Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / xsp / System / Web / UI / HtmlControls / HtmlInputButton.cs / 1 / HtmlInputButton.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * HtmlInputButton.cs * * Copyright (c) 2000 Microsoft Corporation */ namespace System.Web.UI.HtmlControls { using System; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.Web; using System.Web.UI; using System.Globalization; using System.Security.Permissions; ////// [ DefaultEvent("ServerClick"), SupportsEventValidation, ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class HtmlInputButton : HtmlInputControl, IPostBackEventHandler { private static readonly object EventServerClick = new object(); /* * Creates an intrinsic Html INPUT type=button control. */ ////// The ///class defines the methods, /// properties, and events for the HTML Input Button control. This class allows /// programmatic access to the HTML <input type= /// button>, <input type= /// submit>,and <input /// type= /// reset> elements on /// the server. /// /// public HtmlInputButton() : base("button") { } /* * Creates an intrinsic Html INPUT type=button,submit,reset control. */ ///Initializes a new instance of a ///class using /// default values. /// public HtmlInputButton(string type) : base(type) { } ///Initializes a new instance of a ///class using the /// specified string. /// [ WebCategory("Behavior"), DefaultValue(true), ] public virtual bool CausesValidation { get { object b = ViewState["CausesValidation"]; return((b == null) ? true : (bool)b); } set { ViewState["CausesValidation"] = value; } } [ WebCategory("Behavior"), DefaultValue(""), WebSysDescription(SR.PostBackControl_ValidationGroup) ] public virtual string ValidationGroup { get { string s = (string)ViewState["ValidationGroup"]; return((s == null) ? String.Empty : s); } set { ViewState["ValidationGroup"] = value; } } ///Gets or sets whether pressing the button causes page validation to fire. This defaults to True so that when /// using validation controls, the validation state of all controls are updated when the button is clicked, both /// on the client and the server. Setting this to False is useful when defining a cancel or reset button on a page /// that has validators. ////// [ WebCategory("Action"), WebSysDescription(SR.HtmlControl_OnServerClick) ] public event EventHandler ServerClick { add { Events.AddHandler(EventServerClick, value); } remove { Events.RemoveHandler(EventServerClick, value); } } ////// Occurs when an HTML Input Button control is clicked on the browser. /// ///protected internal override void OnPreRender(EventArgs e) { base.OnPreRender(e); if (Page != null && Events[EventServerClick] != null) { Page.RegisterPostBackScript(); } } /* * Override to generate postback code for onclick. */ /// /// /// protected override void RenderAttributes(HtmlTextWriter writer) { RenderAttributesInternal(writer); base.RenderAttributes(writer); // this must come last because of the self-closing / } // VSWhidbey 80882: It needs to be overridden by HtmlInputSubmit internal virtual void RenderAttributesInternal(HtmlTextWriter writer) { bool submitsProgramatically = Events[EventServerClick] != null; if (Page != null) { if (submitsProgramatically) { Util.WriteOnClickAttribute( writer, this, false /* submitsAutomatically */, submitsProgramatically, (CausesValidation && Page.GetValidators(ValidationGroup).Count > 0), ValidationGroup); } else { Page.ClientScript.RegisterForEventValidation(UniqueID); } } } ////// protected virtual void OnServerClick(EventArgs