Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Internal / SrgsCompiler / CfgSemanticTag.cs / 1 / CfgSemanticTag.cs
//---------------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // Description: // SAPI Cfg respresentation for a Semantic Tag // // History: // 5/1/2004 [....] Created from the Sapi Managed code //--------------------------------------------------------------------------- using System; using System.Globalization; using System.Speech.Internal.SrgsParser; using System.Runtime.InteropServices; namespace System.Speech.Internal.SrgsCompiler { ////// Summary description for CfgSemanticTag. /// [StructLayout (LayoutKind.Explicit)] internal struct CfgSemanticTag { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors internal CfgSemanticTag (CfgSemanticTag cfgTag) { _flag1 = cfgTag._flag1; _flag2 = cfgTag._flag2; _flag3 = cfgTag._flag3; _propId = cfgTag._propId; _nameOffset = cfgTag._nameOffset; _varInt = 0; _valueOffset = cfgTag._valueOffset; _varDouble = cfgTag._varDouble; // Initialize StartArcIndex = 0x3FFFFF; } internal CfgSemanticTag (StringBlob symbols, CfgGrammar.CfgProperty property) { //CfgGrammar.TraceInformation ("CSemanticTag::CSemanticTag"); int iWord; _flag1 = _flag2 = _flag3 = 0; _valueOffset = 0; _varInt = 0; _varDouble = 0; _propId = property._ulId; if (property._pszName != null) { _nameOffset = symbols.Add(property._pszName, out iWord); } else { _nameOffset = 0; // Offset must be zero if no string } switch (property._comType) { case 0: case VarEnum.VT_BSTR: if (property._comValue != null) { _valueOffset = symbols.Add((string)property._comValue, out iWord); } else { _valueOffset = 0; // Offset must be zero if no string } break; case VarEnum.VT_I4: _varInt = (int) property._comValue; break; case VarEnum.VT_BOOL: _varInt = (bool) property._comValue ? unchecked ((int) 0xffff) : 0; break; case VarEnum.VT_R8: _varDouble = (double) property._comValue; break; default: System.Diagnostics.Debug.Assert (false, "Unknown Semantic Tag type"); break; } PropVariantType = property._comType; ArcIndex = 0; } #endregion //******************************************************************** // // Internal Properties // //******************************************************************* #region Internal Properties internal uint StartArcIndex { get { return _flag1 & 0x3FFFFF; } set { if (value > 0x3FFFFF) { XmlParser.ThrowSrgsException (SRID.TooManyArcs); } _flag1 &= ~(uint) 0x3FFFFF; _flag1 |= value; } } internal bool StartParallelEpsilonArc { get { return ((_flag1 & 0x400000) != 0); } set { if (value) { _flag1 |= 0x400000; } else { _flag1 &= ~(uint) 0x400000; } } } internal uint EndArcIndex { get { return _flag2 & 0x3FFFFF; } set { if (value > 0x3FFFFF) { XmlParser.ThrowSrgsException (SRID.TooManyArcs); } _flag2 &= ~(uint) 0x3FFFFF; _flag2 |= value; } } internal bool EndParallelEpsilonArc { get { return ((_flag2 & 0x400000) != 0); } set { if (value) { _flag2 |= 0x400000; } else { _flag2 &= ~(uint) 0x400000; } } } internal VarEnum PropVariantType { get { return (VarEnum) (_flag3 & 0xFF); } set { uint varType = (uint) value; if (varType > 0xFF) { XmlParser.ThrowSrgsException (SRID.TooManyArcs); } _flag3 &= ~(uint) 0xFF; _flag3 |= varType; } } internal uint ArcIndex { get { return (_flag3 >> 8) & 0x3FFFFF; } set { if (value > 0x3FFFFF) { XmlParser.ThrowSrgsException (SRID.TooManyArcs); } _flag3 &= ~((uint) 0x3FFFFF << 8); _flag3 |= value << 8; } } #if (CFGDUMP || VSCOMPILE) internal string DumpFlags { get { return string.Format (CultureInfo.InvariantCulture, "flag1: {0:x} flag2: {1:x} flag3: {2:x}", _flag1, _flag2, _flag3); } } #endif #endregion //******************************************************************** // // Internal Fields // //******************************************************************** #region Internal Fields // Should be in the private section but the order for parameters is key [FieldOffset (0)] private uint _flag1; [FieldOffset (4)] private uint _flag2; [FieldOffset (8)] private uint _flag3; [FieldOffset (12)] internal int _nameOffset; [FieldOffset (16)] internal uint _propId; [FieldOffset (20)] internal int _valueOffset; [FieldOffset (24)] internal int _varInt; [FieldOffset (24)] internal double _varDouble; #endregion } [Flags] internal enum GrammarOptions { KeyValuePairs = 0, MssV1 = 1, KeyValuePairSrgs = 2, IpaPhoneme = 4, W3cV1 = 8, STG = 0x10, TagFormat = KeyValuePairs | MssV1 | W3cV1 | KeyValuePairSrgs, SemanticInterpretation = MssV1 | W3cV1 }; } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
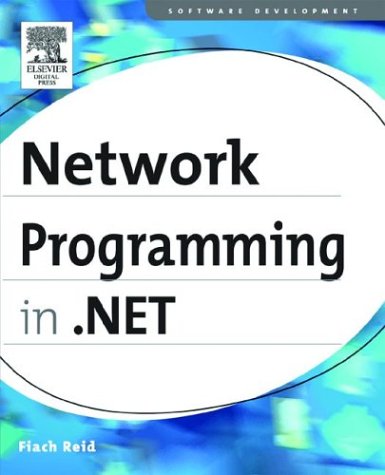
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- OdbcStatementHandle.cs
- Utils.cs
- ObjectMaterializedEventArgs.cs
- ProjectionCamera.cs
- BamlTreeMap.cs
- _Connection.cs
- AssertFilter.cs
- SetStateEventArgs.cs
- MimeObjectFactory.cs
- SectionVisual.cs
- FilteredXmlReader.cs
- TemplateEditingVerb.cs
- TemplateBaseAction.cs
- Help.cs
- BaseCAMarshaler.cs
- AppSettingsReader.cs
- KeyFrames.cs
- GatewayDefinition.cs
- RuntimeWrappedException.cs
- VideoDrawing.cs
- InvokeHandlers.cs
- HtmlInputImage.cs
- ToolStripDropTargetManager.cs
- SubMenuStyleCollection.cs
- TextTreeExtractElementUndoUnit.cs
- ColorDialog.cs
- EntityTypeBase.cs
- SourceFileBuildProvider.cs
- ThicknessAnimation.cs
- ChtmlSelectionListAdapter.cs
- GridViewColumnHeaderAutomationPeer.cs
- ProviderCommandInfoUtils.cs
- TextReader.cs
- BooleanStorage.cs
- X509SecurityToken.cs
- Size3D.cs
- ConnectionManager.cs
- GeometryGroup.cs
- UdpRetransmissionSettings.cs
- RegexWriter.cs
- PropertyPathWorker.cs
- _PooledStream.cs
- Int16Animation.cs
- NetworkInterface.cs
- DiscardableAttribute.cs
- CapabilitiesUse.cs
- DataViewSettingCollection.cs
- HtmlTitle.cs
- LicFileLicenseProvider.cs
- AsymmetricKeyExchangeFormatter.cs
- AppDomainManager.cs
- TimelineCollection.cs
- HtmlSelect.cs
- EncryptedData.cs
- InvalidComObjectException.cs
- HtmlInputRadioButton.cs
- TypeBrowser.xaml.cs
- EnumBuilder.cs
- Accessors.cs
- StandardToolWindows.cs
- ResourceContainer.cs
- BooleanConverter.cs
- BindingNavigator.cs
- ThemeInfoAttribute.cs
- GlyphRun.cs
- ScalarOps.cs
- SoapMessage.cs
- Decorator.cs
- TraceSection.cs
- ToolStripRenderer.cs
- DebugInfo.cs
- CheckBox.cs
- DbConnectionStringBuilder.cs
- BasicCellRelation.cs
- SourceInterpreter.cs
- FacetEnabledSchemaElement.cs
- OrderByLifter.cs
- ListBindingHelper.cs
- Span.cs
- ScrollPattern.cs
- ObjectContext.cs
- NullableDecimalSumAggregationOperator.cs
- StringUtil.cs
- TimerEventSubscription.cs
- TrackingLocationCollection.cs
- Control.cs
- Visual3DCollection.cs
- Journaling.cs
- BitmapEffectInputConnector.cs
- FilterElement.cs
- CacheMemory.cs
- ConfigurationStrings.cs
- AmbientValueAttribute.cs
- FilterRepeater.cs
- XmlUtil.cs
- GuidelineSet.cs
- QueryCursorEventArgs.cs
- SystemIPInterfaceStatistics.cs
- ParamArrayAttribute.cs
- TreeNodeBindingCollection.cs