Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Base / MS / Internal / IO / Packaging / CompoundFile / CompoundFileStreamReference.cs / 1305600 / CompoundFileStreamReference.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // Definition of the CompoundFileStreamReference class. // // History: // 03/27/2002: BruceMac: Initial implementation. // 05/20/2003: RogerCh: Ported to WCP tree. // 08/11/2003: LGolding: Fix Bug 864168 (some of BruceMac's bug fixes were lost // in port to WCP tree). // //----------------------------------------------------------------------------- using System; using System.IO; using System.Text; // for StringBuilder using System.Windows; using MS.Internal.WindowsBase; namespace MS.Internal.IO.Packaging.CompoundFile { ////// Logical reference to a container stream /// ////// Use this class to represent a logical reference to a container stream, /// internal class CompoundFileStreamReference : CompoundFileReference, IComparable { //----------------------------------------------------- // // Public Properties // //----------------------------------------------------- ////// Full path from the root to this stream /// public override string FullName { get { return _fullName; } } //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Constructors ////// Use when you know the full path /// /// whack-delimited name including at least the stream name and optionally including preceding storage names public CompoundFileStreamReference(string fullName) { SetFullName(fullName); } ////// Full featured constructor /// /// optional string describing the storage name - may be null if stream exists in the root storage /// stream name ///streamName cannot be null or empty public CompoundFileStreamReference(string storageName, string streamName) { ContainerUtilities.CheckStringAgainstNullAndEmpty( streamName, "streamName" ); if ((storageName == null) || (storageName.Length == 0)) _fullName = streamName; else { // preallocate space for the stream we are building StringBuilder sb = new StringBuilder(storageName, storageName.Length + 1 + streamName.Length); sb.Append(ContainerUtilities.PathSeparator); sb.Append(streamName); _fullName = sb.ToString(); } } #endregion #region Operators ///Compare for equality /// the CompoundFileReference to compare to public override bool Equals(object o) { if (o == null) return false; // Standard behavior. // support subclassing - our subclasses can call us and do any additive work themselves if (o.GetType() != this.GetType()) return false; CompoundFileStreamReference r = (CompoundFileStreamReference)o; return (String.CompareOrdinal(_fullName.ToUpperInvariant(), r._fullName.ToUpperInvariant()) == 0); } ///Returns an integer suitable for including this object in a hash table public override int GetHashCode() { return _fullName.GetHashCode(); } #endregion #region IComparable ////// Compares two CompoundFileReferences /// /// CompoundFileReference to compare to this one ///Supports the IComparable interface ///Less than zero if this instance is less than the given reference, zero if they are equal /// and greater than zero if this instance is greater than the given reference. Not case sensitive. int IComparable.CompareTo(object o) { if (o == null) return 1; // Standard behavior. // different type? if (o.GetType() != GetType()) throw new ArgumentException( SR.Get(SRID.CanNotCompareDiffTypes)); CompoundFileStreamReference r = (CompoundFileStreamReference)o; return String.CompareOrdinal(_fullName.ToUpperInvariant(), r._fullName.ToUpperInvariant()); } #endregion //------------------------------------------------------ // // Private Methods // //------------------------------------------------------ ////// Initialize _fullName /// ///this should only be called from constructors as references are immutable /// string to parse ///if leading or trailing path delimiter private void SetFullName(string fullName) { ContainerUtilities.CheckStringAgainstNullAndEmpty(fullName, "fullName"); // fail