Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Channels / LayeredChannelFactory.cs / 1 / LayeredChannelFactory.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Channels { using System.Collections.Generic; using System.ServiceModel; using System.Diagnostics; using System.IO; using System.Runtime.Serialization; using System.Text; using System.Threading; abstract class LayeredChannelFactory: ChannelFactoryBase { IChannelFactory innerChannelFactory; public LayeredChannelFactory(IDefaultCommunicationTimeouts timeouts, IChannelFactory innerChannelFactory) : base(timeouts) { this.innerChannelFactory = innerChannelFactory; } protected IChannelFactory InnerChannelFactory { get { return this.innerChannelFactory; } } public override T GetProperty () { if (typeof(T) == typeof(IChannelFactory )) { return (T)(object)this; } T baseProperty = base.GetProperty (); if (baseProperty != null) { return baseProperty; } return this.innerChannelFactory.GetProperty (); } protected override IAsyncResult OnBeginOpen(TimeSpan timeout, AsyncCallback callback, object state) { return this.innerChannelFactory.BeginOpen(timeout, callback, state); } protected override void OnEndOpen(IAsyncResult result) { this.innerChannelFactory.EndOpen(result); } protected override IAsyncResult OnBeginClose(TimeSpan timeout, AsyncCallback callback, object state) { return new ChainedCloseAsyncResult(timeout, callback, state, base.OnBeginClose, base.OnEndClose, this.innerChannelFactory); } protected override void OnEndClose(IAsyncResult result) { ChainedCloseAsyncResult.End(result); } protected override void OnClose(TimeSpan timeout) { TimeoutHelper timeoutHelper = new TimeoutHelper(timeout); base.OnClose(timeoutHelper.RemainingTime()); this.innerChannelFactory.Close(timeoutHelper.RemainingTime()); } protected override void OnOpen(TimeSpan timeout) { this.innerChannelFactory.Open(timeout); } protected override void OnAbort() { base.OnAbort(); this.innerChannelFactory.Abort(); } } abstract class LayeredChannel : ChannelBase where TInnerChannel : class, IChannel { TInnerChannel innerChannel; EventHandler onInnerChannelFaulted; protected LayeredChannel(ChannelManagerBase channelManager, TInnerChannel innerChannel) : base(channelManager) { this.innerChannel = innerChannel; this.onInnerChannelFaulted = new EventHandler(OnInnerChannelFaulted); this.innerChannel.Faulted += this.onInnerChannelFaulted; } protected TInnerChannel InnerChannel { get { return this.innerChannel; } } public override T GetProperty () { T baseProperty = base.GetProperty (); if (baseProperty != null) { return baseProperty; } return this.InnerChannel.GetProperty (); } protected override void OnClosing() { this.innerChannel.Faulted -= this.onInnerChannelFaulted; base.OnClosing(); } protected override void OnAbort() { this.innerChannel.Abort(); } protected override void OnClose(TimeSpan timeout) { this.innerChannel.Close(timeout); } protected override IAsyncResult OnBeginClose(TimeSpan timeout, AsyncCallback callback, object state) { return this.innerChannel.BeginClose(timeout, callback, state); } protected override void OnEndClose(IAsyncResult result) { this.innerChannel.EndClose(result); } protected override void OnOpen(TimeSpan timeout) { this.innerChannel.Open(timeout); } protected override IAsyncResult OnBeginOpen(TimeSpan timeout, AsyncCallback callback, object state) { return this.innerChannel.BeginOpen(timeout, callback, state); } protected override void OnEndOpen(IAsyncResult result) { this.innerChannel.EndOpen(result); } void