Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / DEVDIV / depot / DevDiv / releases / whidbey / QFE / ndp / fx / src / xsp / System / Web / UI / WebParts / WebPartDisplayModeCollection.cs / 2 / WebPartDisplayModeCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls.WebParts { using System; using System.Collections; using System.Security.Permissions; [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class WebPartDisplayModeCollection : CollectionBase { private bool _readOnly; private string _readOnlyExceptionMessage; // Prevent instantiation outside of our assembly. We want third-part code to use the collection // returned by the base method, not create a new collection. internal WebPartDisplayModeCollection() { } public bool IsReadOnly { get { return _readOnly; } } public WebPartDisplayMode this[int index] { get { return (WebPartDisplayMode)List[index]; } } public WebPartDisplayMode this[string modeName] { get { foreach (WebPartDisplayMode displayMode in List) { if (String.Equals(displayMode.Name, modeName, StringComparison.OrdinalIgnoreCase)) { return displayMode; } } return null; } } public int Add(WebPartDisplayMode value) { return List.Add(value); } internal int AddInternal(WebPartDisplayMode value) { bool isReadOnly = _readOnly; _readOnly = false; // Extra try-catch block to prevent elevation of privilege attack via exception filter try { try { return List.Add(value); } finally { _readOnly = isReadOnly; } } catch { throw; } } private void CheckReadOnly() { if (_readOnly) { throw new InvalidOperationException(SR.GetString(_readOnlyExceptionMessage)); } } public bool Contains(WebPartDisplayMode value) { return List.Contains(value); } public void CopyTo(WebPartDisplayMode[] array, int index) { List.CopyTo(array, index); } public int IndexOf(WebPartDisplayMode value) { return List.IndexOf(value); } public void Insert(int index, WebPartDisplayMode value) { List.Insert(index, value); } protected override void OnClear() { throw new InvalidOperationException(SR.GetString(SR.WebPartDisplayModeCollection_CantRemove)); } protected override void OnInsert(int index, object value) { CheckReadOnly(); WebPartDisplayMode displayMode = (WebPartDisplayMode)value; foreach (WebPartDisplayMode existingDisplayMode in List) { if (displayMode.Name == existingDisplayMode.Name) { throw new ArgumentException(SR.GetString( SR.WebPartDisplayModeCollection_DuplicateName, displayMode.Name)); } } base.OnInsert(index, value); } protected override void OnRemove(int index, object value) { throw new InvalidOperationException(SR.GetString(SR.WebPartDisplayModeCollection_CantRemove)); } protected override void OnSet(int index, object oldValue, object newValue) { throw new InvalidOperationException(SR.GetString(SR.WebPartDisplayModeCollection_CantSet)); } protected override void OnValidate(object value) { base.OnValidate(value); if (value == null) { throw new ArgumentNullException("value", SR.GetString(SR.Collection_CantAddNull)); } if (!(value is WebPartDisplayMode)) { throw new ArgumentException(SR.GetString(SR.Collection_InvalidType, "WebPartDisplayMode"), "value"); } } internal void SetReadOnly(string exceptionMessage) { _readOnlyExceptionMessage = exceptionMessage; _readOnly = true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls.WebParts { using System; using System.Collections; using System.Security.Permissions; [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class WebPartDisplayModeCollection : CollectionBase { private bool _readOnly; private string _readOnlyExceptionMessage; // Prevent instantiation outside of our assembly. We want third-part code to use the collection // returned by the base method, not create a new collection. internal WebPartDisplayModeCollection() { } public bool IsReadOnly { get { return _readOnly; } } public WebPartDisplayMode this[int index] { get { return (WebPartDisplayMode)List[index]; } } public WebPartDisplayMode this[string modeName] { get { foreach (WebPartDisplayMode displayMode in List) { if (String.Equals(displayMode.Name, modeName, StringComparison.OrdinalIgnoreCase)) { return displayMode; } } return null; } } public int Add(WebPartDisplayMode value) { return List.Add(value); } internal int AddInternal(WebPartDisplayMode value) { bool isReadOnly = _readOnly; _readOnly = false; // Extra try-catch block to prevent elevation of privilege attack via exception filter try { try { return