Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Channels / ChannelFactoryBase.cs / 1 / ChannelFactoryBase.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Channels { using System.Collections.Generic; using System.ServiceModel; using System.ServiceModel.Dispatcher; using System.Diagnostics; using System.IO; using System.Runtime.Serialization; using System.ServiceModel.Diagnostics; using System.Text; using System.Threading; public abstract class ChannelFactoryBase : ChannelManagerBase, IChannelFactory { TimeSpan closeTimeout = ServiceDefaults.CloseTimeout; TimeSpan openTimeout = ServiceDefaults.OpenTimeout; TimeSpan receiveTimeout = ServiceDefaults.ReceiveTimeout; TimeSpan sendTimeout = ServiceDefaults.SendTimeout; protected ChannelFactoryBase() { } protected ChannelFactoryBase(IDefaultCommunicationTimeouts timeouts) { this.InitializeTimeouts(timeouts); } protected override TimeSpan DefaultCloseTimeout { get { return this.closeTimeout; } } protected override TimeSpan DefaultOpenTimeout { get { return this.openTimeout; } } protected override TimeSpan DefaultReceiveTimeout { get { return this.receiveTimeout; } } protected override TimeSpan DefaultSendTimeout { get { return this.sendTimeout; } } public virtual T GetProperty() where T : class { if (typeof(T) == typeof(IChannelFactory)) { return (T)(object)this; } return default(T); } protected override void OnAbort() { } protected override IAsyncResult OnBeginClose(TimeSpan timeout, AsyncCallback callback, object state) { return new CompletedAsyncResult(callback, state); } protected override void OnClose(TimeSpan timeout) { } protected override void OnEndClose(IAsyncResult result) { CompletedAsyncResult.End(result); } void InitializeTimeouts(IDefaultCommunicationTimeouts timeouts) { if (timeouts != null) { this.closeTimeout = timeouts.CloseTimeout; this.openTimeout = timeouts.OpenTimeout; this.receiveTimeout = timeouts.ReceiveTimeout; this.sendTimeout = timeouts.SendTimeout; } } } public abstract class ChannelFactoryBase : ChannelFactoryBase, IChannelFactory { CommunicationObjectManager channels; protected ChannelFactoryBase() : this(null) { } protected ChannelFactoryBase(IDefaultCommunicationTimeouts timeouts) : base(timeouts) { this.channels = new CommunicationObjectManager (this.ThisLock); } public TChannel CreateChannel(EndpointAddress address) { if (address == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("address"); return this.InternalCreateChannel(address, address.Uri); } public TChannel CreateChannel(EndpointAddress address, Uri via) { if (address == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("address"); if (via == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("via"); return this.InternalCreateChannel(address, via); } TChannel InternalCreateChannel(EndpointAddress address, Uri via) { this.ValidateCreateChannel(); TChannel channel = this.OnCreateChannel(address, via); bool success = false; try { this.channels.Add((IChannel)(object)channel); success = true; } finally { if (!success) ((IChannel)(object)channel).Abort(); } return channel; } protected abstract TChannel OnCreateChannel(EndpointAddress address, Uri via); protected void ValidateCreateChannel() { ThrowIfDisposed(); if (this.State != CommunicationState.Opened) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR.GetString(SR.ChannelFactoryCannotBeUsedToCreateChannels, this.GetType().ToString()))); } } protected