Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Base / MS / Internal / ComponentModel / AttachedPropertyMethodSelector.cs / 1305600 / AttachedPropertyMethodSelector.cs
namespace MS.Internal.ComponentModel { using System; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Security.Permissions; using System.Windows; ////// This is a reflection binder that is used to /// find the right method match for attached properties. /// The default binder in the CLR will not find a /// method match unless the parameters we provide are /// exact matches. This binder will use compatible type /// matching to find a match for any parameters that are /// compatible. /// internal class AttachedPropertyMethodSelector : Binder { ////// The only method we implement. Our goal here is to find a method that best matches the arguments passed. /// We are doing this only with the intent of pulling attached property metadata off of the method. /// If there are ambiguous methods, we simply take the first one as all "Get" methods for an attached /// property should have identical metadata. /// public override MethodBase SelectMethod(BindingFlags bindingAttr, MethodBase[] match, Type[] types, ParameterModifier[] modifiers) { // Short circuit for cases where someone didn't pass in a types array. if (types == null) { if (match.Length > 1) { throw new AmbiguousMatchException(); } else { return match[0]; } } for(int idx = 0; idx < match.Length; idx++) { MethodBase candidate = match[idx]; ParameterInfo[] parameters = candidate.GetParameters(); if (ParametersMatch(parameters, types)) { return candidate; } } return null; } ////// This method checks that the parameters passed in are /// compatible with the provided parameter types. /// private static bool ParametersMatch(ParameterInfo[] parameters, Type[] types) { if (parameters.Length != types.Length) { return false; } // IsAssignableFrom is not cheap. Do this in two passes. // Our first pass checks for exact type matches. Only on // the second pass do we do an IsAssignableFrom. bool compat = true; for(int idx = 0; idx < parameters.Length; idx++) { ParameterInfo p = parameters[idx]; Type t = types[idx]; if (p.ParameterType != t) { compat = false; break; } } if (compat) { return true; } // Second pass uses IsAssignableFrom to check for compatible types. compat = true; for(int idx = 0; idx < parameters.Length; idx++) { ParameterInfo p = parameters[idx]; Type t = types[idx]; if (!t.IsAssignableFrom(p.ParameterType)) { compat = false; break; } } return compat; } ////// We do not implement this. /// public override MethodBase BindToMethod(BindingFlags bindingAttr, MethodBase[] match, ref object[] args, ParameterModifier[] modifiers, CultureInfo culture, string[] names, out object state) { // We are only a method binder. throw new NotImplementedException(); } ////// We do not implement this. /// public override FieldInfo BindToField(BindingFlags bindingAttr, FieldInfo[] match, object value, CultureInfo culture) { // We are only a method binder. throw new NotImplementedException(); } ////// We do not implement this. /// public override object ChangeType(object value, Type type, CultureInfo culture) { // We are only a method binder. throw new NotImplementedException(); } ////// We do not implement this. /// public override void ReorderArgumentArray(ref object[] args, object state) { // We are only a method binder. throw new NotImplementedException(); } ////// We do not implement this. /// public override PropertyInfo SelectProperty(BindingFlags bindingAttr, PropertyInfo[] match, Type returnType, Type[] indexes, ParameterModifier[] modifiers) { // We are only a method binder. throw new NotImplementedException(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. namespace MS.Internal.ComponentModel { using System; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Security.Permissions; using System.Windows; ////// This is a reflection binder that is used to /// find the right method match for attached properties. /// The default binder in the CLR will not find a /// method match unless the parameters we provide are /// exact matches. This binder will use compatible type /// matching to find a match for any parameters that are /// compatible. /// internal class AttachedPropertyMethodSelector : Binder { ////// The only method we implement. Our goal here is to find a method that best matches the arguments passed. /// We are doing this only with the intent of pulling attached property metadata off of the method. /// If there are ambiguous methods, we simply take the first one as all "Get" methods for an attached /// property should have identical metadata. /// public override MethodBase SelectMethod(BindingFlags bindingAttr, MethodBase[] match, Type[] types, ParameterModifier[] modifiers) { // Short circuit for cases where someone