Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataEntity / System / Data / Metadata / Edm / StructuralType.cs / 3 / StructuralType.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.Data.Common; using System.Text; namespace System.Data.Metadata.Edm { ////// Represents the Structural Type /// public abstract class StructuralType : EdmType { #region Constructors ////// Internal parameterless constructor for bootstrapping edmtypes /// internal StructuralType() { _members = new MemberCollection(this); _readOnlyMembers = _members.AsReadOnlyMetadataCollection(); } ////// Initializes a new instance of Structural Type with the given members /// /// name of the structural type /// namespace of the structural type /// version of the structural type /// dataSpace in which this edmtype belongs to ///Thrown if either name, namespace or version arguments are null internal StructuralType(string name, string namespaceName, DataSpace dataSpace) : base(name, namespaceName, dataSpace) { _members = new MemberCollection(this); _readOnlyMembers = _members.AsReadOnlyMetadataCollection(); } #endregion #region Fields private readonly MemberCollection _members; private readonly ReadOnlyMetadataCollection_readOnlyMembers; #endregion #region Properties /// /// Returns the collection of members. /// [MetadataProperty(BuiltInTypeKind.EdmMember, true)] public ReadOnlyMetadataCollectionMembers { get { return _readOnlyMembers; } } #endregion #region Methods /// /// Get the declared only members of a particular type /// internal ReadOnlyMetadataCollectionGetDeclaredOnlyMembers () where T : EdmMember { return _members.GetDeclaredOnlyMembers (); } /// /// Validates the types and sets the readOnly property to true. Once the type is set to readOnly, /// it can never be changed. /// internal override void SetReadOnly() { if (!IsReadOnly) { base.SetReadOnly(); this.Members.Source.SetReadOnly(); } } ////// Validates a EdmMember object to determine if it can be added to this type's /// Members collection. If this method returns without throwing, it is assumed /// the member is valid. /// /// The member to validate internal abstract void ValidateMemberForAdd(EdmMember member); ////// Adds a member to this type /// /// The member to add internal void AddMember(EdmMember member) { EntityUtil.GenericCheckArgumentNull(member, "member"); Util.ThrowIfReadOnly(this); Debug.Assert(this.DataSpace == member.TypeUsage.EdmType.DataSpace || this.BuiltInTypeKind == BuiltInTypeKind.RowType, "Wrong member type getting added in structural type"); //Since we set the DataSpace of the RowType to be -1 in the constructor, we need to initialize it //as and when we add members to it if (BuiltInTypeKind.RowType == this.BuiltInTypeKind) { // Do this only when you are adding the first member if (_members.Count == 0) { this.DataSpace = member.TypeUsage.EdmType.DataSpace; } // We need to build types that span across more than one space. For such row types, we set the // DataSpace to -1 else if (this.DataSpace != (DataSpace)(-1) && member.TypeUsage.EdmType.DataSpace != this.DataSpace) { this.DataSpace = (DataSpace)(-1); } } this._members.Add(member); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.Data.Common; using System.Text; namespace System.Data.Metadata.Edm { ////// Represents the Structural Type /// public abstract class StructuralType : EdmType { #region Constructors ////// Internal parameterless constructor for bootstrapping edmtypes /// internal StructuralType() { _members = new MemberCollection(this); _readOnlyMembers = _members.AsReadOnlyMetadataCollection(); } ////// Initializes a new instance of Structural Type with the given members /// /// name of the structural type /// namespace of the structural type /// version of the structural type /// dataSpace in which this edmtype belongs to ///Thrown if either name, namespace or version arguments are null internal StructuralType(string name, string namespaceName, DataSpace dataSpace) : base(name, namespaceName, dataSpace) { _members = new MemberCollection(this); _readOnlyMembers = _members.AsReadOnlyMetadataCollection(); } #endregion #region Fields private readonly MemberCollection _members; private readonly ReadOnlyMetadataCollection_readOnlyMembers; #endregion #region Properties /// /// Returns the collection of