e) { EventHandler handler = (EventHandler)Events[EventServerClick]; if (handler != null) handler(this, e); } /* * Method of IPostBackEventHandler interface to raise events on post back. * Button fires an OnServerClick event. */ ///Raises the ///event. /// /// void IPostBackEventHandler.RaisePostBackEvent(string eventArgument) { RaisePostBackEvent(eventArgument); } ////// /// protected virtual void RaisePostBackEvent(string eventArgument) { ValidateEvent(UniqueID, eventArgument); if (CausesValidation) { Page.Validate(ValidationGroup); } OnServerClick(EventArgs.Empty); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * HtmlInputButton.cs * * Copyright (c) 2000 Microsoft Corporation */ namespace System.Web.UI.HtmlControls { using System; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.Web; using System.Web.UI; using System.Globalization; using System.Security.Permissions; ////// [ DefaultEvent("ServerClick"), SupportsEventValidation, ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class HtmlInputButton : HtmlInputControl, IPostBackEventHandler { private static readonly object EventServerClick = new object(); /* * Creates an intrinsic Html INPUT type=button control. */ ////// The ///class defines the methods, /// properties, and events for the HTML Input Button control. This class allows /// programmatic access to the HTML <input type= /// button>, <input type= /// submit>,and <input /// type= /// reset> elements on /// the server. /// /// public HtmlInputButton() : base("button") { } /* * Creates an intrinsic Html INPUT type=button,submit,reset control. */ ///Initializes a new instance of a ///class using /// default values. /// public HtmlInputButton(string type) : base(type) { } ///Initializes a new instance of a ///class using the /// specified string. /// [ WebCategory("Behavior"), DefaultValue(true), ] public virtual bool CausesValidation { get { object b = ViewState["CausesValidation"]; return((b == null) ? true : (bool)b); } set { ViewState["CausesValidation"] = value; } } [ WebCategory("Behavior"), DefaultValue(""), WebSysDescription(SR.PostBackControl_ValidationGroup) ] public virtual string ValidationGroup { get { string s = (string)ViewState["ValidationGroup"]; return((s == null) ? String.Empty : s); } set { ViewState["ValidationGroup"] = value; } } ///Gets or sets whether pressing the button causes page validation to fire. This defaults to True so that when /// using validation controls, the validation state of all controls are updated when the button is clicked, both /// on the client and the server. Setting this to False is useful when defining a cancel or reset button on a page /// that has validators. ////// [ WebCategory("Action"), WebSysDescription(SR.HtmlControl_OnServerClick) ] public event EventHandler ServerClick { add { Events.AddHandler(EventServerClick, value); } remove { Events.RemoveHandler(EventServerClick, value); } } ////// Occurs when an HTML Input Button control is clicked on the browser. /// ///protected internal override void OnPreRender(EventArgs e) { base.OnPreRender(e); if (Page != null && Events[EventServerClick] != null) { Page.RegisterPostBackScript(); } } /* * Override to generate postback code for onclick. */ /// /// /// protected override void RenderAttributes(HtmlTextWriter writer) { RenderAttributesInternal(writer); base.RenderAttributes(writer); // this must come last because of the self-closing / } // VSWhidbey 80882: It needs to be overridden by HtmlInputSubmit internal virtual void RenderAttributesInternal(HtmlTextWriter writer) { bool