OnInnerChannelFaulted(object sender, EventArgs e) { this.Fault(); } } class LayeredInputChannel : LayeredChannel , IInputChannel { public LayeredInputChannel(ChannelManagerBase channelManager, IInputChannel innerChannel) : base(channelManager, innerChannel) { } public virtual EndpointAddress LocalAddress { get { return InnerChannel.LocalAddress; } } public Message Receive() { return InnerChannel.Receive(); } public Message Receive(TimeSpan timeout) { return InnerChannel.Receive(timeout); } public IAsyncResult BeginReceive(AsyncCallback callback, object state) { return InnerChannel.BeginReceive(callback, state); } public IAsyncResult BeginReceive(TimeSpan timeout, AsyncCallback callback, object state) { return InnerChannel.BeginReceive(timeout, callback, state); } public Message EndReceive(IAsyncResult result) { return InnerChannel.EndReceive(result); } public IAsyncResult BeginTryReceive(TimeSpan timeout, AsyncCallback callback, object state) { return InnerChannel.BeginTryReceive(timeout, callback, state); } public bool EndTryReceive(IAsyncResult result, out Message message) { return InnerChannel.EndTryReceive(result, out message); } public bool TryReceive(TimeSpan timeout, out Message message) { return InnerChannel.TryReceive(timeout, out message); } public bool WaitForMessage(TimeSpan timeout) { return InnerChannel.WaitForMessage(timeout); } public IAsyncResult BeginWaitForMessage(TimeSpan timeout, AsyncCallback callback, object state) { return InnerChannel.BeginWaitForMessage(timeout, callback, state); } public bool EndWaitForMessage(IAsyncResult result) { return InnerChannel.EndWaitForMessage(result); } } class LayeredDuplexChannel : LayeredInputChannel, IDuplexChannel { IOutputChannel innerOutputChannel; EndpointAddress localAddress; EventHandler onInnerOutputChannelFaulted; public LayeredDuplexChannel(ChannelManagerBase channelManager, IInputChannel innerInputChannel, EndpointAddress localAddress, IOutputChannel innerOutputChannel) : base(channelManager, innerInputChannel) { this.localAddress = localAddress; this.innerOutputChannel = innerOutputChannel; this.onInnerOutputChannelFaulted = new EventHandler(OnInnerOutputChannelFaulted); this.innerOutputChannel.Faulted += this.onInnerOutputChannelFaulted; } public override EndpointAddress LocalAddress { get { return this.localAddress; } } public EndpointAddress RemoteAddress { get { return this.innerOutputChannel.RemoteAddress; } } public Uri Via { get { return innerOutputChannel.Via; } } protected override void OnClosing() { this.innerOutputChannel.Faulted -= this.onInnerOutputChannelFaulted; base.OnClosing(); } protected override void OnAbort() { this.innerOutputChannel.Abort(); base.OnAbort(); } protected override IAsyncResult OnBeginClose(TimeSpan timeout, AsyncCallback callback, object state) { return new ChainedCloseAsyncResult(timeout, callback, state, base.OnBeginClose, base.OnEndClose, this.innerOutputChannel); } protected override void OnEndClose(IAsyncResult result) { ChainedCloseAsyncResult.End(result); } protected override void OnClose(TimeSpan timeout) { TimeoutHelper timeoutHelper = new TimeoutHelper(timeout); this.innerOutputChannel.Close(timeoutHelper.RemainingTime()); base.OnClose(timeoutHelper.RemainingTime()); } protected override IAsyncResult OnBeginOpen(TimeSpan timeout, AsyncCallback callback, object state) { return new ChainedOpenAsyncResult(timeout, callback, state, base.OnBeginOpen, base.OnEndOpen, this.innerOutputChannel); } protected override void OnEndOpen(IAsyncResult