Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Automation / Peers / GridViewColumnHeaderAutomationPeer.cs / 1305600 / GridViewColumnHeaderAutomationPeer.cs
using System; using System.Collections; using System.Collections.Generic; using System.Runtime.InteropServices; using System.Security; using System.Text; using System.Windows; using System.Windows.Automation.Provider; using System.Windows.Controls; using System.Windows.Controls.Primitives; using System.Windows.Interop; using System.Windows.Media; using MS.Internal; using MS.Win32; namespace System.Windows.Automation.Peers { /// public class GridViewColumnHeaderAutomationPeer : FrameworkElementAutomationPeer, IInvokeProvider, ITransformProvider { /// public GridViewColumnHeaderAutomationPeer(GridViewColumnHeader owner) : base(owner) { } /// override protected AutomationControlType GetAutomationControlTypeCore() { return AutomationControlType.HeaderItem; } /// override protected string GetClassNameCore() { return "GridViewColumnHeader"; } /// override public object GetPattern(PatternInterface patternInterface) { if (patternInterface == PatternInterface.Invoke || patternInterface == PatternInterface.Transform) { return this; } else { return base.GetPattern(patternInterface); } } void IInvokeProvider.Invoke() { if (!IsEnabled()) throw new ElementNotEnabledException(); GridViewColumnHeader owner = (GridViewColumnHeader)Owner; owner.AutomationClick(); } #region ITransformProvider bool ITransformProvider.CanMove { get { return false; } } //Note: CanResize can be false if Max/MinWidth,Height has been added on GridViewColumn/ColumnHeader bool ITransformProvider.CanResize { get { return true; } } bool ITransformProvider.CanRotate { get { return false; } } //Note: Don't support Move so far, if users do need this feature to reorder columns, //we can consider to add it later. (One concern is GVCH doesn't support reorder by moving itself) void ITransformProvider.Move(double x, double y) { throw new InvalidOperationException(SR.Get(SRID.UIA_OperationCannotBePerformed)); } void ITransformProvider.Resize(double width, double height) { if (!IsEnabled()) throw new ElementNotEnabledException(); if (width < 0) { throw new ArgumentOutOfRangeException("width"); } if (height < 0) { throw new ArgumentOutOfRangeException("height"); } GridViewColumnHeader header = Owner as GridViewColumnHeader; if (header != null) { if (header.Column != null) { header.Column.Width = width; } header.Height = height; } } void ITransformProvider.Rotate(double degrees) { throw new InvalidOperationException(SR.Get(SRID.UIA_OperationCannotBePerformed)); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections; using System.Collections.Generic; using System.Runtime.InteropServices; using System.Security; using System.Text; using System.Windows; using System.Windows.Automation.Provider; using System.Windows.Controls; using System.Windows.Controls.Primitives; using System.Windows.Interop; using System.Windows.Media; using MS.Internal; using MS.Win32; namespace System.Windows.Automation.Peers { /// public class GridViewColumnHeaderAutomationPeer : FrameworkElementAutomationPeer, IInvokeProvider, ITransformProvider { /// public GridViewColumnHeaderAutomationPeer(GridViewColumnHeader owner) : base(owner) { } /// override protected AutomationControlType GetAutomationControlTypeCore() { return AutomationControlType.HeaderItem; } /// override protected string GetClassNameCore() { return "GridViewColumnHeader"; } /// override public object GetPattern(PatternInterface patternInterface) { if (patternInterface == PatternInterface.Invoke || patternInterface == PatternInterface.Transform) { return this; } else { return base.GetPattern(patternInterface); } } void IInvokeProvider.Invoke() { if (!IsEnabled()) throw new