Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / UI / BaseParser.cs / 1 / BaseParser.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Implements the ASP.NET template parser * * Copyright (c) 1998 Microsoft Corporation */ /********************************* Class hierarchy BaseParser DependencyParser TemplateControlDependencyParser PageDependencyParser UserControlDependencyParser MasterPageDependencyParser TemplateParser BaseTemplateParser TemplateControlParser PageParser UserControlParser MasterPageParser PageThemeParser ApplicationFileParser **********************************/ namespace System.Web.UI { using System; using System.Collections; using System.Web.Hosting; using System.Web.Util; using System.Text.RegularExpressions; using System.Web.RegularExpressions; using System.Security.Permissions; // Internal interface for Parser that have exteranl assembly dependency. internal interface IAssemblyDependencyParser { ICollection AssemblyDependencies { get; } } ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class BaseParser { // The directory used for relative path calculations private VirtualPath _baseVirtualDir; internal VirtualPath BaseVirtualDir { get { return _baseVirtualDir; } } // The virtual path to the file currently being processed private VirtualPath _currentVirtualPath; internal VirtualPath CurrentVirtualPath { get { return _currentVirtualPath; } set { _currentVirtualPath = value; // Can happen in the designer if (value == null) return; _baseVirtualDir = value.Parent; } } internal string CurrentVirtualPathString { get { return System.Web.VirtualPath.GetVirtualPathString(CurrentVirtualPath); } } internal readonly static Regex tagRegex = new TagRegex(); internal readonly static Regex directiveRegex = new DirectiveRegex(); internal readonly static Regex endtagRegex = new EndTagRegex(); internal readonly static Regex aspCodeRegex = new AspCodeRegex(); internal readonly static Regex aspExprRegex = new AspExprRegex(); internal readonly static Regex databindExprRegex = new DatabindExprRegex(); internal readonly static Regex commentRegex = new CommentRegex(); internal readonly static Regex includeRegex = new IncludeRegex(); internal readonly static Regex textRegex = new TextRegex(); // Regexes used in DetectSpecialServerTagError internal readonly static Regex gtRegex = new GTRegex(); internal readonly static Regex ltRegex = new LTRegex(); internal readonly static Regex serverTagsRegex = new ServerTagsRegex(); internal readonly static Regex runatServerRegex = new RunatServerRegex(); /* * Turns relative virtual path into absolute ones */ internal VirtualPath ResolveVirtualPath(VirtualPath virtualPath) { return VirtualPathProvider.CombineVirtualPathsInternal(CurrentVirtualPath, virtualPath); } } }[To be supplied.] ///
Link Menu
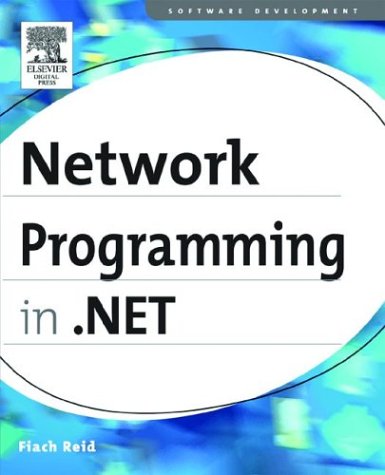
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CryptoKeySecurity.cs
- WebPartsPersonalization.cs
- OracleCommandSet.cs
- DynamicMethod.cs
- MailHeaderInfo.cs
- SrgsElement.cs
- ArgIterator.cs
- HttpGetProtocolReflector.cs
- FragmentQueryProcessor.cs
- CodeMemberEvent.cs
- EntryWrittenEventArgs.cs
- Switch.cs
- DBConnection.cs
- SymbolTable.cs
- sqlstateclientmanager.cs
- shaperfactoryquerycacheentry.cs
- StyleCollection.cs
- RequiredAttributeAttribute.cs
- ResourceDisplayNameAttribute.cs
- UserPreferenceChangingEventArgs.cs
- Application.cs
- MenuItemStyleCollection.cs
- TypeToken.cs
- StreamUpgradeBindingElement.cs
- UnmanagedHandle.cs
- UnionQueryOperator.cs
- WebPartChrome.cs
- ToolStripButton.cs
- QilFactory.cs
- CodeFieldReferenceExpression.cs
- RegexMatchCollection.cs
- SecurityDescriptor.cs
- UserPersonalizationStateInfo.cs
- LicenseException.cs
- PrivilegeNotHeldException.cs
- FocusTracker.cs
- ClipboardProcessor.cs
- UserControl.cs
- DynamicResourceExtension.cs
- SerializableAttribute.cs
- ContainsRowNumberChecker.cs
- CursorConverter.cs
- Transform.cs
- WindowsContainer.cs
- XmlHelper.cs
- RelationshipEndCollection.cs
- GlobalEventManager.cs
- CriticalExceptions.cs
- EventTask.cs
- DataColumnMappingCollection.cs
- GenericFlowSwitchHelper.cs
- ExitEventArgs.cs
- WebExceptionStatus.cs
- RectangleGeometry.cs
- Form.cs
- SimpleFileLog.cs
- CorrelationQueryBehavior.cs
- CustomLineCap.cs
- QueryTreeBuilder.cs
- SafeEventLogWriteHandle.cs
- FieldMetadata.cs
- Metadata.cs
- OperandQuery.cs
- ScriptControl.cs
- PlatformCulture.cs
- HeaderCollection.cs
- CapabilitiesState.cs
- PassportAuthentication.cs
- DbDataSourceEnumerator.cs
- TabRenderer.cs
- GradientStopCollection.cs
- Rect.cs
- Converter.cs
- IconConverter.cs
- Clipboard.cs
- EntityTemplateFactory.cs
- BlurBitmapEffect.cs
- PageFunction.cs
- CallbackBehaviorAttribute.cs
- DataTemplate.cs
- XmlILStorageConverter.cs
- TextSimpleMarkerProperties.cs
- HelpProvider.cs
- MemberProjectedSlot.cs
- Pen.cs
- SplashScreen.cs
- NeedSkipTokenVisitor.cs
- TdsParser.cs
- NavigationService.cs
- MouseGesture.cs
- TouchDevice.cs
- DataGridViewToolTip.cs
- DataGridCaption.cs
- ModifierKeysValueSerializer.cs
- RoleManagerEventArgs.cs
- MethodToken.cs
- XamlHttpHandlerFactory.cs
- LineSegment.cs
- CommandHelper.cs
- SmiEventStream.cs