List.Add(value); } finally { _readOnly = isReadOnly; } } catch { throw; } } private void CheckReadOnly() { if (_readOnly) { throw new InvalidOperationException(SR.GetString(_readOnlyExceptionMessage)); } } public bool Contains(WebPartDisplayMode value) { return List.Contains(value); } public void CopyTo(WebPartDisplayMode[] array, int index) { List.CopyTo(array, index); } public int IndexOf(WebPartDisplayMode value) { return List.IndexOf(value); } public void Insert(int index, WebPartDisplayMode value) { List.Insert(index, value); } protected override void OnClear() { throw new InvalidOperationException(SR.GetString(SR.WebPartDisplayModeCollection_CantRemove)); } protected override void OnInsert(int index, object value) { CheckReadOnly(); WebPartDisplayMode displayMode = (WebPartDisplayMode)value; foreach (WebPartDisplayMode existingDisplayMode in List) { if (displayMode.Name == existingDisplayMode.Name) { throw new ArgumentException(SR.GetString( SR.WebPartDisplayModeCollection_DuplicateName, displayMode.Name)); } } base.OnInsert(index, value); } protected override void OnRemove(int index, object value) { throw new InvalidOperationException(SR.GetString(SR.WebPartDisplayModeCollection_CantRemove)); } protected override void OnSet(int index, object oldValue, object newValue) { throw new InvalidOperationException(SR.GetString(SR.WebPartDisplayModeCollection_CantSet)); } protected override void OnValidate(object value) { base.OnValidate(value); if (value == null) { throw new ArgumentNullException("value", SR.GetString(SR.Collection_CantAddNull)); } if (!(value is WebPartDisplayMode)) { throw new ArgumentException(SR.GetString(SR.Collection_InvalidType, "WebPartDisplayMode"), "value"); } } internal void SetReadOnly(string exceptionMessage) { _readOnlyExceptionMessage = exceptionMessage; _readOnly = true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
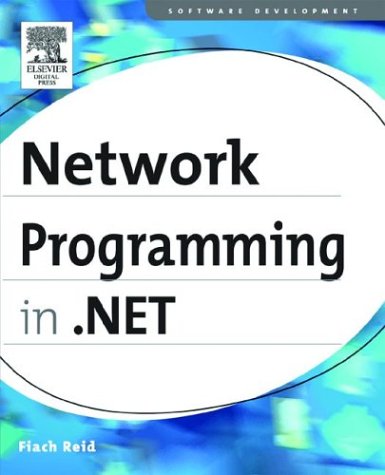
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AsymmetricAlgorithm.cs
- PartialClassGenerationTask.cs
- InputScope.cs
- ProgressBarBrushConverter.cs
- RectAnimationBase.cs
- RefreshEventArgs.cs
- SqlProfileProvider.cs
- NumberFormatInfo.cs
- InputLangChangeRequestEvent.cs
- DependencyObject.cs
- ListItemCollection.cs
- SemaphoreSecurity.cs
- UnmanagedMemoryAccessor.cs
- BooleanAnimationBase.cs
- BinHexDecoder.cs
- BamlRecordHelper.cs
- ProfileSettingsCollection.cs
- FormatterServices.cs
- TdsParserSessionPool.cs
- RawTextInputReport.cs
- DictionaryBase.cs
- _Win32.cs
- Base64Stream.cs
- NegatedCellConstant.cs
- RemotingConfiguration.cs
- URIFormatException.cs
- NumericUpDownAccelerationCollection.cs
- Trigger.cs
- ChildDocumentBlock.cs
- Label.cs
- TextSegment.cs
- WebPartConnectionsCloseVerb.cs
- DataGridViewCellConverter.cs
- ResXResourceReader.cs
- XmlNodeChangedEventManager.cs
- APCustomTypeDescriptor.cs
- MultiTrigger.cs
- TextDecorationCollectionConverter.cs
- EncoderBestFitFallback.cs
- ImageResources.Designer.cs
- SafeRightsManagementPubHandle.cs
- NotFiniteNumberException.cs
- SqlRemoveConstantOrderBy.cs
- SpeechSeg.cs
- ComboBox.cs
- ComplexLine.cs
- FlowDocumentReader.cs
- MouseCaptureWithinProperty.cs
- TextPointer.cs
- Int32RectConverter.cs
- regiisutil.cs
- StyleCollectionEditor.cs
- IisTraceWebEventProvider.cs
- RegistrationProxy.cs
- XamlNamespaceHelper.cs
- UpDownEvent.cs
- ConfigurationCollectionAttribute.cs
- TableChangeProcessor.cs
- GridViewCommandEventArgs.cs
- ContentTextAutomationPeer.cs
- XmlSchemaIdentityConstraint.cs
- CommandDevice.cs
- MemberDomainMap.cs
- AttachedPropertyMethodSelector.cs
- RangeBaseAutomationPeer.cs
- CollectionBuilder.cs
- IgnoreSection.cs
- ClientUrlResolverWrapper.cs
- DnsEndPoint.cs
- webeventbuffer.cs
- SqlDataReaderSmi.cs
- ManagedWndProcTracker.cs
- ListItem.cs
- CodeTypeReferenceCollection.cs
- FacetValues.cs
- FloatUtil.cs
- BrowserCapabilitiesFactory.cs
- ZipIOBlockManager.cs
- OpCopier.cs
- ObjectReaderCompiler.cs
- NotifyInputEventArgs.cs
- CompilationUtil.cs
- GridViewColumnHeader.cs
- WorkflowServiceHost.cs
- CodeNamespace.cs
- CodeTypeReferenceExpression.cs
- MDIWindowDialog.cs
- IsolatedStorageFile.cs
- EventListener.cs
- ITreeGenerator.cs
- Rotation3DKeyFrameCollection.cs
- ToolBarButtonDesigner.cs
- lengthconverter.cs
- ConnectivityStatus.cs
- KeyTime.cs
- _ReceiveMessageOverlappedAsyncResult.cs
- SessionEndingEventArgs.cs
- SymmetricKeyWrap.cs
- TraceSection.cs
- FormClosedEvent.cs