members. /// [MetadataProperty(BuiltInTypeKind.EdmMember, true)] public ReadOnlyMetadataCollectionMembers { get { return _readOnlyMembers; } } #endregion #region Methods /// /// Get the declared only members of a particular type /// internal ReadOnlyMetadataCollectionGetDeclaredOnlyMembers () where T : EdmMember { return _members.GetDeclaredOnlyMembers (); } /// /// Validates the types and sets the readOnly property to true. Once the type is set to readOnly, /// it can never be changed. /// internal override void SetReadOnly() { if (!IsReadOnly) { base.SetReadOnly(); this.Members.Source.SetReadOnly(); } } ////// Validates a EdmMember object to determine if it can be added to this type's /// Members collection. If this method returns without throwing, it is assumed /// the member is valid. /// /// The member to validate internal abstract void ValidateMemberForAdd(EdmMember member); ////// Adds a member to this type /// /// The member to add internal void AddMember(EdmMember member) { EntityUtil.GenericCheckArgumentNull(member, "member"); Util.ThrowIfReadOnly(this); Debug.Assert(this.DataSpace == member.TypeUsage.EdmType.DataSpace || this.BuiltInTypeKind == BuiltInTypeKind.RowType, "Wrong member type getting added in structural type"); //Since we set the DataSpace of the RowType to be -1 in the constructor, we need to initialize it //as and when we add members to it if (BuiltInTypeKind.RowType == this.BuiltInTypeKind) { // Do this only when you are adding the first member if (_members.Count == 0) { this.DataSpace = member.TypeUsage.EdmType.DataSpace; } // We need to build types that span across more than one space. For such row types, we set the // DataSpace to -1 else if (this.DataSpace != (DataSpace)(-1) && member.TypeUsage.EdmType.DataSpace != this.DataSpace) { this.DataSpace = (DataSpace)(-1); } } this._members.Add(member); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
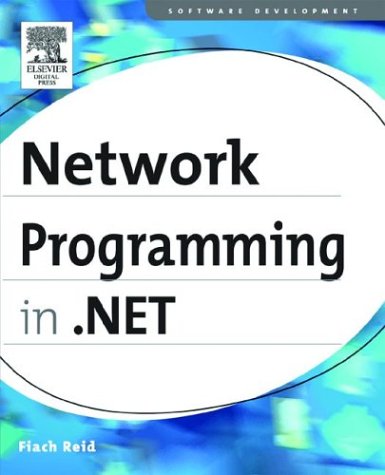
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UrlRoutingHandler.cs
- DataReaderContainer.cs
- EventMap.cs
- DetailsViewInsertedEventArgs.cs
- XsltException.cs
- ISCIIEncoding.cs
- WebEventCodes.cs
- WindowVisualStateTracker.cs
- Config.cs
- Pair.cs
- EntityProviderFactory.cs
- ValueSerializerAttribute.cs
- DataGridAddNewRow.cs
- safelinkcollection.cs
- NumericPagerField.cs
- PostBackOptions.cs
- fixedPageContentExtractor.cs
- BinaryObjectReader.cs
- SortedSetDebugView.cs
- SqlServer2KCompatibilityAnnotation.cs
- ExpressionTextBox.xaml.cs
- SingleStorage.cs
- RectAnimationClockResource.cs
- StateWorkerRequest.cs
- PackageStore.cs
- OutputCacheProfile.cs
- BindingWorker.cs
- InternalBufferOverflowException.cs
- TextViewElement.cs
- DataSourceView.cs
- PreloadedPackages.cs
- RemoteWebConfigurationHostServer.cs
- PrincipalPermission.cs
- TraceLevelStore.cs
- _OSSOCK.cs
- LinqExpressionNormalizer.cs
- RealProxy.cs
- Win32Native.cs
- Selector.cs
- wgx_sdk_version.cs
- RuntimeHelpers.cs
- DateTimeFormatInfo.cs
- XmlQualifiedNameTest.cs
- SqlDataSourceEnumerator.cs
- AnchoredBlock.cs
- UriSection.cs
- ServiceObjectContainer.cs
- SamlConditions.cs
- TraceShell.cs
- ColorPalette.cs
- List.cs
- WebPartMenu.cs
- PenLineCapValidation.cs
- GregorianCalendar.cs
- OdbcPermission.cs
- FrameworkContentElement.cs
- PathFigureCollection.cs
- __Error.cs
- CreateUserWizardStep.cs
- ErrorTableItemStyle.cs
- NameSpaceExtractor.cs
- UndoUnit.cs
- MetadataHelper.cs
- DesignerDataSchemaClass.cs
- GridViewSelectEventArgs.cs
- Lookup.cs
- SmiEventSink_DeferedProcessing.cs
- ResourceDescriptionAttribute.cs
- ConnectionPoint.cs
- TextPattern.cs
- BitmapEffectRenderDataResource.cs
- InputScopeConverter.cs
- HwndHost.cs
- SymbolType.cs
- ScrollProperties.cs
- XPathChildIterator.cs
- MaskInputRejectedEventArgs.cs
- DesigntimeLicenseContext.cs
- XhtmlTextWriter.cs
- VectorCollection.cs
- TextViewSelectionProcessor.cs
- ObjectManager.cs
- SingleTagSectionHandler.cs
- SqlUtil.cs
- SiteIdentityPermission.cs
- ChangeNode.cs
- ServiceNameElementCollection.cs
- ProgressBarHighlightConverter.cs
- ListViewSortEventArgs.cs
- ExceptionRoutedEventArgs.cs
- TagPrefixAttribute.cs
- XmlElement.cs
- ResourceDefaultValueAttribute.cs
- WpfMemberInvoker.cs
- SessionStateItemCollection.cs
- GroupBox.cs
- WizardPanelChangingEventArgs.cs
- SR.cs
- TransactionProtocol.cs
- GenericEnumerator.cs