on leading path separator to match functionality across the board // Although we need to do ToUpperInvariant before we do string comparison, in this case // it is not necessary since PathSeparatorAsString is a path symbol if (fullName.StartsWith(ContainerUtilities.PathSeparatorAsString, StringComparison.Ordinal)) throw new ArgumentException( SR.Get(SRID.DelimiterLeading), "fullName"); _fullName = fullName; string[] strings = ContainerUtilities.ConvertBackSlashPathToStringArrayPath(fullName); if (strings.Length == 0) throw new ArgumentException( SR.Get(SRID.CompoundFilePathNullEmpty), "fullName"); } //----------------------------------------------------- // // Private members // //------------------------------------------------------ // this can never be null - use String.Empty private String _fullName; // whack-path } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // Definition of the CompoundFileStreamReference class. // // History: // 03/27/2002: BruceMac: Initial implementation. // 05/20/2003: RogerCh: Ported to WCP tree. // 08/11/2003: LGolding: Fix Bug 864168 (some of BruceMac's bug fixes were lost // in port to WCP tree). // //----------------------------------------------------------------------------- using System; using System.IO; using System.Text; // for StringBuilder using System.Windows; using MS.Internal.WindowsBase; namespace MS.Internal.IO.Packaging.CompoundFile { ////// Logical reference to a container stream /// ////// Use this class to represent a logical reference to a container stream, /// internal class CompoundFileStreamReference : CompoundFileReference, IComparable { //----------------------------------------------------- // // Public Properties // //----------------------------------------------------- ////// Full path from the root to this stream /// public override string FullName { get { return _fullName; } } //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Constructors ////// Use when you know the full path /// /// whack-delimited name including at least the stream name and optionally including preceding storage names public CompoundFileStreamReference(string fullName) { SetFullName(fullName); } ////// Full featured constructor /// /// optional string describing the storage name - may be null if stream exists in the root storage /// stream name ///streamName cannot be null or empty public CompoundFileStreamReference(string storageName, string streamName) { ContainerUtilities.CheckStringAgainstNullAndEmpty( streamName, "streamName" ); if ((storageName == null) || (storageName.Length == 0)) _fullName = streamName; else { // preallocate space for the stream we are building StringBuilder sb = new StringBuilder(storageName, storageName.Length + 1 + streamName.Length); sb.Append(ContainerUtilities.PathSeparator); sb.Append(streamName); _fullName = sb.ToString(); } } #endregion #region Operators ///Compare for equality /// the CompoundFileReference to compare to public override bool Equals(object o) { if (o == null) return false; // Standard behavior. // support subclassing - our subclasses can call us and do any additive work themselves if (o.GetType() != this.GetType()) return false; CompoundFileStreamReference r = (CompoundFileStreamReference)o; return (String.CompareOrdinal(_fullName.ToUpperInvariant(), r._fullName.ToUpperInvariant()) == 0); } ///Returns an integer suitable for including this object in a hash table public override int GetHashCode() { return _fullName.GetHashCode(); } #endregion #region IComparable ////// Compares two CompoundFileReferences /// /// CompoundFileReference to compare to this one ///Supports the IComparable interface ///Less than zero if this instance is less than the given reference, zero if they are equal /// and greater than zero if this instance is greater than the given reference. Not case sensitive. int IComparable.CompareTo(object o) { if (o == null) return 1; // Standard behavior. // different