return "{" + this.Namespace + "}:" + this.Name; } } } #if SchemaTypeImport ////// Construct new from XmlQualifiedName. Returns singleton Wildcard in case full wildcard /// public static XmlQualifiedNameTest New(XmlQualifiedName name) { if (name.IsEmpty) { return Wildcard; } else { return new XmlQualifiedNameTest(name.Name, name.Namespace, false); } } ////// Construct new "exclusion" name test /// public static XmlQualifiedNameTest New(string ns, bool exclude) { Debug.Assert(ns != null); return new XmlQualifiedNameTest(wildcard, ns, exclude); } ////// Return the result of intersection with other /// public XmlQualifiedNameTest Intersect(XmlQualifiedNameTest other) { // Namespace // this\other ~y * y // ~x x=y ? this|other : null this x!=y ? other : null // * other this|other other // x x!=y ? this : null this x=y ? this|other : null XmlQualifiedNameTest namespaceFrom = IsNamespaceSubsetOf(other) ? this : other.IsNamespaceSubsetOf(this) ? other : null; XmlQualifiedNameTest nameFrom = IsNameSubsetOf(other) ? this : other.IsNameSubsetOf(this) ? other : null; if ((object)namespaceFrom == (object)nameFrom) { return namespaceFrom; } else if (namespaceFrom == null || nameFrom == null) { return null; } else { return new XmlQualifiedNameTest(nameFrom.Name, namespaceFrom.Namespace, namespaceFrom.ExcludeNamespace); } } ////// True if neither name nor namespace is a wildcard /// public bool IsSingleName { get { return (object)this.Name != (object)wildcard && (object)this.Namespace != (object)wildcard && this.exclude == false; } } ////// True if matches any namespace other then this.Namespace /// public bool ExcludeNamespace { get { return this.exclude; } } #endif } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Xml; using System.Xml.Schema; using System.Collections.Generic; using System.Diagnostics; using System.Text; namespace System.Xml.Xsl { ////// XmlQualifiedName extends XmlQualifiedName to support wildcards and adds nametest functionality /// Following are the examples: /// {A}:B XmlQualifiedNameTest.New("B", "A") Match QName with namespace A and local name B /// * XmlQualifiedNameTest.New(null, null) Match any QName /// {A}:* XmlQualifiedNameTest.New(null, "A") Match QName with namespace A and any local name /// XmlQualifiedNameTest.New("A", false) /// *:B XmlQualifiedNameTest.New("B", null) Match QName with any namespace and local name B /// ~{A}:* XmlQualifiedNameTest.New("B", "A") Match QName with namespace not A and any local name /// {~A}:B only as a result of the intersection Match QName with namespace not A and local name B /// internal class XmlQualifiedNameTest : XmlQualifiedName { bool exclude; const string wildcard = "*"; static XmlQualifiedNameTest wc = XmlQualifiedNameTest.New(wildcard, wildcard); ////// Full wildcard /// public static XmlQualifiedNameTest Wildcard { get { return wc; } } ////// Constructor /// private XmlQualifiedNameTest(string name, string ns, bool exclude) : base (name, ns) { this.exclude = exclude; } ////// Construct new from name and namespace. Returns singleton Wildcard in case full wildcard /// public static XmlQualifiedNameTest New(string name, string ns) { if (ns == null && name == null) { return Wildcard; } else { return new XmlQualifiedNameTest(name == null ? wildcard : name, ns == null ? wildcard : ns, false); } } ////// True if matches any name and any namespace /// public bool IsWildcard { get { return (object)this == (object)Wildcard; } } ////// True if matches any name /// public bool IsNameWildcard { get { return (object)this.Name == (object)wildcard; } } ////// True if matches any namespace /// public bool IsNamespaceWildcard { get { return (object)this.Namespace == (object)wildcard; } } private bool IsNameSubsetOf(XmlQualifiedNameTest other) { return other.IsNameWildcard || this.Name == other.Name; } // private bool IsNamespaceSubsetOf(XmlQualifiedNameTest other) { return other.IsNamespaceWildcard || (this.exclude == other.exclude && this.Namespace == other.Namespace) || (other.exclude && !this.exclude && this.Namespace != other.Namespace); } ////// True if this matches every QName other does /// public bool IsSubsetOf(XmlQualifiedNameTest other) { return IsNameSubsetOf(other) && IsNamespaceSubsetOf(other); } ////// Return true if the result of intersection with other is not empty /// public bool HasIntersection(XmlQualifiedNameTest other) { return (IsNamespaceSubsetOf(other) || other.IsNamespaceSubsetOf(this)) && (IsNameSubsetOf(other) || other.IsNameSubsetOf(this)); } ////// String representation /// public override string ToString() { if ((object)this == (object)Wildcard) { return "*"; } else { if (this.Namespace.Length == 0) { return this.Name; } else if ((object)this.Namespace == (object)wildcard) { return "*:" + this.Name; } else if (this.exclude) { return "{~" + this.Namespace + "}:" + this.Name; } else { return "{" + this.Namespace + "}:" + this.Name; } } } #if SchemaTypeImport ////// Construct new from XmlQualifiedName. Returns singleton Wildcard in case full wildcard /// public static XmlQualifiedNameTest New(XmlQualifiedName name) { if (name.IsEmpty) { return Wildcard; } else { return new XmlQualifiedNameTest(name.Name, name.Namespace, false); } } ////// Construct new "exclusion" name test /// public static XmlQualifiedNameTest New(string ns, bool exclude) { Debug.Assert(ns != null); return new XmlQualifiedNameTest(wildcard, ns, exclude); } ////// Return the result of intersection with other /// public XmlQualifiedNameTest Intersect(XmlQualifiedNameTest other) { // Namespace // this\other ~y * y // ~x x=y ? this|other : null this x!=y ? other : null // * other this|other other // x x!=y ? this : null this x=y ? this|other : null XmlQualifiedNameTest namespaceFrom = IsNamespaceSubsetOf(other) ? this : other.IsNamespaceSubsetOf(this) ? other : null; XmlQualifiedNameTest nameFrom = IsNameSubsetOf(other) ? this : other.IsNameSubsetOf(this) ? other : null; if ((object)namespaceFrom == (object)nameFrom) { return namespaceFrom; } else if (namespaceFrom == null || nameFrom == null) { return null; } else { return new XmlQualifiedNameTest(nameFrom.Name, namespaceFrom.Namespace, namespaceFrom.ExcludeNamespace); } } ////// True if neither name nor namespace is a wildcard /// public bool IsSingleName { get { return (object)this.Name != (object)wildcard && (object)this.Namespace != (object)wildcard && this.exclude == false; } } ////// True if matches any namespace other then this.Namespace /// public bool ExcludeNamespace { get { return this.exclude; } } #endif } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
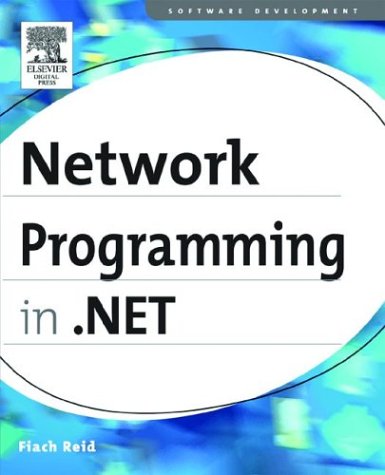
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RefExpr.cs
- MarginsConverter.cs
- StringValidator.cs
- OLEDB_Util.cs
- PropertyToken.cs
- UInt32Storage.cs
- TableAdapterManagerHelper.cs
- UIPropertyMetadata.cs
- ProxyWebPartManager.cs
- CodeBinaryOperatorExpression.cs
- EventLogger.cs
- StoreItemCollection.cs
- HeaderUtility.cs
- IntSecurity.cs
- UpdateCommand.cs
- BooleanStorage.cs
- NodeLabelEditEvent.cs
- DataBinder.cs
- InternalReceiveMessage.cs
- DataServiceClientException.cs
- EventLogException.cs
- ControlPropertyNameConverter.cs
- NumericExpr.cs
- RubberbandSelector.cs
- SoapIncludeAttribute.cs
- List.cs
- AlignmentYValidation.cs
- TreeViewCancelEvent.cs
- CodeRemoveEventStatement.cs
- ControlCachePolicy.cs
- IPAddressCollection.cs
- DataContractJsonSerializerOperationBehavior.cs
- PreviewPrintController.cs
- KeyFrames.cs
- HideDisabledControlAdapter.cs
- TemplateBuilder.cs
- DoubleIndependentAnimationStorage.cs
- BrowserDefinition.cs
- DbLambda.cs
- SchemaNotation.cs
- X509AsymmetricSecurityKey.cs
- AttachedAnnotationChangedEventArgs.cs
- AssociationSetEnd.cs
- HttpResponseInternalBase.cs
- Psha1DerivedKeyGenerator.cs
- PenThread.cs
- DrawingDrawingContext.cs
- DesignerDataParameter.cs
- ManipulationInertiaStartingEventArgs.cs
- Animatable.cs
- FormViewPagerRow.cs
- XNodeNavigator.cs
- EventLogPermission.cs
- DynamicDataExtensions.cs
- SQLSingle.cs
- CustomErrorCollection.cs
- StrongNameSignatureInformation.cs
- CodeSnippetExpression.cs
- DelegatingConfigHost.cs
- HtmlFormWrapper.cs
- MappableObjectManager.cs
- CapabilitiesSection.cs
- SqlUdtInfo.cs
- DataGridViewColumn.cs
- TextEffectCollection.cs
- WorkflowViewElement.cs
- StringUtil.cs
- TreeViewItemAutomationPeer.cs
- WorkflowServiceHost.cs
- QueryOptionExpression.cs
- Bits.cs
- DropTarget.cs
- MediaScriptCommandRoutedEventArgs.cs
- QilChoice.cs
- SafeHandle.cs
- PartialArray.cs
- DataGridViewImageCell.cs
- PolyBezierSegmentFigureLogic.cs
- SqlGenerator.cs
- AuthenticationSection.cs
- ObjectSet.cs
- DataMemberFieldEditor.cs
- FormClosedEvent.cs
- TypeElement.cs
- HttpRawResponse.cs
- CommonGetThemePartSize.cs
- ModelItemCollection.cs
- SmtpException.cs
- WinEventTracker.cs
- FacetChecker.cs
- TextFormatter.cs
- ComponentEvent.cs
- FrameworkRichTextComposition.cs
- MetadataCacheItem.cs
- Publisher.cs
- TdsValueSetter.cs
- MSAAWinEventWrap.cs
- TrackBarDesigner.cs
- CompositeControlDesigner.cs
- X509CertificateClaimSet.cs