submitsProgramatically = Events[EventServerClick] != null; if (Page != null) { if (submitsProgramatically) { Util.WriteOnClickAttribute( writer, this, false /* submitsAutomatically */, submitsProgramatically, (CausesValidation && Page.GetValidators(ValidationGroup).Count > 0), ValidationGroup); } else { Page.ClientScript.RegisterForEventValidation(UniqueID); } } } ////// protected virtual void OnServerClick(EventArgs e) { EventHandler handler = (EventHandler)Events[EventServerClick]; if (handler != null) handler(this, e); } /* * Method of IPostBackEventHandler interface to raise events on post back. * Button fires an OnServerClick event. */ ///Raises the ///event. /// /// void IPostBackEventHandler.RaisePostBackEvent(string eventArgument) { RaisePostBackEvent(eventArgument); } ////// /// protected virtual void RaisePostBackEvent(string eventArgument) { ValidateEvent(UniqueID, eventArgument); if (CausesValidation) { Page.Validate(ValidationGroup); } OnServerClick(EventArgs.Empty); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
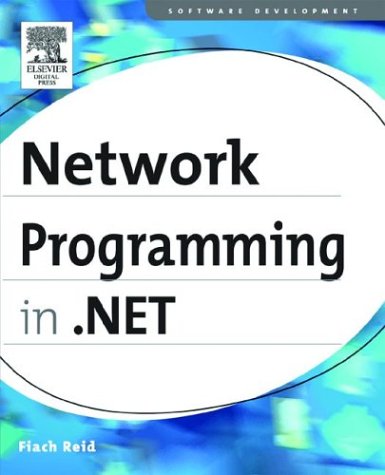
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PocoPropertyAccessorStrategy.cs
- PenThread.cs
- DataBindingHandlerAttribute.cs
- AsnEncodedData.cs
- GPPOINT.cs
- XmlSchemaComplexContentRestriction.cs
- CodeLabeledStatement.cs
- TemplatePagerField.cs
- ProxyGenerator.cs
- UserCancellationException.cs
- PolicyException.cs
- EntryWrittenEventArgs.cs
- IntegerValidatorAttribute.cs
- CodeAccessPermission.cs
- DataSourceSerializationException.cs
- XmlSchemaComplexContent.cs
- StickyNoteHelper.cs
- LogicalTreeHelper.cs
- SingleConverter.cs
- QilVisitor.cs
- Stylesheet.cs
- SettingsProperty.cs
- DbConnectionClosed.cs
- DSASignatureDeformatter.cs
- SchemaObjectWriter.cs
- DataGridTextBox.cs
- InfiniteTimeSpanConverter.cs
- VerificationAttribute.cs
- Matrix.cs
- WrappedIUnknown.cs
- RegisteredArrayDeclaration.cs
- TokenBasedSetEnumerator.cs
- SqlDataSourceCommandParser.cs
- DeviceContext2.cs
- EntityViewContainer.cs
- DescendantOverDescendantQuery.cs
- CompiledIdentityConstraint.cs
- WebResponse.cs
- MasterPageBuildProvider.cs
- Emitter.cs
- HotCommands.cs
- MatrixCamera.cs
- GridViewColumn.cs
- Rect3D.cs
- PassportAuthentication.cs
- DataTable.cs
- SchemaDeclBase.cs
- oledbmetadatacollectionnames.cs
- ObjectManager.cs
- Site.cs
- CodeAccessSecurityEngine.cs
- ActivityPropertyReference.cs
- Permission.cs
- OracleDataReader.cs
- SeekableReadStream.cs
- autovalidator.cs
- XmlILConstructAnalyzer.cs
- InvalidPrinterException.cs
- SBCSCodePageEncoding.cs
- EntityTypeEmitter.cs
- StylusPoint.cs
- ProfileSettings.cs
- PerfCounterSection.cs
- ScrollData.cs
- _SafeNetHandles.cs
- InvalidWMPVersionException.cs
- OleServicesContext.cs
- OutputCacheSettings.cs
- ResourceDisplayNameAttribute.cs
- HScrollProperties.cs
- OleDbParameter.cs
- LicenseManager.cs
- DesignerSerializationManager.cs
- _SpnDictionary.cs
- XmlWriterTraceListener.cs
- DLinqDataModelProvider.cs
- AutomationIdentifier.cs
- TextWriterTraceListener.cs
- TraceInternal.cs
- HtmlMeta.cs
- _TimerThread.cs
- PtsPage.cs
- RegistryKey.cs
- PersonalizationDictionary.cs
- CookieHandler.cs
- ChildChangedEventArgs.cs
- DataSourceViewSchemaConverter.cs
- WebPartMinimizeVerb.cs
- TemplateControlParser.cs
- TextComposition.cs
- TextModifierScope.cs
- MailHeaderInfo.cs
- DSASignatureDeformatter.cs
- FormsAuthenticationEventArgs.cs
- RequestBringIntoViewEventArgs.cs
- GridProviderWrapper.cs
- TableRow.cs
- MenuItemAutomationPeer.cs
- _LocalDataStoreMgr.cs
- WriteableBitmap.cs