Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / Data / System / Data / DataKey.cs / 1 / DataKey.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data { using System; using System.Diagnostics; using System.ComponentModel; internal struct DataKey { internal const Int32 COLUMN = unchecked((int)0x0000FFFF); internal const Int32 DESCENDING = unchecked((int)0x80000000); private const int maxColumns = 32; private readonly DataColumn[] columns; ////// internal DataKey(DataColumn[] columns, bool copyColumns) { if (columns == null) throw ExceptionBuilder.ArgumentNull("columns"); if (columns.Length == 0) throw ExceptionBuilder.KeyNoColumns(); if (columns.Length > maxColumns) throw ExceptionBuilder.KeyTooManyColumns(maxColumns); for (int i = 0; i < columns.Length; i++) { if (columns[i] == null) throw ExceptionBuilder.ArgumentNull("column"); } for (int i = 0; i < columns.Length; i++) { for (int j = 0; j < i; j++) { if (columns[i] == columns[j]) { throw ExceptionBuilder.KeyDuplicateColumns(columns[i].ColumnName); } } } if (copyColumns) { // Need to make a copy of all columns this.columns = new DataColumn [columns.Length]; for (int i = 0; i < columns.Length; i++) this.columns[i] = columns[i]; } else { // take ownership of the array passed in this.columns = columns; } CheckState(); } internal DataColumn[] ColumnsReference { get { return columns; } } internal bool HasValue { get { return (null != columns); } } internal DataTable Table { get { return columns[0].Table; } } internal void CheckState() { DataTable table = columns[0].Table; if (table == null) { throw ExceptionBuilder.ColumnNotInAnyTable(); } for (int i = 1; i < columns.Length; i++) { if (columns[i].Table == null) { throw ExceptionBuilder.ColumnNotInAnyTable(); } if (columns[i].Table != table) { throw ExceptionBuilder.KeyTableMismatch(); } } } internal bool ColumnsEqual(DataKey key) { //check to see if this.columns && key2's columns are equal regardless of order DataColumn[] column1=columns; DataColumn[] column2=((DataKey)key).columns; if (column1 == column2) { return true; } else if (column1 == null || column2 == null) { return false; } else if (column1.Length != column2.Length) { return false; } else { int i, j; for (i=0; i[To be supplied.] ///// Copyright (c) Microsoft Corporation. All rights reserved. // // [....] //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data { using System; using System.Diagnostics; using System.ComponentModel; internal struct DataKey { internal const Int32 COLUMN = unchecked((int)0x0000FFFF); internal const Int32 DESCENDING = unchecked((int)0x80000000); private const int maxColumns = 32; private readonly DataColumn[] columns; ////// internal DataKey(DataColumn[] columns, bool copyColumns) { if (columns == null) throw ExceptionBuilder.ArgumentNull("columns"); if (columns.Length == 0) throw ExceptionBuilder.KeyNoColumns(); if (columns.Length > maxColumns) throw ExceptionBuilder.KeyTooManyColumns(maxColumns); for (int i = 0; i < columns.Length; i++) { if (columns[i] == null) throw ExceptionBuilder.ArgumentNull("column"); } for (int i = 0; i < columns.Length; i++) { for (int j = 0; j < i; j++) { if (columns[i] == columns[j]) { throw ExceptionBuilder.KeyDuplicateColumns(columns[i].ColumnName); } } } if (copyColumns) { // Need to make a copy of all columns this.columns = new DataColumn [columns.Length]; for (int i = 0; i < columns.Length; i++) this.columns[i] = columns[i]; } else { // take ownership of the array passed in this.columns = columns; } CheckState(); } internal DataColumn[] ColumnsReference { get { return columns; } } internal bool HasValue { get { return (null != columns); } } internal DataTable Table { get { return columns[0].Table; } } internal void CheckState() { DataTable table = columns[0].Table; if (table == null) { throw ExceptionBuilder.ColumnNotInAnyTable(); } for (int i = 1; i < columns.Length; i++) { if (columns[i].Table == null) { throw ExceptionBuilder.ColumnNotInAnyTable(); } if (columns[i].Table != table) { throw ExceptionBuilder.KeyTableMismatch(); } } } internal bool ColumnsEqual(DataKey key) { //check to see if this.columns && key2's columns are equal regardless of order DataColumn[] column1=columns; DataColumn[] column2=((DataKey)key).columns; if (column1 == column2) { return true; } else if (column1 == null || column2 == null) { return false; } else if (column1.Length != column2.Length) { return false; } else { int i, j; for (i=0; i[To be supplied.] ///
Link Menu
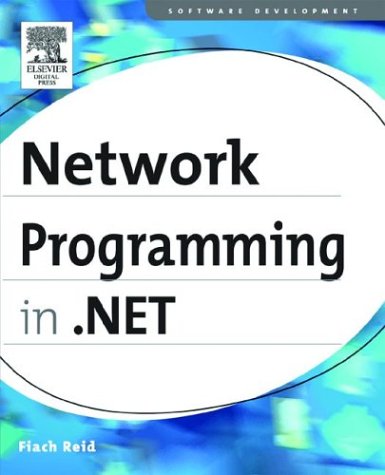
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BroadcastEventHelper.cs
- OrderPreservingSpoolingTask.cs
- DataGridViewControlCollection.cs
- BaseDataBoundControlDesigner.cs
- RequestUriProcessor.cs
- DoubleAnimationClockResource.cs
- KeyValueSerializer.cs
- BamlBinaryReader.cs
- AttachmentService.cs
- CookieProtection.cs
- QueryOperationResponseOfT.cs
- IncrementalCompileAnalyzer.cs
- RouteCollection.cs
- DelegateOutArgument.cs
- PerfProviderCollection.cs
- MeshGeometry3D.cs
- SqlNotificationEventArgs.cs
- ChannelServices.cs
- Trace.cs
- FormatSettings.cs
- DataSetUtil.cs
- CornerRadiusConverter.cs
- FileUpload.cs
- IBuiltInEvidence.cs
- RemotingConfigParser.cs
- MachineKeyConverter.cs
- GenericWebPart.cs
- CompilerError.cs
- BindableTemplateBuilder.cs
- TraceSection.cs
- FlowPosition.cs
- SqlMethodAttribute.cs
- HtmlUtf8RawTextWriter.cs
- CounterSampleCalculator.cs
- TypeLibConverter.cs
- UpdateCompiler.cs
- SectionRecord.cs
- SqlBooleanMismatchVisitor.cs
- NameValueConfigurationElement.cs
- CloudCollection.cs
- ExpressionVisitor.cs
- ICspAsymmetricAlgorithm.cs
- ToolBar.cs
- FontStyles.cs
- TextFindEngine.cs
- XmlNodeList.cs
- ErrorHandler.cs
- DecoderExceptionFallback.cs
- DesignerVerb.cs
- SoapSchemaImporter.cs
- EntityDataSourceEntitySetNameItem.cs
- SqlNodeAnnotations.cs
- TextEmbeddedObject.cs
- ThreadInterruptedException.cs
- MSG.cs
- DataViewManager.cs
- UntypedNullExpression.cs
- CodeLinePragma.cs
- CachedBitmap.cs
- cookieexception.cs
- DataListGeneralPage.cs
- ParseElementCollection.cs
- GlyphRun.cs
- SqlFunctionAttribute.cs
- FixedSOMImage.cs
- OSFeature.cs
- SupportsEventValidationAttribute.cs
- HandlerFactoryWrapper.cs
- TransformerInfoCollection.cs
- BaseParser.cs
- BitmapEffectvisualstate.cs
- CodeDelegateCreateExpression.cs
- BitmapMetadataBlob.cs
- FocusTracker.cs
- Attributes.cs
- ValidationError.cs
- DLinqColumnProvider.cs
- BamlMapTable.cs
- CounterSampleCalculator.cs
- AudioStateChangedEventArgs.cs
- QilXmlReader.cs
- UseManagedPresentationElement.cs
- Funcletizer.cs
- WindowsFormsSectionHandler.cs
- AuthenticationManager.cs
- XPathDocument.cs
- FlowLayout.cs
- Evaluator.cs
- ElementMarkupObject.cs
- XamlReaderHelper.cs
- UriTemplateVariableQueryValue.cs
- basevalidator.cs
- DockProviderWrapper.cs
- TemplatedWizardStep.cs
- DataListItemCollection.cs
- XmlDataSourceNodeDescriptor.cs
- IisNotInstalledException.cs
- DataServiceHost.cs
- SqlDependencyListener.cs
- EntityRecordInfo.cs