Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Channels / RemoteEndpointMessageProperty.cs / 1 / RemoteEndpointMessageProperty.cs
//---------------------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------------------- namespace System.ServiceModel.Channels { using System; using System.Net; using System.Collections.Specialized; using System.ServiceModel.Activation; public sealed class RemoteEndpointMessageProperty { string address; int port; IPEndPoint remoteEndPoint; HostedRequestContainer hostedRequestContainer; InitializationState state; object thisLock = new object(); public RemoteEndpointMessageProperty(string address, int port) { if (string.IsNullOrEmpty(address)) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("address"); } if (port < IPEndPoint.MinPort || port > IPEndPoint.MaxPort) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument("port", SR.GetString(SR.ValueMustBeInRange, IPEndPoint.MinPort, IPEndPoint.MaxPort)); } this.port = port; this.address = address; this.state = InitializationState.All; } internal RemoteEndpointMessageProperty(HostedRequestContainer hostedRequestContainer) { this.hostedRequestContainer = hostedRequestContainer; } internal RemoteEndpointMessageProperty(IPEndPoint remoteEndPoint) { this.remoteEndPoint = remoteEndPoint; } public static string Name { get { return "System.ServiceModel.Channels.RemoteEndpointMessageProperty"; } } public string Address { get { if ((this.state & InitializationState.Address) != InitializationState.Address) { lock (ThisLock) { if ((this.state & InitializationState.Address) != InitializationState.Address) { Initialize(false); } } } return this.address; } } public int Port { get { if ((this.state & InitializationState.Port) != InitializationState.Port) { lock (ThisLock) { if ((this.state & InitializationState.Port) != InitializationState.Port) { Initialize(true); } } } return this.port; } } object ThisLock { get { return thisLock; } } void Initialize(bool getHostedPort) { if (remoteEndPoint != null) { this.address = remoteEndPoint.Address.ToString(); this.port = remoteEndPoint.Port; this.state = InitializationState.All; this.remoteEndPoint = null; } else { if ((this.state & InitializationState.Address) != InitializationState.Address) { this.address = hostedRequestContainer.GetRemoteAddress(); this.state |= InitializationState.Address; } if (getHostedPort) { this.port = hostedRequestContainer.GetRemotePort(); this.state |= InitializationState.Port; this.hostedRequestContainer = null; } } } [Flags] enum InitializationState { None = 0, Address = 1, Port = 2, All = 3 } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
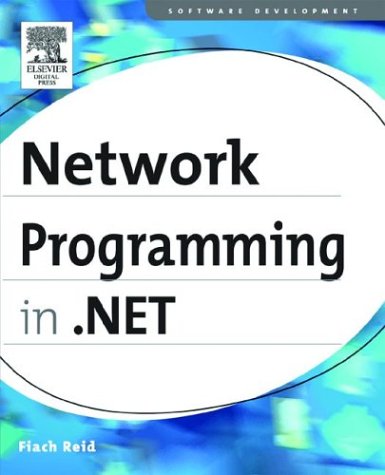
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CodeAssignStatement.cs
- MouseGestureValueSerializer.cs
- PathSegmentCollection.cs
- SqlUdtInfo.cs
- brushes.cs
- DomainUpDown.cs
- TypeConverterAttribute.cs
- RectConverter.cs
- ClientConvert.cs
- Operand.cs
- SqlFormatter.cs
- DefaultObjectMappingItemCollection.cs
- EdmToObjectNamespaceMap.cs
- ExceptionUtil.cs
- UpdatePanelControlTrigger.cs
- ObjectViewEntityCollectionData.cs
- PolicyValidationException.cs
- DiscoveryDocument.cs
- ConfigurationFileMap.cs
- PointAnimationUsingPath.cs
- SettingsContext.cs
- MailDefinition.cs
- CreateUserWizardAutoFormat.cs
- FactoryGenerator.cs
- DocumentGridContextMenu.cs
- MailMessageEventArgs.cs
- WindowsToolbarItemAsMenuItem.cs
- HwndSubclass.cs
- Query.cs
- altserialization.cs
- SettingsPropertyNotFoundException.cs
- CatalogZoneAutoFormat.cs
- ChannelFactoryBase.cs
- PlainXmlSerializer.cs
- Popup.cs
- ISO2022Encoding.cs
- DataGridHeadersVisibilityToVisibilityConverter.cs
- QuaternionAnimation.cs
- SqlCacheDependency.cs
- SafeNativeMethodsCLR.cs
- FormClosedEvent.cs
- ModelTreeEnumerator.cs
- ListItem.cs
- MobileControlsSectionHelper.cs
- ObjectViewQueryResultData.cs
- XPathNavigator.cs
- XmlComment.cs
- State.cs
- AsyncSerializedWorker.cs
- ByeMessageApril2005.cs
- PageCodeDomTreeGenerator.cs
- ConfigurationManagerInternalFactory.cs
- AppSecurityManager.cs
- Collection.cs
- MetadataArtifactLoaderComposite.cs
- ConsoleTraceListener.cs
- CompareInfo.cs
- SoapElementAttribute.cs
- cookieexception.cs
- BaseDataListPage.cs
- ConfigurationStrings.cs
- NavigatingCancelEventArgs.cs
- DocumentsTrace.cs
- XmlSchemaNotation.cs
- XsltArgumentList.cs
- CustomGrammar.cs
- TraceContextRecord.cs
- MultipleViewPatternIdentifiers.cs
- DetailsViewDeletedEventArgs.cs
- ActiveXHelper.cs
- ArraySet.cs
- DesignerView.cs
- Screen.cs
- TemplateContentLoader.cs
- TimelineCollection.cs
- JournalEntryListConverter.cs
- TextSelectionHighlightLayer.cs
- GroupBoxRenderer.cs
- EventData.cs
- KeyValuePairs.cs
- MsmqIntegrationBindingCollectionElement.cs
- EmbeddedObject.cs
- SafeFindHandle.cs
- RegionData.cs
- OrderedDictionary.cs
- ProviderSettingsCollection.cs
- WebDescriptionAttribute.cs
- SourceSwitch.cs
- RequestStatusBarUpdateEventArgs.cs
- log.cs
- GroupItemAutomationPeer.cs
- SecurityRuntime.cs
- FrameSecurityDescriptor.cs
- securitymgrsite.cs
- SecurityPermission.cs
- ArrayList.cs
- TreeViewItem.cs
- JournalEntryStack.cs
- WebCodeGenerator.cs
- XPathAxisIterator.cs