Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / Data / System / Data / Filter / UnaryNode.cs / 1 / UnaryNode.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data { using System; using System.Collections.Generic; using System.Diagnostics; using System.Data.Common; using System.Data.SqlTypes; internal sealed class UnaryNode : ExpressionNode { internal readonly int op; internal ExpressionNode right; internal UnaryNode(DataTable table, int op, ExpressionNode right) : base(table) { this.op = op; this.right = right; } internal override void Bind(DataTable table, Listlist) { BindTable(table); right.Bind(table, list); } internal override object Eval() { return Eval(null, DataRowVersion.Default); } internal override object Eval(DataRow row, DataRowVersion version) { return EvalUnaryOp(op, right.Eval(row, version)); } internal override object Eval(int[] recordNos) { return right.Eval(recordNos); } private object EvalUnaryOp(int op, object vl) { object value = DBNull.Value; if (DataExpression.IsUnknown(vl)) return DBNull.Value; StorageType storageType; switch (op) { case Operators.Noop: return vl; case Operators.UnaryPlus: storageType = DataStorage.GetStorageType(vl.GetType()); if (ExpressionNode.IsNumericSql(storageType)) { return vl; } throw ExprException.TypeMismatch(this.ToString()); case Operators.Negative: // the have to be better way for doing this.. storageType = DataStorage.GetStorageType(vl.GetType()); if (ExpressionNode.IsNumericSql(storageType)) { switch(storageType) { case StorageType.Byte: value = -(Byte) vl; break; case StorageType.Int16: value = -(Int16) vl; break; case StorageType.Int32: value = -(Int32) vl; break; case StorageType.Int64: value = -(Int64) vl; break; case StorageType.Single: value = -(Single) vl; break; case StorageType.Double: value = -(Double) vl; break; case StorageType.Decimal: value = -(Decimal) vl; break; case StorageType.SqlDecimal: value = -(SqlDecimal) vl; break; case StorageType.SqlDouble: value = -(SqlDouble) vl; break; case StorageType.SqlSingle: value = -(SqlSingle) vl; break; case StorageType.SqlMoney: value = -(SqlMoney) vl; break; case StorageType.SqlInt64: value = -(SqlInt64) vl; break; case StorageType.SqlInt32: value = -(SqlInt32) vl; break; case StorageType.SqlInt16: value = -(SqlInt16) vl; break; default: Debug.Assert(false, "Missing a type conversion"); value = DBNull.Value; break; } return value; } throw ExprException.TypeMismatch(this.ToString()); case Operators.Not: if (vl is SqlBoolean){ if (((SqlBoolean)vl).IsFalse){ return SqlBoolean.True; } else if (((SqlBoolean)vl).IsTrue) { return SqlBoolean.False; } throw ExprException.UnsupportedOperator(op); // or should the result of not SQLNull be SqlNull ? } else{ if (DataExpression.ToBoolean(vl) != false) return false; return true; } default: throw ExprException.UnsupportedOperator(op); } } internal override bool IsConstant() { return(right.IsConstant()); } internal override bool IsTableConstant() { return(right.IsTableConstant()); } internal override bool HasLocalAggregate() { return(right.HasLocalAggregate()); } internal override bool HasRemoteAggregate() { return(right.HasRemoteAggregate()); } internal override bool DependsOn(DataColumn column) { return(right.DependsOn(column)); } internal override ExpressionNode Optimize() { right = right.Optimize(); if (this.IsConstant()) { object val = this.Eval(); return new ConstNode(table, ValueType.Object, val, false); } else return this; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data { using System; using System.Collections.Generic; using System.Diagnostics; using System.Data.Common; using System.Data.SqlTypes; internal sealed class UnaryNode : ExpressionNode { internal readonly int op; internal ExpressionNode right; internal UnaryNode(DataTable table, int op, ExpressionNode right) : base(table) { this.op = op; this.right = right; } internal override void Bind(DataTable table, Listlist) { BindTable(table); right.Bind(table, list); } internal override object Eval() { return Eval(null, DataRowVersion.Default); } internal override object Eval(DataRow row, DataRowVersion version) { return EvalUnaryOp(op, right.Eval(row, version)); } internal override object Eval(int[] recordNos) { return right.Eval(recordNos); } private object EvalUnaryOp(int op, object vl) { object value = DBNull.Value; if (DataExpression.IsUnknown(vl)) return DBNull.Value; StorageType storageType; switch (op) { case Operators.Noop: return vl; case Operators.UnaryPlus: storageType = DataStorage.GetStorageType(vl.GetType()); if (ExpressionNode.IsNumericSql(storageType)) { return vl; } throw