Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / GeometryGroup.cs / 1305600 / GeometryGroup.cs
//---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: Implementation of GeometryGroup // // History: // 2004/11/11-Michka // Created it // //--------------------------------------------------------------------------- using System; using MS.Internal; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Reflection; using System.Collections; using System.Text; using System.Globalization; using System.Windows.Media; using System.Windows; using System.Windows.Media.Composition; using System.Text.RegularExpressions; using System.Windows.Media.Animation; using System.Windows.Markup; using System.Runtime.InteropServices; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { #region GeometryGroup ////// GeometryGroup /// [ContentProperty("Children")] public sealed partial class GeometryGroup : Geometry { #region Constructors ////// Default constructor /// public GeometryGroup() { } #endregion #region Overrides ////// GetPathGeometryData - returns a struct which contains this Geometry represented /// as a path geometry's serialized format. /// internal override PathGeometryData GetPathGeometryData() { PathGeometry pathGeometry = GetAsPathGeometry(); return pathGeometry.GetPathGeometryData(); } internal override PathGeometry GetAsPathGeometry() { PathGeometry pg = new PathGeometry(); pg.AddGeometry(this); pg.FillRule = FillRule; Debug.Assert(pg.CanFreeze); return pg; } #endregion #region GetPathFigureCollection internal override PathFigureCollection GetTransformedFigureCollection(Transform transform) { // Combine the transform argument with the internal transform Transform combined = new MatrixTransform(GetCombinedMatrix(transform)); PathFigureCollection result = new PathFigureCollection(); GeometryCollection children = Children; if (children != null) { for (int i = 0; i < children.Count; i++) { PathFigureCollection pathFigures = children.Internal_GetItem(i).GetTransformedFigureCollection(combined); if (pathFigures != null) { int count = pathFigures.Count; for (int j = 0; j < count; ++j) { result.Add(pathFigures[j]); } } } } return result; } #endregion #region IsEmpty ////// Returns true if this geometry is empty /// public override bool IsEmpty() { GeometryCollection children = Children; if (children == null) { return true; } for (int i=0; i/// Returns true if this geometry may have curved segments /// public override bool MayHaveCurves() { GeometryCollection children = Children; if (children == null) { return false; } for (int i = 0; i < children.Count; i++) { if (((Geometry)children[i]).MayHaveCurves()) { return true; } } return false; } } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
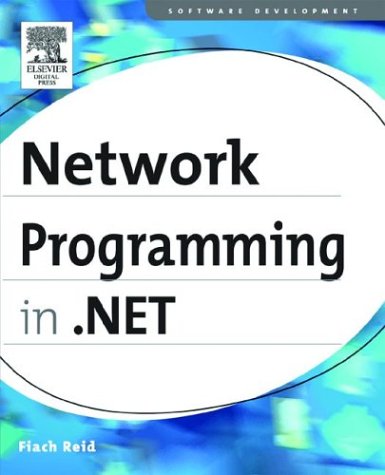
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EventLogPermissionHolder.cs
- RequiredFieldValidator.cs
- Image.cs
- HtmlControlPersistable.cs
- MultipartContentParser.cs
- DataGridViewCellParsingEventArgs.cs
- PropertyPathConverter.cs
- DynamicQueryableWrapper.cs
- PropertyTabChangedEvent.cs
- Exception.cs
- DiscardableAttribute.cs
- GCHandleCookieTable.cs
- Token.cs
- PathSegmentCollection.cs
- FieldNameLookup.cs
- TemplateBamlTreeBuilder.cs
- MetabaseServerConfig.cs
- UnknownBitmapEncoder.cs
- DataDocumentXPathNavigator.cs
- ToolStripDropDownItem.cs
- WsdlImporterElementCollection.cs
- mansign.cs
- ParentQuery.cs
- ThousandthOfEmRealDoubles.cs
- SRGSCompiler.cs
- DataServiceSaveChangesEventArgs.cs
- ColorConvertedBitmap.cs
- AuthenticationException.cs
- EventPropertyMap.cs
- Vector3D.cs
- GeneratedCodeAttribute.cs
- HelpInfo.cs
- ResourcesChangeInfo.cs
- SafeRightsManagementPubHandle.cs
- NonVisualControlAttribute.cs
- MsmqIntegrationBindingCollectionElement.cs
- DataGridViewColumnCollectionDialog.cs
- MenuEventArgs.cs
- PageCodeDomTreeGenerator.cs
- SortableBindingList.cs
- CellTreeNodeVisitors.cs
- MultiAsyncResult.cs
- DesignerTransactionCloseEvent.cs
- DivideByZeroException.cs
- SqlUtils.cs
- SwitchElementsCollection.cs
- MaterialGroup.cs
- PathSegment.cs
- ReadOnlyHierarchicalDataSourceView.cs
- Popup.cs
- UpdatePanelControlTrigger.cs
- BinaryConverter.cs
- ProtocolProfile.cs
- ObjectManager.cs
- ReliabilityContractAttribute.cs
- EventLogPropertySelector.cs
- SpecularMaterial.cs
- CompleteWizardStep.cs
- FieldBuilder.cs
- AllMembershipCondition.cs
- WCFModelStrings.Designer.cs
- ListViewInsertedEventArgs.cs
- AnnotationResourceChangedEventArgs.cs
- CodeMemberEvent.cs
- TemplateInstanceAttribute.cs
- PerformanceCounter.cs
- AggregationMinMaxHelpers.cs
- DocumentsTrace.cs
- ManagementClass.cs
- CodeDOMProvider.cs
- TypeNameConverter.cs
- FilteredAttributeCollection.cs
- TextTreeUndoUnit.cs
- SafeEventLogReadHandle.cs
- FormViewPagerRow.cs
- DataGridViewDataErrorEventArgs.cs
- ActivityCodeDomSerializer.cs
- StructuralType.cs
- StorageSetMapping.cs
- WorkflowTransactionOptions.cs
- HyperLink.cs
- DoubleLink.cs
- TransformerTypeCollection.cs
- AccessDataSourceView.cs
- BitmapEncoder.cs
- OdbcCommand.cs
- _SecureChannel.cs
- TreeViewAutomationPeer.cs
- ClientApiGenerator.cs
- XmlMapping.cs
- RepeaterCommandEventArgs.cs
- InkCanvas.cs
- CharUnicodeInfo.cs
- SelectedGridItemChangedEvent.cs
- RtfControls.cs
- ObjectReaderCompiler.cs
- InfoCardArgumentException.cs
- UriTemplateLiteralQueryValue.cs
- SystemException.cs
- OleDbConnectionInternal.cs