didn't pass in a types array. if (types == null) { if (match.Length > 1) { throw new AmbiguousMatchException(); } else { return match[0]; } } for(int idx = 0; idx < match.Length; idx++) { MethodBase candidate = match[idx]; ParameterInfo[] parameters = candidate.GetParameters(); if (ParametersMatch(parameters, types)) { return candidate; } } return null; } ////// This method checks that the parameters passed in are /// compatible with the provided parameter types. /// private static bool ParametersMatch(ParameterInfo[] parameters, Type[] types) { if (parameters.Length != types.Length) { return false; } // IsAssignableFrom is not cheap. Do this in two passes. // Our first pass checks for exact type matches. Only on // the second pass do we do an IsAssignableFrom. bool compat = true; for(int idx = 0; idx < parameters.Length; idx++) { ParameterInfo p = parameters[idx]; Type t = types[idx]; if (p.ParameterType != t) { compat = false; break; } } if (compat) { return true; } // Second pass uses IsAssignableFrom to check for compatible types. compat = true; for(int idx = 0; idx < parameters.Length; idx++) { ParameterInfo p = parameters[idx]; Type t = types[idx]; if (!t.IsAssignableFrom(p.ParameterType)) { compat = false; break; } } return compat; } ////// We do not implement this. /// public override MethodBase BindToMethod(BindingFlags bindingAttr, MethodBase[] match, ref object[] args, ParameterModifier[] modifiers, CultureInfo culture, string[] names, out object state) { // We are only a method binder. throw new NotImplementedException(); } ////// We do not implement this. /// public override FieldInfo BindToField(BindingFlags bindingAttr, FieldInfo[] match, object value, CultureInfo culture) { // We are only a method binder. throw new NotImplementedException(); } ////// We do not implement this. /// public override object ChangeType(object value, Type type, CultureInfo culture) { // We are only a method binder. throw new NotImplementedException(); } ////// We do not implement this. /// public override void ReorderArgumentArray(ref object[] args, object state) { // We are only a method binder. throw new NotImplementedException(); } ////// We do not implement this. /// public override PropertyInfo SelectProperty(BindingFlags bindingAttr, PropertyInfo[] match, Type returnType, Type[] indexes, ParameterModifier[] modifiers) { // We are only a method binder. throw new NotImplementedException(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
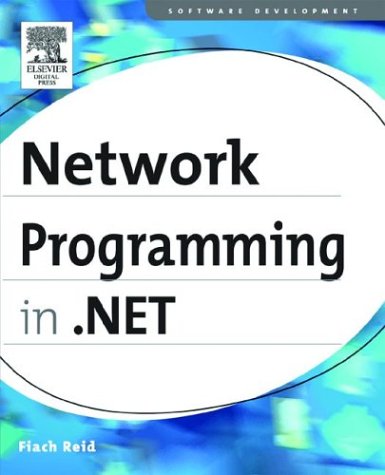
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MachineKeyConverter.cs
- XmlQueryCardinality.cs
- OracleNumber.cs
- MSHTMLHostUtil.cs
- BitmapEffectInput.cs
- MarshalByValueComponent.cs
- MultiView.cs
- XsdDuration.cs
- TableProviderWrapper.cs
- WCFModelStrings.Designer.cs
- ContentElement.cs
- CodeAttributeArgument.cs
- NameTable.cs
- XamlSerializer.cs
- XmlReflectionImporter.cs
- ProgramPublisher.cs
- Odbc32.cs
- FontCollection.cs
- Select.cs
- GeneralTransform3DGroup.cs
- HitTestDrawingContextWalker.cs
- VariableQuery.cs
- WebSysDescriptionAttribute.cs
- ConnectionStringSettingsCollection.cs
- TextServicesHost.cs
- DES.cs
- SelectedDatesCollection.cs
- MarshalDirectiveException.cs
- _NtlmClient.cs
- SqlUDTStorage.cs
- SqlDataSourceQuery.cs
- ParallelDesigner.cs
- HashHelper.cs
- PathFigureCollectionConverter.cs
- BookmarkNameHelper.cs
- WorkflowServiceOperationListItem.cs
- SqlIdentifier.cs
- DirtyTextRange.cs
- ProtocolsConfiguration.cs
- MetadataUtilsSmi.cs
- FtpWebRequest.cs
- CollectionBase.cs
- CacheChildrenQuery.cs
- DesignerPerfEventProvider.cs
- RtType.cs
- IndicCharClassifier.cs
- LogStore.cs
- PointAnimationBase.cs
- MailMessage.cs
- MultiBinding.cs
- DescendantQuery.cs
- Part.cs
- ProviderSettingsCollection.cs
- ContractSearchPattern.cs
- MouseGestureValueSerializer.cs
- PathFigure.cs
- XmlChildEnumerator.cs
- DispatchChannelSink.cs
- TextBoxLine.cs
- SkewTransform.cs
- BitmapEffectInput.cs
- PenCursorManager.cs
- CmsUtils.cs
- ComponentManagerBroker.cs
- TimelineClockCollection.cs
- ButtonBase.cs
- DisplayNameAttribute.cs
- UpdateTranslator.cs
- DataServiceStreamProviderWrapper.cs
- DataObjectSettingDataEventArgs.cs
- CodeDirectiveCollection.cs
- FocusChangedEventArgs.cs
- DrawingImage.cs
- XmlSchemaAny.cs
- ChangeTracker.cs
- PieceDirectory.cs
- PenContexts.cs
- HtmlDocument.cs
- ConditionalAttribute.cs
- EntityCommand.cs
- NotCondition.cs
- Image.cs
- MultiByteCodec.cs
- RegexRunnerFactory.cs
- CommandBinding.cs
- Timer.cs
- WebPartHeaderCloseVerb.cs
- EntityClientCacheEntry.cs
- BaseParagraph.cs
- XPathParser.cs
- StateDesigner.Layouts.cs
- StreamInfo.cs
- WebScriptMetadataFormatter.cs
- ProviderCollection.cs
- ScopelessEnumAttribute.cs
- WpfXamlType.cs
- TextParaClient.cs
- CellParagraph.cs
- InvalidCastException.cs
- AggregationMinMaxHelpers.cs