Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Linq / Parallel / Enumerables / AggregationMinMaxHelpers.cs / 1305376 / AggregationMinMaxHelpers.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // AggregationMinMaxHelpers.cs // //[....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Collections.Generic; using System.Linq.Parallel; using System.Diagnostics.Contracts; namespace System.Linq { internal static class AggregationMinMaxHelpers{ //------------------------------------------------------------------------------------ // Helper method to find the minimum or maximum element in the source. // private static T Reduce(IEnumerable source, int sign) { Contract.Assert(source != null); Contract.Assert(sign == -1 || sign == 1); Func , T, Pair > intermediateReduce = MakeIntermediateReduceFunction(sign); Func , Pair , Pair > finalReduce = MakeFinalReduceFunction(sign); Func , T> resultSelector = MakeResultSelectorFunction(); AssociativeAggregationOperator , T> aggregation = new AssociativeAggregationOperator , T>(source, new Pair (false, default(T)), null, true, intermediateReduce, finalReduce, resultSelector, default(T) != null, QueryAggregationOptions.AssociativeCommutative); return aggregation.Aggregate(); } //----------------------------------------------------------------------------------- // Helper method to find the minimum element in the source. // internal static T ReduceMin(IEnumerable source) { return Reduce(source, -1); } //----------------------------------------------------------------------------------- // Helper method to find the maximum element in the source. // internal static T ReduceMax(IEnumerable source) { return Reduce(source, 1); } //----------------------------------------------------------------------------------- // These methods are used to generate delegates to perform the comparisons. // private static Func , T, Pair > MakeIntermediateReduceFunction(int sign) { Comparer comparer = Util.GetDefaultComparer (); // Note that we capture the 'sign' argument and 'comparer' local, and therefore the C# // compiler will transform this into an instance-based delegate, incurring an extra (hidden) // object allocation. return delegate(Pair accumulator, T element) { // If this is the first element, or the sign of the result of comparing the element with // the existing accumulated result is equal to the sign requested by the function factory, // we will return a new pair that contains the current element as the best item. We will // ignore null elements (for reference and nullable types) in the input stream. if ((default(T) != null || element != null) && (!accumulator.First || Util.Sign(comparer.Compare(element, accumulator.Second)) == sign)) { return new Pair (true, element); } // Otherwise, just return the current accumulator result. return accumulator; }; } private static Func , Pair , Pair > MakeFinalReduceFunction(int sign) { Comparer comparer = Util.GetDefaultComparer (); // Note that we capture the 'sign' argument and 'comparer' local, and therefore the C# // compiler will transform this into an instance-based delegate, incurring an extra (hidden) // object allocation. return delegate(Pair accumulator, Pair element) { // If the intermediate reduction is empty, we will ignore it. Otherwise, if this is the // first element, or the sign of the result of comparing the element with the existing // accumulated result is equal to the sign requested by the function factory, we will // return a new pair that contains the current element as