result) { ChainedOpenAsyncResult.End(result); } protected override void OnOpen(TimeSpan timeout) { TimeoutHelper timeoutHelper = new TimeoutHelper(timeout); base.OnOpen(timeoutHelper.RemainingTime()); innerOutputChannel.Open(timeoutHelper.RemainingTime()); } public void Send(Message message) { this.Send(message, this.DefaultSendTimeout); } public void Send(Message message, TimeSpan timeout) { this.innerOutputChannel.Send(message, timeout); } public IAsyncResult BeginSend(Message message, AsyncCallback callback, object state) { return this.BeginSend(message, this.DefaultSendTimeout, callback, state); } public IAsyncResult BeginSend(Message message, TimeSpan timeout, AsyncCallback callback, object state) { return this.innerOutputChannel.BeginSend(message, timeout, callback, state); } public void EndSend(IAsyncResult result) { this.innerOutputChannel.EndSend(result); } void OnInnerOutputChannelFaulted(object sender, EventArgs e) { this.Fault(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
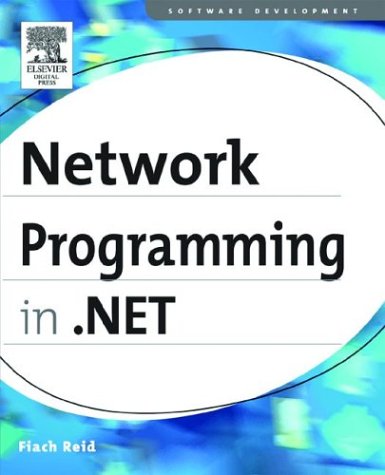
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ClientConvert.cs
- EventTrigger.cs
- ConsoleEntryPoint.cs
- QilVisitor.cs
- EventLogRecord.cs
- PKCS1MaskGenerationMethod.cs
- DesignerActionUI.cs
- EntryPointNotFoundException.cs
- CancelEventArgs.cs
- DropSource.cs
- XmlQueryRuntime.cs
- ApplicationFileCodeDomTreeGenerator.cs
- Attributes.cs
- SharedDp.cs
- ReadOnlyObservableCollection.cs
- EncoderParameter.cs
- AssemblyName.cs
- ConfigurationManagerHelperFactory.cs
- _ShellExpression.cs
- Cursors.cs
- IPGlobalProperties.cs
- ButtonField.cs
- X509SubjectKeyIdentifierClause.cs
- PriorityBindingExpression.cs
- DataGridPagingPage.cs
- ListViewHitTestInfo.cs
- DataKey.cs
- ToolStripContentPanel.cs
- BamlResourceDeserializer.cs
- DrawListViewColumnHeaderEventArgs.cs
- AdobeCFFWrapper.cs
- ApplicationContext.cs
- ListViewContainer.cs
- CodeExpressionStatement.cs
- OraclePermission.cs
- AspNetRouteServiceHttpHandler.cs
- PixelFormats.cs
- UnionCodeGroup.cs
- FormCollection.cs
- SoapCodeExporter.cs
- EntityDataSourceSelectedEventArgs.cs
- Decimal.cs
- SignatureConfirmationElement.cs
- PeerEndPoint.cs
- TextFormatter.cs
- ValidationHelper.cs
- GeneralTransform3DGroup.cs
- TextTrailingWordEllipsis.cs
- ParameterToken.cs
- XmlChildEnumerator.cs
- TextRangeSerialization.cs
- RuleAction.cs
- FontStretch.cs
- SafeHGlobalHandleCritical.cs
- ResourceReferenceKeyNotFoundException.cs
- MapPathBasedVirtualPathProvider.cs
- TreeViewItem.cs
- WebPartDisplayModeCollection.cs
- ImmutableObjectAttribute.cs
- AttachedAnnotationChangedEventArgs.cs
- Geometry.cs
- MembershipUser.cs
- RecommendedAsConfigurableAttribute.cs
- DragEventArgs.cs
- GuidelineSet.cs
- NavigationService.cs
- SchemaManager.cs
- Types.cs
- GlyphTypeface.cs
- ToolStripTemplateNode.cs
- TextBox.cs
- ReflectionServiceProvider.cs
- XmlILModule.cs
- SymbolMethod.cs
- NullableDecimalMinMaxAggregationOperator.cs
- ToolCreatedEventArgs.cs
- XmlSchemaCollection.cs
- DefaultPropertiesToSend.cs
- MouseActionValueSerializer.cs
- CodeTypeDelegate.cs
- DataMemberFieldConverter.cs
- SqlFactory.cs
- _ShellExpression.cs
- SerializationBinder.cs
- Point3DKeyFrameCollection.cs
- SqlDataSourceQueryEditor.cs
- SimplePropertyEntry.cs
- ComboBoxDesigner.cs
- DoubleCollectionValueSerializer.cs
- BatchWriter.cs
- XmlWrappingReader.cs
- SystemColorTracker.cs
- _BufferOffsetSize.cs
- EventLogPermissionEntry.cs
- CodeNamespace.cs
- mongolianshape.cs
- Processor.cs
- TypeExtension.cs
- BasicBrowserDialog.designer.cs
- XmlNamespaceManager.cs