ExprException.TypeMismatch(this.ToString()); case Operators.Negative: // the have to be better way for doing this.. storageType = DataStorage.GetStorageType(vl.GetType()); if (ExpressionNode.IsNumericSql(storageType)) { switch(storageType) { case StorageType.Byte: value = -(Byte) vl; break; case StorageType.Int16: value = -(Int16) vl; break; case StorageType.Int32: value = -(Int32) vl; break; case StorageType.Int64: value = -(Int64) vl; break; case StorageType.Single: value = -(Single) vl; break; case StorageType.Double: value = -(Double) vl; break; case StorageType.Decimal: value = -(Decimal) vl; break; case StorageType.SqlDecimal: value = -(SqlDecimal) vl; break; case StorageType.SqlDouble: value = -(SqlDouble) vl; break; case StorageType.SqlSingle: value = -(SqlSingle) vl; break; case StorageType.SqlMoney: value = -(SqlMoney) vl; break; case StorageType.SqlInt64: value = -(SqlInt64) vl; break; case StorageType.SqlInt32: value = -(SqlInt32) vl; break; case StorageType.SqlInt16: value = -(SqlInt16) vl; break; default: Debug.Assert(false, "Missing a type conversion"); value = DBNull.Value; break; } return value; } throw ExprException.TypeMismatch(this.ToString()); case Operators.Not: if (vl is SqlBoolean){ if (((SqlBoolean)vl).IsFalse){ return SqlBoolean.True; } else if (((SqlBoolean)vl).IsTrue) { return SqlBoolean.False; } throw ExprException.UnsupportedOperator(op); // or should the result of not SQLNull be SqlNull ? } else{ if (DataExpression.ToBoolean(vl) != false) return false; return true; } default: throw ExprException.UnsupportedOperator(op); } } internal override bool IsConstant() { return(right.IsConstant()); } internal override bool IsTableConstant() { return(right.IsTableConstant()); } internal override bool HasLocalAggregate() { return(right.HasLocalAggregate()); } internal override bool HasRemoteAggregate() { return(right.HasRemoteAggregate()); } internal override bool DependsOn(DataColumn column) { return(right.DependsOn(column)); } internal override ExpressionNode Optimize() { right = right.Optimize(); if (this.IsConstant()) { object val = this.Eval(); return new ConstNode(table, ValueType.Object, val, false); } else return this; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
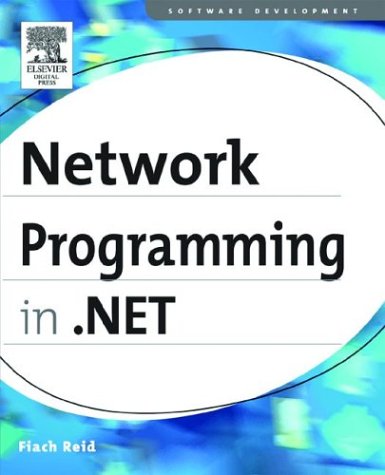
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ConfigurationValidatorAttribute.cs
- StoreItemCollection.Loader.cs
- SiteMapNodeCollection.cs
- WsdlImporterElement.cs
- EnumValAlphaComparer.cs
- InternalResources.cs
- BrowserDefinition.cs
- UnsignedPublishLicense.cs
- Control.cs
- ConfigXmlElement.cs
- ProcessModelInfo.cs
- Merger.cs
- RefreshEventArgs.cs
- CoTaskMemSafeHandle.cs
- Color.cs
- SoundPlayerAction.cs
- OleStrCAMarshaler.cs
- FunctionQuery.cs
- NativeCppClassAttribute.cs
- SectionVisual.cs
- InvalidDocumentContentsException.cs
- SQLCharsStorage.cs
- dsa.cs
- HttpServerChannel.cs
- CodeBinaryOperatorExpression.cs
- dtdvalidator.cs
- RegexStringValidatorAttribute.cs
- CustomSignedXml.cs
- XmlSchemaAny.cs
- BindingMemberInfo.cs
- GestureRecognitionResult.cs
- TextBox.cs
- GradientStop.cs
- ArrayMergeHelper.cs
- IntSecurity.cs
- TransformPatternIdentifiers.cs
- XmlWhitespace.cs
- ColorContext.cs
- RightsManagementEncryptedStream.cs
- PersonalizationProvider.cs
- MutexSecurity.cs
- XsltCompileContext.cs
- ProfileInfo.cs
- VoiceSynthesis.cs
- HyperLinkDataBindingHandler.cs
- UndoEngine.cs
- WeakReference.cs
- Color.cs
- ScriptReferenceEventArgs.cs
- PerfService.cs
- CodeDirectiveCollection.cs
- PropertyConverter.cs
- HttpCapabilitiesSectionHandler.cs
- RowVisual.cs
- DataGridViewLinkCell.cs
- HtmlInputButton.cs
- PersonalizationStateInfoCollection.cs
- CacheRequest.cs
- ISAPIWorkerRequest.cs
- CheckBoxField.cs
- ModelItemDictionary.cs
- NonSerializedAttribute.cs
- DefaultProfileManager.cs
- mda.cs
- SocketElement.cs
- CompositeScriptReferenceEventArgs.cs
- UnsafeNativeMethods.cs
- RadioButton.cs
- SystemWebSectionGroup.cs
- FontFamilyIdentifier.cs
- TraceContextRecord.cs
- ItemsPanelTemplate.cs
- WebControlAdapter.cs
- CodeAccessPermission.cs
- GenericIdentity.cs
- COM2PropertyDescriptor.cs
- FacetDescription.cs
- IDQuery.cs
- RequestQueue.cs
- ZipIOCentralDirectoryFileHeader.cs
- Transaction.cs
- ProcessHostMapPath.cs
- ITextView.cs
- Char.cs
- PropertyChangedEventManager.cs
- CacheAxisQuery.cs
- ProfilePropertyNameValidator.cs
- MemberMaps.cs
- DispatcherOperation.cs
- LayoutEditorPart.cs
- ArraySegment.cs
- XmlSerializationWriter.cs
- OleDbError.cs
- UIElement.cs
- MethodSignatureGenerator.cs
- UserNameSecurityToken.cs
- PropertyOverridesDialog.cs
- WebZone.cs
- SelectingProviderEventArgs.cs
- MemberDomainMap.cs