the best item. if (element.First && (!accumulator.First || Util.Sign(comparer.Compare(element.Second, accumulator.Second)) == sign)) { Contract.Assert(default(T) != null || element.Second != null, "nulls unexpected in final reduce"); return new Pair (true, element.Second); } // Otherwise, just return the current accumulator result. return accumulator; }; } private static Func , T> MakeResultSelectorFunction() { // If we saw at least one element in the source stream, the right pair element will contain // the element we're looking for -- so we return that. In the case of non-nullable value // types, the aggregation API will have thrown an exception before calling us for // empty sequences. Else, we will just return the element, which may be null for other types. return delegate(Pair accumulator) { Contract.Assert(accumulator.First || default(T) == null, "for non-null types we expect an exception to be thrown before getting here"); return accumulator.Second; }; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
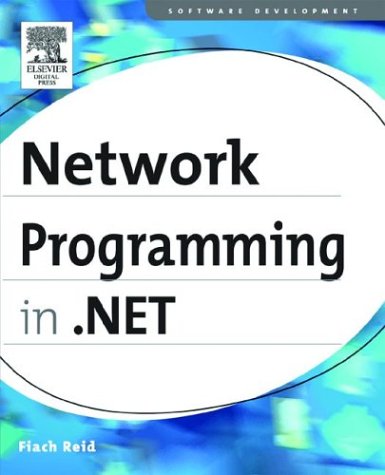
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HttpCachePolicy.cs
- BaseDataListDesigner.cs
- ObjectItemLoadingSessionData.cs
- LogSwitch.cs
- IResourceProvider.cs
- CursorInteropHelper.cs
- ReflectionPermission.cs
- ManifestSignatureInformation.cs
- RegexCompiler.cs
- CompilationSection.cs
- TerminatorSinks.cs
- AttachmentService.cs
- TreeNodeMouseHoverEvent.cs
- StringPropertyBuilder.cs
- SiteMapNodeItemEventArgs.cs
- FormViewUpdateEventArgs.cs
- XmlSchemaObjectCollection.cs
- LeafCellTreeNode.cs
- SqlRecordBuffer.cs
- DesignerView.cs
- BamlResourceSerializer.cs
- AlignmentYValidation.cs
- WindowShowOrOpenTracker.cs
- Baml2006KnownTypes.cs
- BitVector32.cs
- Html32TextWriter.cs
- SystemIPv6InterfaceProperties.cs
- ToolStripContentPanelRenderEventArgs.cs
- ToolboxDataAttribute.cs
- FocusTracker.cs
- CodeStatement.cs
- DataBinder.cs
- MethodCallTranslator.cs
- ScrollableControl.cs
- UnaryNode.cs
- InputLangChangeRequestEvent.cs
- VirtualPathUtility.cs
- CodeSnippetCompileUnit.cs
- MediaElementAutomationPeer.cs
- QueryableDataSourceEditData.cs
- UnsafeMethods.cs
- DES.cs
- SoapIncludeAttribute.cs
- peernodeimplementation.cs
- PerformanceCountersElement.cs
- Compiler.cs
- EventListener.cs
- WinEventQueueItem.cs
- BindableTemplateBuilder.cs
- ColumnResizeAdorner.cs
- CollectionChangedEventManager.cs
- WebPartZoneBase.cs
- ObfuscationAttribute.cs
- ValidatingReaderNodeData.cs
- RecordsAffectedEventArgs.cs
- Console.cs
- XmlComplianceUtil.cs
- Pair.cs
- HttpCookiesSection.cs
- _CookieModule.cs
- BreakRecordTable.cs
- HttpModuleActionCollection.cs
- ProfilePropertyNameValidator.cs
- DataSourceView.cs
- ImageProxy.cs
- MatrixUtil.cs
- XPathParser.cs
- TextTreeTextNode.cs
- PropertyChange.cs
- Function.cs
- PhysicalOps.cs
- RunInstallerAttribute.cs
- NetSectionGroup.cs
- FloatAverageAggregationOperator.cs
- BitmapEffectrendercontext.cs
- Row.cs
- DragDeltaEventArgs.cs
- initElementDictionary.cs
- PerfCounterSection.cs
- storepermission.cs
- CompiledXpathExpr.cs
- COM2IDispatchConverter.cs
- RequestNavigateEventArgs.cs
- GetMemberBinder.cs
- StreamWithDictionary.cs
- XPathDescendantIterator.cs
- SQLGuidStorage.cs
- SharedDp.cs
- RoleService.cs
- ClientRuntime.cs
- CodeIndexerExpression.cs
- HtmlTableRow.cs
- ConnectionManagementSection.cs
- WinFormsComponentEditor.cs
- FunctionGenerator.cs
- AppSettingsReader.cs
- SmiRecordBuffer.cs
- UnhandledExceptionEventArgs.cs
- AuthenticationService.cs
- TemplateXamlTreeBuilder.cs