ElementNotEnabledException(); GridViewColumnHeader owner = (GridViewColumnHeader)Owner; owner.AutomationClick(); } #region ITransformProvider bool ITransformProvider.CanMove { get { return false; } } //Note: CanResize can be false if Max/MinWidth,Height has been added on GridViewColumn/ColumnHeader bool ITransformProvider.CanResize { get { return true; } } bool ITransformProvider.CanRotate { get { return false; } } //Note: Don't support Move so far, if users do need this feature to reorder columns, //we can consider to add it later. (One concern is GVCH doesn't support reorder by moving itself) void ITransformProvider.Move(double x, double y) { throw new InvalidOperationException(SR.Get(SRID.UIA_OperationCannotBePerformed)); } void ITransformProvider.Resize(double width, double height) { if (!IsEnabled()) throw new ElementNotEnabledException(); if (width < 0) { throw new ArgumentOutOfRangeException("width"); } if (height < 0) { throw new ArgumentOutOfRangeException("height"); } GridViewColumnHeader header = Owner as GridViewColumnHeader; if (header != null) { if (header.Column != null) { header.Column.Width = width; } header.Height = height; } } void ITransformProvider.Rotate(double degrees) { throw new InvalidOperationException(SR.Get(SRID.UIA_OperationCannotBePerformed)); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
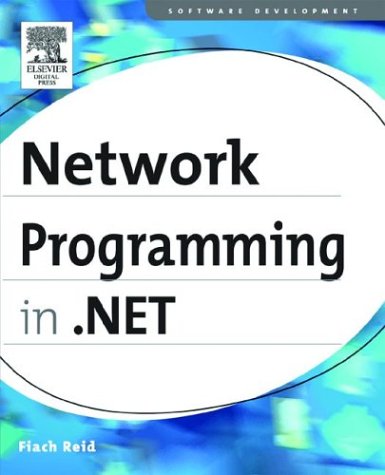
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DynamicPropertyReader.cs
- GridViewUpdatedEventArgs.cs
- FocusManager.cs
- ToolStripContainerDesigner.cs
- SvcFileManager.cs
- TypeGeneratedEventArgs.cs
- SqlClientMetaDataCollectionNames.cs
- InitializationEventAttribute.cs
- DbCommandDefinition.cs
- FacetValueContainer.cs
- PenThread.cs
- StructuredType.cs
- AutoScrollHelper.cs
- EdmToObjectNamespaceMap.cs
- WorkflowInstanceTerminatedRecord.cs
- SerializationSectionGroup.cs
- UpdateTranslator.cs
- WebBrowser.cs
- StreamGeometry.cs
- HyperlinkAutomationPeer.cs
- RadioButtonStandardAdapter.cs
- SwitchLevelAttribute.cs
- DependsOnAttribute.cs
- IdentityReference.cs
- FormClosingEvent.cs
- ITreeGenerator.cs
- remotingproxy.cs
- SecurityChannel.cs
- EventData.cs
- SocketPermission.cs
- LayeredChannelFactory.cs
- DesignerForm.cs
- SortAction.cs
- Int32Animation.cs
- DocumentOutline.cs
- PerformanceCounterManager.cs
- DataSourceBooleanViewSchemaConverter.cs
- Transform.cs
- ObjectComplexPropertyMapping.cs
- FontWeights.cs
- TypeSemantics.cs
- XmlObjectSerializerWriteContext.cs
- RootBrowserWindowProxy.cs
- CompoundFileReference.cs
- PreservationFileWriter.cs
- DataGridViewSortCompareEventArgs.cs
- PermissionRequestEvidence.cs
- XmlToDatasetMap.cs
- ObjectHandle.cs
- XmlILCommand.cs
- WindowsRichEditRange.cs
- WindowsTab.cs
- ProjectionCamera.cs
- IntranetCredentialPolicy.cs
- FontInfo.cs
- HttpWebRequest.cs
- TablePattern.cs
- BitmapEffectvisualstate.cs
- IisTraceListener.cs
- CodeParameterDeclarationExpressionCollection.cs
- ConfigurationElement.cs
- ElementNotAvailableException.cs
- BuildDependencySet.cs
- SelectionGlyphBase.cs
- TagNameToTypeMapper.cs
- Evidence.cs
- StickyNoteHelper.cs
- EventArgs.cs
- InternalControlCollection.cs
- Unit.cs
- PenThreadWorker.cs
- SemanticResultKey.cs
- ContainerParaClient.cs
- WebEventTraceProvider.cs
- InternalBase.cs
- DesignerContextDescriptor.cs
- ModuleConfigurationInfo.cs
- SecurityTokenProvider.cs
- Border.cs
- CompareValidator.cs
- EnumValidator.cs
- GlyphInfoList.cs
- XmlDataImplementation.cs
- StorageRoot.cs
- FirewallWrapper.cs
- Converter.cs
- Span.cs
- PublishLicense.cs
- WorkflowRequestContext.cs
- SqlError.cs
- SkipStoryboardToFill.cs
- DropDownButton.cs
- SecurityChannelListener.cs
- WindowsListViewScroll.cs
- MailMessage.cs
- BasicDesignerLoader.cs
- EntityKeyElement.cs
- SpeechUI.cs
- NetMsmqSecurity.cs
- QueryTask.cs