override void OnAbort() { IChannel[] currentChannels = this.channels.ToArray(); foreach (IChannel channel in currentChannels) channel.Abort(); this.channels.Abort(); } protected override void OnClose(TimeSpan timeout) { IChannel[] currentChannels = this.channels.ToArray(); TimeoutHelper timeoutHelper = new TimeoutHelper(timeout); foreach (IChannel channel in currentChannels) channel.Close(timeoutHelper.RemainingTime()); this.channels.Close(timeoutHelper.RemainingTime()); } protected override IAsyncResult OnBeginClose(TimeSpan timeout, AsyncCallback callback, object state) { return new ChainedCloseAsyncResult(timeout, callback, state, this.channels.BeginClose, this.channels.EndClose, this.channels.ToArray()); } protected override void OnEndClose(IAsyncResult result) { ChainedCloseAsyncResult.End(result); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
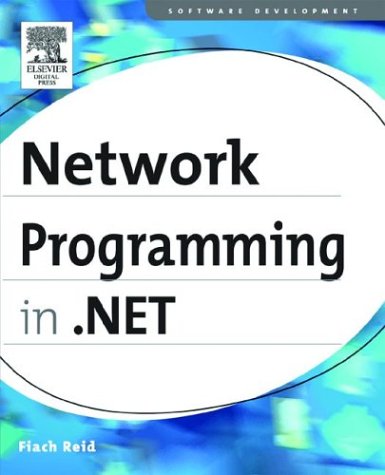
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FontDriver.cs
- WebResourceUtil.cs
- Predicate.cs
- AssociationTypeEmitter.cs
- CommandBindingCollection.cs
- DependencyPropertyKey.cs
- TextBoxView.cs
- NegotiateStream.cs
- ApplicationSecurityManager.cs
- RenderDataDrawingContext.cs
- DecimalAnimationBase.cs
- ToolboxItemCollection.cs
- AlternateView.cs
- DesignTimeResourceProviderFactoryAttribute.cs
- PersonalizableAttribute.cs
- Span.cs
- GridViewPageEventArgs.cs
- CompoundFileStreamReference.cs
- AdRotator.cs
- ArrayMergeHelper.cs
- CodeMemberField.cs
- ScriptingJsonSerializationSection.cs
- ModifierKeysConverter.cs
- ModelFactory.cs
- SiteMapProvider.cs
- ResourcesBuildProvider.cs
- Cursors.cs
- RenderData.cs
- Themes.cs
- StrokeCollection.cs
- TrackingLocationCollection.cs
- EntityKey.cs
- ExpressionConverter.cs
- ConfigurationManagerHelperFactory.cs
- PropertyGeneratedEventArgs.cs
- ResourceReferenceExpressionConverter.cs
- SubqueryRules.cs
- WebPartMenuStyle.cs
- cryptoapiTransform.cs
- PostBackOptions.cs
- webclient.cs
- ToolboxComponentsCreatingEventArgs.cs
- BlockingCollection.cs
- SynchronizationLockException.cs
- XPathParser.cs
- ColumnResizeAdorner.cs
- BinaryMessageEncoder.cs
- Int32CAMarshaler.cs
- IdleTimeoutMonitor.cs
- PanelStyle.cs
- CqlIdentifiers.cs
- XmlArrayItemAttributes.cs
- QueueSurrogate.cs
- ChtmlImageAdapter.cs
- BooleanAnimationUsingKeyFrames.cs
- EdmMember.cs
- regiisutil.cs
- Hash.cs
- FontUnitConverter.cs
- IFlowDocumentViewer.cs
- MultiView.cs
- XmlReaderSettings.cs
- StoreAnnotationsMap.cs
- GlyphCache.cs
- filewebresponse.cs
- DynamicPropertyHolder.cs
- WebPartCatalogAddVerb.cs
- ResponseStream.cs
- JsonFormatWriterGenerator.cs
- SelectionItemProviderWrapper.cs
- TopClause.cs
- RepeaterCommandEventArgs.cs
- XNodeValidator.cs
- ColumnReorderedEventArgs.cs
- SimpleApplicationHost.cs
- FunctionUpdateCommand.cs
- SevenBitStream.cs
- SponsorHelper.cs
- ExtendedProperty.cs
- AuthorizationSection.cs
- Screen.cs
- TdsParserStateObject.cs
- PolyBezierSegment.cs
- StorageRoot.cs
- ParserExtension.cs
- PageAction.cs
- EntityCommandExecutionException.cs
- InheritedPropertyChangedEventArgs.cs
- Vector3DIndependentAnimationStorage.cs
- EmptyCollection.cs
- IdentityReference.cs
- ConfigurationElementCollection.cs
- SafeMILHandleMemoryPressure.cs
- UIElementParaClient.cs
- TokenBasedSetEnumerator.cs
- List.cs
- MenuEventArgs.cs
- AutoResetEvent.cs
- CursorConverter.cs
- NativeMethods.cs