type? if (o.GetType() != GetType()) throw new ArgumentException( SR.Get(SRID.CanNotCompareDiffTypes)); CompoundFileStreamReference r = (CompoundFileStreamReference)o; return String.CompareOrdinal(_fullName.ToUpperInvariant(), r._fullName.ToUpperInvariant()); } #endregion //------------------------------------------------------ // // Private Methods // //------------------------------------------------------ ////// Initialize _fullName /// ///this should only be called from constructors as references are immutable /// string to parse ///if leading or trailing path delimiter private void SetFullName(string fullName) { ContainerUtilities.CheckStringAgainstNullAndEmpty(fullName, "fullName"); // fail on leading path separator to match functionality across the board // Although we need to do ToUpperInvariant before we do string comparison, in this case // it is not necessary since PathSeparatorAsString is a path symbol if (fullName.StartsWith(ContainerUtilities.PathSeparatorAsString, StringComparison.Ordinal)) throw new ArgumentException( SR.Get(SRID.DelimiterLeading), "fullName"); _fullName = fullName; string[] strings = ContainerUtilities.ConvertBackSlashPathToStringArrayPath(fullName); if (strings.Length == 0) throw new ArgumentException( SR.Get(SRID.CompoundFilePathNullEmpty), "fullName"); } //----------------------------------------------------- // // Private members // //------------------------------------------------------ // this can never be null - use String.Empty private String _fullName; // whack-path } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
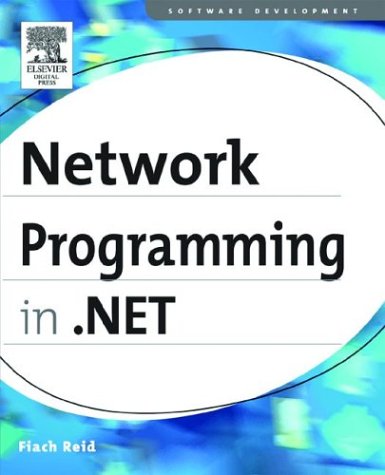
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AttachmentCollection.cs
- Permission.cs
- LocalFileSettingsProvider.cs
- DragDeltaEventArgs.cs
- XamlTreeBuilder.cs
- DrawListViewItemEventArgs.cs
- PartManifestEntry.cs
- _HeaderInfo.cs
- SizeLimitedCache.cs
- AutomationIdentifierGuids.cs
- CodeAttachEventStatement.cs
- DependsOnAttribute.cs
- Decimal.cs
- Figure.cs
- EncryptedKeyIdentifierClause.cs
- updateconfighost.cs
- TokenBasedSet.cs
- CryptoStream.cs
- SqlProfileProvider.cs
- Geometry.cs
- NameSpaceExtractor.cs
- DeferredElementTreeState.cs
- LoginName.cs
- SqlConnection.cs
- TextStore.cs
- DataSourceUtil.cs
- DrawingVisualDrawingContext.cs
- HashCoreRequest.cs
- NumberFormatInfo.cs
- ExtendLockCommand.cs
- APCustomTypeDescriptor.cs
- HTMLTagNameToTypeMapper.cs
- _PooledStream.cs
- CompiledQueryCacheKey.cs
- ListDictionary.cs
- GatewayDefinition.cs
- NativeMethods.cs
- AutoGeneratedFieldProperties.cs
- ThicknessAnimation.cs
- DataTableTypeConverter.cs
- XPathSelfQuery.cs
- ScrollProperties.cs
- LoadedEvent.cs
- ColorDialog.cs
- DoubleLink.cs
- WasHttpHandlersInstallComponent.cs
- HttpContext.cs
- GeometryGroup.cs
- IdentityManager.cs
- TextTreeTextElementNode.cs
- ApplicationDirectory.cs
- TraceEventCache.cs
- AuthenticationConfig.cs
- ArcSegment.cs
- RoutedPropertyChangedEventArgs.cs
- Grant.cs
- IOThreadTimer.cs
- RegistryExceptionHelper.cs
- SQLByteStorage.cs
- RenderData.cs
- ProfileProvider.cs
- WizardPanel.cs
- ToolStripOverflow.cs
- StylusPointDescription.cs
- RawMouseInputReport.cs
- XmlSerializerFaultFormatter.cs
- NodeInfo.cs
- Matrix3DValueSerializer.cs
- Crc32Helper.cs
- DataGridViewCellFormattingEventArgs.cs
- BooleanConverter.cs
- DocumentSchemaValidator.cs
- GraphicsState.cs
- Win32.cs
- PauseStoryboard.cs
- _Semaphore.cs
- UnmanagedHandle.cs
- TypeConverterHelper.cs
- DoubleAverageAggregationOperator.cs
- ListViewUpdatedEventArgs.cs
- ArglessEventHandlerProxy.cs
- ControlBuilder.cs
- SoapExtension.cs
- EnumDataContract.cs
- TagMapCollection.cs
- Empty.cs
- SafeFindHandle.cs
- CodeObject.cs
- AttributeCollection.cs
- compensatingcollection.cs
- Globals.cs
- TransformDescriptor.cs
- KnownColorTable.cs
- MD5CryptoServiceProvider.cs
- SerialPinChanges.cs
- StorageMappingItemCollection.cs
- TaskCanceledException.cs
- SerTrace.cs
- ShapingWorkspace.cs
- ListBindingConverter.cs